Overcoming Road Data Challenges in Vehicle Routing with OptaPlanner
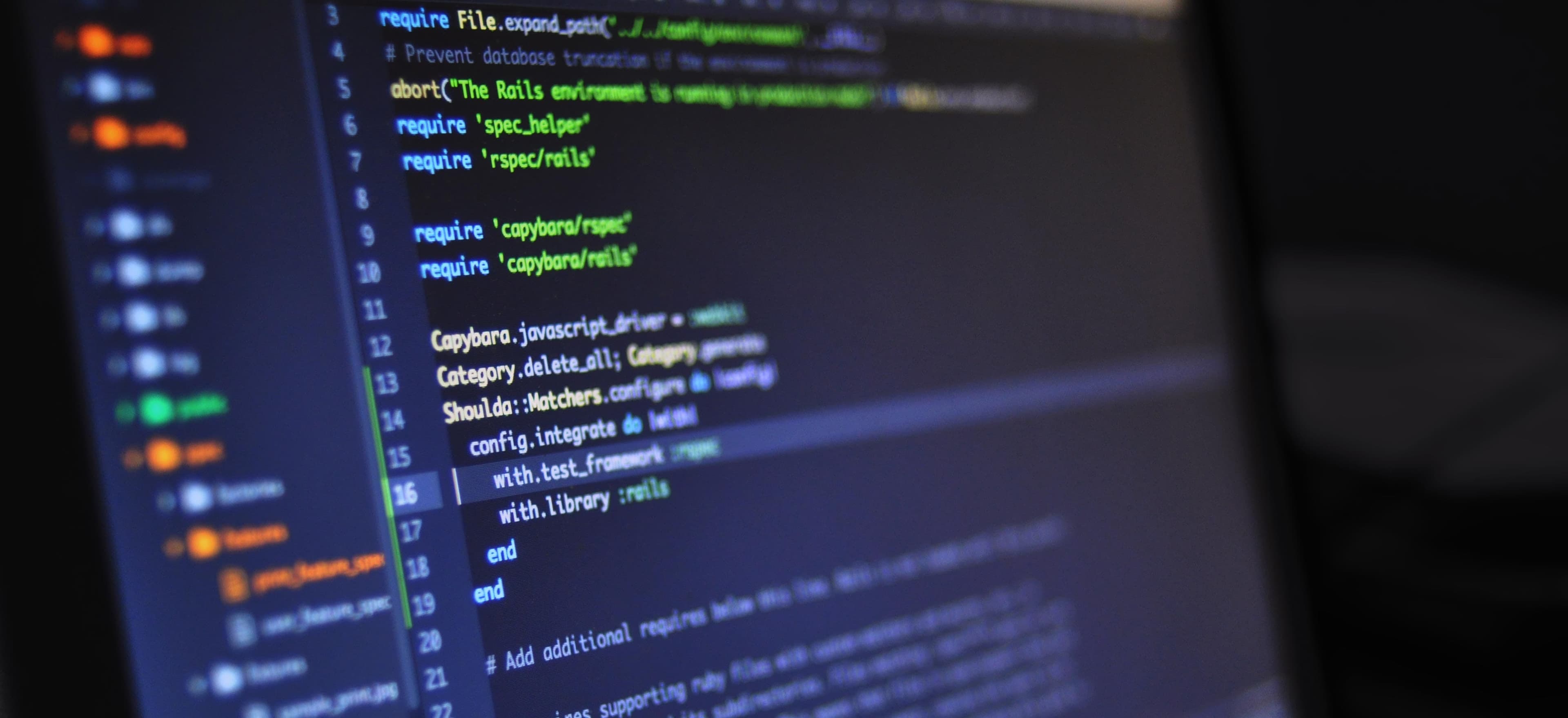
- Published on
Overcoming Road Data Challenges in Vehicle Routing with OptaPlanner
Vehicle routing is a complex optimization problem faced by many organizations, especially those involved in logistics and transportation. With the increase in digital data and real-time communication technologies, the challenge has evolved. Today, routing needs to account for dynamic road conditions, multiple delivery locations, and vehicle constraints. This is where OptaPlanner, a powerful Java-based constraint solver, comes to the rescue.
In this post, we will delve into the intricacies of overcoming road data challenges in vehicle routing using OptaPlanner. We'll explore how you can leverage its capabilities to enhance your routing strategies, improve efficiency, and offer real-time solutions.
Understanding the Vehicle Routing Problem (VRP)
Vehicle Routing Problems involve designing optimal routes for a fleet of vehicles to service a set of customers. The problems can be characterized by several factors:
- Multiple vehicles: There can be a fleet of vehicles that need to serve multiple locations.
- Constraints and penalties: Different constraints such as time windows, vehicle capacity, and penalties for late deliveries.
- Changing environments: Road data can change due to traffic conditions, accidents, and construction.
For a deeper dive into the different types of VRPs, you can check out the Vehicle Routing Problem Wikipedia.
Essentials at a Glance to OptaPlanner
OptaPlanner is an open-source constraint solver that helps optimize and plan complex problems such as VRP. It uses different algorithms to efficiently solve these optimization problems while allowing you to define constraints in a clear and concise manner. Built on Java, it seamlessly integrates with your Java applications and can leverage your existing data models.
Key Features of OptaPlanner
- Constraint Programming: Allows you to express complex rules that need to be satisfied.
- Performance: Capable of solving large problem instances quickly.
- Customizability: Highly customizable to suit specific routing needs.
- Dynamic Replanning: Able to adjust solutions based on real-time data.
You can visit the OptaPlanner official documentation for a more detailed overview.
The Challenges of Road Data
Before implementing a solution, it's important to identify what challenges road data present in the context of vehicle routing.
1. Incomplete or Inaccurate Data
Incomplete road maps or inaccurate data can lead to suboptimal routes. This may occur due to:
- Lack of real-time traffic information.
- Road closures not being updated.
- Missing geographic coordinates for delivery points.
2. Dynamic Traffic Conditions
Traffic conditions are never static; they change due to various factors, such as:
- Accidents.
- Roadwork.
- Weather conditions.
3. Time Windows and Constraints
Service locations often have specific time windows for deliveries. Balancing these constraints while minimizing travel costs is a significant challenge.
4. Capacity Restrictions
Each vehicle has its own capacity limitations. This must be taken into account when planning routes.
Utilizing OptaPlanner to Address Road Data Challenges
Next, we’ll look into how to implement OptaPlanner to overcome these challenges effectively.
Setting Up OptaPlanner
Before diving into code, ensure you have the necessary dependencies in your pom.xml
. Here's how to include OptaPlanner in a Maven project:
<dependency>
<groupId>org.optaplanner</groupId>
<artifactId>optaplanner-spring-boot-starter</artifactId>
<version>8.13.0.Final</version>
</dependency>
Creating Your Domain Model
In a typical vehicle routing application, you would have different entities such as Vehicle
, Location
, and Delivery
. Here’s an example of how to set up these classes:
public class Vehicle {
private String id;
private int capacity;
private List<Location> locations;
// Constructors, getters and setters
}
public class Location {
private String address;
private int demand;
// Constructors, getters and setters
}
Defining Constraints
OptaPlanner allows you to define constraints using a constraint provider. You can use the ConstraintProvider
interface to create rules that ensure routes respect delivery windows and avoid exceeding vehicle capacities.
Here's an example of defining a constraint:
public class VehicleRoutingConstraintProvider implements ConstraintProvider {
@Override
public Constraint defineConstraints(ConstraintFactory constraintFactory) {
return constraintFactory.from(Vehicle.class)
.filter(vehicle -> vehicle.getLocations().size() > vehicle.getCapacity())
.penalize("Capacity violation", HardSoftScore.ONE_HARD);
}
}
Implementing Real-Time Data Handling
One of the significant advantages of using OptaPlanner is its capability to perform dynamic replanning based on real-time data. Integrating APIs to fetch real-time traffic data can significantly improve route efficiency.
Fetching Real-Time Traffic Data
Here's an example of how to fetch traffic data using a REST API. You might consider using services like Google Maps for traffic updates:
public List<TrafficInfo> retrieveTrafficData() {
RestTemplate restTemplate = new RestTemplate();
String url = "https://api.google.com/maps/traffic?parameters";
TrafficInfo[] trafficInfos = restTemplate.getForObject(url, TrafficInfo[].class);
return Arrays.asList(trafficInfos);
}
Updating Routes
Using the real-time data obtained, you can update your vehicle routes dynamically:
public void updateRoutesWithTraffic(Vehicle vehicle, List<TrafficInfo> trafficInfos) {
// Use traffic data to adjust vehicle's route.
// This might involve recalculating distances and reassigning delivery schedules.
}
Integrating Everything Together
The last step involves bringing all the components together in a Spring Boot application. Ensure you set up your services and repositories correctly.
@SpringBootApplication
public class VehicleRoutingApplication {
public static void main(String[] args) {
SpringApplication.run(VehicleRoutingApplication.class, args);
}
@Bean
public SolverFactory<VehicleRoutingSolution> solverFactory() {
return SolverFactory.createFromXmlResource("solverConfig.xml");
}
@Bean
public PlanningSolution<VehicleRoutingSolution> planningSolution() {
return new VehicleRoutingSolution();
}
}
Running Your Application
Compile your Java application and run it. OptaPlanner will work in the background to evaluate the defined constraints and create the most optimal route for your vehicles.
Key Takeaways
Overcoming road data challenges in vehicle routing is no small feat, but by harnessing the power of OptaPlanner, organizations can optimize their vehicle routing strategies effectively. From defining constraints to integrating real-time data feeds, OptaPlanner provides a flexible and powerful framework for tackling the complexities of vehicle routing.
If you’re looking to enhance your logistics operations with intelligent routing solutions, OptaPlanner is definitely worth your consideration. For more examples and detailed tutorials, don't forget to access the OptaPlanner community page.
Further Reading
With technologies continually evolving, staying ahead with efficient, real-time vehicle routing is crucial for businesses to maintain their competitive edge. OptaPlanner is here to help you achieve that with robust solutions tailored to your routing needs.
Checkout our other articles