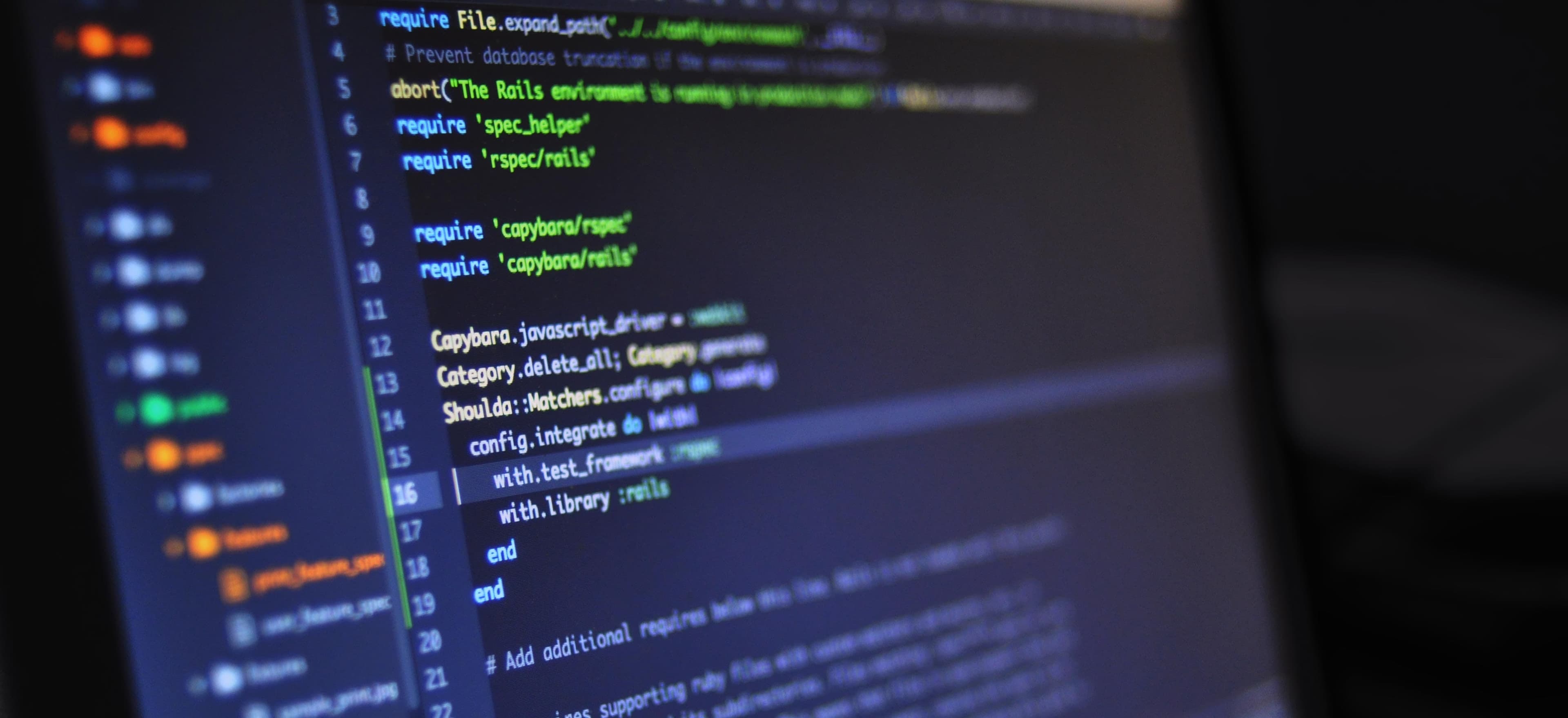
- Published on
Common Pitfalls in Domain Modeling with Spring Data Neo4j
As more applications evolve to utilize graph databases, the integration of these databases with popular frameworks such as Spring Data Neo4j becomes essential. While the advantages of using a graph database for complex relationships and data models are numerous, several common pitfalls can occur during domain modeling. This blog post will delve into these common pitfalls, illustrating each with relevant code snippets, and ultimately guide you on how to avoid them.
Understanding Domain Modeling in Neo4j
Domain modeling is the process of creating an abstract model of the domain that incorporates the key entities and their relationships. Spring Data Neo4j provides a convenient API for working with Neo4j, allowing developers to create domain models that map business models to Neo4j entities.
Before we dive into the pitfalls, it is crucial to understand that Neo4j utilizes a graph structure, comprising nodes, relationships, and properties. This structure may differ significantly from the traditional relational databases many developers are accustomed to.
Pitfall 1: Misunderstanding the Nature of Graph Data
One of the most significant misunderstandings occurs when developers attempt to forcefully fit relational data models into a graph database. This often leads to complex queries that defeat the purpose of using a graph.
Example of Misused Relationships:
@NodeEntity
public class User {
@GraphId
private Long id;
private String name;
@Relationship(type = "FRIENDS_WITH")
private List<User> friends = new ArrayList<>();
}
In this case, if the developers associate many relationships to represent complex hierarchies, it can become cumbersome. Rather than treating relationships as just a means to connect nodes, it’s important to evaluate how relationships inherently provide context.
Solution: Recognize the Relationship Types
Focus on the relationship's nature rather than creating excessive entities. The best practice is often to create specific relationship types tailored to your use case.
@NodeEntity
public class User {
@GraphId
private Long id;
private String name;
private List<String> interests = new ArrayList<>();
}
@NodeEntity
public class Interest {
@GraphId
private Long id;
private String name;
}
In this case, the relationship can be derived based on how nodes interact rather than explicitly declared in a cumbersome manner.
Pitfall 2: Overusing Annotations
Annotations such as @Relationship
or @Property
are powerful tools when defining your domain model. However, excessive use of these can both clutter your code and lead to performance issues.
Example of Overusing Annotations:
@NodeEntity
public class Post {
@GraphId
private Long id;
private String title;
@Property("content")
private String postContent;
@Relationship(type = "TAGGED_WITH")
private List<Tag> tags;
@Relationship(type = "CREATED_BY")
private User creator;
@Relationship(type = "COMMENTED_ON")
private List<Comment> comments;
}
Here, the Post
entity is overloaded with multiple relationships, potentially complicating the retrieval of data.
Solution: Streamline Relationships
Only utilize necessary relationships to ensure your domain model remains efficient.
@NodeEntity
public class Post {
@GraphId
private Long id;
private String title;
private String content;
}
In this streamlined model, we separate the tagging system, comments, and user relationships. This enables each concept to be represented without unnecessary complexity.
Pitfall 3: Ignoring Cyclic Relationships
Cyclic relationships can create becomes a significant challenge in graph databases. Failing to address them might lead to unexpected performance issues, such as infinite loops or stack overflow errors.
Example of Cyclic Relationships:
@NodeEntity
public class A {
@GraphId
private Long id;
@Relationship(type = "LINKED_TO")
private List<B> linkedBs;
}
@NodeEntity
public class B {
@GraphId
private Long id;
@Relationship(type = "LINKED_TO")
private List<A> linkedAs;
}
If entities A and B reference each other, it can lead to problems when fetching data if not carefully managed.
Solution: Limit Cyclic References
In some cases, mutual relationships might be necessary, but try to limit the depth of the cyclic relationships or use DTOs to decouple the entities when exposing them.
@NodeEntity
public class A {
@GraphId
private Long id;
// Extract detailed information into DTOs to avoid deep link observations
}
// Use a DTO when exposing API data, so you can flatten cycles
public class ADto {
private Long id;
private List<String> linkedBIds;
}
By transforming object structures into DTOs, you mitigate the risk of cycling through your model.
Pitfall 4: Not Considering Query Performance
Developers often focus solely on their domain model without running performance queries on that model. Neo4j excels in traversing data but poorly-structured queries can lead to subpar performance.
Example of Poor Query Design:
MATCH (u:User)-[:FRIENDS_WITH]->(f:User)-[:FRIENDS_WITH]->(fof:User)
RETURN fof
This query could form a large and complex execution path in Neo4j, leading to increased processing time.
Solution: Optimize Your Queries
Refining your queries will often involve leveraging specific indexing strategies and understanding relationships deeply. Review your data structure to optimize these paths.
CREATE INDEX ON :User(name);
Creating an index on the user’s name allows for faster retrieval rather than scanning through all relationship paths.
Pitfall 5: Failing to Use Transactions Effectively
In Spring Data Neo4j, the management of transactions is vital for maintaining your data integrity. Developers sometimes set up operations with no transaction handling, potentially causing loss of data integrity and rollback issues.
Example of Inefficient Transaction Management:
public void createUserProfile(User user) {
userRepository.save(user);
// Other logic that could fail
}
This example doesn’t account for transaction failures.
Solution: Use Transaction Management
Always ensure your operations are included in a transaction. This helps rollback any changes if part of the process fails.
@Transactional
public void createUserProfile(User user) {
userRepository.save(user);
// Other logic that could fail
}
Adding the @Transactional
annotation ensures that the entire method’s process is protected as a single operation.
Wrapping Up
Domain modeling within Spring Data Neo4j can present developers with several pitfalls that can lead to cumbersome, inefficient, and error-prone implementations. By understanding these common issues and following best practices—simplifying your relationships, leveraging DTOs, optimizing queries, and using transactions effectively—you can enjoy the benefits of a graph database while maintaining a clean and efficient domain model.
For further reading, check out Spring Data Neo4j Official Documentation for more insights, or explore the Neo4j Documentation for advanced strategies on dealing with complex relationships in your domain model.
The transition to graph databases can be a steep learning curve but understanding these fundamental modeling strategies will facilitate a smoother journey.
Checkout our other articles