Streamlining Subdomains: A Java Approach to Redirection
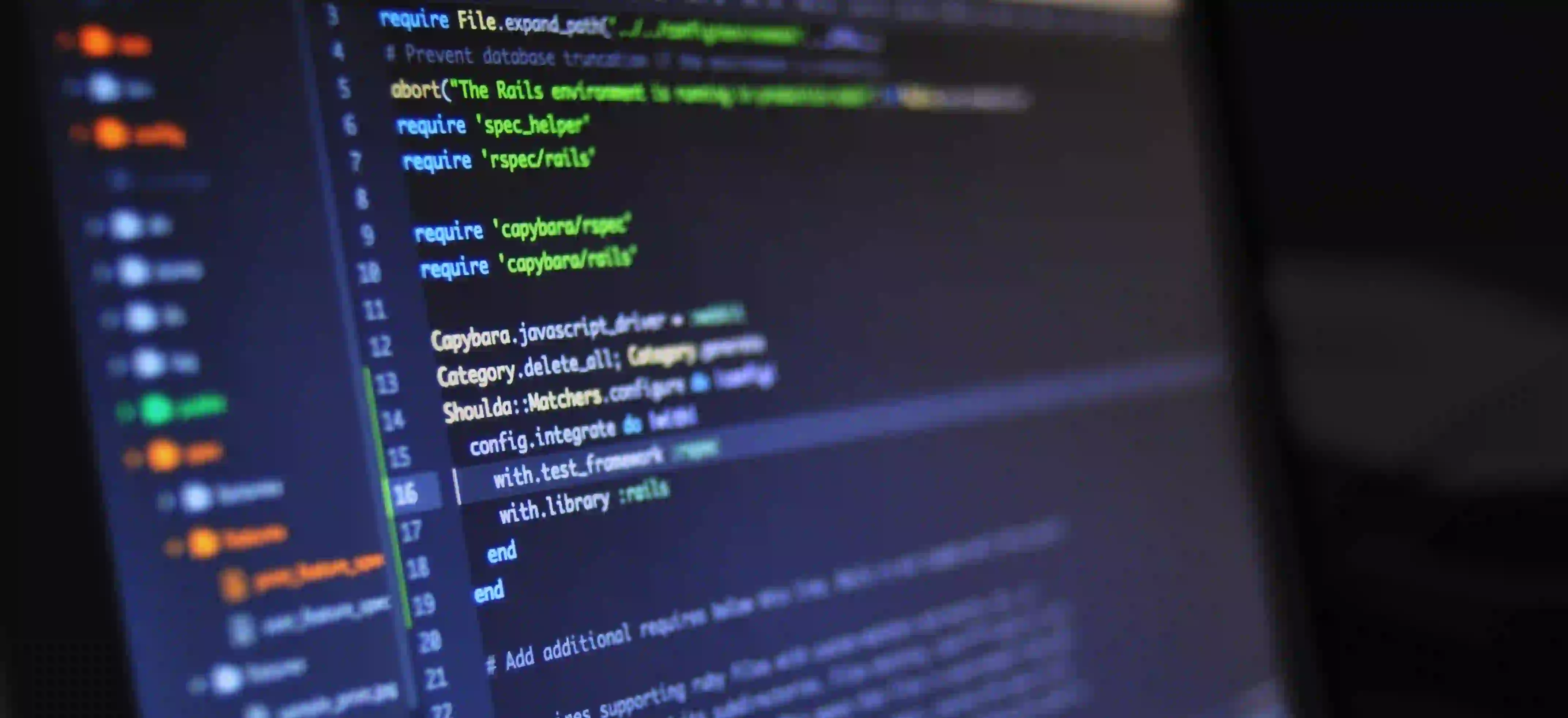
Streamlining Subdomains: A Java Approach to Redirection
Managing multiple subdomains can often lead to complexity, especially in managing URLs and directing traffic correctly. An efficient way to handle this is by redirecting all subdomains to a single subdomain. This approach not only improves user experience but also enhances your website's SEO. In this blog post, we will explore how to implement subdomain redirection using Java, making sure you'll streamline your web infrastructure.
Why Redirect Subdomains to One Main Subdomain?
Redirecting subdomains to one specific subdomain has several benefits:
-
Improved SEO: Search engines favor a consolidated domain structure. This means that instead of spreading link equity and authority across multiple subdomains, all of it focuses on one domain.
-
Simplicity for Users: Users may not remember the specific subdomains but will easily remember the main subdomain. This reduces confusion and directs users to the intended destination.
-
Enhanced Web Analytics: By concentrating traffic on one subdomain, it becomes easier to gauge performance and analyze visitor behavior.
For a deeper understanding of URL redirection concepts, take a look at the article titled Redirecting All Subdomains to One Subdomain.
Setting Up Your Java Environment
Before we dive into the code, make sure you have a working Java environment. You can install Java from Oracle's website or use an IDE like IntelliJ IDEA or Eclipse to ease the development process.
Required Libraries
We will use the Spring Boot
framework for this implementation as it simplifies the configuration and deployment of web applications. To get started, add the following dependencies to your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Main Java Class for Redirection
Here’s a crucial part of the redirection process. We need a controller that listens for requests and decides where the traffic should go.
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.view.RedirectView;
@Controller
public class SubdomainRedirectController {
@RequestMapping(value = "/")
public RedirectView redirectSubdomain(@RequestParam(value = "host", required = false) String host) {
String targetDomain = "https://www.main-subdomain.com"; // Change this to your main subdomain
if (host != null && !host.equals("www.main-subdomain.com")) {
String redirectUrl = targetDomain;
return new RedirectView(redirectUrl);
}
// If user is already on the main subdomain, just show the home page
return new RedirectView(targetDomain + "/home");
}
}
Explanation of the Code
-
Controller Class: We define a Spring Controller named
SubdomainRedirectController
. This is responsible for handling HTTP requests. -
Redirect Method: The method
redirectSubdomain
checks the request'shost
parameter. If it is not the main subdomain, it prepares a redirect to the definedtargetDomain
. -
RedirectView: The
RedirectView
class is used to specify the new URL to which the request will be redirected. -
Flexible Redirection: This method can easily be adjusted to redirect to different subdomains based on criteria defined in your application logic.
Handling Different Subdomains
In cases where you have multiple subdomains that need to be redirected to one main subdomain, you may want to improve the logic further. Here’s how to achieve this:
@RequestMapping(value = "/")
public RedirectView redirectSubdomain(@RequestParam(value = "host", required = false) String host) {
String targetDomain = "https://www.main-subdomain.com"; // Change this to your main subdomain
// Logic for redirecting subdomains to main subdomain
if (host != null) {
switch (host) {
case "sub1.yourdomain.com":
case "sub2.yourdomain.com":
case "sub3.yourdomain.com":
return new RedirectView(targetDomain);
default:
break;
}
}
return new RedirectView(targetDomain + "/home");
}
Explanation of Altered Code
The switch statement allows for easy modification if you have multiple subdomains:
-
Switch Case: The structure checks against various subdomains and simplifies future scalability where adding or modifying subdomain logic becomes less cumbersome.
-
Single Point of Redirection: The logic retains a single point for redirection, making it easy to manage and maintain.
Testing Your Implementation
After you have created your application, it's essential to test the redirection. You can use tools like Postman or your browser to check:
- Enter different subdomain URLs in the address bar.
- Confirm that each subdomain correctly redirects to the specified main subdomain.
- Check the network tab in your browser for HTTP status codes; a 301 (permanent redirect) or 302 (temporary redirect) should appear.
Bringing It All Together
Redirecting all subdomains to a single main subdomain can simplify many aspects of web traffic management. Using Java and Spring Boot, we can set up a straightforward yet robust mechanism to achieve this.
The benefits of improved SEO, clearer user experience, and streamlined analytics all align to create a more effective web presence. If you need more insights into managing your subdomains and their redirection, refer to the complete [Redirecting All Subdomains to One Subdomain] article.
Further Reading
- To gain more knowledge about advanced routing in Spring, check out the official Spring MVC documentation.
- Explore Java best practices with Effective Java to solidify your skills in Java development.
By adopting these practices and leveraging these strategies, you can ensure that your subdomain management becomes not only efficient but also promotes a better overall user experience on your website. Happy coding!