Thread Safety Issues in Stateless Actor Systems
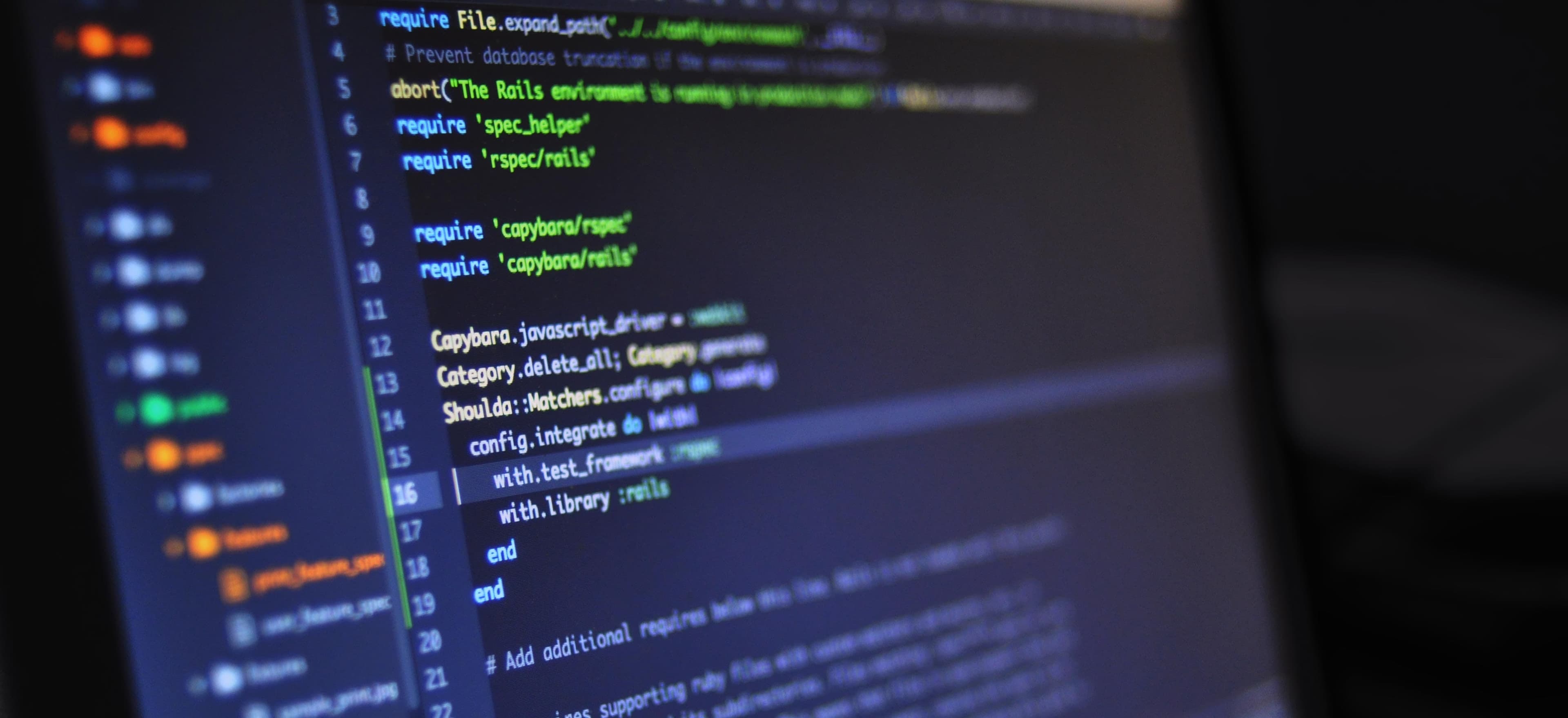
- Published on
Thread Safety Issues in Stateless Actor Systems
The Actor Model is a powerful abstraction for concurrent programming. It allows developers to design systems with components (actors) that can communicate asynchronously. These components can share data and respond to messages without worrying about traditional thread management. However, despite their inherent advantages, stateless actor systems can encounter thread safety issues. This blog post will explore the thread safety concerns associated with stateless actors, their implications for software design, and illustrate best practices with Java code examples.
Understanding the Actor Model
In the Actor Model, each actor is an independent unit of computation that:
- Encapsulates state.
- Processes messages sequentially.
- Communicates with other actors solely through asynchronous message passing.
This paradigm suggests that since the state is encapsulated, race conditions and other concurrency issues common in shared-state programming should be minimized. Nonetheless, stateless actor systems can still face specific challenges.
The Nature of Stateless Actors
Stateless actors are designed to operate without maintaining internal state across messages. Instead, they compute results based solely on the input messages they receive. This design principle accelerates processing and reduces complexity since there's no need for synchronization mechanisms.
However, although these actors do not maintain state, they can still potentially run into thread safety issues when interacting with shared mutable data outside their confines – a pitfall that can lead to data corruption or unexpected behavior.
Key Issues with Thread Safety
-
Shared Mutable State: When multiple actors access a mutable object, race conditions can occur. This situation can corrupt data or lead to inconsistent states.
-
Message Passing Timing: The timing of message processing can lead to scenarios where an actor processes messages out of order or receives unexpected states from external interactions.
-
External Dependencies: If an actor leverages services or data from external sources (like databases or APIs), the immutable state assumption breaks down. The actor may encounter issues depending on the timing of those external interactions.
In the following sections, we'll see how these issues manifest and how to mitigate them using Java.
Example of a Stateless Actor
Let's start with a simple stateless actor implemented in Java. We will create an actor that processes user registration info and sends a confirmation email. We won't maintain internal state; we will only compute based on the information received.
public class RegistrationActor {
public void onMessage(UserRegistration registration) {
// Process registration
sendConfirmationEmail(registration);
}
private void sendConfirmationEmail(UserRegistration registration) {
// Logic to send email
System.out.println("Sending confirmation email to " + registration.getEmail());
}
}
Why This is Stateless?
In the code above, the RegistrationActor
does not hold any internal state; it merely processes incoming messages (user registrations) and executes a function based on them. This actor is entirely stateless; any shared mutable resources it might (if it were using any) must be managed carefully.
Managing Shared Mutable State
If the RegistrationActor
accesses a shared resource, such as a database or a list of registered emails, we must ensure thread safety. Here’s how you might integrate a shared resource while avoiding thread safety issues using synchronized blocks or concurrent collections.
Using Java Concurrency Utilities
The Java concurrency package offers several classes designed to handle shared resources safely. Here’s an example of how you could use a ConcurrentHashMap
to store registered emails.
import java.util.concurrent.ConcurrentHashMap;
public class RegistrationActor {
private static final ConcurrentHashMap<String, Boolean> registeredEmails = new ConcurrentHashMap<>();
public void onMessage(UserRegistration registration) {
if (registerEmail(registration.getEmail())) {
sendConfirmationEmail(registration);
} else {
System.out.println("Email already registered: " + registration.getEmail());
}
}
private boolean registerEmail(String email) {
// Register email only if not already present
return registeredEmails.putIfAbsent(email, true) == null;
}
private void sendConfirmationEmail(UserRegistration registration) {
System.out.println("Sending confirmation email to " + registration.getEmail());
}
}
Why Use a ConcurrentHashMap?
-
Thread Safety:
ConcurrentHashMap
allows for concurrent read and write access, making it a good choice for shared data. -
Performance: The underlying structure is optimized for concurrent use, providing a better performance profile than explicit locks.
-
Simplicity: Using such utilities reduces the complexity of managing synchronization explicitly.
Avoiding Message Processing Issues
One of the critical features of the actor model is that actors handle one message at a time, leading to inherent synchronization. However, when stateless actors rely heavily on external data, this assumption isn't absolute. Below is a conceptual way to check incoming messages against external data safely.
Example with External Data
Imagine your actor checks a user’s role from an external service (like a database) every time it receives a registration request:
public class RoleCheckActor {
private final RoleService roleService;
public RoleCheckActor(RoleService roleService) {
this.roleService = roleService;
}
public void onMessage(UserRegistration registration) {
Role role = roleService.getRoleForUser(registration.getEmail());
processRegistration(registration, role);
}
private void processRegistration(UserRegistration registration, Role role) {
System.out.println("Processing registration for " + registration.getEmail() + " with role " + role);
}
}
Why is This a Problem?
If getRoleForUser
is slow or if it communicates with a non-thread-safe service, this actor could block or act unpredictably under load since it depends on external service states apart from its operations.
Mitigation Strategies
-
Use Asynchronous Patterns: Utilize futures or Completable futures to prevent blocking calls. This allows your actors to remain responsive and decoupled from external service latencies.
-
Circuit Breakers: Implement circuit breakers to gracefully handle failures from external services, ensuring the actor doesn’t keep retrying failed interactions ineffectively.
-
Request-Response Segregation: Consider employing a separate actor to handle external communications. This can isolate state management away from your main processing logic.
The Bottom Line
While stateless actors encapsulate many benefits for concurrent processing, their interaction with shared mutable state or external dependencies can introduce tricky thread safety issues. By understanding these challenges, leveraging Java's concurrency utilities, and following best practices, developers can minimize risks.
For readers looking to deepen their understanding of the Actor Model, I recommend visiting Akka Documentation for more insights on building resilient actor systems. Additionally, the Java Concurrency in Practice book is an excellent resource for mastering thread safety.
The potential complexities of concurrent programming should not deter developers from utilizing stateless actor systems. With proper precautions and strategies in place, you can harness the full power of the Actor Model while maintaining thread safety in your applications. Happy coding!
Checkout our other articles