Java Frameworks for Analyzing Historical Data Trends
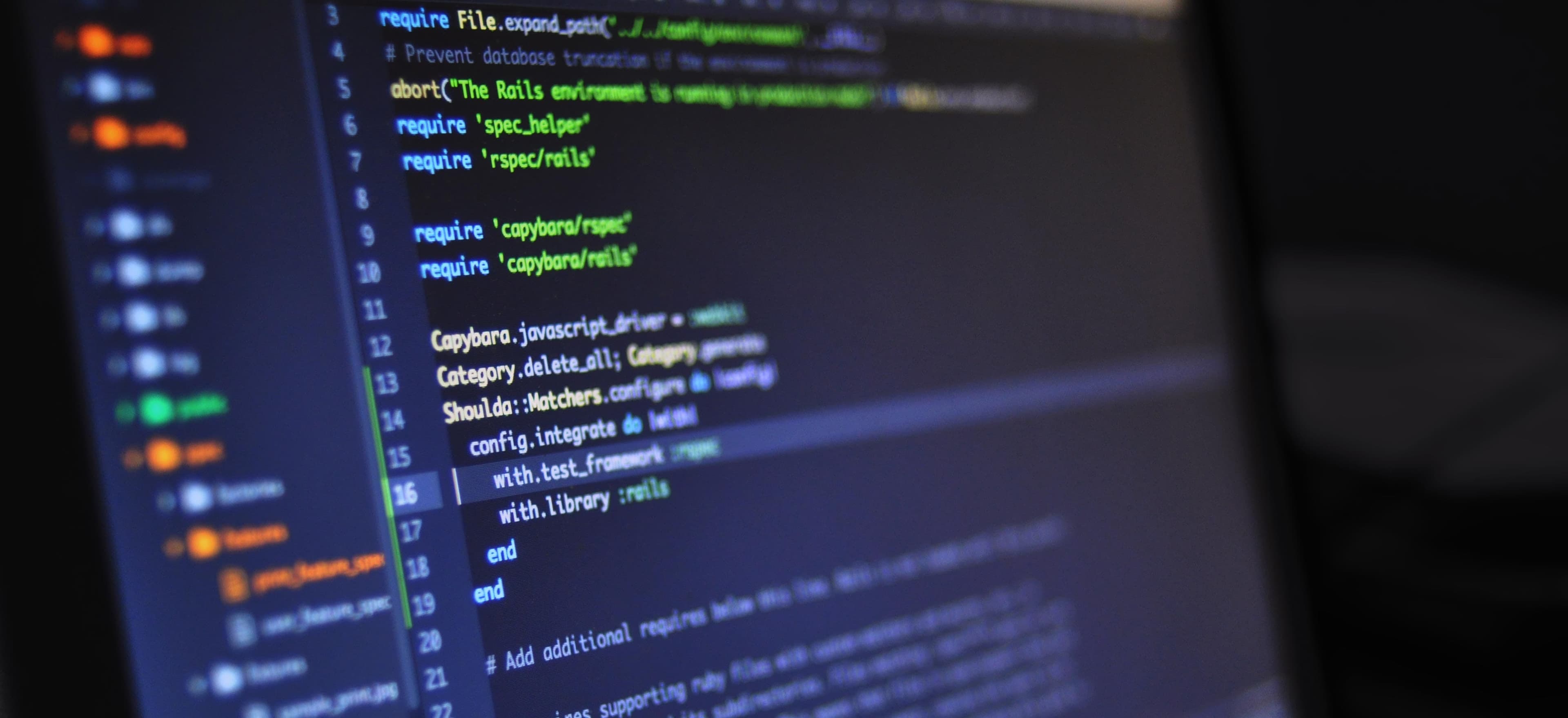
- Published on
Java Frameworks for Analyzing Historical Data Trends
Analyzing historical data trends is a crucial task in various fields, including economics, sociology, and history. With the plethora of data available today, effectively deciphering and extracting meaningful patterns from historical datasets has become more vital than ever. In this blog post, we will explore Java frameworks that are particularly suited for analyzing historical data trends, showcasing why they are advantageous and how they can be implemented with code snippets for practical understanding.
Why Java for Data Analysis?
Java is a strong contender for data analysis for several reasons:
- Platform Independence: Write once, run anywhere. Java’s portability makes it an attractive option for data analysis applications.
- Robust Libraries: Java has an extensive ecosystem of libraries that simplify the implementation of complex data analysis algorithms.
- Performance: With its high-performance capabilities, Java excels in processing large datasets efficiently.
Key Java Frameworks for Historical Data Analysis
1. Apache Commons Math
Apache Commons Math is a powerful library that provides tools for advanced mathematics and statistics, making it ideal for analyzing historical trends in data. It includes functionality for complex calculations including regression analysis and interpolation.
Code Example: Linear Regression
The following code snippet demonstrates how to perform a simple linear regression to analyze a dataset comprising historical temperatures.
import org.apache.commons.math3.stat.regression.SimpleRegression;
public class LinearRegressionExample {
public static void main(String[] args) {
SimpleRegression regression = new SimpleRegression();
// Adding data points (year, temperature)
regression.addData(2000, 14.5);
regression.addData(2001, 14.7);
regression.addData(2002, 15.2);
regression.addData(2003, 15.5);
regression.addData(2004, 15.8);
// Predict the temperature for the year 2005
double predictedTemp = regression.predict(2005);
System.out.println("Predicted temperature for 2005: " + predictedTemp);
}
}
In this example, we use SimpleRegression
to model the trend of historical temperatures. The method addData
is instrumental for feeding data points into our model, allowing us to fit a straight line and make predictions.
2. JFreeChart
Data visualization is crucial when analyzing historical trends, and JFreeChart offers a simple way to create professional charts and graphs. This framework allows analysts to represent their findings in a visually appealing manner.
Code Example: Creating a Line Chart
Below is an example that illustrates how to create a line chart for visualizing historical sales data.
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.data.xy.XYSeries;
import org.jfree.data.xy.XYSeriesCollection;
import javax.swing.*;
public class LineChartExample extends JFrame {
public LineChartExample(String title) {
super(title);
XYSeries series = new XYSeries("Sales Data");
// Adding data points (year, sales)
series.add(2000, 150);
series.add(2001, 160);
series.add(2002, 190);
series.add(2003, 210);
series.add(2004, 220);
XYSeriesCollection dataset = new XYSeriesCollection(series);
JFreeChart chart = ChartFactory.createXYLineChart(
"Historical Sales Trends",
"Year",
"Sales",
dataset
);
ChartPanel panel = new ChartPanel(chart);
panel.setPreferredSize(new java.awt.Dimension(800, 600));
setContentPane(panel);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
LineChartExample example = new LineChartExample("Line Chart Example");
example.setSize(800, 600);
example.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
example.setVisible(true);
});
}
}
In this code, we prepare a dataset representing sales over time and then create a line chart using ChartFactory
. This visualization assists in quickly understanding trends and spikes in historical data.
3. Apache Spark
For larger datasets and sophisticated analysis, Apache Spark’s capabilities stand out. With its support for both batch and stream processing, Spark allows for analyzing real-time data trends as well as historical data.
Code Example: DataFrame Analysis
Using Spark, you can conduct complex operations like filtering, aggregation, and transformation. Below is how you can perform simple data analysis using Spark’s DataFrame API.
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class SparkDataFrameExample {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("Historical Data Analysis")
.master("local")
.getOrCreate();
// Sample historical sales data as a DataFrame
Dataset<Row> salesData = spark.read().option("header", true)
.csv("path/to/sales_data.csv");
// Show dataset
salesData.show();
// Analyze average sales by year
salesData.groupBy("year").avg("sales").show();
spark.stop();
}
}
This snippet performs exploratory data analysis by loading a CSV file, displaying the data, and calculating average sales by year using the powerful DataFrame API.
4. Weka
Weka is an extensive suite of machine learning software written in Java. It is extremely useful for dataset analysis, allowing users to apply machine learning techniques to historical data.
Code Example: Using Weka for Classification
Below, you can see a code snippet that demonstrates how to use the Weka library to make predictions based on historical data.
import weka.classifiers.Classifier;
import weka.classifiers.trees.J48;
import weka.core.DenseInstance;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class WekaExample {
public static void main(String[] args) throws Exception {
// Load dataset
DataSource source = new DataSource("path/to/historical_data.arff");
Instances data = source.getDataSet();
data.setClassIndex(data.numAttributes() - 1); // Set the last attribute as the class
// Build classifier
Classifier classifier = new J48(); // Using the J48 decision tree algorithm
classifier.buildClassifier(data);
// Create a new instance for prediction
DenseInstance newInstance = new DenseInstance(data.numAttributes());
// add attributes data here...
double label = classifier.classifyInstance(newInstance);
System.out.println("Predicted Class: " + data.classAttribute().value((int) label));
}
}
This example displays how to load a dataset in ARFF format and apply a J48 decision tree classifier to predict classes based on historical attributes.
The Bottom Line
Through this exploration, we've seen how various Java frameworks empower data analysts to dive deep into historical data trends. Whether it’s through statistical analysis with Apache Commons Math, creating charts with JFreeChart, or executing robust data processing in Apache Spark, Java provides the tools necessary to reveal insights obscured within piles of data.
Additionally, for context around the relevancy of analyzing historical trends in today's world, consider visiting Aufstieg und Fall: Das Deutsche Reich (1871-1914). Understanding past events is essential to predicting future patterns, making tools and methodologies for analyzing such data ever more crucial.
The Java ecosystem continues to evolve, bringing robust solutions for historical data analysis. Embracing these frameworks will undoubtedly enhance your ability to derive meaningful insights from historical datasets.
Checkout our other articles