Mastering Gradient Fills: Enhance JavaFX with SVG Patterns
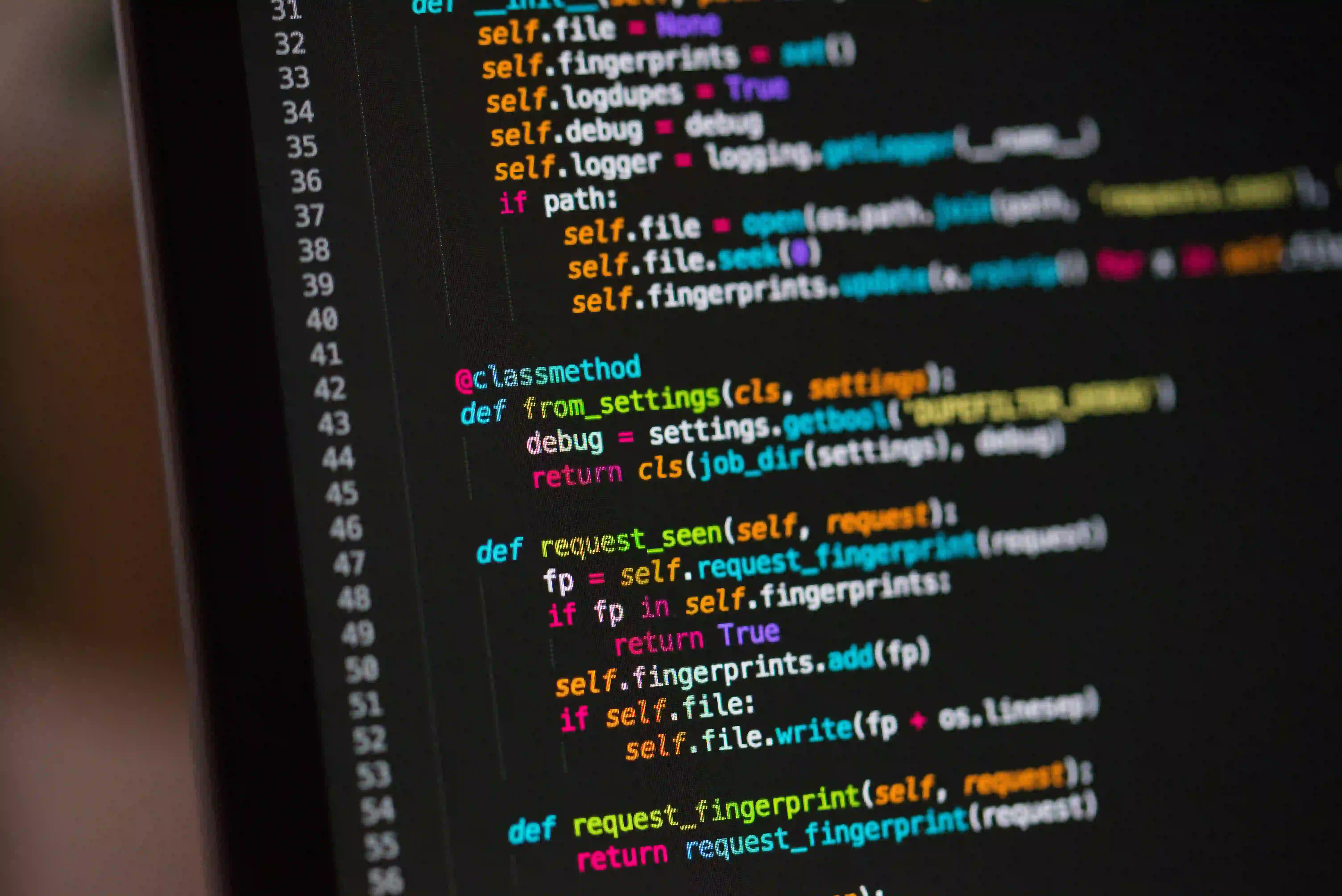
Mastering Gradient Fills: Enhance JavaFX with SVG Patterns
JavaFX has become one of the go-to platforms for building rich client applications in Java. Among its many features, the use of gradient fills can dramatically enhance the aesthetics of your application. In this blog post, we will explore how you can leverage SVG (Scalable Vector Graphics) patterns to create stunning visual effects in JavaFX, specifically focusing on gradient fills.
What Are SVG Patterns?
SVG patterns are reusable components that can define shapes and textures in your vector graphics. They can be used to create complex designs without pixelation issues, as they are inherently scalable. By transforming SVG patterns into gradients, you can add depth and dimension to your applications.
For an in-depth exploration of transforming SVG patterns, check out the article Transforming SVG Patterns into Stunning Gradient Fills.
Why Use Gradient Fills?
Gradient fills provide various benefits:
- Visual Appeal: Gradients can enhance the visual appeal of your application, making it more engaging to users.
- Focus Attention: They can guide the user’s focus to important UI elements.
- Depth and Dimension: Gradients add a sense of depth, making a flat design feel more dynamic.
Implementing Gradient Fills in JavaFX
To implement gradient fills in JavaFX, one common approach is to use the LinearGradient
and RadialGradient
classes. These classes allow you to create both linear and radial gradient effects. Below, we will demonstrate how to implement these gradients.
Setting Up Your JavaFX Application
First, ensure you have the JavaFX library set up. If you're using a build tool like Maven, ensure you include the necessary dependencies. Here is a simple setup:
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>19.0.0</version>
</dependency>
Creating a Linear Gradient Fill
Linear gradients transition colors in a straight line. It can be particularly useful for backgrounds or button styles.
Here’s how you can implement a linear gradient fill in your JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.LinearGradient;
import javafx.scene.paint.Color;
import javafx.scene.paint.CycleMethod;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class LinearGradientExample extends Application {
@Override
public void start(Stage primaryStage) {
Rectangle rectangle = new Rectangle(400, 300);
// Creating a linear gradient
LinearGradient linearGradient = new LinearGradient(
0, 0, 1, 1, // Start and end points of the gradient
true, // proportional
CycleMethod.NO_CYCLE, // cycle behavior
new Stop(0, Color.RED), // Start Stop
new Stop(1, Color.BLUE) // End Stop
);
rectangle.setFill(linearGradient);
StackPane root = new StackPane();
root.getChildren().add(rectangle);
Scene scene = new Scene(root, 400, 300);
primaryStage.setTitle("Linear Gradient Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Breakdown
- Stop: Represents a color at a particular point in the gradient. In our example, we define two stops: red at 0% and blue at 100%.
- CycleMethod: It defines how colors transition. We have chosen
NO_CYCLE
, meaning the gradient does not repeat.
This simple example shows how to create a linear gradient filling a rectangle. Gradients could also be applied to Circle
, Polygon
, etc., enabled through the same setFill()
method.
Creating a Radial Gradient Fill
Radial gradients radiate from a central point. They can be ideal for creating effects like buttons or spotlight highlights. Here is how to implement it:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.RadialGradient;
import javafx.scene.paint.Color;
import javafx.scene.paint.CycleMethod;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class RadialGradientExample extends Application {
@Override
public void start(Stage primaryStage) {
Circle circle = new Circle(200);
// Creating a radial gradient
RadialGradient radialGradient = new RadialGradient(
0, 0, // Focus: no offset
0.5, 0.5, // Center at 50% of the circle
0.5, // Radius
true, // proportional
CycleMethod.REPEAT, // cycle behavior
new Stop(0, Color.GREEN), // Start Stop
new Stop(1, Color.YELLOW) // End Stop
);
circle.setFill(radialGradient);
StackPane root = new StackPane();
root.getChildren().add(circle);
Scene scene = new Scene(root, 400, 400);
primaryStage.setTitle("Radial Gradient Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Breakdown
- The
RadialGradient
allows you to set a central point and a focus point, determining how the colors will spread out from the center. - The
CycleMethod.REPEAT
allows the colors to repeat with every cycle.
Integrating SVG Patterns
Now, let’s integrate SVG patterns to create custom gradient fills. To use SVG in JavaFX, convert the SVG data into appropriate JavaFX shapes, and utilize their fill properties. This follows the idea presented in the previously referenced article.
Here’s a simplified example of how you might do this:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.ImagePattern;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import javafx.scene.image.Image;
public class SvgPatternExample extends Application {
@Override
public void start(Stage primaryStage) {
Rectangle rectangle = new Rectangle(400, 300);
Image svgImage = new Image("path/to/your/svg/pattern.svg"); // Load your SVG as an Image
rectangle.setFill(new ImagePattern(svgImage));
StackPane root = new StackPane();
root.getChildren().add(rectangle);
Scene scene = new Scene(root, 400, 300);
primaryStage.setTitle("SVG Pattern Fill Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Breakdown
- We load the SVG image using
Image
class. - The
ImagePattern
class creates a fill pattern from the SVG image, effectively turning our SVG into background texture.
Best Practices for Using Gradients in JavaFX
- Avoid Overcomplicating: Keep gradients simple for cleaner interfaces.
- Optimize Performance: Use gradients judiciously; excessive use can impact rendering performance.
- Test on Devices: Gradients can look different on various devices; ensure they maintain their desired appearance.
My Closing Thoughts on the Matter
Incorporating gradient fills into your JavaFX applications using SVG patterns opens a whole new realm of design possibilities. By creating visually captivating interfaces, you not only improve user experience but also elevate your applications' overall aesthetics.
As you experiment with these techniques, consider the user experience at the forefront. Colors, transitions, and patterns should communicate and enhance your application's purpose. For more advanced techniques in using SVG patterns, revisit the ideas covered in Transforming SVG Patterns into Stunning Gradient Fills.
By mastering the art of gradient fills, you create not just applications but engaging experiences that resonate with users. Happy coding!