Mastering Secondary Sorting in MapReduce: Common Pitfalls
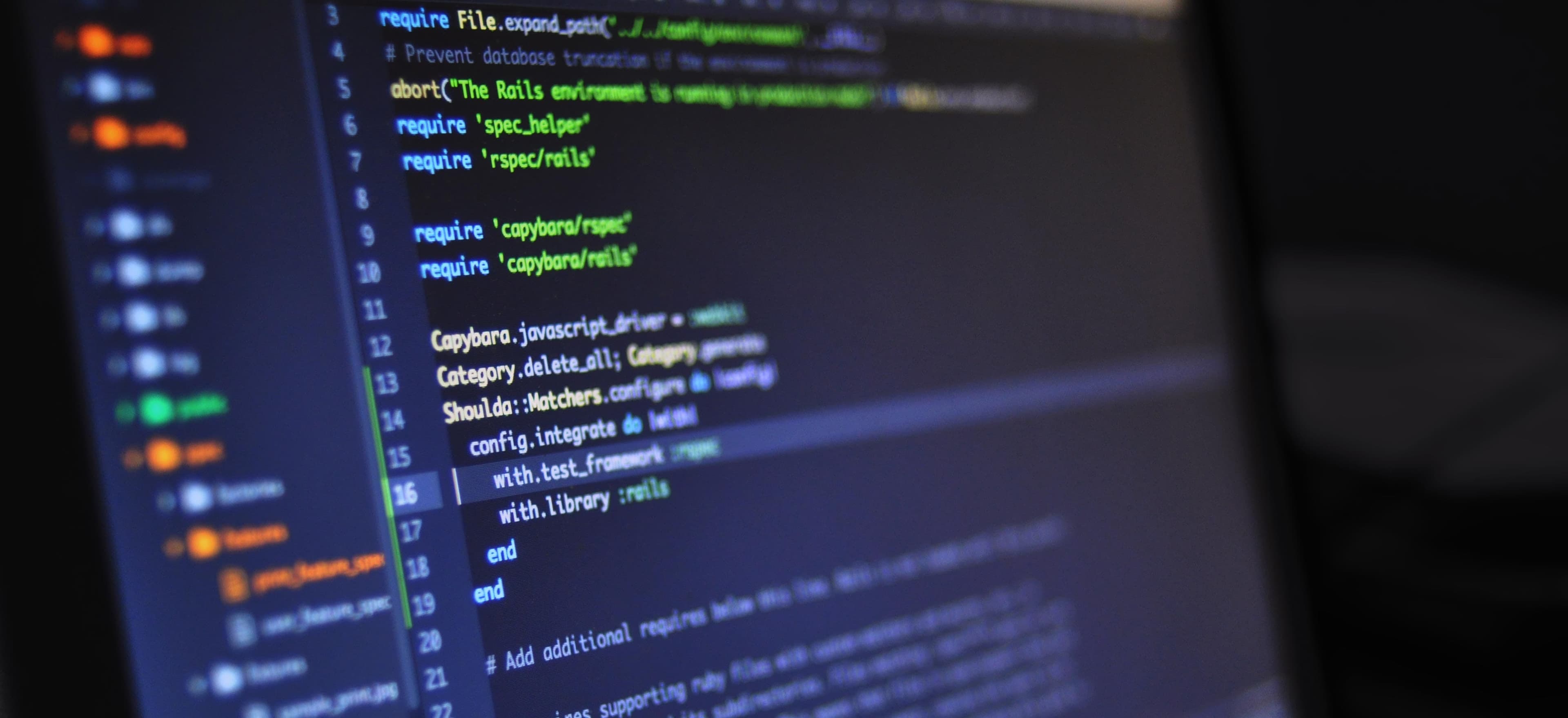
- Published on
Mastering Secondary Sorting in MapReduce: Common Pitfalls
MapReduce, a programming model for processing large data sets, offers developers a way to efficiently process these sets in parallel across a distributed cluster. While the basic mechanisms of MapReduce are powerful, secondary sorting often presents unique challenges. This article delves into the concept of secondary sorting in MapReduce, highlights common pitfalls developers may encounter, and provides solutions to ensure efficient implementation.
Understanding Secondary Sorting
The Basics of MapReduce
To grasp secondary sorting, it's essential to understand the fundamental components of MapReduce. The process consists of the following main phases:
- Map Phase: Input data is processed and transformed into a set of key-value pairs.
- Shuffle and Sort Phase: The MapReduce framework automatically sorts the output from the mappers. This step groups the values by key.
- Reduce Phase: The grouped key-value pairs are processed to produce the final output.
The Approach to Secondary Sorting
In situations where the primary key doesn’t suffice for sorting—such as needing to sort values based on a secondary attribute—secondary sorting comes into play. For instance, imagine sorting sales data first by sales person (the primary key) and then by sales amount (the secondary key).
Common Pitfalls in Secondary Sorting
While implementing secondary sorting in MapReduce can be straightforward, many developers encounter specific pitfalls. Here’s a breakdown of these challenges and how to overcome them:
1. Misunderstanding the Sorting Mechanism
One common misconception is that the built-in sorting mechanism of MapReduce can be directly extended for secondary sorting. In reality, secondary sorting requires careful control over how the output is formed before it arrives at the reduce phase.
Solution: Customize the Key Class
To enable secondary sorting, you need to create a composite key that combines both sorting criteria. Here’s an example Java implementation:
import org.apache.hadoop.io.WritableComparable;
import org.apache.hadoop.io.WritableComparator;
public class CompositeKey implements WritableComparable<CompositeKey> {
private String firstKey;
private int secondKey;
public CompositeKey() {}
public CompositeKey(String firstKey, int secondKey) {
this.firstKey = firstKey;
this.secondKey = secondKey;
}
// Getters, setters, hashCode, equals methods here...
@Override
public int compareTo(CompositeKey other) {
int compareFirst = this.firstKey.compareTo(other.firstKey);
if (compareFirst != 0) {
return compareFirst;
}
return Integer.compare(this.secondKey, other.secondKey);
}
}
// Comparator for sorting
public class CompositeKeyComparator extends WritableComparator {
public CompositeKeyComparator() {
super(CompositeKey.class, true);
}
@Override
public int compare(Object a, Object b) {
CompositeKey key1 = (CompositeKey) a;
CompositeKey key2 = (CompositeKey) b;
int cmp = key1.compareTo(key2);
return (cmp == 0) ? 0 : (cmp < 0 ? -1 : 1);
}
}
Commentary
In this code snippet, we define a composite key class that implements WritableComparable
. The compareTo
method allows us to specify the primary and secondary sorting criteria. The CompositeKeyComparator
is utilized during the shuffle and sort phase, enabling the side-by-side sorting of keys.
2. Inefficient I/O Operations
Another pitfall is falling into the trap of excessive I/O operations during the map phase, especially if secondary sorting requires multiple passes through data.
Solution: Optimize Data Processing
Always aim to build your data processing pipeline with efficiency in mind. For instance, consider using buffered writing. The following example illustrates how to batch writes rather than writing per entry:
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class BatchWriter {
private BufferedWriter writer;
public BatchWriter(String filePath) throws IOException {
writer = new BufferedWriter(new FileWriter(filePath, true));
}
public void writeKeyValuePair(CompositeKey key, String value) throws IOException {
writer.write(key.toString() + "\t" + value);
writer.newLine();
// Batch processing logic can be added here
}
public void close() throws IOException {
writer.close();
}
}
Commentary
In this example, we create a BatchWriter
class for buffered writing of key-value pairs. By minimizing the frequency of write operations, we reduce the time spent in I/O, thus boosting the overall performance of the MapReduce job.
3. Incorrect Result Interpretation
Developers often misinterpret the output of their MapReduce jobs after secondary sorting has been implemented. The final data structure may not represent the data that was intended because of improper assumptions about how the data is sorted.
Solution: Implement Unit Tests
To avoid misinterpretation, writing unit tests that validate the output of your MapReduce job is vital. Here’s a skeletal test case using JUnit:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class SecondarySortingTest {
@Test
public void testSecondarySorting() {
CompositeKey key1 = new CompositeKey("Alice", 300);
CompositeKey key2 = new CompositeKey("Alice", 200);
assertEquals(-1, key1.compareTo(key2)); // Alice, 300 > Alice, 200
}
}
Commentary
This simple unit test checks if the secondary sorting works as intended. When implementing your MapReduce job, ensure such tests are part of your verification process. They will help capture and address errors early in the development cycle.
Best Practices for Implementing Secondary Sorting
- Define Composite Keys: Always use composite keys to manage multi-level sorting.
- Utilize Efficient Comparators: Implement custom comparators that explicitly define the sorting order.
- Batch Your Operations: Minimize I/O during the map phase by batching writes when possible.
- Unit Testing: Establish rigorous unit tests to verify output correctness.
- Monitor Performance: Regularly profile MapReduce jobs to identify and resolve performance bottlenecks.
Key Takeaways
Mastering secondary sorting in MapReduce is critical for applications that require multi-level sorting. By understanding the common pitfalls and effectively implementing solutions, developers can enhance the functionality and efficiency of their data processing jobs. Utilize best practices and real-world examples to ensure your MapReduce applications are robust, efficient, and easy to interpret.
For further reading, dive into the Hadoop documentation, which offers extensive details on the inner workings of MapReduce and secondary sorting implementations. Happy coding!