Maximizing Hibernate Performance with Hawtio and Jolokia
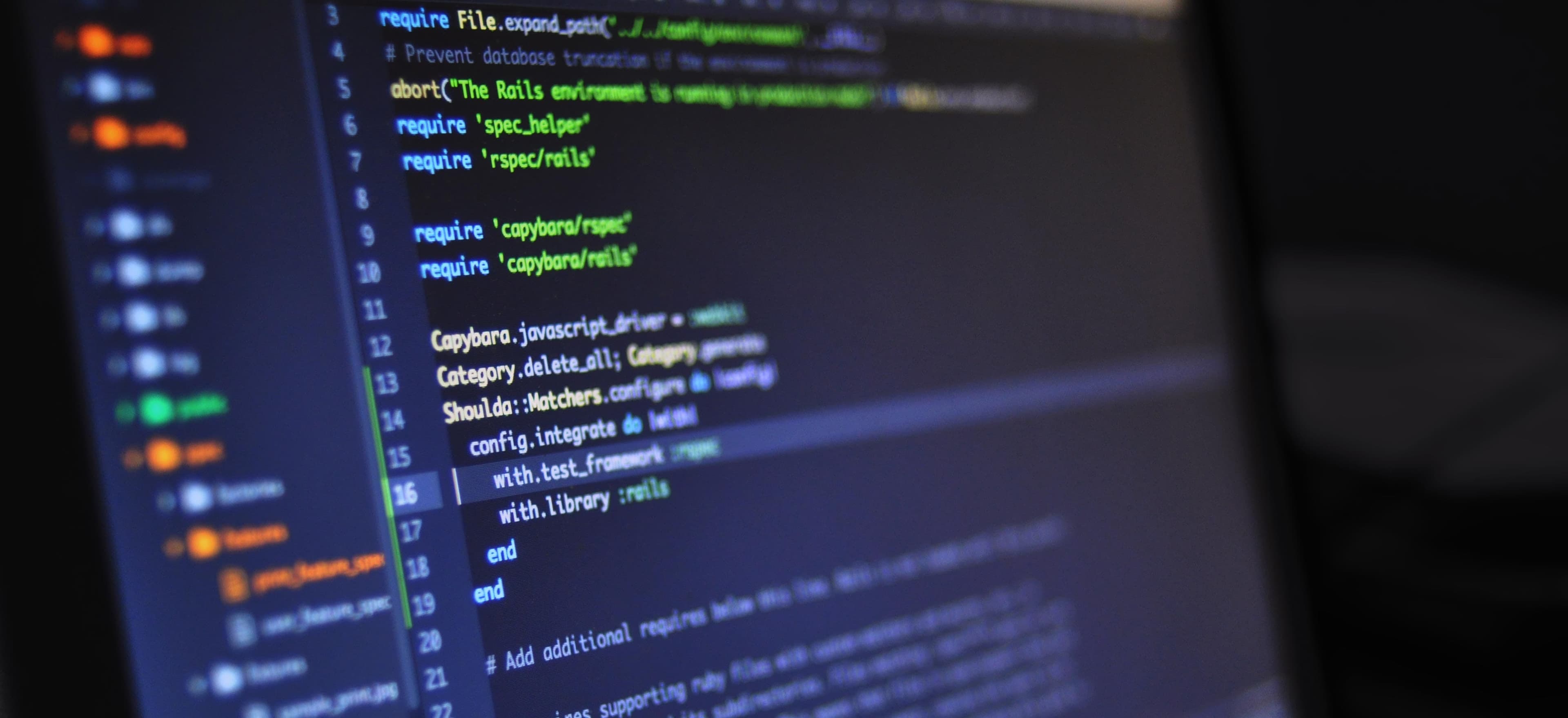
- Published on
Maximizing Hibernate Performance with Hawtio and Jolokia
Hibernate is a powerful ORM (Object-Relational Mapping) framework for Java, widely used to map Java objects to database tables. It simplifies database interactions and enhances productivity. However, like any technology, proper tuning and monitoring are critical to achieving optimal performance. In this blog post, we will explore how to maximize Hibernate performance with Hawtio and Jolokia.
Understanding Hibernate Performance Challenges
Hibernate abstracts away a lot of the cumbersome database interactions but comes with its challenges, including lazy loading issues, N+1 queries, and inefficient use of caching. The Hibernate User Guide provides detailed insights into these topics, but here are some high-level issues to consider:
- Lazy Loading: Loading associated entities or collections when they are accessed may lead to excessive database hits if not managed effectively.
- N+1 Select Problem: A classic pitfall where one query retrieves the parent entity and subsequent queries retrieve child entities. This can significantly inflate the number of database calls.
- Caching Mechanism: Misconfigured caching strategies can lead to stale data or cache misses.
Thus, it is essential to address these challenges proactively to optimize performance.
Introducing Hawtio and Jolokia
Before diving into how to leverage Hawtio and Jolokia, let’s define what they are.
Hawtio
Hawtio is a lightweight, modular web management console for managing Java applications. Its features include:
- Monitoring: Provides detailed insights into your application's health and performance.
- Dynamic: It allows real-time management of Java applications on any JVM-powered infrastructure.
Jolokia
Jolokia is an agent-based bridge to JMX (Java Management Extensions). The combination of Jolokia with Hawtio allows us to extract monitoring data from our applications effectively.
Why Use Hawtio and Jolokia Together?
Combining these two powerful tools provides a robust monitoring solution for Hibernate applications. You can monitor JDBC connection pools, Hibernate sessions, query execution times, and more, giving you actionable insights into your application's performance.
Setting Up Hawtio with Jolokia
To utilize Hawtio for monitoring Hibernate, you need to ensure Jolokia is integrated into your application. Here’s how to set it up:
Step 1: Add Jolokia to Your Project
You can add Jolokia as a Maven dependency. Here’s the relevant snippet:
<dependency>
<groupId>org.jolokia</groupId>
<artifactId>jolokia-core</artifactId>
<version>1.6.0</version>
</dependency>
Step 2: Configure Jolokia
Configure Jolokia in the web.xml
file or Java configuration. A basic configuration in web.xml
looks like this:
<servlet>
<servlet-name>Jolokia</servlet-name>
<servlet-class>org.jolokia.http.AgentServlet</servlet-class>
<init-param>
<param-name>jolokiaConfig</param-name>
<param-value>/jolokia</param-value>
</init-param>
</servlet>
This configuration exposes Jolokia at /jolokia
.
Step 3: Integrate Hawtio
You can set up Hawtio by including it in your dependency management:
<dependency>
<groupId>io.hawt</groupId>
<artifactId>hawtio-core</artifactId>
<version>2.6.0</version>
</dependency>
Once configured, you can access Hawtio by navigating to http://<your-server>:<port>/hawtio
.
Monitoring Hibernate with Hawtio
In the Hawtio interface, you'll find various tabs and dashboards dedicated to JMX management. But, how exactly can you use this to enhance your Hibernate performance? Let's break it down.
Monitoring JDBC DataSource
One of the first things to check is the JDBC DataSource. This will help you observe the JDBC connection pool status. A properly configured connection pool is crucial for ensuring that your application performs well under load.
Here's how you might see it displayed:
- Active connections
- Idle connections
- Max connections
Observing Hibernate Sessions
Monitor the Hibernate session metrics, which can help identify:
- Open sessions
- Session duration
- Transaction status
The following JMX bean is often crucial in assessing session performance:
org.hibernate.stat.Statistics
You can query session statistics like this:
Statistics stats = sessionFactory.getStatistics();
System.out.println("Entity loads: " + stats.getEntityLoadCount());
By keeping an eye on session statistics, you can identify performance bottlenecks.
Detecting Queries
Monitoring executed Hibernate queries is vital as it helps identify slow or inefficient queries. In Hawtio, check the Query Count
metric. You can also set a logging level for displaying executed queries:
hibernate.show_sql=true
hibernate.format_sql=true
Example Query Monitoring
To illustrate, consider the following example defining a method to fetch users optimized with Hibernate:
public List<User> fetchUsers() {
return session.createQuery("FROM User", User.class).list();
}
If this method leads to excessive database calls, implementing batch fetching is advisable:
public List<User> fetchUsers() {
List<User> users = session.createQuery("FROM User", User.class)
.setHint("org.hibernate.fetchSize", 50)
.list();
return users;
}
Batch fetching reduces the number of individual calls and drastically improves performance. Monitor the performance using Hawtio to validate this approach.
Optimizing Caching Strategies
A well-structured caching mechanism is essential for maximizing Hibernate's performance. Utilize both first-level and second-level caches to optimize data retrieval. Here’s how you can enable second-level caching:
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
In your entity classes, define caching like so:
@Entity
@Cacheable
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class User {
// properties, getters, setters
}
Monitor these caches in Hawtio to see cache hit/miss metrics to further refine your approach.
The Closing Argument
Maximizing Hibernate performance using tools like Hawtio and Jolokia is not only possible but a highly effective strategy. The ability to monitor, diagnose, and resolve performance bottlenecks in real-time empowers developers to create efficient and robust applications.
Whether you are combating lazy loading issues, optimizing query performance, or implementing an effective caching strategy, utilizing these monitoring tools can provide significant insights. Take full advantage of Hawtio and Jolokia as they arm you with the visibility needed to make data-driven optimizations.
Implement these strategies and start reaping the benefits of enhanced Hibernate performance today!