Overcoming ANTLR's Complexity: Building a Generic MetaModel
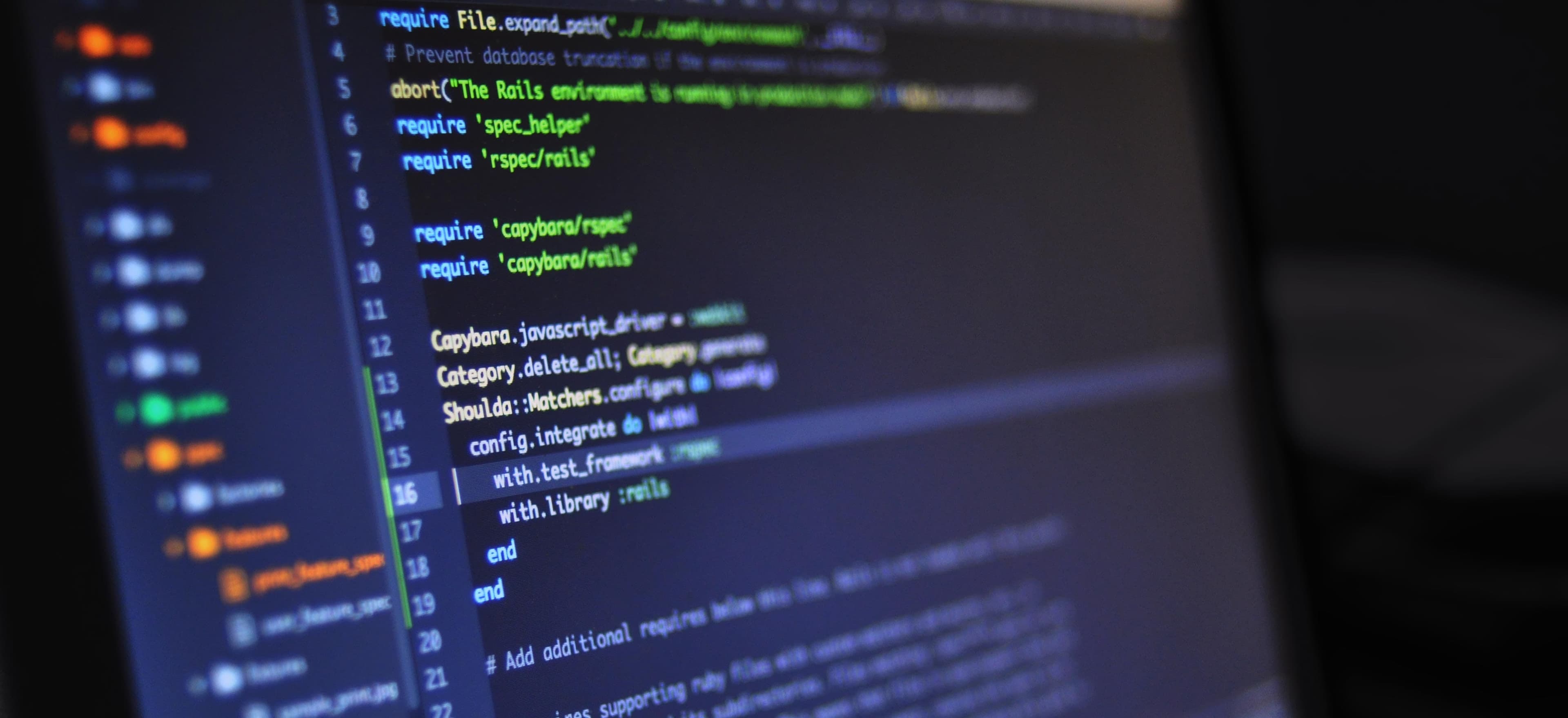
- Published on
Overcoming ANTLR's Complexity: Building a Generic MetaModel
ANTLR (ANother Tool for Language Recognition) is a powerful parser generator used for reading, processing, executing, or translating structured text or binary files. It generates code that can recognize and parse grammars defined in a writable format. While its capabilities are vast, the complexity involved in designing efficient grammars and parsers can be overwhelming.
In this blog post, we will discuss how to build a generic MetaModel using ANTLR. This approach enables developers to leverage the features of ANTLR more effectively and reduces the complexity that often accompanies it.
What is a MetaModel?
Before we dive into building a MetaModel, let's clarify what a MetaModel is. In simple terms, a MetaModel is an abstract model that describes how data can be structured. It acts as a blueprint for creating models and can be instrumental when creating domain-specific languages (DSLs) or custom parsers.
With a MetaModel, you can:
- Define structure: Describe the relationships between different data entities.
- Encapsulate behavior: Include the rules and constraints governing the data structure.
- Promote reusability: Create new structure by building on existing models.
Why Use ANTLR for MetaModel?
ANTLR provides robust features for building languages and parsers, making it an ideal choice for creating a MetaModel that can parse custom-defined languages. It also simplifies error handling and can generate parsers in multiple programming languages.
Getting Started with ANTLR
To begin, if you haven't already, install ANTLR by following the instructions on the ANTLR website. Once installed, create a new Java project in your IDE.
Step 1: Define Your Grammar
The first step in building a MetaModel with ANTLR involves defining a grammar. Below is a sample grammar for a simple expression language which can be a part of your MetaModel.
grammar Expr;
// Grammar rules
expr : term (('+' | '-') term)* ;
term : factor (('*' | '/') factor)* ;
factor : INT | '(' expr ')' ;
// Lexer rules
INT : [0-9]+ ;
WS : [ \t\r\n]+ -> skip ; // Ignore whitespaces
Here, we've defined an expression language that supports addition, subtraction, multiplication, and division. We have also included basic integer handling.
This grammar can later serve as a backbone for more complex models by incorporating additional rules and structures.
Step 2: Generate the Parser
Once the grammar is defined, use ANTLR to generate the lexers and parsers:
java -jar antlr-4.9.2-complete.jar Expr.g4 -o output
The above command generates files in the output
directory. These files are Java classes for the lexer and parser generated based on your grammar.
Step 3: Building the MetaModel in Java
Next, let's create a Java class that uses the generated parser. This class will allow us to create a simple MetaModel that can interact with our expression language.
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.tree.*;
public class MetaModel {
public static void main(String[] args) {
String expression = "3 + 5 * (10 - 4)";
// Create a CharStream from the given expression
CharStream input = CharStreams.fromString(expression);
// Create a lexer and a parser
ExprLexer lexer = new ExprLexer(input);
CommonTokenStream tokens = new CommonTokenStream(lexer);
ExprParser parser = new ExprParser(tokens);
// Parse the expression
ParseTree tree = parser.expr();
// Print the Parse Tree
System.out.println(tree.toStringTree(parser));
}
}
In this example, we begin by importing the necessary ANTLR classes. We define a main method that accepts an expression as a string, generates a CharStream
, and processes it through the lexer and parser.
Regarding the Code Snippet:
- Why Use CharStream?: CharStream represents the input source, which can be a file, string, or anything else. It standardizes the transition between raw input and parsed tokens.
- Why Create a Token Stream?: CommonTokenStream is critical for managing tokens post-lexical analysis. It simplifies token manipulation, which is vital when processing larger and more complex languages.
Enhancing the MetaModel
Now that we have a basic framework set up, we can enhance our MetaModel further. By defining different rules and integrating them, we can manage and interpret much more elaborate data structures and languages.
Additional Grammar Example
Let’s say we want to extend our expression language to include variable assignment. We can modify our grammar as follows:
grammar Expr;
// Additional rule for assignment
statement : ID '=' expr ';' ;
expr : term (('+' | '-') term)* ;
term : factor (('*' | '/') factor)* ;
factor : INT | ID | '(' expr ')' ;
// Lexer rules
ID : [a-zA-Z_]+ ;
INT : [0-9]+ ;
WS : [ \t\r\n]+ -> skip ;
Now, we can parse statements that include variable assignments.
Updated Java Code Snippet
Here's how we might adapt our Java code to handle this new grammar.
public class EnhancedMetaModel {
public static void main(String[] args) {
String statement = "x = 3 + 5 * (10 - 4);";
CharStream input = CharStreams.fromString(statement);
ExprLexer lexer = new ExprLexer(input);
CommonTokenStream tokens = new CommonTokenStream(lexer);
ExprParser parser = new ExprParser(tokens);
// Parsing a statement now
ParseTree tree = parser.statement();
// Print the updated Parse Tree
System.out.println(tree.toStringTree(parser));
}
}
Error Handling in ANTLR
Effective error handling is also a crucial part of developing with ANTLR. To handle errors gracefully, you can implement custom error listeners.
parser.removeErrorListeners();
parser.addErrorListener(new BaseErrorListener() {
@Override
public void syntaxError(Recognizer<?, ?> recognizer,
Token offendingSymbol,
int line, int charPositionInLine,
String msg,
RecognitionException e) {
System.err.println("Error at line " + line + ":" + charPositionInLine + " - " + msg);
}
});
By removing the default error listeners, you can insert your own logic for handling parsing errors. Here, a custom message is printed whenever the parser encounters an error.
Building a Complete MetaModel
To truly overcome ANTLR's complexity and build a functional MetaModel, you'll want to expand on what we've covered.
- Abstract Syntax Tree (AST): Implement functionality to generate an AST from the Parse Tree to represent the parsed input programmatically.
- Visitor Pattern: Consider using the Visitor pattern to traverse the tree and perform operations, making it easier to analyze and interpret the structure.
- Extensions: Create more sophisticated grammars that can evolve with your application needs.
Additional Resources for ANTLR and Language Development
If you're eager to learn more about ANTLR and sophisticated parsing frameworks, consider checking out the following resources:
- ANTLR Mega Tutorial – Comprehensive guide covering installation, grammar development, and parsing techniques.
- ANTLR Documentation – Official documentation providing in-depth details about the tool’s capabilities.
My Closing Thoughts on the Matter
Building a generic MetaModel using ANTLR allows developers to harness the full potential of this powerful parsing tool, enabling flexibility and reducing complexity in handling custom languages.
As you dive into developing your own MetaModels, remember to modularize your grammar and leverage ANTLR’s capabilities for parsing, error handling, and tree processing effectively.
By taking incremental steps and expanding upon foundational concepts, you can overcome the complexities of ANTLR and build robust and reusable MetaModels suited to your domain-specific needs.