Mastering Java 9 Collections: Overcoming Static Factory Confusion
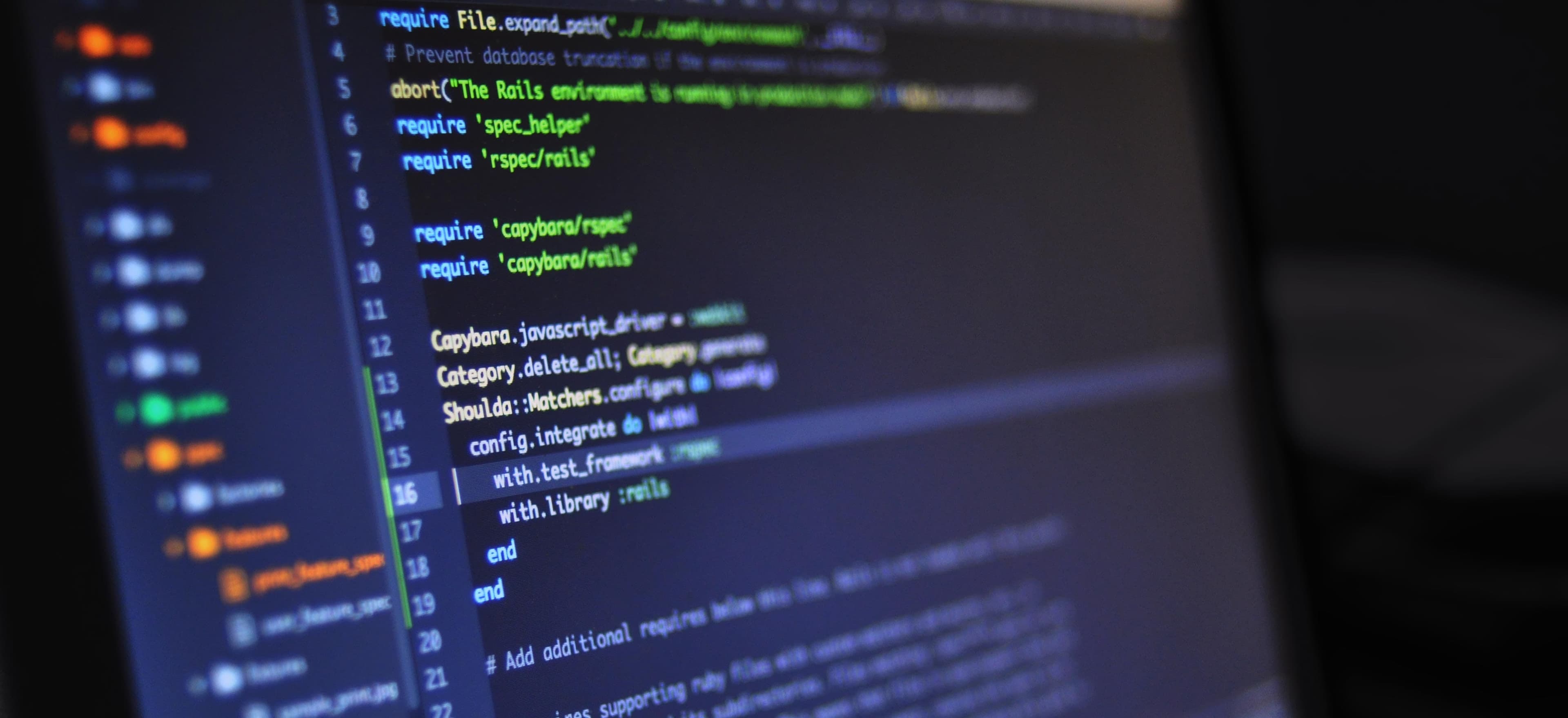
- Published on
Mastering Java 9 Collections: Overcoming Static Factory Confusion
Java 9 has brought us numerous enhancements in the realm of collections. With these updates, developers are often left scratching their heads over new functionalities, particularly the introduction of static factory methods. This blog post will offer an in-depth look into Java 9 collections while dispelling the confusion surrounding the new static factory methods.
Understanding Java Collections Framework
Before diving into the specifics, it’s essential to grasp what the Java Collections Framework (JCF) is. The JCF provides a unified architecture to store and manipulate collections, enabling developers to manage data in an efficient way.
Java collections can be broadly categorized into two types:
- Collection Interface: This is the root interface for the collection framework, representing groups of objects.
- Map Interface: This represents a mapping between key-value pairs, providing a way to associate data.
Java collections include interfaces, implementations (e.g., lists, sets, maps), and algorithms. Understanding the foundation of these components helps provide context for the changes brought about in Java 9.
The Power of Static Factory Methods
Static factory methods are a useful alternative to constructors. They allow for a more readable and flexible instantiation of objects. Prior to Java 9, collections could be created using constructors. However, the introduction of static factory methods in Java 9 has changed this dynamic somewhat.
Why Use Static Factory Methods?
Here are a few reasons to consider static factory methods:
- Clarity: They can give meaningful context to object creation.
- Controlled Instantiation: You can return existing instances or create new instances as needed.
- Type Stability: They can allow you to create a more flexible API for the consumer.
In Java 9, static factory methods were enhanced by providing additional methods for collections. This improvement allows for more concise and expressive code.
The Easier Path: New Static Factory Methods in Java 9
Java 9 introduced new factory methods in the List
, Set
, and Map
interfaces. Let's explore these methods in detail.
Creating a List
In Java 9, you can create an unmodifiable list using the factory method List.of()
. Here's how it works:
import java.util.List;
public class ListFactoryExample {
public static void main(String[] args) {
List<String> fruits = List.of("Apple", "Banana", "Orange");
// Printing the list
System.out.println(fruits);
// Example accessing elements
System.out.println("First fruit: " + fruits.get(0));
// Attempting to add an element will throw an UnsupportedOperationException
// fruits.add("Grapes"); // Uncommenting will throw an exception
}
}
Why Use List.of()
?
- Immutability: It creates an immutable list, ensuring that the contents cannot be modified after creation.
- Efficiency: Internally, it optimizes storage and avoids unnecessary array copying.
Creating a Set
Similarly, creating an unmodifiable set has been simplified with Set.of()
:
import java.util.Set;
public class SetFactoryExample {
public static void main(String[] args) {
Set<String> uniqueFruits = Set.of("Apple", "Banana", "Orange", "Apple");
// Printing the set
System.out.println(uniqueFruits);
// Attempting to add an element will throw an UnsupportedOperationException
// uniqueFruits.add("Grapes"); // Uncommenting will throw an exception
}
}
Why Use Set.of()
?
- Automatic Duplication Handling: If you try to add duplicate elements, it simply ignores them.
- Compact and Readable: Constructs sets with less code.
Creating a Map
Java 9 also introduced Map.of()
method for creating immutable maps:
import java.util.Map;
public class MapFactoryExample {
public static void main(String[] args) {
Map<String, Integer> fruitPrices = Map.of("Apple", 1, "Banana", 2, "Orange", 3);
// Printing the map
System.out.println(fruitPrices);
// Accessing a value using key
System.out.println("Price of Banana: $" + fruitPrices.get("Banana"));
// Attempting to modify will throw UnsupportedOperationException
// fruitPrices.put("Grapes", 4); // Uncommenting will throw an exception
}
}
Why Use Map.of()
?
- Key-Value Pair Addition: Easily create a map with a designated set of key-value pairs without needing a constructor.
- Immutability: Once created, maps cannot be modified.
Exception Handling
When working with Java collections, particularly using static factory methods, understanding exception handling is crucial. As demonstrated above, the newly formed collections are immutable and any mutation attempts will result in an UnsupportedOperationException
. This behavior guarantees that the collection's integrity is preserved.
Handling UnsupportedOperationException
To gracefully handle errors, you can wrap your code in a try-catch block:
try {
fruits.add("Grapes");
} catch (UnsupportedOperationException e) {
System.out.println("Cannot modify an immutable list: " + e.getMessage());
}
Practical Applications: Why Choose Static Factory Methods?
The static factory methods introduced in Java 9 streamline the collection creation process by eliminating redundancy, ensuring consistency, and promoting best practices.
Performance Optimization
Static factory methods can enhance performance. The runtime can cache immutable instances, leading to significant memory savings due to reduced object churn.
Code Readability
Using static factory methods makes code more readable. Instead of wrestling with varying constructor arguments, developers can use clear factory methods that communicate the intended use of the collection type directly.
Wrapping Up
Java 9 collections with static factory methods have revolutionized the way we create and manage collections. The clarity, performance optimization, and immutability afforded by these methods provide both seasoned and novice developers with enhanced tools to streamline their code.
As you transition to using static factory methods, consider how these can improve your coding practices and boost the maintainability of your projects.
For further reading on Java Collections, you can check the official Java documentation. Learning about these advancements can offer practical benefits, from code efficiency to reduced error rates.
Happy coding, and embrace the power of Java 9 collections!