Common CI/CD Pitfalls in Spring Boot with Travis CI
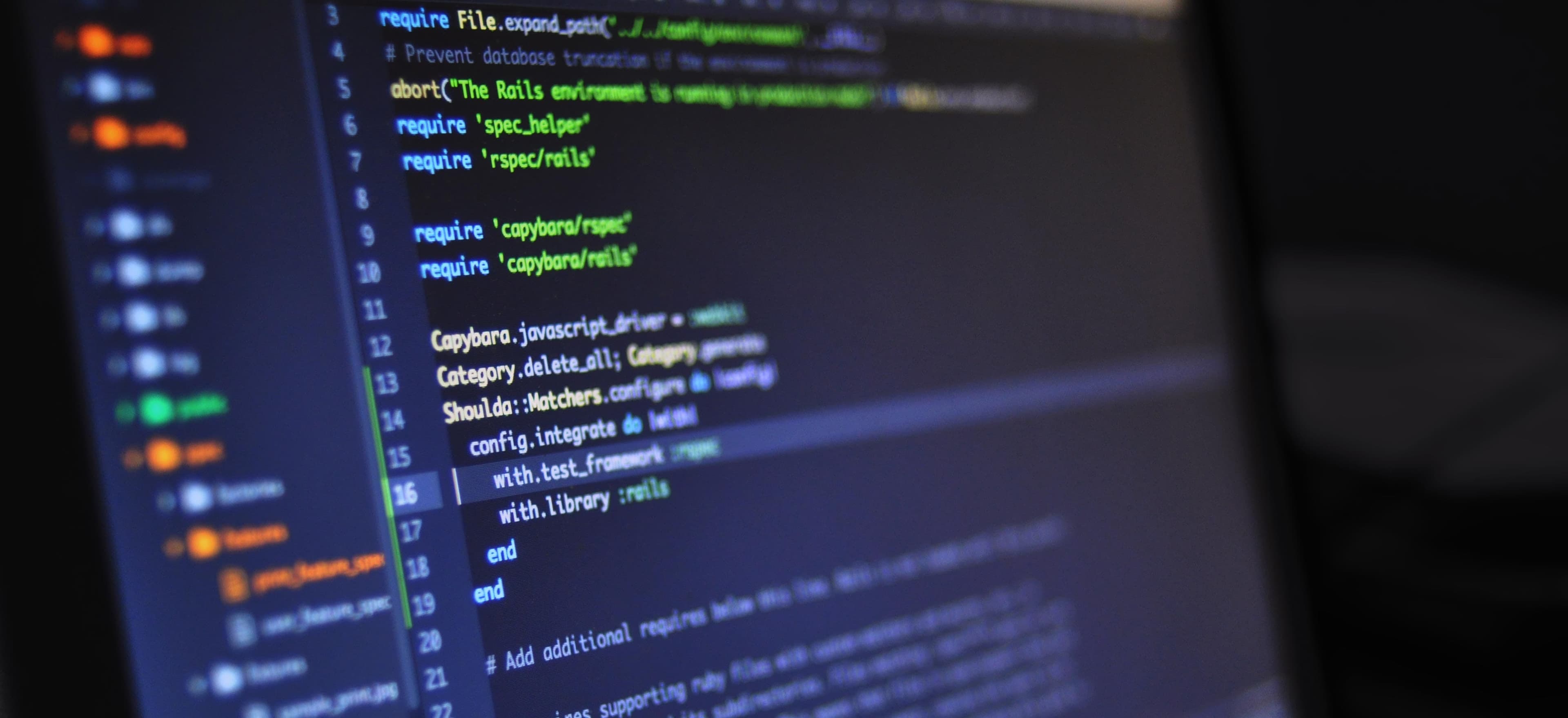
- Published on
Common CI/CD Pitfalls in Spring Boot with Travis CI
Continuous Integration and Continuous Deployment (CI/CD) is a vital part of modern software development, enhancing reliability, speed, and consistency in the release process. While working with Spring Boot and Travis CI, developers may encounter a variety of pitfalls that could derail their deployment process. This article explores these common issues and provides solutions to overcome them.
Understanding CI/CD with Spring Boot and Travis CI
Before diving into the pitfalls, it's beneficial to understand the integration of Spring Boot with Travis CI. Spring Boot simplifies the development of Java applications, while Travis CI offers a hosted continuous integration service, automating the process of testing and deploying software.
Why Use Travis CI with Spring Boot?
- Automation: Travis CI automates tasks, allowing developers to focus on coding instead of repetitive tasks.
- Cloud-Based: Being cloud-hosted, it provides easy setup and requires no maintenance.
- Integration: It easily integrates with GitHub, enabling seamless collaboration.
For an ideal setup, your project repository should contain a .travis.yml
file, which helps Travis CI understand how to build and test your project.
Sample .travis.yml Configuration
language: java
jdk:
- openjdk11
branches:
only:
- main
script:
- ./mvnw clean test
- ./mvnw package
- language: Specifies that we are using Java.
- jdk: Defines the Java version to use.
- branches: Restricts CI to run only on the main branch.
- script: Commands to run tests and build the application.
Now that you have a basic understanding, let's discuss common pitfalls.
1. Ignoring Test Failures
Issue Description
One of the most common pitfalls is not addressing failing tests in the CI/CD pipeline. Ignoring these failures can lead to deploying broken applications, affecting user experience and system reliability.
Solution
Ensure the CI/CD pipeline is configured to stop on test failures. This requires a robust testing strategy where each commit triggers a test and build process.
In your .travis.yml
file, keep the script section as follows:
script:
- ./mvnw clean test
By running tests before the build step, you'll catch issues early.
Good Practice
Use a testing framework like JUnit for unit and integration tests. This ensures comprehensive coverage of your application.
2. Not Managing Secrets Properly
Issue Description
Deployments often require API keys and credentials, and mishandling these during the CI/CD process can lead to security vulnerabilities. Hardcoding secrets into your code is a bad practice and can expose sensitive information.
Solution
Utilize environment variables and Travis CI's secure variable feature to manage secrets effectively.
-
Set up environment variables in the Travis CI settings interface or using the CLI:
travis encrypt MY_SECRET_KEY=your_secret --add
-
Reference the variable in your code:
String secretKey = System.getenv("MY_SECRET_KEY");
This ensures that sensitive data remains protected and is not exposed in version control.
3. Not Using a Dependency Management Tool
Issue Description
Spring Boot applications often rely on numerous dependencies. Failing to manage these properly can lead to version conflicts, which might cause the build to fail or behave unexpectedly.
Solution
Use a build management tool like Maven or Gradle. These tools provide a clear structure for managing dependencies and ensure that your application builds consistently in different environments.
For example, a basic Maven dependency block looks like this:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.4</version>
</dependency>
</dependencies>
More on Dependency Management
Properly versioning your dependencies ensures compatibility and stability. Sites like Maven Repository can help you find and manage these dependencies effectively.
4. Configuration Language and Environment Discrepancies
Issue Description
Differences between local environments and the CI/CD environment can cause unexpected failures. Hardcoded configurations or environment-specific profiles may lead to inconsistencies.
Solution
Utilize Spring Profiles to manage different configurations seamlessly. Create a application-{profile}.properties
file for each environment (test, development, production).
# application-test.properties
server.port=8081
spring.datasource.url=jdbc:h2:mem:testdb
Use the SPRING_PROFILES_ACTIVE
environment variable in Travis CI to specify which profile to load:
env:
- SPRING_PROFILES_ACTIVE=test
This ensures that your application behaves consistently across different environments.
5. Neglecting Logging and Monitoring
Issue Description
Often developers overlook logging and monitoring in CI/CD pipelines. Lack of insights makes it difficult to trace issues in production that were not caught during testing.
Solution
Integrate logging frameworks like SLF4J or Logback into your Spring Boot application. Include logging configurations in your application properties:
logging.level.root=INFO
logging.file.name=app.log
Additionally, consider integrating monitoring tools like Prometheus or ELK Stack to track application health in real time.
6. Not Using Artifacts Efficiently
Issue Description
Another pitfall arises when developers treat applications as a single deployable unit without utilizing build artifacts. This can make rollbacks complicated if something goes wrong post-deployment.
Solution
Package your application into artifacts (JAR, WAR) and ensure that these are versioned. For example, you can customize your Maven build to use the project version:
<build>
<finalName>${project.artifactId}-${project.version}</finalName>
</build>
In your .travis.yml
, enable artifact creation:
after_success:
- cd target
- tar -czvf myapp-${TRAVIS_TAG}.tar.gz myapp.jar
This way, each release is a self-contained artifact that can easily be rolled back if necessary.
Bringing It All Together
Successfully implementing CI/CD with Spring Boot and Travis CI requires diligence to avoid common pitfalls. From addressing failing tests and managing secrets, to ensuring proper dependency management and consistent environments, these steps enhance the reliability and security of your applications.
As you continue to integrate CI/CD in your development workflow, remember these strategies to minimize risks and streamline your processes. Your application and team will thank you!
For further reading, consider exploring these resources:
Stay ahead of the curve in software development by learning from these common pitfalls, ensuring a smoother CI/CD pipeline for your Spring Boot applications. Happy coding!
Checkout our other articles