Mastering Parallel Database Streams in Java 8: Common Pitfalls
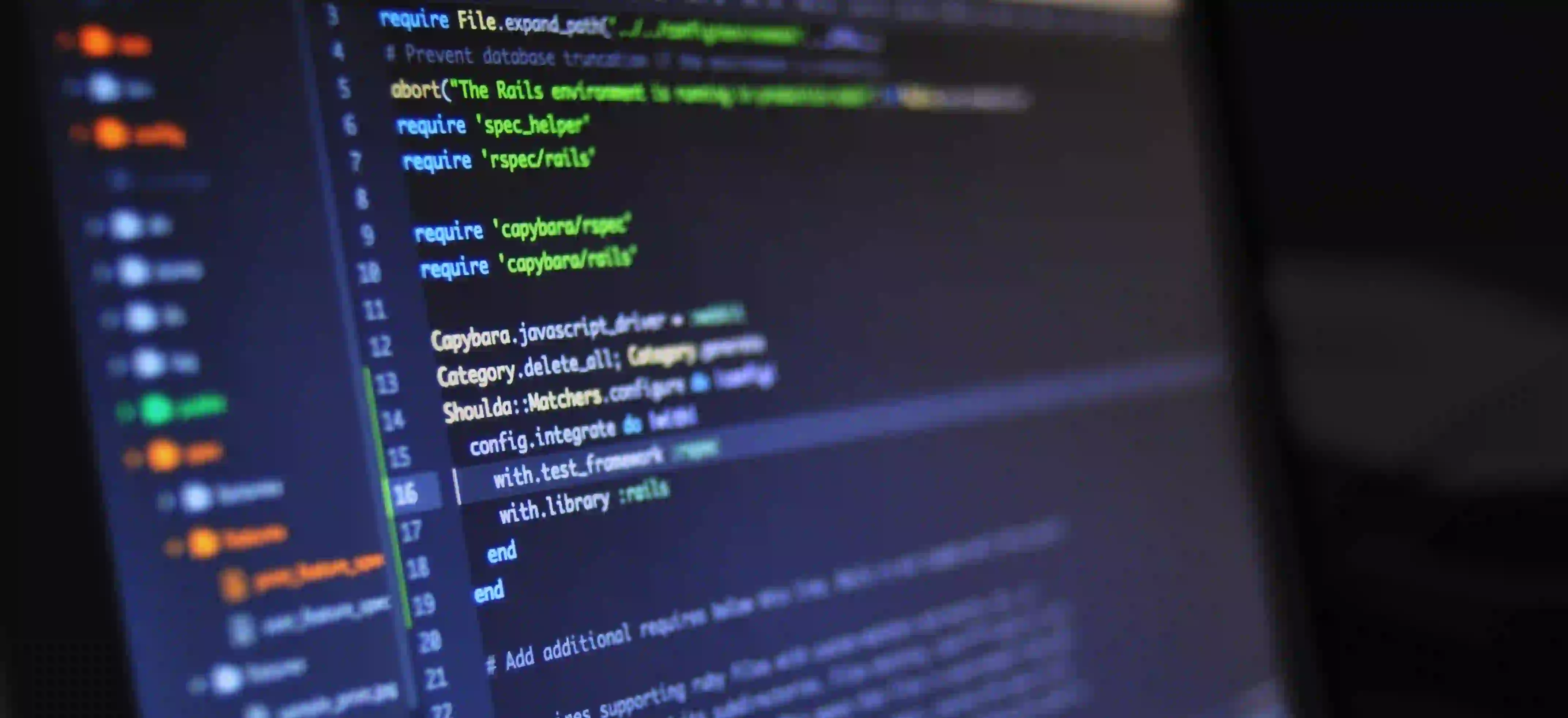
Mastering Parallel Database Streams in Java 8: Common Pitfalls
The advent of Java 8 brought a significant overhaul in how developers could handle streams of data. With the introduction of the Stream API, developers were equipped with a powerful tool for processing sequences of elements, potentially in parallel. However, with great power comes great responsibility. In particular, using parallel streams with databases can lead to performance bottlenecks or even incorrect results if not managed correctly. In this blog post, we will explore common pitfalls when leveraging parallel database streams in Java 8 and how to navigate them for optimal performance.
Understanding Parallel Streams
Before diving into the pitfalls, let's clarify what parallel streams are. Parallel streams allow us to process collections efficiently by dividing them into multiple parts and processing them concurrently using multiple threads. This is particularly useful for large data sets where processing can be a time-consuming task.
Example of a Sequential Stream:
List<String> data = Arrays.asList("one", "two", "three");
List<String> processed = data.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
Example of a Parallel Stream:
List<String> processedParallel = data.parallelStream()
.map(String::toUpperCase)
.collect(Collectors.toList());
While the second example uses parallelism, it is crucial to consider when and how to use it, especially with database interactions.
Pitfall 1: Thread Safety and Data Integrity
One of the most common issues with parallel streams is ensuring thread safety. When multiple threads operate on shared data, it can lead to inconsistent states or data corruption.
Example:
Consider a scenario where multiple threads are updating a database table. If these updates are not managed properly, one thread could overwrite the changes made by another thread, leading to lost updates.
Recommendation:
To mitigate this, ensure that any database operations performed in parallel streams are independent. Focus on using operations that do not modify shared state. If updates are unavoidable, consider using synchronized blocks or other concurrency controls.
synchronized (this) {
// Update database here
}
Pitfall 2: Connection Pool Exhaustion
When using parallel streams, each task may open a connection to the database. If the number of tasks exceeds the number of available connections in the pool, you could exhaust available connections, leading to potential application slowdowns or crashes.
Example:
Imagine you have a connection pool of size 10, with a parallel stream processing 1000 records. Each thread might attempt to request a connection that isn’t available, causing delays.
Recommendation:
Limit the number of threads used by your parallel stream. You can control the level of parallelism by using the ForkJoinPool
:
ForkJoinPool customThreadPool = new ForkJoinPool(4);
customThreadPool.submit(() ->
data.parallelStream().forEach(record -> updateDatabase(record))
).get();
This example will limit the parallel execution to four threads, helping manage the load on your connection pool.
Pitfall 3: Unpredictable Order of Operations
With parallel streams, the order in which operations are executed is not guaranteed. This can lead to issues where the outcome depends on the order of processing, particularly during aggregation operations.
Example:
If tasks depend on previous results, you could end up with an inconsistent state:
List<Integer> results = data.parallelStream()
.map(this::computeValue)
.collect(Collectors.toList());
If computeValue
depends on a shared state, results may vary.
Recommendation:
Use a sequential stream if the order of operations is critical, or ensure that operations are independent of one another.
Pitfall 4: Performance Overhead on Small Data Sets
While parallel streams can enhance performance with large data sets, they incur overhead in managing threads and tasks. For small data sizes, the overhead can negate the benefits of parallelism.
Example:
With a list of only a few items, the time taken to split tasks could exceed the time saved by processing in parallel.
Recommendation:
Perform extensive testing to determine the optimal threshold for switching from sequential to parallel processing. A good rule of thumb is to use parallel streams only for data sets larger than a few hundred elements.
Best Practices for Using Parallel Streams with Databases
-
Understand Your Data and Use Case: Analyze whether your data processing needs benefit from parallel execution. For small datasets, stick with sequential processing.
-
Keep Operations Stateless: Ensure that the operations performed in the stream are stateless and do not manipulate shared resources.
-
Monitor Database Connections: Regularly check your application's database connection pool to avoid exhaustion.
-
Utilize Proper Transaction Management: Use transaction management effectively to ensure data integrity, especially during updates.
-
Test, Test, Test: Always benchmark your application with varying data sizes. Monitor how parallel streams affect performance compared to sequential ones.
My Closing Thoughts on the Matter
Mastering parallel database streams in Java 8 can unleash powerful performance enhancements in your applications. However, it is essential to recognize and address the common pitfalls outlined in this post. By maintaining thread safety, ensuring reliable database connections, understanding the implications of order, and being cautious with small data sets, you can effectively utilize parallel streams while safeguarding your application's integrity and performance.
For further reading on working with collections in Java 8, consider checking out Java Documentation on Streams.
Remember, like any powerful tool, parallel streams require understanding and caution to yield the best results. Happy coding!
Additional Resources
- Effective Java by Joshua Bloch
- Java Concurrency in Practice by Brian Goetz
- Java 8 Streams API: A Tutorial
This guide serves to crystallize your understanding of parallel streams within the context of database interactions in Java 8, emphasizing not only the common pitfalls but also effective countermeasures to enhance reliability and performance.