Transform Legacy Java Applications into Modern WordPress Sites
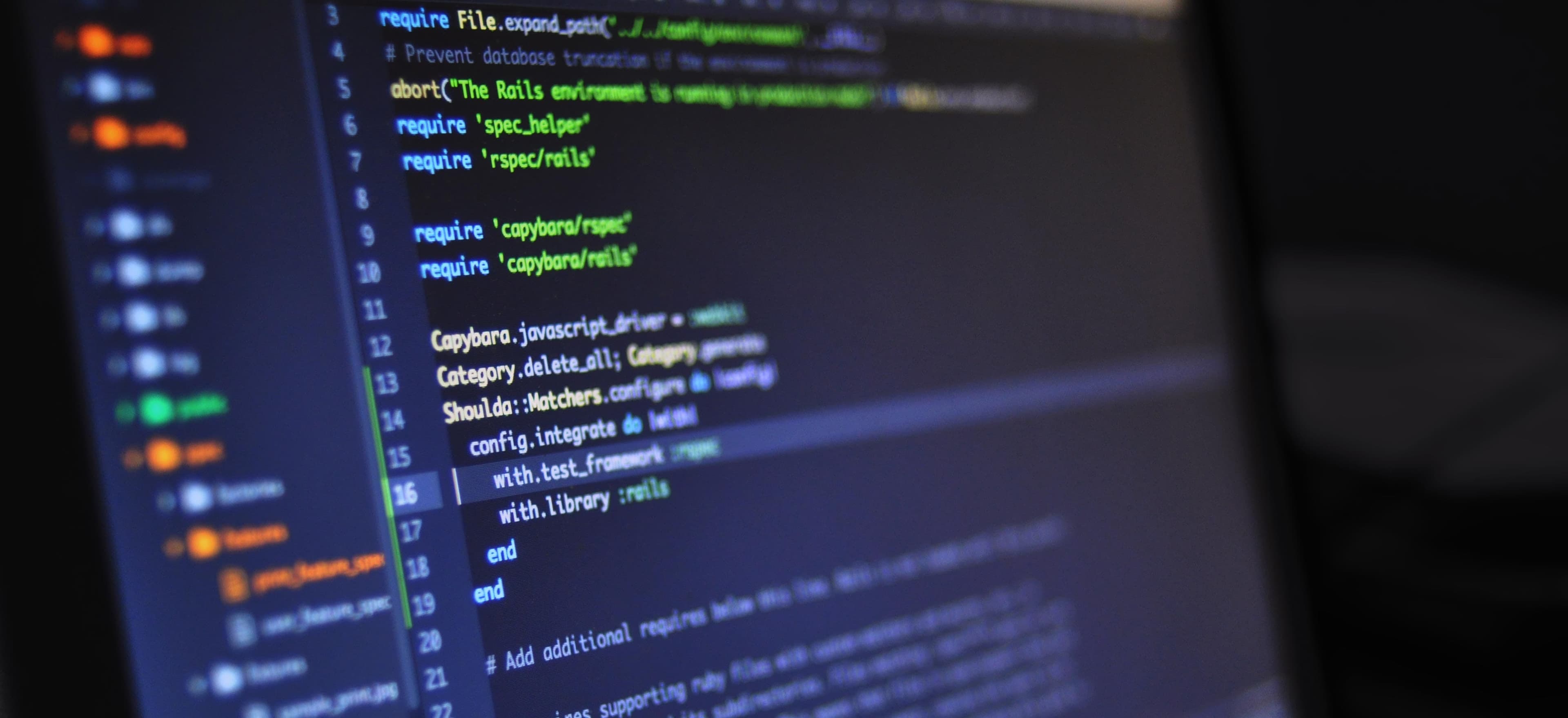
- Published on
Transform Legacy Java Applications into Modern WordPress Sites
Legacy Java applications have been the backbone of many businesses for years. However, with the rapidly changing digital landscape, stakeholders often seek ways to modernize these applications without completely overhauling them. One practical solution is to integrate legacy Java applications into a modern content management system (CMS) like WordPress. In this blog post, we'll explore the steps and techniques to achieve this transformation effectively.
Why Migrate to WordPress?
WordPress powers over 40% of the internet and provides robust features out of the box that can enhance user engagement and streamline content management. By migrating to WordPress, businesses can take advantage of:
- User-Friendly Interface: WordPress offers an intuitive dashboard that simplifies content management for non-technical users.
- SEO Optimization: WordPress has built-in SEO features and plugins that help improve visibility online.
- Extensive Plugins: With thousands of plugins available, you can extend WordPress's core functionality easily, enhancing your application’s capabilities.
- Community Support: WordPress has a vast community with ample resources, tutorials, and forums for support.
For further insights on revamping existing frameworks, check out Revamp Your Site: Integrate Old Content into WordPress.
Assessing Your Legacy Java Application
Before diving into integration, conduct a thorough audit of your Java application. Assess its features, database connections, user interfaces, and dependencies. This understanding lays the groundwork for the integration process.
Key Questions to Ask:
- What functionalities are critical?
- Can the business logic remain in Java, while the front end transitions to WordPress?
- How will users be impacted during the transition?
Mapping the Legacy Functionality to WordPress
Once you have a grasp of your legacy application, it’s time to create a roadmap. In this map, note how each legacy feature can be represented in WordPress.
Create Custom Post Types
In WordPress, you can create Custom Post Types (CPTs) to represent legacy data. For example, if your application manages "Products," you can create a CPT for that. Here’s a simple code snippet to register a custom post type:
function create_product_cpt() {
$args = array(
'labels' => array(
'name' => 'Products',
'singular_name' => 'Product',
),
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'thumbnail'),
);
register_post_type('product', $args);
}
add_action('init', 'create_product_cpt');
Explanation:
public
: This parameter makes sure that the post type is publicly accessible.has_archive
: This enables an archive page for the post type, allowing easy access to all products listed.supports
: This array specifies what features the post type supports (e.g., featured images).
Adopting a CPT approach allows you to leverage WordPress's robust content management while maintaining existing Java functionalities in the background.
Database Integration
Merging your legacy application's database with WordPress can be complex, but understanding your existing data structure is crucial. Using the WordPress database is typically preferred, as it simplifies data access.
Using WPDB for Custom Queries
The WPDB class is provided by WordPress for safe and effective database interactions. Here’s a sample code showing how to retrieve product data:
global $wpdb;
$results = $wpdb->get_results("SELECT * FROM legacy_products_table");
foreach ( $results as $row ) {
// Map the legacy data to Custom Post Types
// For example, creating a new product
$new_post = array(
'post_title' => $row->product_name,
'post_content' => $row->description,
'post_status' => 'publish',
'post_type' => 'product',
);
// Insert the post into the database
wp_insert_post($new_post);
}
Explanation:
get_results()
: This method retrieves the results from the legacy database.wp_insert_post()
: This WordPress function inserts a new post into the WP_post table, creating a record for each product.
This code will help you systematically port legacy data into your new WordPress framework while adhering to WordPress standards.
Implementing Front-End Functionality with Java
While the back end may remain in Java, you may want to enhance user experience on the WordPress front end. This can be accomplished through the use of Java-based RESTful services.
Creating a REST API in Java
If your legacy Java application has a REST API, you can use it to communicate between the WordPress front end and the Java back end. Here’s an illustration of how you might call a Java API from WordPress using JavaScript (via the WordPress enqueue function):
function enqueue_frontend_scripts() {
wp_enqueue_script('fetch-api', get_template_directory_uri() . '/js/fetch-api.js', array('jquery'), null, true);
}
add_action('wp_enqueue_scripts', 'enqueue_frontend_scripts');
JavaScript to Call the API
Place this in your fetch-api.js
file to pull data dynamically:
fetch('https://your-java-api.com/products')
.then(response => response.json())
.then(data => {
data.forEach(product => {
// Implement logic to render product data on the webpage
console.log(product);
});
});
Explanation:
- The
fetch()
function retrieves data from the Java service. - The
then()
method handles the response from the API, enabling you to manipulate and display data as needed.
Utilizing WordPress Plugins for Enhanced Features
WordPress's plugin ecosystem provides bespoke solutions that can enhance your application's capabilities. Whether you need eCommerce capabilities, SEO enhancements, or performance optimizations, there’s likely a plugin that can help.
- WooCommerce: For any eCommerce features.
- Yoast SEO: To improve your site's visibility and ranking.
- WP Super Cache: For performance improvements.
Testing and Validation
Testing is a critical phase that should not be overlooked. Ensure you thoroughly test both the front and back ends:
- Unit Testing Java Code: Keep your legacy logic intact by running unit tests on the Java code to ensure stability.
- User Acceptance Testing: Get feedback from actual users to refine the transition.
- Performance Testing: Monitor the performance of the newly integrated WordPress site versus the legacy application.
Final Considerations
Integrating legacy Java applications into WordPress offers a compelling pathway to modernization without abandoning valuable resources. With careful planning, code mapping, database integration, and leveraging WordPress's rich feature set, your legacy app can become a functional, user-friendly website.
In this journey, continue to leverage resources and articles, such as Revamp Your Site: Integrate Old Content into WordPress, to refine your strategy.
Final Thoughts
Modernizing your legacy Java applications does not have to be daunting. With WordPress’s flexibility, scalability, and user-centric design, you can create a seamless transition that meets both current needs and future growth. Your journey toward revitalizing your application is just beginning!