Custom Validation Annotations: Overcoming Common Pitfalls
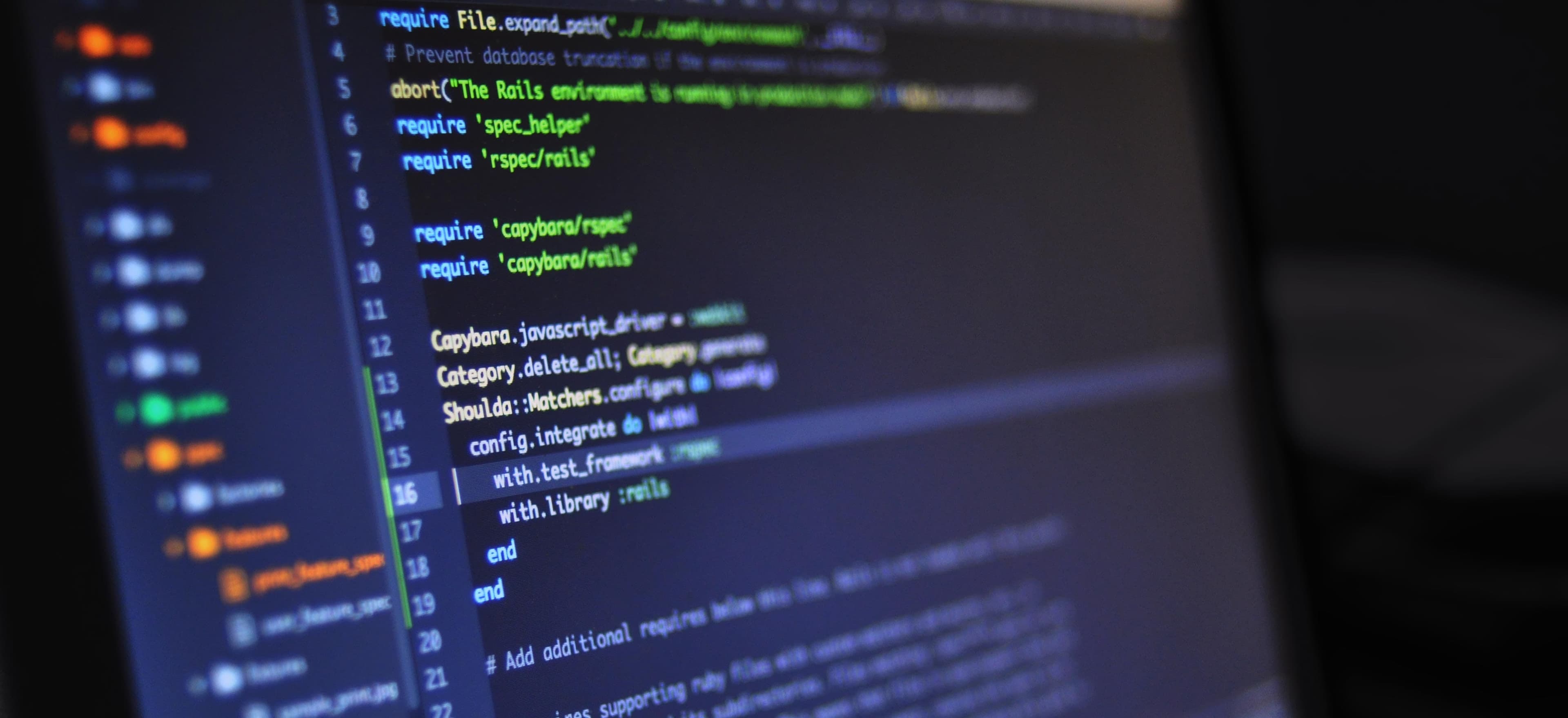
- Published on
Custom Validation Annotations: Overcoming Common Pitfalls
Validation is a cornerstone of software development, ensuring that data adheres to specified rules before it gets processed or stored. In Java, custom validation annotations offer a powerful way to enforce these rules within your applications. However, creating these custom annotations can come with its share of challenges. In this blog post, we will explore the common pitfalls when working with custom validation annotations and discuss how to overcome them with effective coding practices.
Understanding Custom Validation Annotations
What are Custom Validation Annotations?
Custom validation annotations in Java are user-defined annotations that annotate class fields, methods, or parameters to enforce rules on the data they represent. These annotations are part of the Bean Validation framework introduced in Java with the javax.validation
package.
Why Use Custom Validation Annotations?
- Reusability: Define validation logic once and reuse it across various classes.
- Improved Readability: Increase the clarity of validation rules by making them part of the data model.
- Maintainability: Changes can be made in one place, avoiding duplication and potential inconsistency.
Common Pitfalls When Creating Custom Validation Annotations
Despite the benefits, developers often encounter challenges. Let’s delve into these common pitfalls and their solutions.
1. Failing to Implement the ConstraintValidator
Interface Properly
Every custom validation annotation must have an accompanying validator class that implements the ConstraintValidator
interface. A common mistake is to neglect this requirement.
Example Solution
Here’s how you can create a custom annotation and an implement validator class:
import javax.validation.Constraint;
import javax.validation.Payload;
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
// Define the custom annotation
@Constraint(validatedBy = PhoneNumberValidator.class)
@Target({ ElementType.METHOD, ElementType.FIELD })
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidPhoneNumber {
String message() default "Invalid phone number"; // Default error message
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
// Implement the Validator
public class PhoneNumberValidator implements ConstraintValidator<ValidPhoneNumber, String> {
@Override
public boolean isValid(String phoneNumber, ConstraintValidatorContext context) {
// Null values are considered valid
if (phoneNumber == null) return true;
// Regex pattern for validating phone numbers
return phoneNumber.matches("^\\+?[0-9. ()-]{7,25}$");
}
}
Why This Matters
Implementing the ConstraintValidator
interface helps encapsulate the validation logic and ensures that your custom annotations work seamlessly with the validation framework.
2. Ignoring the Message Customization
When developing custom validation annotations, you need to consider how the error messages are conveyed to the end user. Often, developers overlook providing a user-friendly error message.
Crafting Meaningful Messages
In the @ValidPhoneNumber
annotation, we provided a default message. It is paramount to allow this message to be overridden as follows:
@ValidPhoneNumber(message = "Please enter a valid phone number.")
private String phoneNumber;
This practice enhances user experience by providing clear, actionable feedback.
3. Not Utilizing Validation Groups
Validation groups can be a game-changer. If your application has different validation needs for different scenarios, not using groups can lead to unexpected behavior.
Example Implementation
Here’s an example of how to use validation groups:
public interface FirstStage {}
public interface SecondStage {}
// Custom validation annotation
@Constraint(validatedBy = PhoneNumberValidator.class)
@Target({ ElementType.METHOD, ElementType.FIELD })
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidPhoneNumber {
String message() default "Invalid phone number";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
// Applying different groups
@ValidPhoneNumber(groups = FirstStage.class)
private String firstStagePhoneNumber;
@ValidPhoneNumber(groups = SecondStage.class)
private String secondStagePhoneNumber;
Why Groups Matter
By defining groups, you can specify validation logic that varies depending on the application's state, improving flexibility and control.
4. Failing to Test Custom Validators
One of the most overlooked aspects of creating custom validation annotations is comprehensive testing. Not adequately testing your validators can lead to undetected bugs.
Testing with JUnit
Here’s how to write a simple unit test for your validator:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class PhoneNumberValidatorTest {
private final PhoneNumberValidator validator = new PhoneNumberValidator();
@Test
public void testValidPhoneNumber() {
assertTrue(validator.isValid("+1234567890", null));
}
@Test
public void testInvalidPhoneNumber() {
assertFalse(validator.isValid("123abc", null));
}
}
Why Testing is Essential
Testing ensures that your custom annotation behaves as expected and allows you to catch any edge cases early in the development process.
5. Forgetting to Document the Annotations
Proper documentation is crucial. Neglecting to document how and when to use your annotations can confuse other developers down the line.
Best Documentation Practices
- Javadoc: Add clear descriptions to your annotations.
- Usage Examples: Include sample code demonstrating how to use your annotations.
Example of Documentation
/**
* Validates that the annotated phone number has a valid format.
* <p>
* The valid format includes numbers, optional country code, and specific lengths.
* </p>
*/
@Constraint(validatedBy = PhoneNumberValidator.class)
@Target({ ElementType.METHOD, ElementType.FIELD })
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidPhoneNumber {
...
}
The Closing Argument
Custom validation annotations in Java can greatly enhance the quality and maintainability of your code when used correctly. By being mindful of these common pitfalls and implementing the suggested solutions, you ensure a smoother development experience and a user-friendly application.
For more in-depth discussions on Java validation, check out the Java EE Validation documentation or visit the Hibernate Validator page, which is the reference implementation for Java Bean Validation.
By embracing best practices when creating custom validation annotations, you enhance your application's robustness and ensure it meets user expectations effectively. Happy coding!
Checkout our other articles