Overcoming Common Pitfalls in Jakarta NoSQL Integration
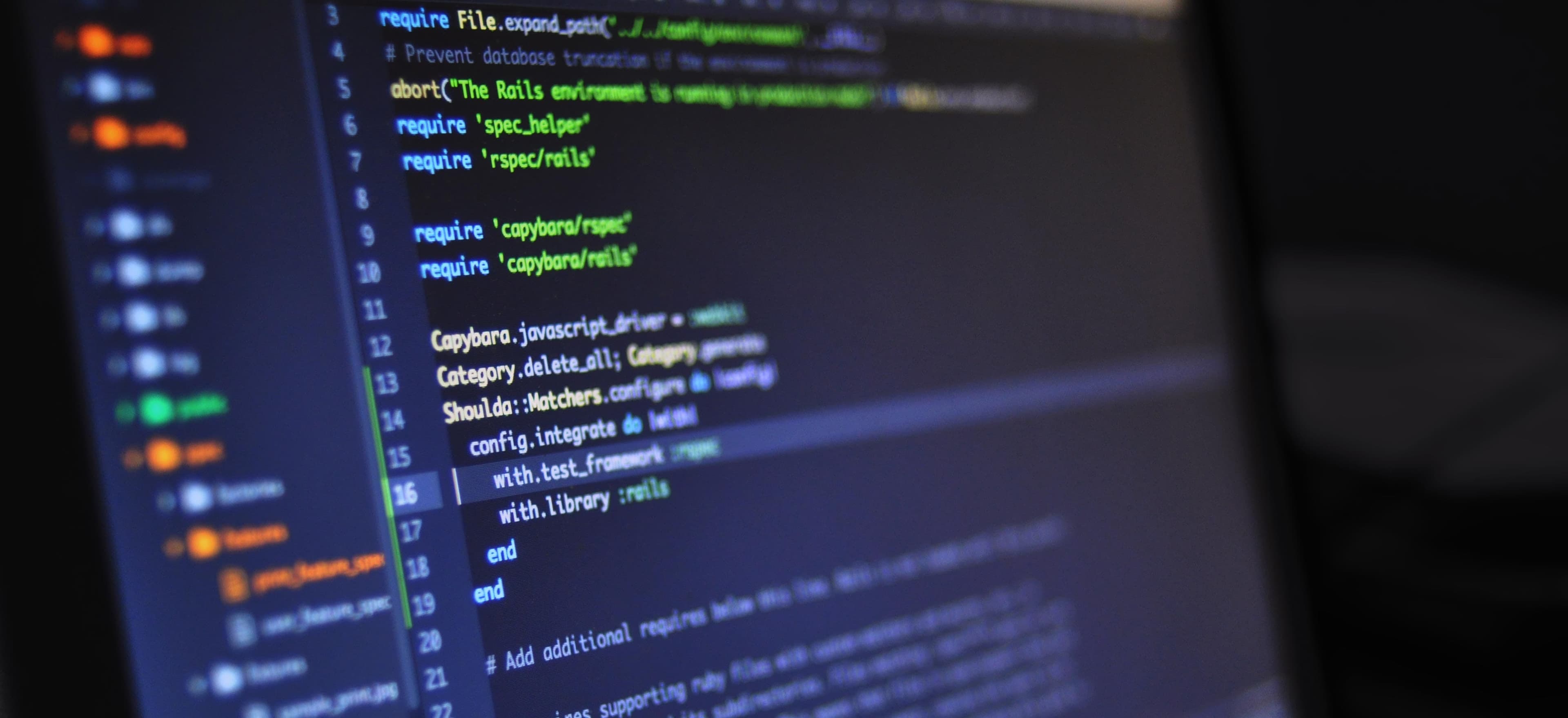
- Published on
Overcoming Common Pitfalls in Jakarta NoSQL Integration
In today's data-driven landscape, integrating NoSQL databases with Jakarta EE applications is becoming increasingly popular. The flexibility and scalability offered by NoSQL solutions make them excellent for applications that require rapid development and adaptability. However, developers often encounter specific challenges during this integration process. In this blog post, we will explore these common pitfalls and discuss effective strategies for overcoming them in your Jakarta EE applications.
Table of Contents
- Understanding NoSQL
- Common Pitfalls in Jakarta NoSQL Integration
- 2.1 Inadequate Schema Design
- 2.2 Lack of Familiarity with NoSQL Databases
- 2.3 Inefficient Data Querying
- 2.4 Ignoring Transaction Management
- 2.5 Poor Performance Tuning
- Best Practices for Smooth Integration
- Conclusion
1. Understanding NoSQL
NoSQL databases differ from traditional relational databases in that they do not rely on a fixed schema. This allows for greater flexibility in how data is stored and manipulated. Some of the most common types of NoSQL databases include:
- Document Stores (like MongoDB): Documents are stored as JSON or BSON formats, facilitating easy retrieval.
- Key-Value Stores (like Redis): Data is stored as a collection of key-value pairs.
- Column Families (like Cassandra): Data is organized into columns and rows but can maintain a non-uniform schema.
Why Choose NoSQL?
NoSQL databases are particularly beneficial for applications that require rapid iteration, scalability, and support for unstructured data. For an in-depth look at NoSQL features, visit NoSQL Database Types.
2. Common Pitfalls in Jakarta NoSQL Integration
Integrating NoSQL databases with Jakarta EE can come with its challenges. Below, we outline some of the most common pitfalls developers face.
2.1 Inadequate Schema Design
Although NoSQL databases allow flexible schema designs, the lack of a defined schema can lead to significant issues down the line. Often, developers either admit a strict schema altogether or create one that becomes cumbersome to maintain.
Solution: Define a Basic Structure
Think through the data model before diving into implementation. It is essential to have a basic understanding of the entities and relationships. Use tools or libraries that help visualize your data schema to avoid potential pitfalls early on.
// Basic user document structure in MongoDB
import org.bson.Document;
Document user = new Document("name", "John Doe")
.append("email", "john.doe@example.com")
.append("age", 30);
2.2 Lack of Familiarity with NoSQL Databases
Many developers are accustomed to working with SQL databases and may not fully grasp how NoSQL databases manage data differently. This knowledge gap can lead to inefficient coding practices and poor integration.
Solution: Invest Time in Learning
Take the time to learn about your chosen NoSQL database's capabilities and limitations. Look for relevant tutorials, online courses, and documentation specific to your database type.
For MongoDB, you might start with MongoDB University which offers numerous free courses.
2.3 Inefficient Data Querying
Another common mistake is the assumption that querying in NoSQL systems is as straightforward as in relational databases. Poor querying strategies can lead to slower performance and increased complexity.
Solution: Optimize Your Queries
Use proper indexing strategies and understand the query capabilities of your NoSQL database.
Here's an example of indexing in MongoDB to speed up query performance.
// Create an index on the 'email' field to optimize queries
collection.createIndex(Indexes.ascending("email"));
2.4 Ignoring Transaction Management
NoSQL databases often have limitations on transaction management compared to SQL databases. Ignoring these limitations can lead to inconsistent data states.
Solution: Utilize Document-Level Transactions
If your NoSQL database supports them, utilize document-level transactions to ensure certain operations are grouped together. For example, in MongoDB, you can use transactions across multiple collections if needed.
try (ClientSession session = client.startSession()) {
session.startTransaction();
try {
collection.insertOne(session, new Document("name", "Jane Smith"));
// Imagine there are other operations here...
session.commitTransaction();
} catch (Exception e) {
session.abortTransaction();
}
}
2.5 Poor Performance Tuning
As with traditional databases, performance tuning is crucial for NoSQL systems. Neglecting this aspect can result in poor application performance.
Solution: Regularly Monitor Performance
Utilize monitoring tools to analyze the performance of your queries and promptly adjust indexes, data structures, or other parameters as necessary. Most NoSQL databases offer built-in tools to assist with monitoring and performance tuning.
3. Best Practices for Smooth Integration
To successfully integrate NoSQL databases with Jakarta EE applications, consider the following best practices:
3.1 Choose the Right NoSQL Database
Different NoSQL databases serve different use cases. Choose a database that aligns with your application’s requirements.
3.2 Use Established Libraries
Utilize established libraries and frameworks for data access, such as Jakarta NoSQL, to simplify integration.
3.3 Invest in Testing
Ensure rigorous testing of your database interactions, especially if you're implementing complex operations.
3.4 Data Validation
Implement data validation mechanisms to enforce data correctness before insertion into the NoSQL store.
3.5 Stay Updated
NoSQL databases frequently update and evolve, as do their associated libraries and frameworks. Keep your development environment and libraries updated to take advantage of performance and security improvements.
4. Conclusion
Integrating Jakarta EE with NoSQL databases poses challenges, but careful planning and attention to potential pitfalls can lead to successful implementations. By understanding the nature of NoSQL databases and aligning them with best practices, you can optimize performance, maintain data integrity, and create scalable applications.
As you embark on your journey of integration, remember to prioritize schema design, query optimization, transaction handling, and continuous monitoring. By adopting these strategies, you will not only mitigate common pitfalls but also harness the full potential of NoSQL databases in your Jakarta EE applications.
For more insights on Jakarta EE and further resources, visit Jakarta EE Community.
By applying the methodologies discussed in this blog post, you're better positioned to succeed in your Jakarta NoSQL integration journey. Happy coding!
Checkout our other articles