Enhancing Java Graphics with SVG Gradient Fills
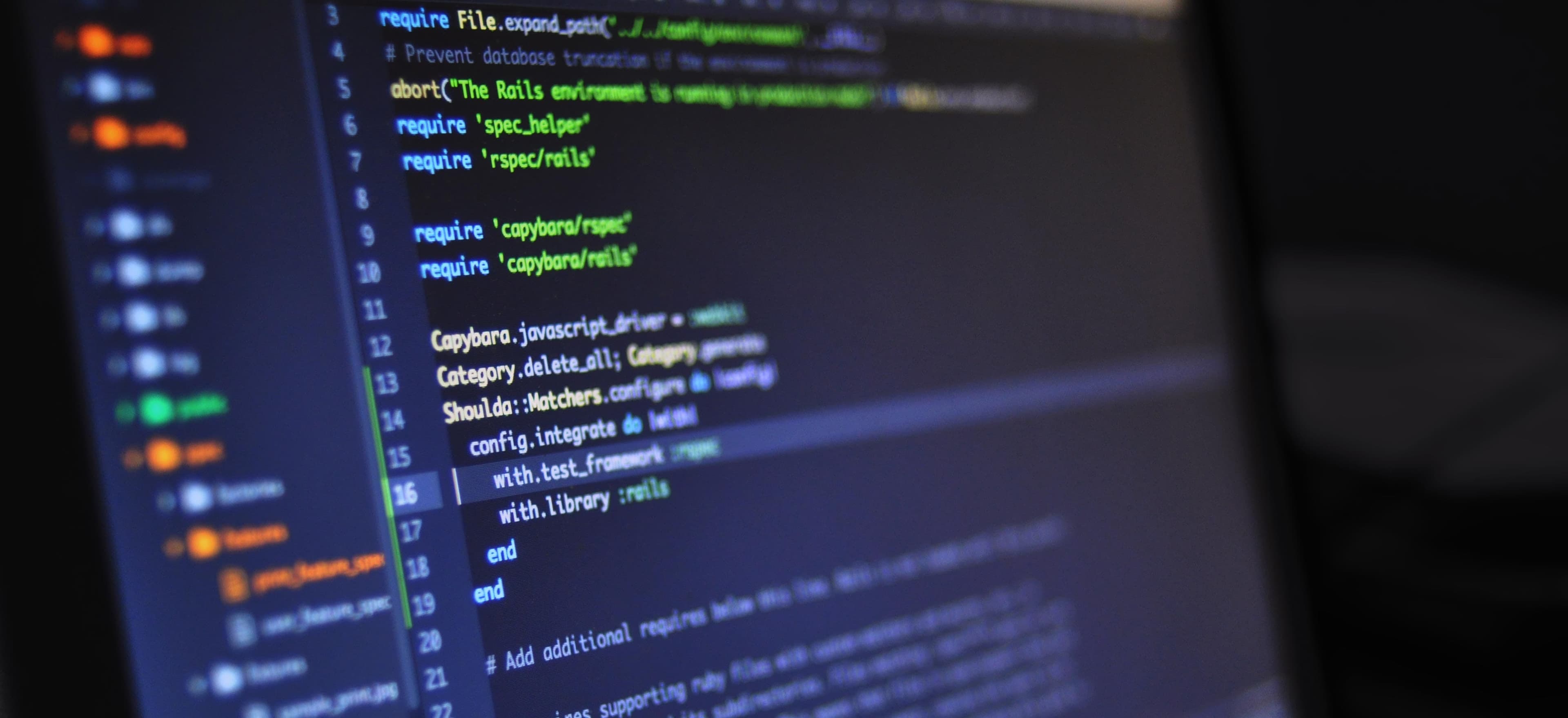
- Published on
Enhancing Java Graphics with SVG Gradient Fills
In the realm of graphics programming, Java offers a rich array of features that allow developers to create stunning visual representations. With libraries such as Java 2D, you can generate and manipulate images and shapes. However, many developers are unaware of how to elevate their graphics to new heights by integrating SVG (Scalable Vector Graphics) gradient fills into their Java applications.
This blog post will guide you through the process of enhancing your Java graphics using SVG gradient fills, enabling you to create visually appealing content. We will also discuss the integration of SVG into Java applications, and you'll gain insights into the common pitfalls and best practices associated with this approach.
Understanding SVG Gradient Fills
SVG is a powerful vector graphics format that enables you to define shapes, paths, and more using XML syntax. One of its most compelling features is the ability to create gradient fills. Gradients can enhance the visual appeal of graphics by providing depth and texture. In SVG, there are two primary types of gradients: linear gradients and radial gradients.
Linear Gradient
A linear gradient creates a transition between colors along a straight line. Here's an example of how to define a linear gradient in SVG:
<linearGradient id="myLinearGradient" x1="0%" y1="0%" x2="100%" y2="100%">
<stop offset="0%" style="stop-color:#ff0000; stop-opacity:1" />
<stop offset="100%" style="stop-color:#0000ff; stop-opacity:1" />
</linearGradient>
In this snippet, we define a linear gradient that transitions from red (#ff0000
) to blue (#0000ff
). The stop
elements determine where each color begins and ends within the gradient.
Radial Gradient
A radial gradient creates a transition of colors radiating from a central point. Here’s an example:
<radialGradient id="myRadialGradient" cx="50%" cy="50%" r="50%">
<stop offset="0%" style="stop-color:#ffff00; stop-opacity:1" />
<stop offset="100%" style="stop-color:#00ff00; stop-opacity:1" />
</radialGradient>
In this example, the radial gradient transitions from yellow (#ffff00
) at the center to green (#00ff00
) at the edges.
Integrating SVG Gradients in Java
To make use of SVG gradients in a Java application, you'll likely need to use an external library since Java does not natively understand SVG. One such library is Apache Batik, which offers powerful capabilities for reading and rendering SVG files within Java applications.
Setting Up Your Environment
First, ensure you have the Batik library in your project's build path. You can include it via Maven with the following dependency:
<dependency>
<groupId>org.apache.xmlgraphics</groupId>
<artifactId>batik-swing</artifactId>
<version>1.14</version>
</dependency>
After adding Batik to your project, you can proceed to create your SVG graphics.
Creating and Rendering SVG
Here’s a simplified example of how to create an SVG image with a gradient fill and render it in a Java application:
import org.apache.batik.swing.JSVGCanvas;
import org.apache.batik.transcoder.TranscoderException;
import javax.swing.*;
import java.awt.*;
import java.io.File;
import java.io.IOException;
public class SVGGradientExample {
public static void main(String[] args) {
SwingUtilities.invokeLater(SVGGradientExample::createAndShowGUI);
}
private static void createAndShowGUI() {
JFrame frame = new JFrame("SVG Gradient Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JSVGCanvas svgCanvas = new JSVGCanvas();
String svgFilePath = "path/to/your/svg_file.svg"; // Replace with your actual SVG file path
try {
svgCanvas.setURI(new File(svgFilePath).toURI().toString());
} catch (Exception e) {
e.printStackTrace();
}
frame.getContentPane().add(svgCanvas, BorderLayout.CENTER);
frame.setSize(800, 600);
frame.setVisible(true);
}
}
Explanation of the Code
In this code:
- We import necessary Batik libraries to create and render SVG.
- The
createAndShowGUI()
method initializes a JFrame and adds anJSVGCanvas
. - The
svgFilePath
specifies the path to your SVG file containing gradients. - We set the URI of the canvas to the SVG file, allowing it to be rendered as part of the Swing application.
A Closer Look at SVG Gradients
To get the most out of SVG gradients, you must understand how they interact with various elements in your graphics. For example, you can apply gradients directly to shapes like rectangles, circles, or any custom path. This flexibility empowers you to create unique visual elements in your Java application.
Consider this simple example of drawing a rectangle with a linear gradient fill:
import javax.swing.*;
import java.awt.*;
public class GradientRectangle extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
// Define gradient paint
GradientPaint gradientPaint = new GradientPaint(0, 0, Color.RED, 100, 100, Color.BLUE);
g2d.setPaint(gradientPaint);
g2d.fillRect(50, 50, 200, 100);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Gradient Rectangle");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(new GradientRectangle());
frame.setSize(400, 300);
frame.setVisible(true);
}
}
Key Code Insights
- This example utilizes a
GradientPaint
object, which is an easy way to create linear gradients in Java without SVG. - The
fillRect
method applies the gradient to a rectangle. With this implementation, you'll see how easily Java handles gradients.
Best Practices for Using SVG in Java Graphics
-
Optimize SVG Files: Large SVG files can slow down rendering times. Use tools like SVGOMG to optimize your SVG files without losing quality.
-
Familiarize with Path Commands: Understanding how SVG paths work will allow you to leverage the full power of SVG graphics, including complex shapes and effects.
-
Utilize External Resources: For extensive use of SVGs in your application, consider leveraging existing libraries and resources. You may find articles, like Transforming SVG Patterns into Stunning Gradient Fills, which offer additional insights.
-
Test on Multiple Platforms: SVG rendering may differ across platforms. Test your application on different setups to ensure consistency.
-
Keep It Simple: When experimenting with complex gradients, remember to keep the design simple for faster rendering.
The Last Word
Integrating SVG gradient fills into Java graphics can significantly enhance the visual appeal of your applications. By understanding SVG basics and utilizing libraries like Apache Batik effectively, you can create compelling graphical elements that set your applications apart.
Whether you're an experienced developer or just starting, integrating gradients can open a new dimension of creativity for your Java projects. Start exploring SVG today, and watch your applications come to life with breathtaking visuals!