Mastering JBock: Simplifying Java Command Line Interfaces
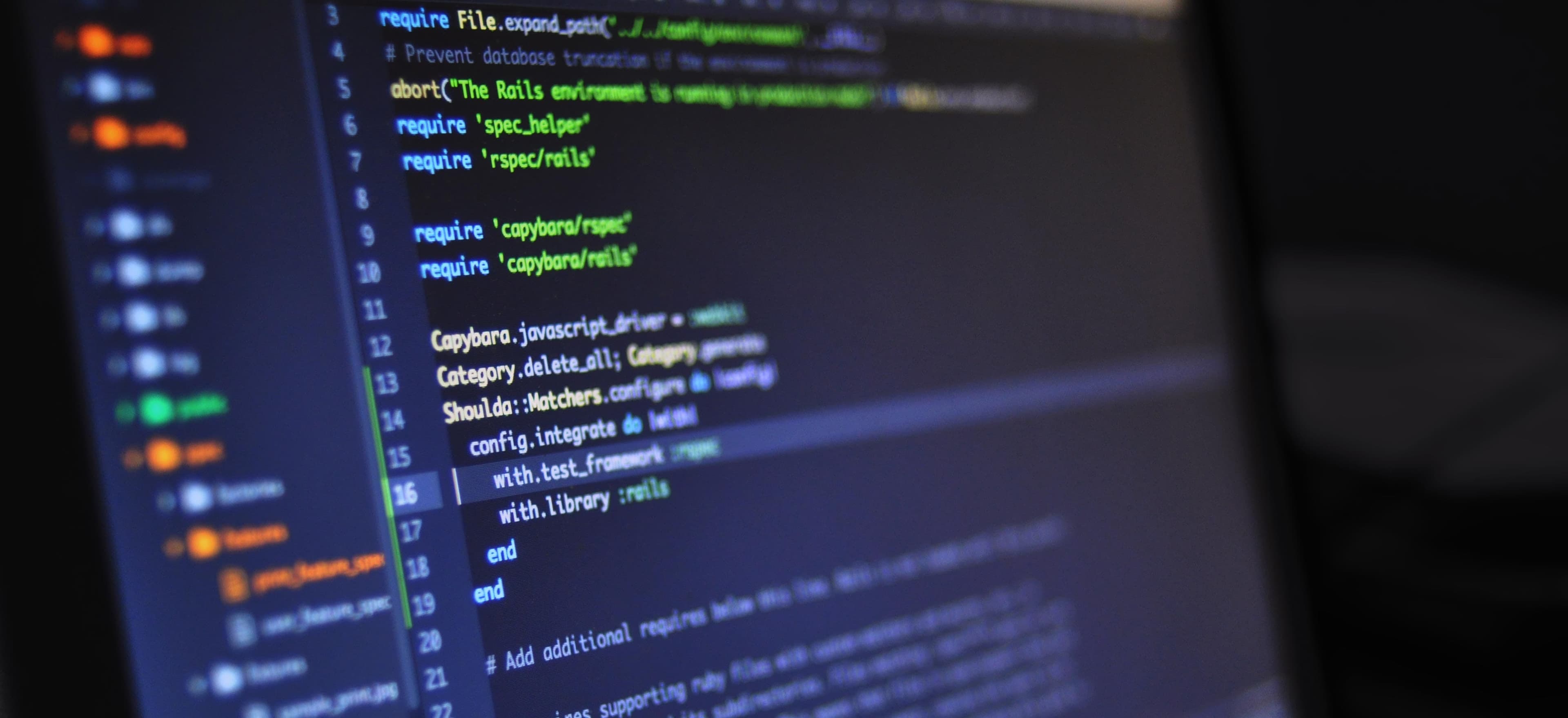
- Published on
Mastering JBock: Simplifying Java Command Line Interfaces
Java is a versatile and powerful programming language, widely used for building robust applications. However, creating command-line interfaces (CLI) in Java can often be cumbersome and time-consuming. Enter JBock—an innovative approach to simplifying CLI development in Java. In this blog post, we will explore what JBock is, how it works, and why it can be a game-changer for Java developers. We will also provide code snippets and clear examples to demonstrate its utility in practice.
What is JBock?
JBock is a lightweight library that provides an intuitive and expressive API for creating command-line interfaces in Java. It streamlines the process of parsing command-line arguments and options, making it easier for developers to implement complex CLI applications without getting lost in the maze of raw input processing.
Unlike traditional approaches, which often involve parsing args
arrays manually or using heavyweight frameworks, JBock simplifies this process by introducing annotations and a fluent builder style. This not only reduces boilerplate code but also enhances readability and maintainability.
Why Use JBock?
- Simplicity: JBock's straightforward API allows developers to create command-line interfaces quickly without extensive boilerplate code.
- Readability: The annotation-driven style makes it easy to see what options are available and how they are used.
- Type Safety: With JBock, you can define argument types, which provides better compile-time checks and reduces runtime errors.
- Flexibility: JBock supports advanced use cases like option groups and subcommands, making it flexible for a variety of CLI applications.
- Community Support: As JBock gains traction in the Java community, it is constantly updated with improvements and additional features.
Getting Started with JBock
To start using JBock, you need to add it as a dependency. If you're using Maven, you can add the following to your pom.xml
:
<dependency>
<groupId>org.jbock</groupId>
<artifactId>jbock</artifactId>
<version>1.0.1</version> <!-- Replace with the latest version -->
</dependency>
For Gradle users, simply add the following to your build.gradle
:
implementation 'org.jbock:jbock:1.0.1' // Replace with the latest version
Creating a Basic Command Line Application
Let’s create a simple command-line application that takes user input and performs operations based on that input. This example will allow the user to perform basic arithmetic operations such as addition and subtraction.
Step 1: Define Command Options
We will begin by creating a class to represent our command-line options. JBock uses annotations to define the available commands and options directly in your classes.
import org.jbock.Command;
import org.jbock.Option;
@Command(name = "calculator", description = "A simple CLI calculator")
public class CalculatorCommand {
@Option(name = "--add", description = "Add two numbers", required = false)
double add;
@Option(name = "--subtract", description = "Subtract two numbers", required = false)
double subtract;
@Option(name = "--first", description = "First number", required = true)
double first;
@Option(name = "--second", description = "Second number", required = true)
double second;
}
Commentary on Code Snippet
In this code, we have defined a CalculatorCommand
class to represent our CLI commands. The @Command
annotation helps annotate the command name and description. Each mathematical operation is represented as an option with the @Option
annotation.
Step 2: Processing Command- Line Arguments
Now, we need a way to process the command-line arguments. JBock takes care of argument parsing and automatically matches them to the appropriate fields.
import org.jbock.Jbock;
import static org.jbock.Jbock.*;
public class Main {
public static void main(String[] args) {
CalculatorCommand command = parse(args, CalculatorCommand.class);
if (command.add != 0) {
System.out.println("Result: " + (command.first + command.second));
}
if (command.subtract != 0) {
System.out.println("Result: " + (command.first - command.second));
}
}
}
Commentary on Code Snippet
In the Main
class, we're invoking parse()
with the command-line arguments and our CalculatorCommand
class. This function automatically maps the arguments to the corresponding fields based on the defined options.
The program checks if the add
or subtract
option was specified and performs the respective calculations.
Step 3: Running the Application
To run the application, compile it and execute it from the command line.
java -cp target/your-jar-file.jar Main --first 10 --second 5 --add
The above command will output:
Result: 15.0
Alternatively, for subtraction:
java -cp target/your-jar-file.jar Main --first 10 --second 5 --subtract
This will output:
Result: 5.0
Advanced Features of JBock
JBock also provides advanced features that are beneficial for larger applications. Here are a couple of notable ones:
Subcommands
You can create a CLI with multiple subcommands, each handling a different set of operations. This structure enhances the organization and scalability of your application.
@Command(name = "calculator", description = "A CLI calculator")
public class Calculator {
@Subcommand(name = "math", description = "Mathematical operations")
public static class MathCommand {
@Option(name = "--operation", description = "Operation to perform")
String operation;
@Option(name = "--number1", description = "First number")
double number1;
@Option(name = "--number2", description = "Second number")
double number2;
}
public static void main(String[] args) {
// Logic to handle the 'math' subcommand
}
}
Commentary on Code Snippet
In this snippet, we define a subcommand called math
, which encapsulates mathematical operations under the main calculator
command. This design allows the CLI application to grow without becoming chaotic.
Grouping Options
Grouping options is another powerful feature of JBock, allowing you to structure related options together, enhancing code clarity.
@Command(name = "file", description = "File operations")
public class FileCommand {
@OptionGroup(name = "file-options")
String filePath;
@Option(name = "--read", description = "Read from file")
boolean read;
@Option(name = "--write", description = "Write to file")
boolean write;
}
Commentary on Code Snippet
In the FileCommand
class, we use @OptionGroup
to encapsulate related options. This approach keeps the interface organized and makes it easier for users to recognize associated commands.
Lessons Learned
In summary, JBock is an excellent library for Java developers looking to create efficient, clear, and maintainable command-line interfaces. Its simplicity and flexibility, combined with powerful features such as subcommands and option grouping, make it a fantastic tool for CLI development.
Whether you are building a simple calculator or a complex system management tool, mastering JBock can significantly streamline your development process, allowing you to focus on core functionalities rather than argument parsing.
For more information, refer to the official JBock documentation. Happy coding!
Checkout our other articles