Mastering State Management in Java Apps with Redux
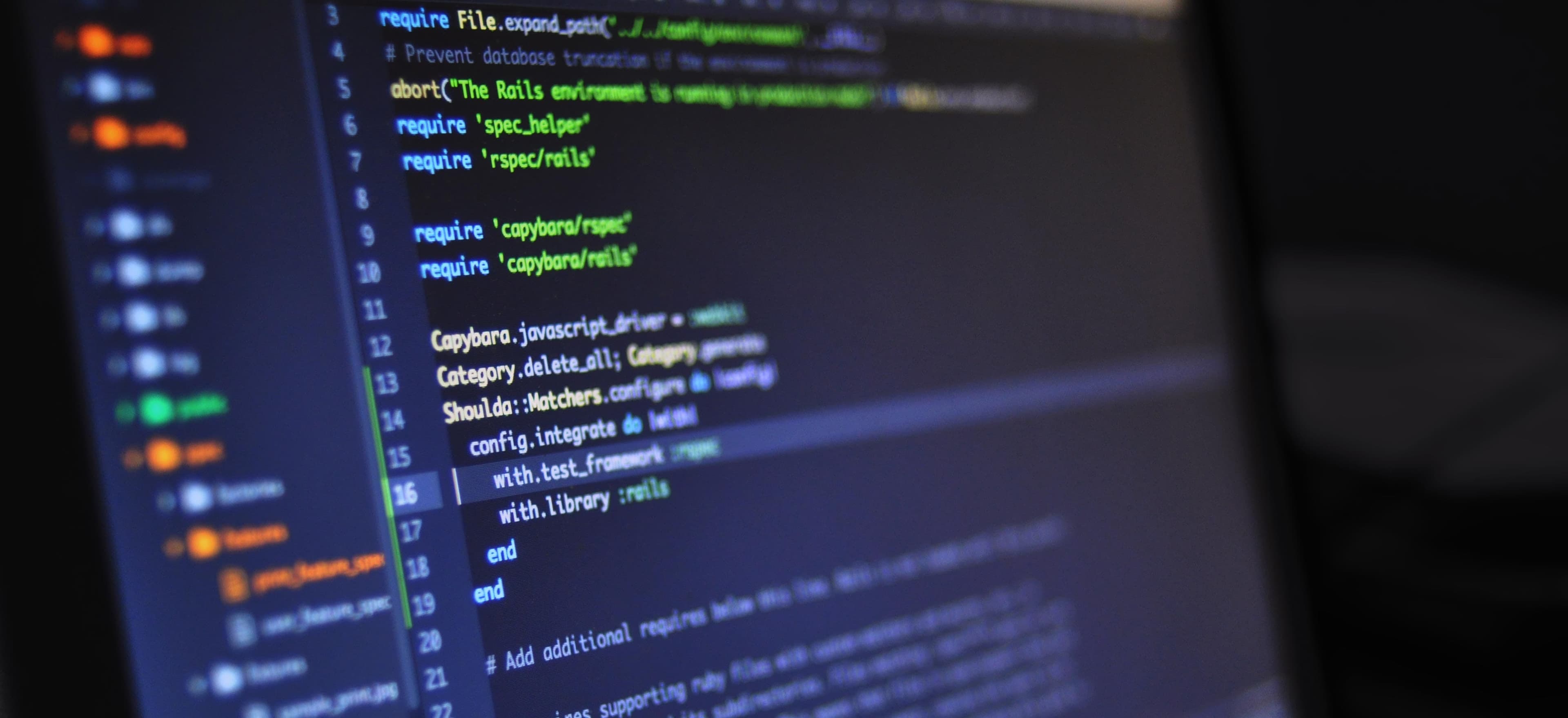
- Published on
Mastering State Management in Java Apps with Redux
State management is a cornerstone of modern app development, especially for Java applications that incorporate complex user interfaces. Redux, while originally created for JavaScript, has emerged as a powerful tool for state management in Java applications as well. This article delves into the principles of Redux, how to implement it in Java, and why it’s crucial for managing state effectively. If you’re interested in a deep dive into Redux in another context, check out "Taming Redux: Simplify State Management in Apps" at infinitejs.com/posts/simplify-redux-state-management.
What is Redux?
Redux is a predictable state container for JavaScript apps, and it can be adapted for Java. It operates on a simple principle: single source of truth. This means maintaining all application state in a single, centralized store. The state of your application is immutable, which allows for greater predictability and easier debugging.
Benefits of Using Redux
- Centralized State Management: All state is managed in a single store, making it easier to track changes.
- Predictability: Since state cannot be mutated directly, it’s easier to understand how and when changes occur.
- Maintainability: Clear separation of concerns allows different parts of your app to focus on their own functionality.
- Time-travel Debugging: The immutability of state allows for powerful debugging tools where you can "travel" through the state history.
Setting Up Redux for Java Applications
To use Redux in your Java applications, you can utilize libraries such as ReduxJava. This library provides a Java implementation inspired by Redux principles and gives you the tools to effectively manage state.
Installing ReduxJava
You can add ReduxJava to your project by including the following dependency in your pom.xml
if you're using Maven:
<dependency>
<groupId>com.github.capricorn86</groupId>
<artifactId>redux-java</artifactId>
<version>1.0</version>
</dependency>
Understanding Actions and Reducers
In Redux, state changes are initiated by actions. An action is a plain Java object that contains a type and an optional payload. Reducers, on the other hand, are pure functions that take the current state and action as arguments and return the next state.
Example Action
Here’s a simple action to add a user:
public class AddUserAction {
private final String type = "ADD_USER";
private final User user;
public AddUserAction(User user) {
this.user = user;
}
public String getType() {
return type;
}
public User getUser() {
return user;
}
}
Why this matters:
- The action includes a clear type, which is crucial for identifying actions in the reducer logic.
- The payload (the user object) carries the necessary data to update the state.
Example Reducer
Reducers specify how the application's state changes in response to actions:
public class UserReducer {
public State reduce(State state, Action action) {
if ("ADD_USER".equals(action.getType())) {
List<User> updatedUsers = new ArrayList<>(state.getUsers());
updatedUsers.add(action.getUser());
return new State(updatedUsers);
}
return state;
}
}
Why this matters:
- This reducer listens for the
ADD_USER
action and updates the user list accordingly. - It maintains immutability by returning a new state instance instead of modifying the existing one.
Integrating Redux with Your Java Application
Now that we understand the core concepts and how to define actions and reducers, let’s see how we can integrate Redux into an application.
Setting Up the Store
To manage your state effectively, you'll need to create a Redux store:
public class Store {
private State state;
private final List<Middleware> middlewares;
public Store(Reducer reducer, State initialState) {
this.state = initialState;
this.middlewares = new ArrayList<>();
}
public State getState() {
return state;
}
public void dispatch(Action action) {
state = reducer.reduce(state, action);
}
}
Why this matters:
- The
Store
class centralizes the application state, provides the current state on request, and also processes dispatched actions.
Middleware for Asynchronous Actions
Redux middleware can intercept actions before they reach the reducer. This is especially useful for handling asynchronous tasks like API calls.
Here’s a simple example of middleware that logs actions:
public class LoggerMiddleware implements Middleware {
public void intercept(Action action) {
System.out.println("Dispatching action: " + action.getType());
}
}
Why this matters:
- Logging actions helps in debugging the flow of state changes, making it easier to track issues.
Example: Building a Simple User Management App
Let's develop a simple user management application using Redux for state management. This app will allow adding users and displaying them.
Define the User Entity
You'll need a User
class to represent each user:
public class User {
private final String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Create the Initial State
Define the initial state of your application:
public class State {
private final List<User> users;
public State(List<User> users) {
this.users = users;
}
public List<User> getUsers() {
return users;
}
}
Instantiate Store and Reducers
Now let’s tie everything together in a main class:
public class UserManagementApp {
public static void main(String[] args) {
State initialState = new State(new ArrayList<>());
Store store = new Store(new UserReducer(), initialState);
// Add new users
store.dispatch(new AddUserAction(new User("Alice")));
store.dispatch(new AddUserAction(new User("Bob")));
// Display users
store.getState().getUsers().forEach(user -> System.out.println(user.getName()));
}
}
Explanation of the Implementation
- The main method initializes the store with a reducer and an empty state.
- Two users are dispatched as actions to the store, showcasing the simplicity of state modification via actions.
- Finally, it retrieves and prints the list of users, demonstrating how easy it is to access the centralized state.
Final Thoughts
Mastering state management in your Java applications using Redux can significantly improve your application's structure. It provides predictability, maintainability, and a clearer understanding of state changes. By utilizing actions, reducers, and middleware, your applications become easier to debug and manage.
For further reading on state management, do not forget to check out the article "Taming Redux: Simplify State Management in Apps" at infinitejs.com/posts/simplify-redux-state-management.
By leveraging the core principles of Redux in your Java applications, you can create more robust, scalable, and maintainable software solutions. This guide should set you on the right path toward mastering state management with Redux in the Java ecosystem. Happy coding!