Unlocking Intuition: Java's Mystical Algorithms Explained
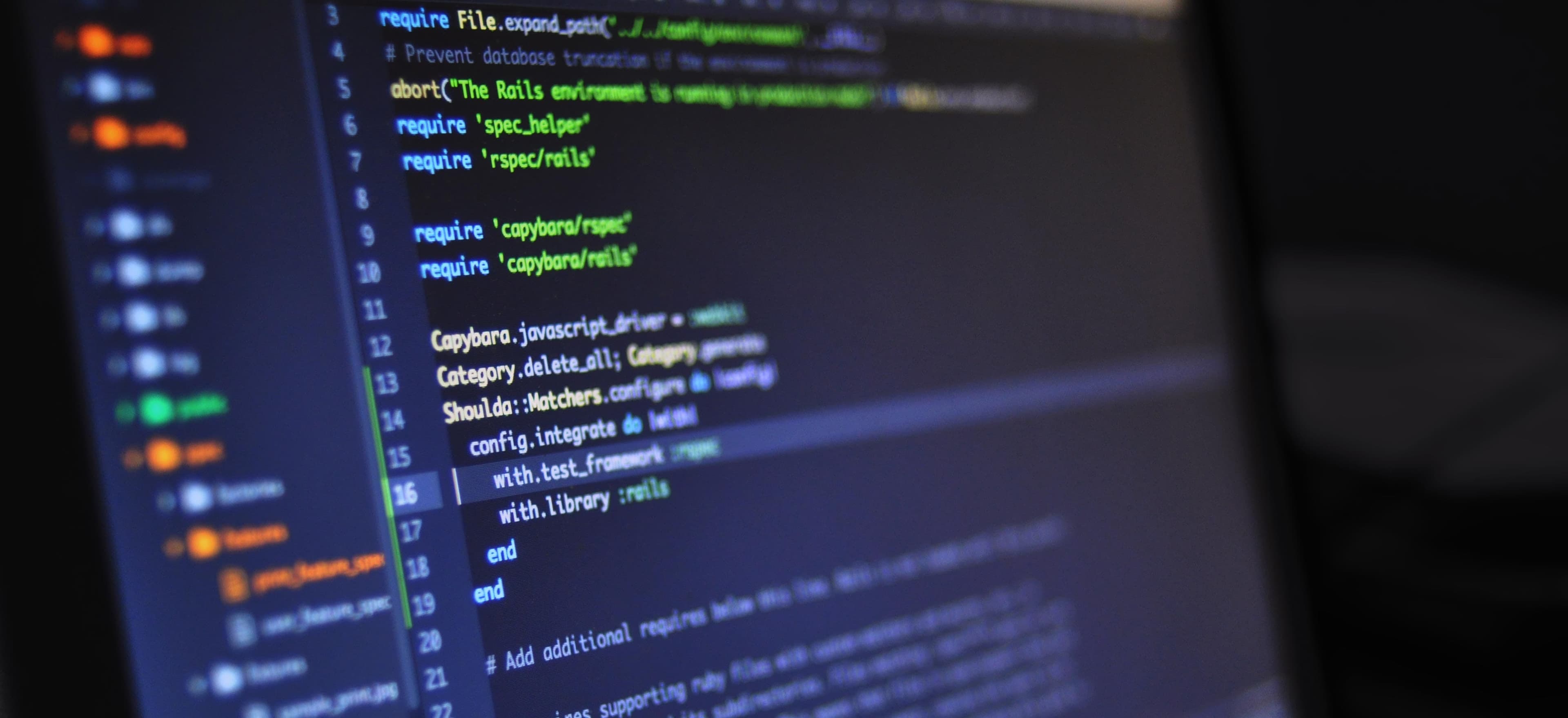
- Published on
Unlocking Intuition: Java's Mystical Algorithms Explained
In a realm where the worlds of technology and intuition converge, the field of algorithms in programming languages like Java stands as a bridge. Algorithms, in essence, are recipes for problem-solving. Much like the art and intuition involved in tarot reading as discussed in the article "Die Geheimnisse des Tarot: Kunst, Intuition und Magie", coding requires an understanding of patterns and instincts. This post will delve into key Java algorithms, unearthing their depths and their application in software development, while keeping that mystical connection to intuition.
Table of Contents
Understanding Algorithms
An algorithm is a step-by-step procedure for calculations. In Java, algorithms are implemented through structured code, but to appreciate their power, one must understand their operational essence. Like the tarot cards, which help to narrate a story through symbols and intuition, algorithms guide the computer through data manipulation and output.
The importance of learning algorithms includes:
- Problem Solving: They teach methods of approaching and breaking down complex challenges.
- Efficiency: A well-chosen algorithm can significantly reduce computational time and resource usage.
- Foundation for Data Structures: Most data structures are built with algorithms in mind.
In the coming sections, we'll explore various algorithms fundamental to Java programming.
Sorting Algorithms
Sorting algorithms arrange the items in a collection, such as arrays or lists, in a specified order (e.g., ascending or descending). They are essential for optimizing search operations and enhancing the performance of other algorithms.
Bubble Sort
Bubble Sort is one of the simplest sorting algorithms. It iterates through a list, repeatedly swapping adjacent elements if they are in the wrong order.
public class BubbleSort {
public static void bubbleSort(int[] arr) {
int n = arr.length;
boolean swapped;
for (int i = 0; i < n - 1; i++) {
swapped = false;
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true;
}
}
// If no two elements were swapped by inner loop, then break
if (!swapped) break;
}
}
}
Why Bubble Sort?
While not the most efficient (O(n²) in worst-case time complexity), understanding Bubble Sort helps grasp fundamental concepts like iteration and condition checking. For small datasets, it is relatively easy to implement and understand.
Quick Sort
Quick Sort is a more advanced, divide-and-conquer sorting algorithm that is more efficient than Bubble Sort for larger datasets. It selects a 'pivot' element and partitions the other elements into two sub-arrays according to whether they are less than or greater than the pivot.
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
// Swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// Swap arr[i + 1] and arr[high] (or pivot)
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
}
Why Quick Sort?
With an average time complexity of O(n log n), Quick Sort is one of the most efficient sorting algorithms. Its usage in real-world applications is extensive, showcasing the value of choosing the right algorithm for the task at hand.
Searching Algorithms
Searching algorithms look for a specific item in a collection. Just as tarot practitioners might look for guidance based on their chosen cards, programmers seek data through these algorithms.
Linear Search
Linear Search, or sequential search, checks each element of a list in order until it finds the target value or reaches the end of the list.
public class LinearSearch {
public static int linearSearch(int[] arr, int key) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == key) {
return i; // Key found at index i
}
}
return -1; // Key not found
}
}
Why Linear Search?
While this method has a time complexity of O(n), it requires no prior arrangement of data and is easiest to implement. It is particularly useful for unsorted data sets or small lists.
Binary Search
Binary Search is a much faster algorithm but can only be applied to sorted arrays. It works by repeatedly dividing the search interval in half, reducing the time complexity to O(log n).
public class BinarySearch {
public static int binarySearch(int[] arr, int key) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == key) {
return mid; // Key found at index mid
}
if (arr[mid] < key) {
left = mid + 1; // Search in the right sub-array
} else {
right = mid - 1; // Search in the left sub-array
}
}
return -1; // Key not found
}
}
Why Binary Search?
Binary Search is preferred when dealing with sorted datasets, as it significantly reduces the number of comparisons needed. It highlights the idea that understanding the structure of your data can lead to much more efficient solutions.
Recursive Algorithms
Recursion is a programming technique where a method calls itself to solve smaller instances of the same problem. It’s akin to a tarot reader using one card to interpret the meaning of subsequent cards in a spread.
Here’s an example of the classic recursive solution to the Fibonacci sequence:
public class Fibonacci {
public static int fibonacci(int n) {
if (n <= 1) return n; // Base case
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
}
Why Recursion?
Recursion simplifies code and can lead to more elegant solutions. However, developers must be cautious of the potential for stack overflow errors and increased time complexity in cases where memoization isn’t applied.
Closing Remarks
In this journey of unraveling Java's algorithms, we’ve unlocked the mystical nature of coding through an understanding of different sorting, searching, and recursive methods. Just as the intricate symbols in tarot guide a reader through realms of intuition, algorithms guide programmers in the realm of technology—helping them solve problems and optimize solutions.
For deeper insights into using intuition and understanding patterns, you can explore the article “Die Geheimnisse des Tarot: Kunst, Intuition und Magie” at auraglyphs.com/post/die-geheimnisse-des-tarot-kunst-intuition-und-magie.
Every algorithm tells a story; understanding them helps unlock the secrets of computational efficiency and problem-solving in Java. What will your next line of code reveal?
Checkout our other articles