Troubleshooting JPA Autodiscovery in Grails Applications
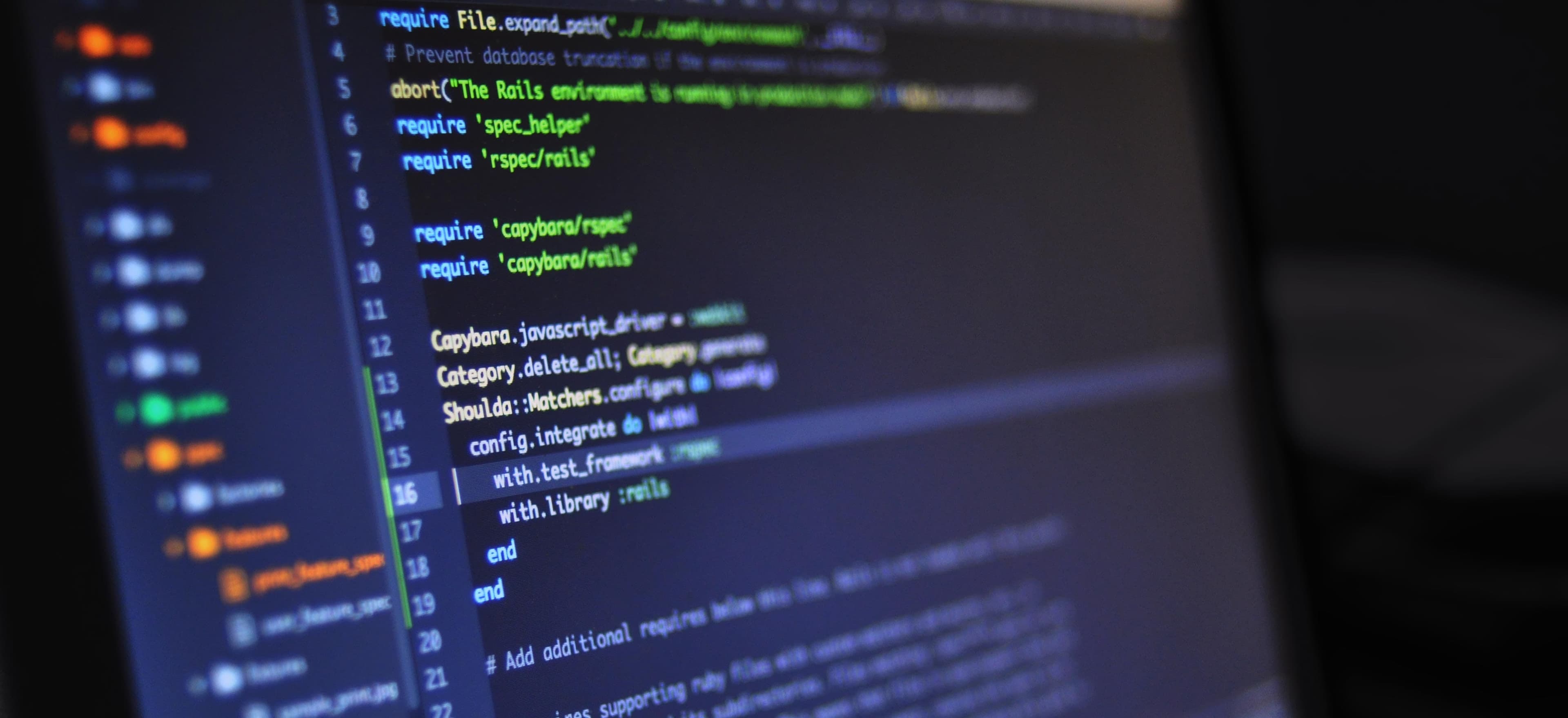
- Published on
Troubleshooting JPA Autodiscovery in Grails Applications
Grails is a powerful framework that simplifies web application development in Groovy and Java. One of its prominent features is the seamless integration with the Java Persistence API (JPA) for handling database interactions. However, issues may occasionally arise during JPA autodiscovery in Grails applications. This blog post will guide you through troubleshooting common pitfalls and optimizing your setup for hassle-free persistence management.
Understanding JPA Autodiscovery in Grails
Before diving into troubleshooting, it's essential to understand what JPA autodiscovery entails. Autodiscovery allows Grails to automatically configure the persistence layer, mapping domain classes to database tables without requiring extensive manual setup. This feature leverages Grails' convention-over-configuration paradigm, making it easier for developers to create data models.
Why JPA?
JPA is widely adopted in the Java ecosystem for object-relational mapping (ORM). It provides a higher-level abstraction than JDBC, allowing developers to work with Java objects while JPA handles relationships and SQL interactions under the hood. Using JPA in Grails facilitates:
- Code Simplicity: Reduces boilerplate code related to database operations.
- Flexibility: Easily switch between different databases.
- Annotations and Configuration: Utilize JPA annotations to customize mapping.
Common Issues in JPA Autodiscovery
Despite its advantages, JPA autodiscovery can lead to various issues. Here are several common problems developers may encounter:
- Missing Domain Classes
- Configuration Errors
- Classpath Issues
- Improper Annotations
- Unsupported Database Types
Let’s explore how to troubleshoot and resolve these problems effectively.
1. Missing Domain Classes
If your application isn't recognizing domain classes, the first step is to ensure that they are in the correct package. Grails typically scans the grails-app/domain
directory for domain classes.
Action Steps:
- Confirm that the domain classes are correctly placed inside the
grails-app/domain
directory. - Validate that your class names follow Grails naming conventions (for example, the name should be singular).
Example:
package com.example
class Book {
String title
String author
Date published
static constraints = {
title nullable: false, blank: false
author nullable: false
}
}
2. Configuration Errors
Grails requires specific configuration properties to properly set up JPA. Ensure that your application.yml
or application.groovy
file contains the necessary configurations.
Action Steps:
- Check for missing or incorrectly set configuration values. Here’s an example of configuration using H2 database:
dataSource:
pooled: true
driverClassName: org.h2.Driver
username: sa
password: ''
dbCreate: update
url: jdbc:h2:mem:testDb;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE
- Ensure that JPA properties are correctly defined if you're using Hibernate as the JPA provider.
3. Classpath Issues
Classpath issues can hinder Grails from locating your entity classes. It’s helpful to verify that all required dependencies are included in your build.gradle
.
Action Steps:
- Check if the necessary JPA dependencies exist.
dependencies {
compile "org.springframework.boot:spring-boot-starter-data-jpa"
compile "org.hibernate:hibernate-core"
compile "com.h2database:h2"
}
- Run
./gradlew cleanEclipse eclipse
or similar commands tailored to your environment to refresh your classpath.
4. Improper Annotations
JPA relies on specific annotations to define the relationships between entities. This entails using annotations like @Entity, @Table, @Id, and more. Missing or improperly configured annotations can disrupt autodiscovery.
Action Steps:
- Ensure you are using the
@Entity
annotation on your domain classes.
Example:
import javax.persistence.*
@Entity
@Table(name = "books")
class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
Long id
String title
String author
// Other fields...
}
- Review your relationships and ensure all associations are annotated correctly.
5. Unsupported Database Types
Not all database types are fully supported by JPA or Grails. Ensure that the database you are using is compatible with JPA.
Action Steps:
- Verify the compatibility of your database driver with JPA.
- If using a new or uncommon database, consult the documentation or community forums for known issues or solutions.
Useful Tools and Tips
Using Grails Console
The Grails console is a powerful tool that can help you troubleshoot JPA issues. By running commands like grails> domainClass(Book)
, you can check the status and mappings of your entity.
Logging
Enable SQL logging to see what queries Grails is generating behind the scenes. This can provide clarity on what’s happening when your application runs.
logging:
level:
org.hibernate.SQL: DEBUG
org.hibernate.type.descriptor.sql.BasicBinder: TRACE
Testing Your Configuration
Unit tests are a standard practice in Grails development. Writing tests for your domain classes ensures that they function as expected:
class BookSpec extends Specification {
void "test book creation"() {
when:
def book = new Book(title: "Grails in Action", author: "Manning")
then:
book.validate()
book.save(flush: true)
Book.count() == 1
}
}
The Closing Argument
Troubleshooting JPA autodiscovery in Grails applications may seem daunting at first. However, by following a systematic approach to identifying and resolving issues, you can ensure a smooth development experience. Remember to check domain class placements, validate configurations, use the correct annotations, and manage dependencies effectively.
For further reading, you can explore the official Grails documentation, which covers JPA and data access in detail. With these insights, you should be well-equipped to tackle any JPA-related challenges you encounter in your Grails projects.
Additional Resources
- JPA Documentation
- Spring Data JPA
- Grails User Guide
Feel free to reach out with issues you encounter, and happy coding!