Avoiding OutOfMemoryError: Top Antipatterns to Watch For
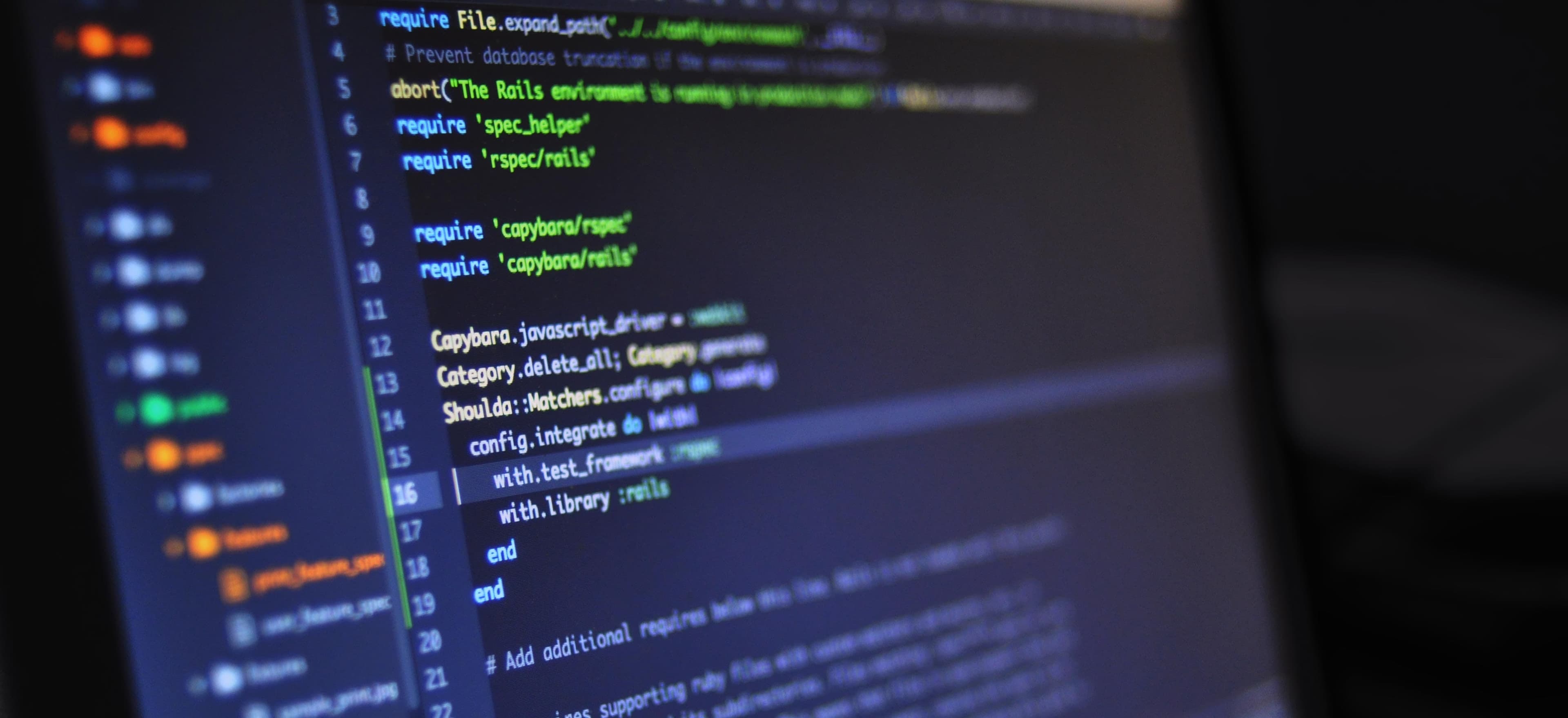
- Published on
Avoiding OutOfMemoryError: Top Antipatterns to Watch For in Java Development
Java is a powerful programming language known for its portability and flexibility, but it's not immune to the dreaded OutOfMemoryError
. This error occurs when the Java Virtual Machine (JVM) runs out of memory required to allocate new objects. In this blog post, we will explore the common antipatterns that lead to OutOfMemoryError
and how you can avoid them. By being aware of these pitfalls, you can write more efficient and effective Java applications.
What is OutOfMemoryError?
Before diving into antipatterns, let's clarify what an OutOfMemoryError is. This error is a subclass of Error
in Java, and it signals that the JVM cannot allocate any more memory for new objects. When this happens, your program crashes unexpectedly, which can lead to a frustrating experience for users and developers alike.
Key Types of OutOfMemoryError
To better understand how to avoid them, it's crucial to recognize the main types of OutOfMemoryError
:
- Java heap space: Occurs when the Java heap is exhausted.
- GC overhead limit exceeded: Happens when the garbage collector spends too much time trying to free up memory without success.
- Metaspace: Pertains to the area used for storing class metadata, mainly in Java 8 and later.
Now, let’s dive into the main antipatterns that can lead to these issues.
1. Memory Leaks
What is a Memory Leak?
A memory leak occurs when objects that are no longer needed are not de-referenced, causing the memory allocated to these objects to remain occupied. Gradually, this can lead to exhaustion of memory resources.
Example of a Memory Leak
Consider the following code snippet that creates a memory leak by holding references to unneeded objects in a list:
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakExample {
private List<Object> leakList = new ArrayList<>();
public void addToLeakList(Object obj) {
leakList.add(obj); // Potential for memory leak
}
}
Why This is a Problem
In the above code, every time addToLeakList
is called, an object is added to leakList
, and if we never clear this list, the memory for these objects will never be released. As the application runs, the heap could fill up quickly.
How to Avoid It
Use weak references where possible, particularly in caches or collections where you do not want the collection to prevent the objects from being garbage collected.
import java.lang.ref.WeakReference;
import java.util.HashMap;
public class Cache {
private HashMap<String, WeakReference<Object>> cache = new HashMap<>();
public void put(String key, Object value) {
cache.put(key, new WeakReference<>(value));
}
public Object get(String key) {
WeakReference<Object> ref = cache.get(key);
return ref != null ? ref.get() : null; // May return null if the object has been GC'ed
}
}
By using WeakReference
, you allow the garbage collector to free up memory when needed.
2. Large Object Allocation
Understand Object Sizes
Creating large objects like big arrays or collections can quickly deplete heap space. The JVM needs to allocate contiguous blocks of memory, which can become a challenge with large objects.
Example of Large Object Allocation
public class LargeArray {
public void createLargeArray() {
int[] largeArray = new int[Integer.MAX_VALUE]; // May lead to OutOfMemoryError
}
}
Why This is a Problem
The system may not have enough contiguous free memory to fulfill this request, leading to an OutOfMemoryError
.
How to Avoid It
Instead of allocating large arrays, consider breaking the data down into smaller manageable lists or using memory-efficient structures like ArrayList
or LinkedList
.
import java.util.ArrayList;
import java.util.List;
public class ManageableArray {
public void createManageableLists() {
List<int[]> lists = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
lists.add(new int[10000]); // Multiple smaller arrays
}
}
}
3. Inefficient Garbage Collection
Understand Your GC Settings
Inefficient usage of garbage collection can lead to GC overhead limit exceeded
. This happens when the application spends most of the time in garbage collection without making significant progress.
How to Identify the Issue
Using tools like VisualVM or Eclipse Memory Analyzer (MAT) can help diagnose memory use and GC performance.
How to Optimize
Review your JVM parameters to ensure you allocate enough heap space, or use different garbage collectors (like G1 GC or ZGC) optimized for large heaps:
java -Xms512m -Xmx4g -XX:+UseG1GC -jar YourApplication.jar
You may also wish to periodically review memory usage and GC-related statistics to understand how your application behaves under load.
4. Static Collections
Problem with Static Fields
Static collections that hold references to objects can easily lead to memory leaks, as they persist throughout the program's lifetime.
Example of Static Collection Issues
public class StaticCollectionExample {
private static List<Object> staticList = new ArrayList<>();
public static void addObject(Object obj) {
staticList.add(obj); // Objects are never removed, leading to potential leaks
}
}
Why is it Problematic?
Because staticList
remains engaged for the entire runtime of the application, and it won't be cleared unless the application shuts down.
How to Avoid It
Use instance variables instead, or clear the collection made to hold temporary data regularly.
public class InstanceCollectionExample {
private List<Object> instanceList = new ArrayList<>();
public void clearList() {
instanceList.clear(); // Regularly clear if items are not needed
}
}
5. Inefficient Use of Caching
Understand Caching Principles
Caching can improve performance but can also lead to memory issues if not implemented wisely. Keeping too many entries in memory can quickly lead to an OutOfMemoryError.
How to Implement Proper Caching
Use cache eviction strategies, such as Least Recently Used (LRU) to limit memory usage while ensuring cached objects are accessible.
import java.util.LinkedHashMap;
import java.util.Map;
public class LRUCache<K, V> extends LinkedHashMap<K, V> {
private final int maxSize;
public LRUCache(int maxSize) {
super(maxSize, 0.75f, true);
this.maxSize = maxSize;
}
@Override
protected boolean removeEldestEntry(Map.Entry<K, V> eldest) {
return size() > maxSize;
}
}
Why Use This Approach?
This implementation of LinkedHashMap
will automatically remove the least recently accessed entry when a new entry is added that exceeds the specified size.
Final Thoughts
By being mindful of these common antipatterns leading to OutOfMemoryError
, you can greatly enhance the stability and performance of your Java applications. Memory management requires diligence and a proactive approach to prevent crashes and improve user experience.
Leverage profiling tools, use efficient data structures, and always clear data collections that are no longer needed. For further reading, check out the Oracle Java Documentation on data types and Java Performance for JVM tuning tips.
Embrace these best practices, make your Java applications resilient, and avoid the pit of OutOfMemoryError
. Happy coding!
Checkout our other articles