Understanding JSON Patch vs JSON Merge Patch in Java
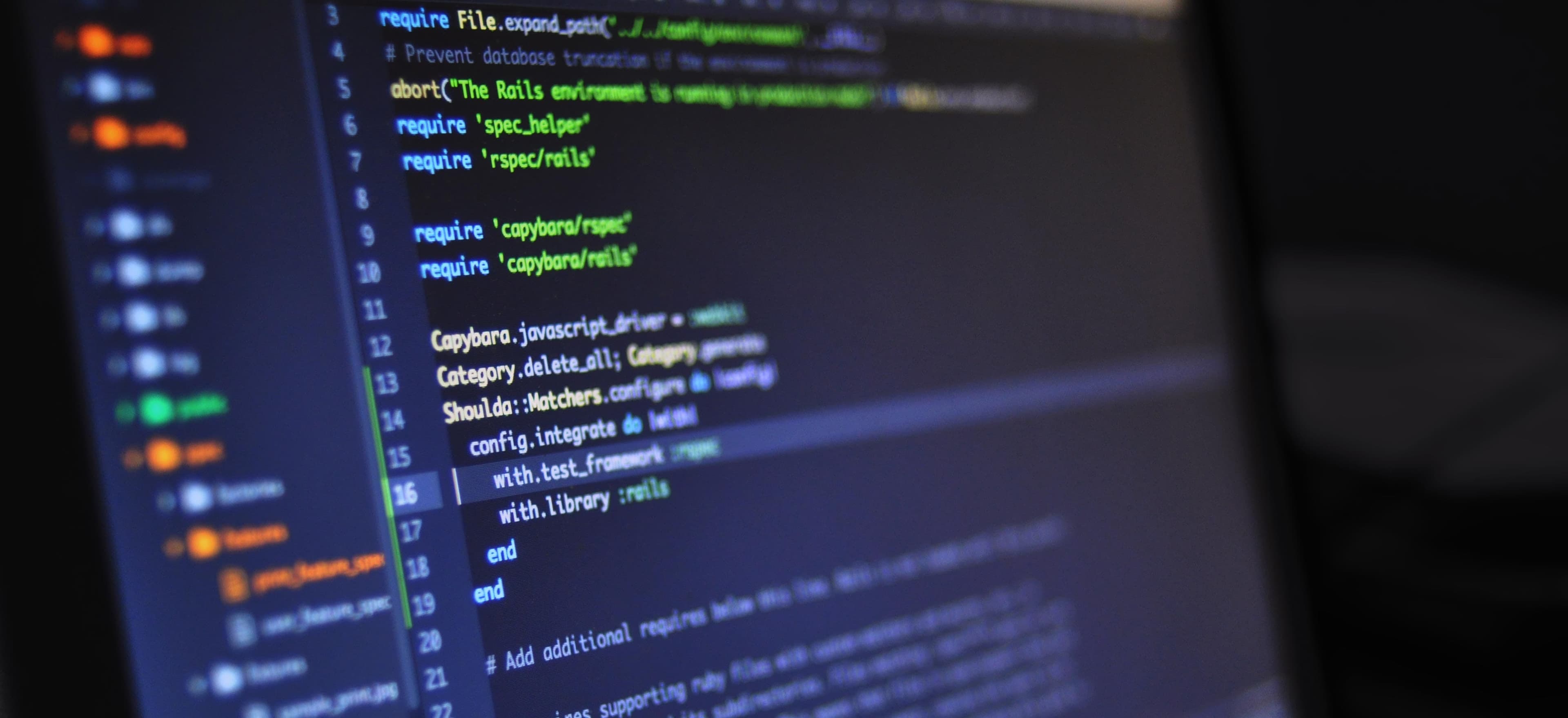
- Published on
Understanding JSON Patch vs JSON Merge Patch in Java
In the world of web development, particularly when working with REST APIs, JSON (JavaScript Object Notation) has emerged as a dominant format for data interchange. When dealing with updates to resources, two widely used standards are JSON Patch and JSON Merge Patch. Both serve the purpose of updating JSON documents but do so in different ways, each with its unique use cases. This blog post will guide you through the intricacies of both methods, particularly focusing on how they can be implemented in Java.
What is JSON Patch?
JSON Patch is a standard format described in RFC 6902 that defines a way to describe changes to a JSON document. It represents a sequence of operations, each of which specifies how to modify the JSON structure. Common operations include add
, remove
, and replace
.
Example JSON Patch
Let’s suppose you have the following JSON document:
{
"firstName": "John",
"lastName": "Doe",
"age": 30
}
If you want to change "age" to 31 and add a new field for "email", a JSON Patch document might look like this:
[
{ "op": "replace", "path": "/age", "value": 31 },
{ "op": "add", "path": "/email", "value": "john.doe@example.com" }
]
Implementation in Java
To implement JSON Patch in Java, you can use the popular library Jackson. Make sure to add the following dependency to your project:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.3</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-json-patch</artifactId>
<version>2.12.3</version>
</dependency>
Here’s how you can apply a JSON Patch to a JSON document in Java:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.jsonpatch.JsonPatch;
import com.fasterxml.jackson.dataformat.jsonpatch.JsonPatchException;
public class JsonPatchExample {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
try {
String originalJson = "{\"firstName\":\"John\", \"lastName\":\"Doe\", \"age\":30}";
JsonNode originalNode = mapper.readTree(originalJson);
String patchJson = "[{\"op\":\"replace\", \"path\":\"/age\", \"value\":31}, " +
"{\"op\":\"add\", \"path\":\"/email\", \"value\":\"john.doe@example.com\"}]";
JsonPatch patch = mapper.readValue(patchJson, JsonPatch.class);
JsonNode patchedNode = patch.apply(originalNode);
System.out.println(patchedNode.toPrettyString());
} catch (JsonPatchException | IOException e) {
e.printStackTrace();
}
}
}
Explanation of the Code
- ObjectMapper: This is the central class of Jackson for converting JSON to Java objects and vice versa.
- readTree: Converts the original JSON string into a
JsonNode
. - JsonPatch: A representation of a JSON Patch that can be read from a string.
- apply: The method that applies the patch operations to the original JSON node.
Why Use JSON Patch?
- Fine-grained control: You can specify exact changes you wish to make.
- Efficiency: By applying only the necessary changes, it reduces the data transferred over the network.
What is JSON Merge Patch?
JSON Merge Patch is described in RFC 7396 and is simpler compared to JSON Patch. Instead of a list of operations, it uses a partial document that indicates how to merge with the existing JSON structure. It allows for straightforward updates without explicitly defining the operations.
Example JSON Merge Patch
Continuing with the initial JSON document, if you want to change the "age" to 31 and add a new "email" field, the JSON Merge Patch would look like:
{
"age": 31,
"email": "john.doe@example.com"
}
Implementation in Java
You can also use Jackson to implement JSON Merge Patch. Here is how you do it:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
public class JsonMergePatchExample {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
try {
String originalJson = "{\"firstName\":\"John\", \"lastName\":\"Doe\", \"age\":30}";
JsonNode originalNode = mapper.readTree(originalJson);
String mergePatchJson = "{\"age\":31, \"email\":\"john.doe@example.com\"}";
JsonNode mergePatchNode = mapper.readTree(mergePatchJson);
JsonNode patchedNode = originalNode.deepCopy();
((ObjectNode) patchedNode).put("age", mergePatchNode.get("age").asInt());
((ObjectNode) patchedNode).put("email", mergePatchNode.get("email").asText());
System.out.println(patchedNode.toPrettyString());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explanation of the Code
- deepCopy: This is used to create a copy of the original JSON tree. It's essential because we want to preserve the original data.
- put: Updates or adds the fields in the patched node using the JSON Merge Patch data.
Why Use JSON Merge Patch?
- Simplicity: Easier to construct and understand for simple updates.
- Less verbose: Doesn’t require specifying operations, making the payload smaller.
Key Differences Between JSON Patch and JSON Merge Patch
| Feature | JSON Patch | JSON Merge Patch | |-----------------|---------------------------------|--------------------------------| | Format | Array of operations | Simple JSON object | | Operations | Add, Remove, Replace | Simple key-value replaces | | Structure | Requires knowledge of JSON paths| Merges based on keys | | Complexity | More complex | Simpler to use |
When to Use Which?
- JSON Patch is preferable when you require full control over your updates, particularly in scenarios where precise manipulation of data structures is necessary.
- JSON Merge Patch is ideal for simpler use cases and when the update payload needs to be compact.
In Conclusion, Here is What Matters
JSON Patch and JSON Merge Patch are two powerful tools at your disposal when managing JSON data in Java. Understanding their differences and use cases will empower you to choose the right one based on your project's needs. Most importantly, both approaches contribute to more efficient data management and communication in your applications.
Feel free to explore the official Jackson Documentation for more information and advanced use cases regarding JSON handling in Java.
By knowing when and how to use JSON Patch vs JSON Merge Patch, you'll be better equipped to handle updates within your JSON structures in a robust and efficient manner. Choose wisely!