Tackling Java Memory Management: Lessons from NodeJS
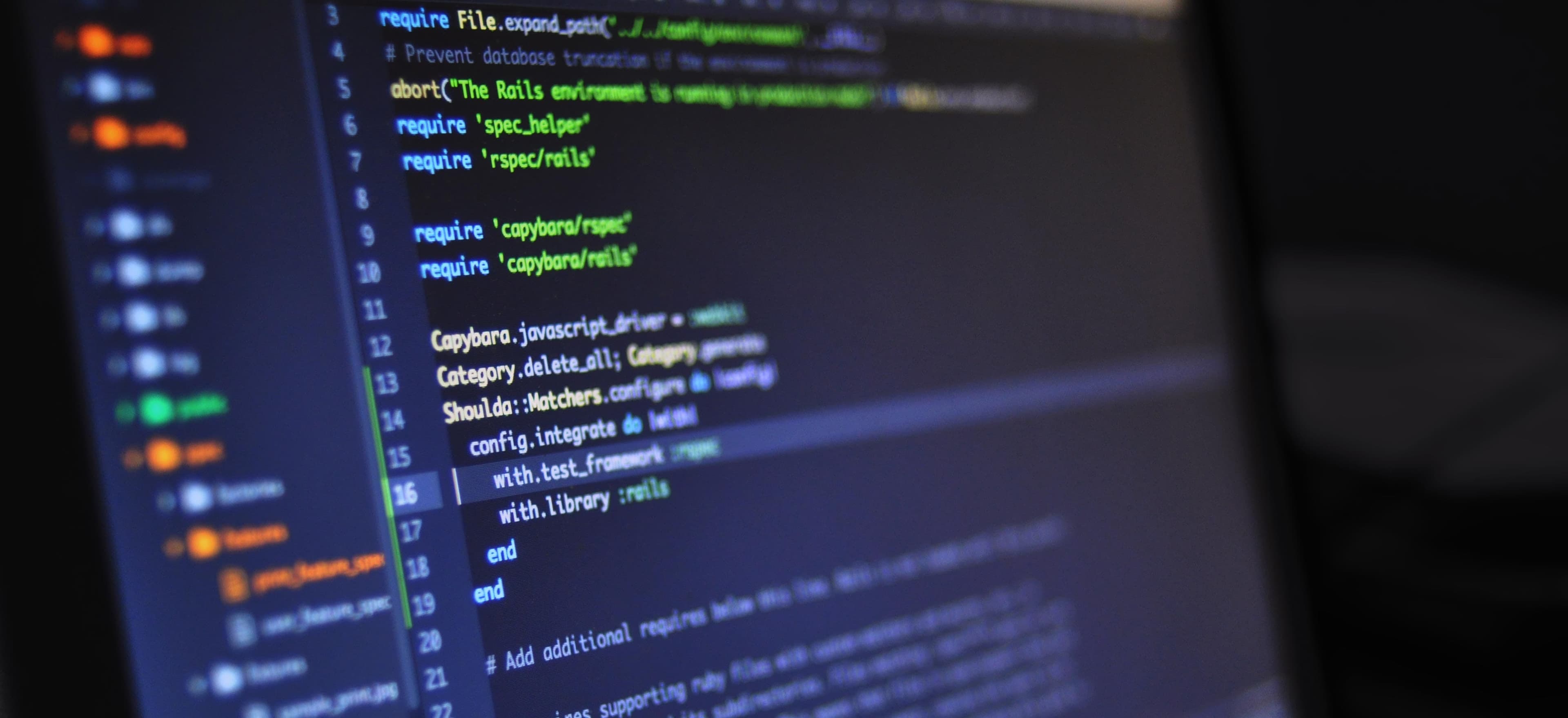
- Published on
Tackling Java Memory Management: Lessons from NodeJS
Managing memory effectively is a critical skill for any Java developer. Just as JavaScript developers face challenges with memory management in NodeJS, Java developers navigate similar waters. In this post, we will explore the significance of memory management in Java, drawing insights from NodeJS strategies to bolster our Java practices.
Understanding Memory Management in Java
Memory management in Java involves the allocation and deallocation of memory space for Java objects and variables during application execution. Unlike languages requiring manual memory management, Java uses automatic garbage collection (GC) to reclaim memory. However, this does not eliminate the need for developers to understand and actively manage memory utilization.
Key Concepts
- Heap Memory: This is where all the class instances and arrays are allocated. The Java heap is managed by the Garbage Collector.
- Stack Memory: This is used for method invocation, variable storage, and is generally faster than heap memory. Each thread in a Java application has its stack.
- Garbage Collection: A process by which the Java heap is cleaned up, freeing up memory occupied by objects that are no longer in use.
Why Memory Leaks Matter
Memory leaks occur when allocated memory is not released after it is no longer needed. In both Java and NodeJS, memory leaks can lead to slow application performance and even application crashes. In Java, leaks often arise from static references, unintentional object retention, or improperly closed resources.
Lessons from NodeJS: Conquering Memory Leaks
In an insightful article titled "Conquer NodeJS Memory Leaks: Strategies and Solutions", various methods are presented to combat memory leaks. While those techniques are specific to NodeJS, many translate well to Java programming. Let’s explore some of these strategies.
1. Object Scoping
In NodeJS, developers are often reminded to limit the scope of objects. This can also be applied in Java. By controlling the scope of variables and object references, you minimize the chances of memory being held longer than necessary.
public void process() {
MyObject obj = new MyObject();
// Use obj here
} // obj becomes eligible for garbage collection after this line
Why? By limiting the visibility of obj
to the process()
method, we ensure that it can be garbage collected as soon as we're done using it.
2. Weak References
Just like NodeJS offers tools to manage memory, Java has its own mechanisms, notably using WeakReference
. Weak references allow the garbage collector to reclaim the referenced object if no strong references exist.
import java.lang.ref.WeakReference;
public class Cache {
private WeakReference<MyObject> cachedObject;
public void cacheObject(MyObject obj) {
cachedObject = new WeakReference<>(obj);
}
public MyObject getCachedObject() {
return cachedObject.get(); // May return null if object has been collected
}
}
Why? Using weak references helps in scenarios where we need to cache objects without preventing their memory from being reclaimed.
3. Resource Management
For NodeJS applications, properly releasing resources is paramount. In Java, we have similar considerations. Always close resources like streams and connections in a finally
block, or use try-with-resources.
try (InputStream input = new FileInputStream("file.txt")) {
// Process the file
} catch (IOException e) {
e.printStackTrace();
}
// No need for finally block to close input
Why? This automatically closes the InputStream
after use, which prevents memory leaks associated with unclosed resources.
4. Profiling and Monitoring Memory Usage
Just as NodeJS developers rely on tools like Chrome DevTools to analyze memory, Java developers can use tools such as VisualVM, Java Mission Control, or Eclipse Memory Analyzer. These tools allow us to visualize memory usage and track down memory leaks.
// Simulating memory-intensive task
public void simulateMemoryUsage() {
List<MyObject> list = new ArrayList<>();
for (int i = 0; i < 100000; i++) {
list.add(new MyObject());
}
// Memory profiler can be used here to track the usage
}
Why? Monitoring tools help developers pinpoint memory consumption hotspots and identify objects that should be eligible for garbage collection but aren't.
5. Immutable Objects
In Java, immutable objects can be a game changer. Similar to how immutable structures in NodeJS reduce mutability-related bugs, Java's String
, Integer
, and classes like LocalDate
are immutable by design.
public final class ImmutableObject {
private final String name;
public ImmutableObject(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Why? Immutable objects are inherently thread-safe and do not leave mutable state in memory, which helps reduce unintended memory retention.
The Bottom Line
As we juggle the intricacies of memory management in Java, it is essential to draw lessons from other technologies like NodeJS. Techniques such as limiting object scope, utilizing weak references, managing resources appropriately, profiling memory, and embracing immutability can help us write better Java applications.
By developing a robust understanding of memory management and employing these strategies, Java developers can mitigate memory leaks effectively, leading to smoother, more responsive applications. Always remember that memory should be treated as a valuable resource that must be managed carefully.
For more insights and tips into tackling performance and memory-related issues in NodeJS, don’t forget to check out the article Conquer NodeJS Memory Leaks: Strategies and Solutions.
As we continue our journey through the world of Java, let's leverage the lessons learned from our peers in NodeJS to enhance our coding practices, ultimately creating more efficient and reliable applications. Happy coding!
Checkout our other articles