How to Avoid Common Pitfalls of the Single Responsibility Principle
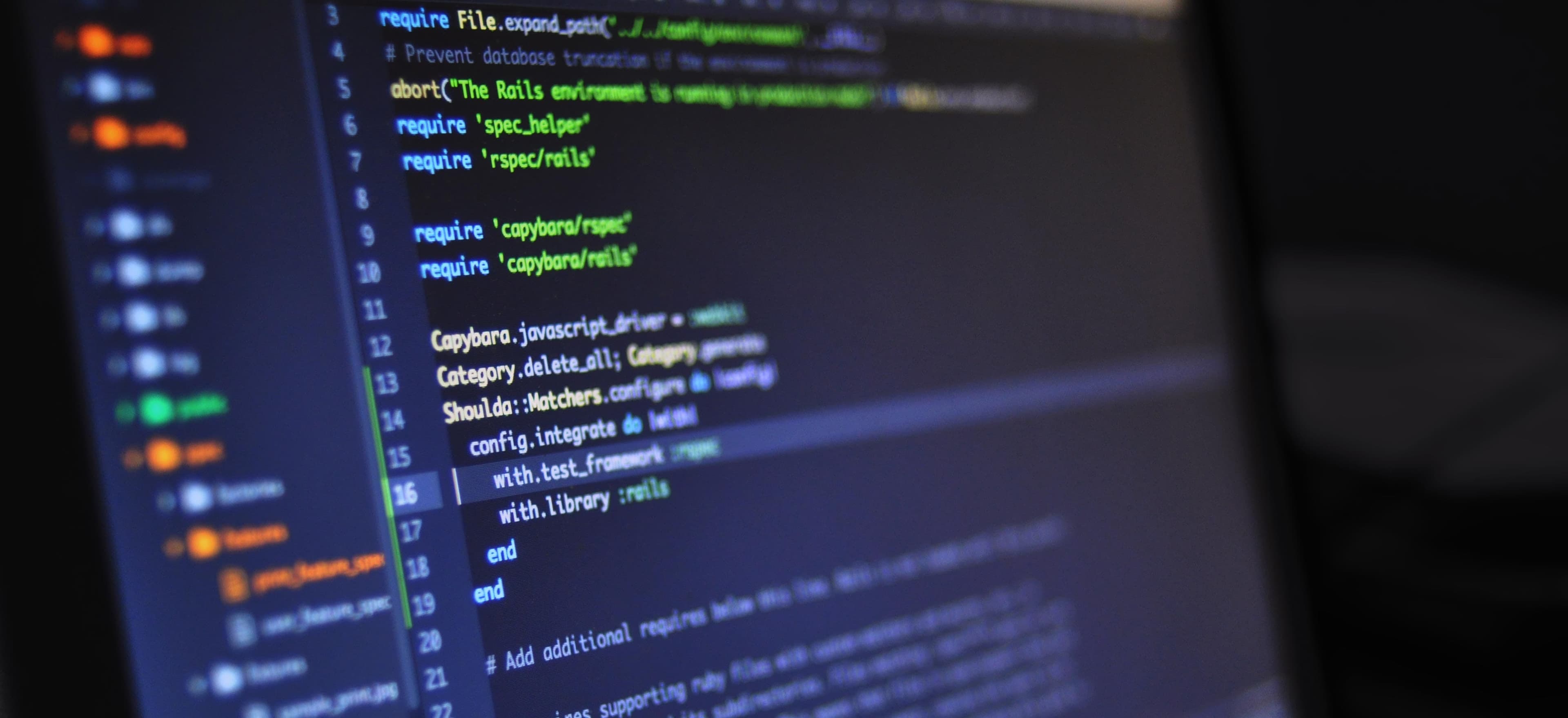
- Published on
How to Avoid Common Pitfalls of the Single Responsibility Principle
The Single Responsibility Principle (SRP) is one of the five SOLID principles of object-oriented design. This principle states that a class should have only one reason to change, meaning it should only have one job or responsibility. Upholding this principle is essential for creating maintainable, scalable, and easy-to-test software. In this blog post, we will explore common pitfalls of the Single Responsibility Principle and how to avoid them through careful design and refactoring strategies.
Understanding the Single Responsibility Principle
Before diving deep, let's explore what SRP entails. Simply put, SRP fosters clarity and simplicity in your code. When a class has multiple responsibilities, changes in one part of the code can lead to unexpected consequences in another. This is a clear violation of SRP and can result in bloated classes that are hard to reason about.
Why Embrace SRP?
The benefits of adhering to SRP include:
- Easier Maintenance: Smaller, focused classes are easier to manage over time.
- Enhanced Testability: Isolated responsibilities permit comprehensive unit testing.
- Better Reusability: Classes designed with a single responsibility can be reused in different contexts.
Common Pitfalls of the Single Responsibility Principle
While SRP is simple to understand, it's easy to misapply. Here are some common pitfalls and how to avoid them.
1. Over-Splitting Responsibilities
While SRP encourages separation of concerns, over-splitting can lead to an excessive number of tiny classes. This often results in overly complex interdependencies.
How to Avoid Over-Splitting:
-
Identify Core Responsibilities: Ensure that the responsibilities you separate are meaningful and substantial.
// Example of over-splitting public class User { private String name; // Responsibility: User data handling } public class UserDao { // Responsibility: Handle database interactions related to User } public class UserEmailService { // Responsibility: Handle sending emails related to Users } public class UserNotificationService { // Responsibility: Handle notifying users }
In this example, we have several classes dedicated to trivial functionalities. Instead, consider grouping related functionalities into fewer classes that encompass broader responsibilities.
2. Ignoring Dependencies Between Responsibilities
Often, separating responsibilities may introduce unwanted dependencies between classes that should remain decoupled. This might inhibit flexibility and introduce tightly coupled components.
How to Address Dependencies:
-
Design Interfaces: Utilize interfaces to reduce coupling.
public interface NotificationService { void notifyUser(String message); } public class EmailService implements NotificationService { @Override public void notifyUser(String message) { // Implementation to send an email notification } }
This approach allows for multiple implementations of the notification service, ensuring that changes to one implementation do not affect others.
3. Failing to Identify Real Responsibilities
A frequent issue arises when developers are not explicit about a class's responsibilities, leading to confusion and ambiguity.
How to Clarify Responsibilities:
-
Use Clear Naming: The class name should reflect its responsibilities.
-
Comment Judiciously: Provide documentation to clarify responsibilities.
public class ReportGenerator { private ReportRepository reportRepository; // Responsible for generating and formatting reports public Report generateReport() { // Generation logic } }
Ensure that your classes clearly communicate their intended functions through both naming and comments.
4. Neglecting the Business Domain
When designing classes and separating responsibilities, remember that they should correspond to real-world concepts or business domains. Misalignment can make the code convoluted and disconnected from the actual business logic.
How to Align with Business Domain:
-
Domain-Driven Design (DDD): Consider using DDD principles to guide your design choices and class responsibilities related to actual business needs.
public class Order { private List<Item> items; private Customer customer; // Responsible for managing the order lifecycle public void processOrder() { // Processing logic } }
Ensure that your classes and their responsibilities reflect true business needs.
5. Neglecting Performance Bottlenecks
Separating responsibilities can sometimes cause performance issues if not designed thoughtfully. Function calls may increase, leading to performance bottlenecks.
How to Monitor Performance:
- Benchmark and Profile: Regularly measure the performance of your classes and methods to identify slowing processes. Make use of tools such as JProfiler or VisualVM for monitoring.
Refactoring for SRP
Refactoring frequently to ensure compliance with SRP is crucial. Here are some effective strategies to implement SRP during refactoring:
-
Identify and Remove God Classes: These are classes that perform numerous responsibilities. Break them down into smaller, focused classes.
-
Create Helper Classes: If specific tasks must be executed, create helper classes to handle those tasks outside of your main classes.
-
Use Design Patterns: Certain design patterns like Strategy, Observer, and Factory naturally align with SRP principles. For example, using the Strategy pattern can help separate algorithms from the classes that use them.
Wrapping Up
The Single Responsibility Principle is a powerful tool for enhancing code quality. By avoiding common pitfalls such as over-splitting responsibilities, ignoring dependencies, and misaligning with business domains, you can create a more maintainable and flexible codebase.
As you continue developing your Java applications, remember that continuous refactoring and attention to SRP can simplify your code structure, improving both readability and testability.
For further reading about SOLID principles, consider checking out Martin Fowler's article or Robert C. Martin's book, Clean Code.
By following these guidelines, you'll not only improve your adherence to the Single Responsibility Principle but also elevate the overall quality of your software design. Happy coding!
This post is structured in an SEO-friendly manner, using relevant keywords such as "Single Responsibility Principle," "common pitfalls," and "Java coding best practices." Be sure to keep your code examples relevant while highlighting their importance for clarity.
Checkout our other articles