Streamline Your Java App: Redirect Subdomains Efficiently
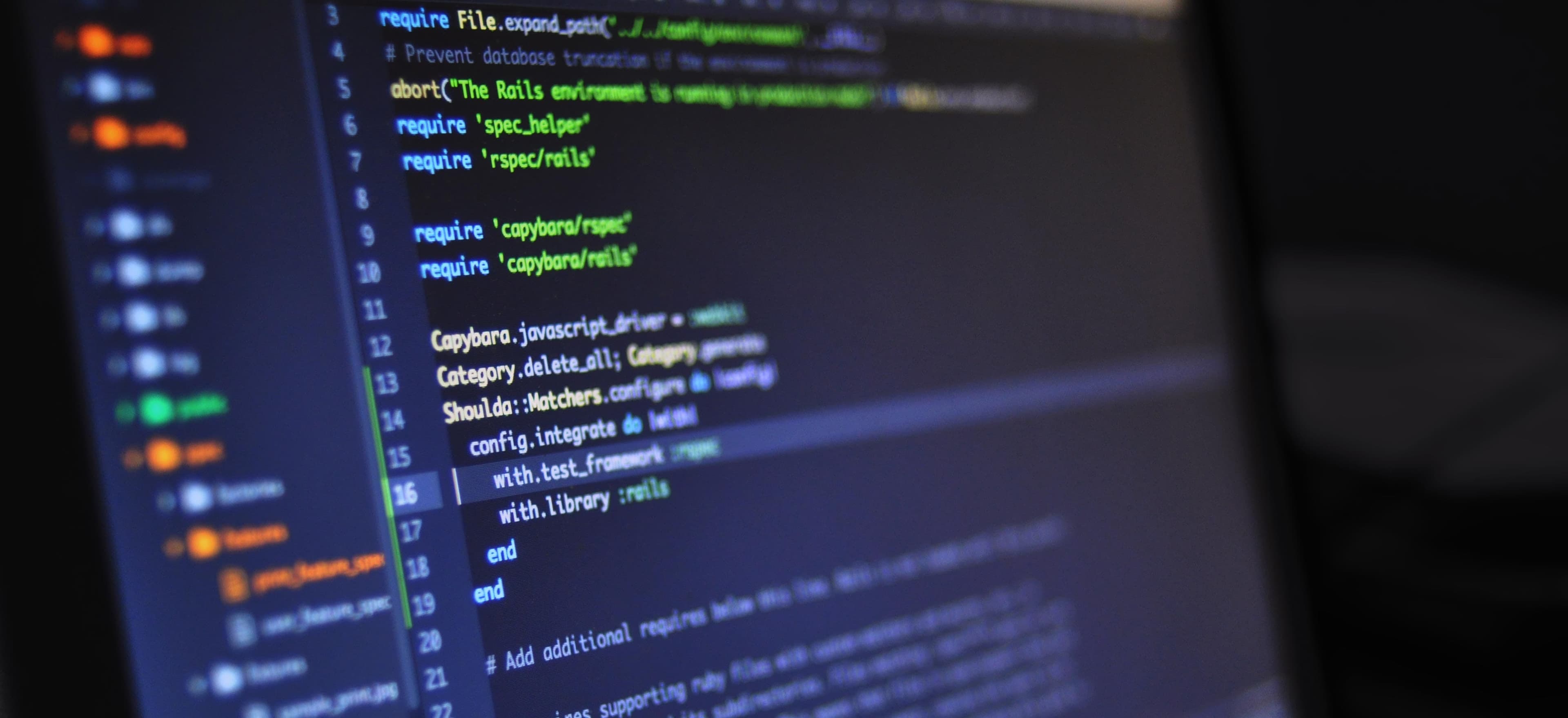
- Published on
Streamline Your Java App: Redirect Subdomains Efficiently
In today’s digital landscape, managing subdomains effectively is crucial for optimizing web applications. Redirection can enhance user experience, consolidate your website's traffic, and improve SEO. In this blog post, we'll discuss how to efficiently redirect all subdomains to a specific subdomain in your Java application, ensuring a seamless experience for your users and a robust architecture for your application.
Why Redirect Subdomains?
Before diving into the code, let’s explore the why of redirecting subdomains:
- User Experience: A consistent URL structure can make navigation clearer for users.
- SEO Benefits: Consolidating your website's traffic can improve your search rankings.
- Simplified Maintenance: Focusing on a single subdomain can simplify application management.
In the existing article, "Redirecting All Subdomains to One Subdomain", the importance of this practice is further highlighted, reinforcing the necessity of clear redirection strategies.
Setting Up Your Java Application
The most common approach to handle URL redirection in a Java web application is through the use of servlet filters. In this section, you will learn how to create a filter that redirects all requests from subdomains to a specified subdomain.
Step 1: Create a Servlet Filter
First, we will create a Servlet Filter that intercepts incoming HTTP requests. This filter checks if the subdomain is not the desired one, and redirects accordingly.
Here's an exemplary code snippet:
import javax.servlet.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class SubdomainRedirectFilter implements Filter {
private static final String TARGET_SUBDOMAIN = "www"; // Change to your desired subdomain
@Override
public void init(FilterConfig filterConfig) throws ServletException {
// Initialization logic, if needed
}
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletRequest httpRequest = (HttpServletRequest) request;
HttpServletResponse httpResponse = (HttpServletResponse) response;
String serverName = httpRequest.getServerName();
// Check if the request originates from the correct subdomain
if (!serverName.equals(TARGET_SUBDOMAIN + ".yourdomain.com")) {
String newURL = "https://" + TARGET_SUBDOMAIN + ".yourdomain.com" + httpRequest.getRequestURI();
httpResponse.sendRedirect(newURL);
return; // Early exit to avoid calling the next filter or resource
}
// Continue the filter chain
chain.doFilter(request, response);
}
@Override
public void destroy() {
// Cleanup logic, if needed
}
}
Explanation of the Code
-
Target Subdomain: The
TARGET_SUBDOMAIN
constant defines the subdomain you want to redirect to. You should adjust it based on your requirements. -
Request Handling: The
doFilter
method intercepts all incoming requests. By checking theserverName
, we determine if the request is coming from a subdomain other than the target. -
Redirection Logic: When the conditions are met, a
sendRedirect
call sends the user to the appropriate URL while preserving the request URI. This helps retain the path users were trying to access. -
Filter Chain: If the user is on the correct subdomain, we allow the request to proceed down the chain by calling
chain.doFilter(...)
.
Step 2: Register the Filter
To activate the filter, you need to register it in your web.xml
:
<filter>
<filter-name>subdomainRedirectFilter</filter-name>
<filter-class>com.yourpackage.SubdomainRedirectFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>subdomainRedirectFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Why Use Servlet Filters?
- Centralized Management: Having a dedicated filter for redirection means that you can easily manage your redirection strategy in one place.
- Efficiency: Filters operate at a low level within the request-response cycle, ensuring that your application’s resources are used efficiently.
Testing Your Implementation
After setting up the filter and registering it, it’s important to test the implementation. Here are some tips for effective testing:
- Browser Testing: Use different browsers to ensure that the redirection works consistently across platforms.
- Subdomain Variations: Test various subdomains to ensure that all incorrectly directed requests are appropriately handled.
Monitor Traffic and SEO Impact
Once deployed, monitoring user traffic patterns will give insights into the efficacy of your redirects. Use SEO tools like Google Analytics to analyze the inbound traffic and assess any changes in user behavior.
Lessons Learned
Redirecting all subdomains to a single subdomain is an efficient practice that not only enhances user experience but also contributes positively to your website’s SEO performance. By implementing a servlet filter in your Java application, you can effortlessly manage this redirection.
If you’re interested in delving deeper into the world of subdomain management and best practices, refer back to the article, "Redirecting All Subdomains to One Subdomain". Understanding the nuances of subdomain handling can further empower you to optimize your Java applications effectively.
By applying the techniques mentioned in this blog post, your applications will not only be more streamlined but also prepared to adapt to future challenges in an ever-evolving digital landscape. Happy coding!
Checkout our other articles