Avoiding Common Java Setup Pitfalls for Beginners
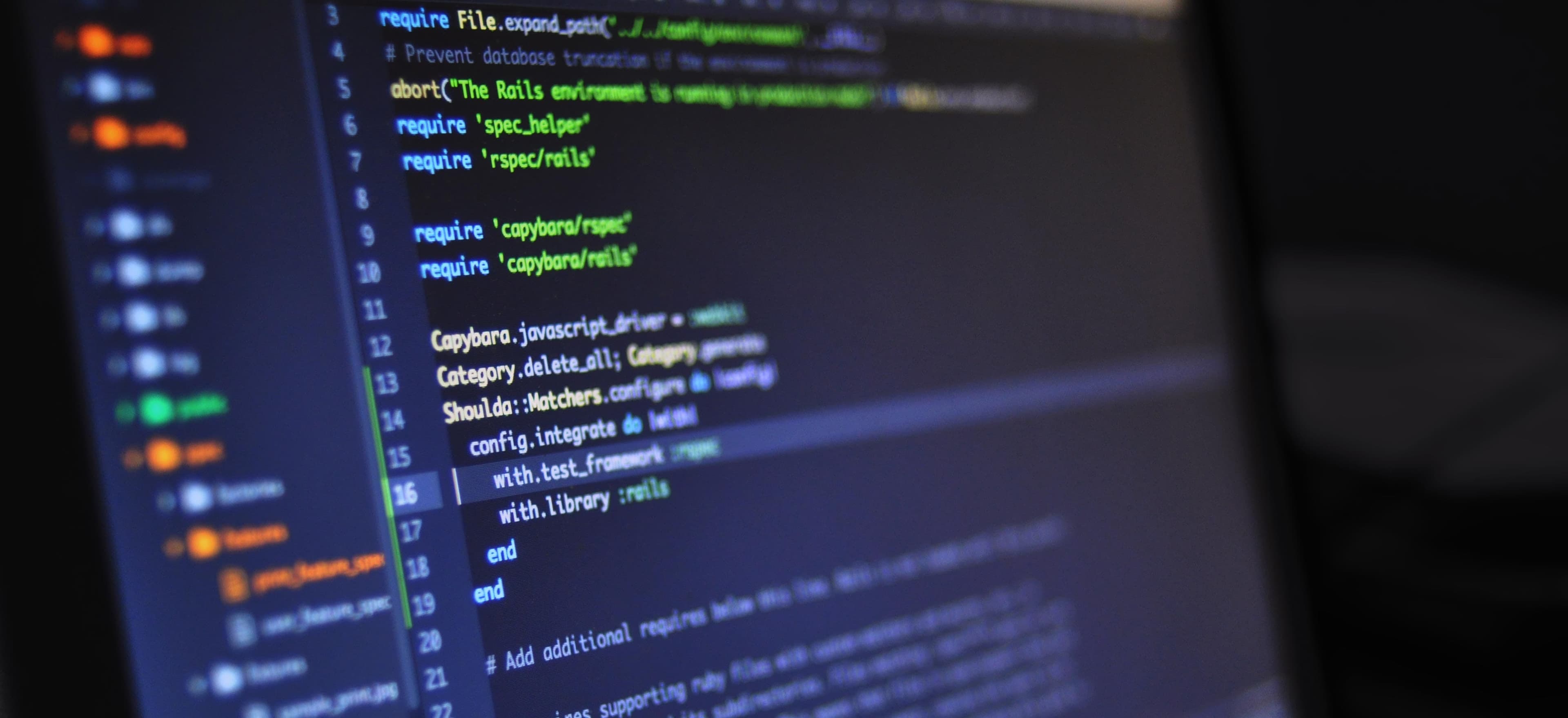
- Published on
Avoiding Common Java Setup Pitfalls for Beginners
Setting up Java for development can be both an exciting and daunting experience. For beginners, the initial hurdles can deter them from embracing this powerful programming language. But fear not—this guide aims to provide practical and straightforward solutions to common setup pitfalls you may encounter. As we dive into the specifics, we'll maintain a balanced rhythm between detailed discussion and concise guidance.
Understanding the Java Environment
Before you can start writing Java code, you need to ensure you have the right tools in place. This includes the Java Development Kit (JDK), a suitable Integrated Development Environment (IDE), and proper configuration.
What is the JDK?
The JDK is a software development kit used to develop Java applications. It includes the Java Runtime Environment (JRE), compiler (javac), and other necessary tools. You can download it from the official Oracle website.
Why do you need the JDK?
Without the JDK, you cannot compile or run Java programs, which defeats the purpose of learning Java.
Choosing an IDE
While you can write Java code in plain text files, using an IDE significantly enhances productivity. Popular IDEs for Java development include:
- Eclipse: A popular open-source IDE with an extensive ecosystem of plugins.
- IntelliJ IDEA: Known for its advanced code completion and intelligent refactoring support.
- NetBeans: A lightweight, open-source IDE that supports multiple languages.
Why is an IDE important?
An IDE provides features like syntax highlighting, debugging tools, and project management, making the coding experience smoother and more enjoyable.
Installation Steps
1. Installing the JDK
Let's walk through the process of installing the JDK step-by-step:
Step 1: Download the JDK
Go to the Oracle JDK download page, select your operating system, and download the installer.
Step 2: Install
Follow the installation prompts. It's usually a straightforward process—just accept the terms and click through the steps.
Step 3: Set Environment Variables
Setting up the PATH variable allows the system to find the Java executables. Here’s how to do it:
For Windows:
- Right-click on
This PC
and selectProperties
. - Click on
Advanced system settings
. - Click
Environment Variables
. - Under
System Variables
, find thePath
variable and edit it. - Add the path to the
bin
directory of your JDK installation (e.g.,C:\Program Files\Java\jdk-11\bin
).
For macOS/Linux: You can set the PATH variable in your shell profile file (.bashrc, .bash_profile, or .zshrc).
export JAVA_HOME=/Library/Java/JavaVirtualMachines/jdk-11.jdk/Contents/Home
export PATH=$JAVA_HOME/bin:$PATH
Why set the PATH?
This makes it easier to run Java commands (like java
and javac
) from the command line, contributing to a seamless developer experience.
2. Installing Your IDE
Download and install your IDE of choice. Each IDE will have its own specific installation instructions, but they are generally user-friendly.
Example: Installing IntelliJ IDEA
- Go to the IntelliJ website.
- Download the Community edition which is free.
- Follow the installation instructions for your operating system.
Why use IntelliJ IDEA?
IntelliJ IDEA is well-known for its powerful features that help simplify the coding process, making it an excellent choice for beginners and advanced users alike.
Creating Your First Java Project
With the JDK installed and your IDE ready, it’s time to create your first Java project.
- Open IntelliJ IDEA.
- Select
New Project
. - Choose
Java
, ensure the correct JDK is selected, and clickNext
. - Name your project and click
Finish
.
Basic Java Code Example
Let’s write your first Java program now—a classic "Hello, World!" example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Why do we use System.out.println
?
This command prints the text in parentheses to the console, allowing you to see the output of your code.
Running Your Program
You can run your program directly from the IDE.
- Right-click on the file containing your
main
method. - Select
Run HelloWorld.main()
.
If everything is set up correctly, you should see "Hello, World!" printed in the console.
Common Setup Issues and Solutions
Despite following instructions, issues may arise during setup. Below are common pitfalls and how to overcome them:
1. JAVA_HOME Not Set
If you receive an error stating that Java cannot be found, ensure that JAVA_HOME
is set correctly.
Solution: Revisit the environment variables and confirm the path points to the JDK directory.
2. Version Conflicts
Conflicts occur when multiple Java versions are installed. This can lead to confusion over which version is being used.
Solution: Uninstall older versions of Java or manage multiple versions using tools like JEnv.
3. Poor IDE Performance
Beginners may experience sluggishness or lag when using an IDE.
Solution: Increase memory allocation for the IDE by adjusting its configuration files appropriately.
Closing the Chapter
Setting up Java can have its challenges, but staying informed about the common pitfalls allows you to navigate the installation process effectively. By following the outlined steps and recommendations, you'll be well on your way to a successful Java programming experience.
If you're venturing into another environment, consider checking out the article Jumpstart Your Node Journey: Solving First-Time Setup Woes for tips that could further ease your transitions.
Additional Resources
By understanding the setup process and avoiding common missteps, you are not just preparing to code—you are laying a solid foundation for a future in software development. Happy coding!
Checkout our other articles