Mastering Input Validation for RESTful API Security
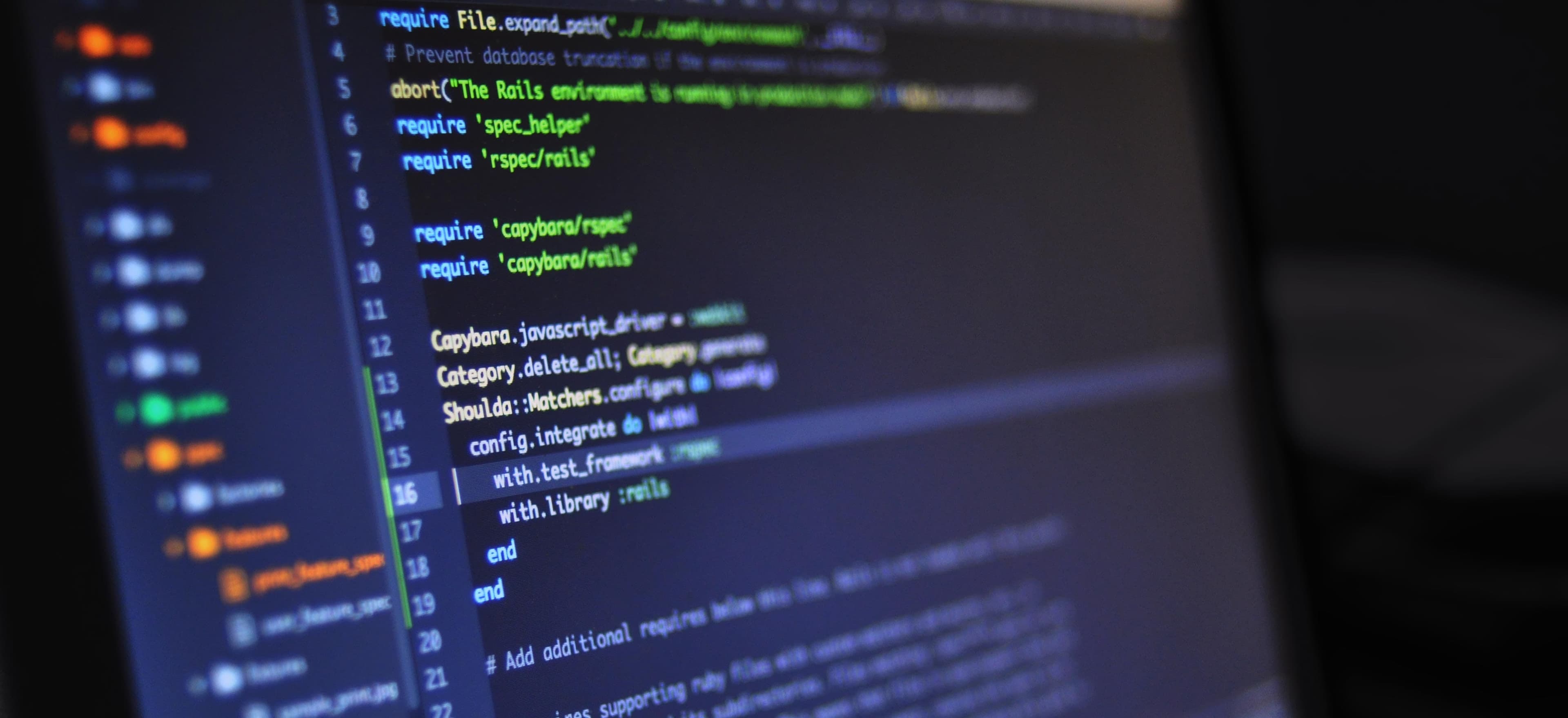
- Published on
Mastering Input Validation for RESTful API Security
In the era of digital transformation, secure APIs are paramount. A well-designed RESTful API can streamline communications and enhance user experiences, but it can also be a target for malicious attacks if not properly secured. Among the countless security measures available, input validation stands out as a critical first line of defense against a variety of vulnerabilities such as SQL injection, Cross-Site Scripting (XSS), and more.
In this blog post, we’ll delve into the concept of input validation, explore its significance, discuss various techniques, and provide practical examples using Java. By the end, you will have a comprehensive understanding of how to effectively implement input validation to bolster your API's security.
What is Input Validation?
Input validation is the process of verifying whether the data received by an application is of the expected format, type, length, and range before processing it. It helps ensure that only safe and legitimate inputs are accepted, thereby preventing potentially harmful data from causing damage.
Why is Input Validation Important?
- Security: The primary motive is to protect against threats like injection attacks and data breaches.
- Data Integrity: It ensures that only valid data is processed and stored, preserving the integrity of your database.
- User Experience: By providing clear feedback on input errors, you can enhance user experience, thus increasing user satisfaction.
Common Vulnerabilities Addressed by Input Validation
- SQL Injection: Attackers can manipulate SQL queries by sending malicious input, leading to unauthorized access or data breaches.
- Cross-Site Scripting (XSS): This vulnerability allows attackers to inject malicious scripts into web pages viewed by other users, potentially compromising their data.
- Command Injection: Malicious inputs can lead to the execution of arbitrary commands on your server, allowing an attacker to gain control over it.
Effective Input Validation Techniques
1. Whitelist Validation
Whitelist validation is a proactive approach where only explicitly defined acceptable inputs are permitted. This can be achieved through regex patterns or specific data formats and types.
Example: In a user registration API, you may only allow alphanumeric characters for usernames.
public boolean isValidUsername(String username) {
// Regex pattern to allow only alphanumeric characters and underscores
String regex = "^[a-zA-Z0-9_]{3,15}$";
return username.matches(regex);
}
Why? Using a whitelist approach minimizes the risk of accepting unwanted inputs that could lead to security vulnerabilities.
2. Length Validation
Validating the size of input data is crucial in avoiding buffer overflow attacks. Always specify minimum and maximum lengths for inputs.
Example: Ensure that a password adheres to a specified range of lengths.
public boolean isValidPassword(String password) {
return password.length() >= 8 && password.length() <= 20;
}
Why? Limiting password lengths enhances security, preventing overly long inputs that could exploit vulnerabilities.
3. Type Validation
Check whether the input matches the expected data type. This is especially important in numerical fields to avoid errors and potential exploits.
Example: When accepting age as an input parameter.
public boolean isValidAge(String age) {
try {
int parsedAge = Integer.parseInt(age);
return parsedAge >= 0 && parsedAge <= 120; // Realistic age range
} catch (NumberFormatException e) {
return false; // Not a valid integer
}
}
Why? Type validation ensures that only valid data types are processed, effectively reducing the likelihood of exploits.
4. Regular Expression Validation
Regular expressions (regex) provide a powerful way to specify complex input patterns, such as email addresses or URLs.
Example: Validate an email format.
public boolean isValidEmail(String email) {
String regex = "^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$";
return email.matches(regex);
}
Why? Accurate regex support can minimize the risk that less-valid inputs represent.
Frameworks and Libraries for Input Validation
When developing a Java RESTful API, validating inputs can be made simpler with the help of libraries. One such library is Java Bean Validation (JSR 380), which allows developers to annotate models for validation.
Using Hibernate Validator for Input Validation
Hibernate Validator is the reference implementation of JSR 380. Below is a demonstration of how it works.
Step 1: Add the dependency to your Maven pom.xml
.
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
<version>6.2.0.Final</version>
</dependency>
Step 2: Create a model class with validation annotations.
import javax.validation.constraints.Email;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Size;
public class User {
@NotBlank(message = "Username cannot be empty")
@Size(min = 3, max = 15, message = "Username must be between 3 and 15 characters long")
private String username;
@NotBlank(message = "Email is required")
@Email(message = "Email should be valid")
private String email;
@NotBlank(message = "Password cannot be empty")
@Size(min = 8, message = "Password must be at least 8 characters long")
private String password;
// Getters and Setters
}
Step 3: Validate the input in your REST controller.
import org.springframework.web.bind.annotation.*;
import javax.validation.Valid;
@RestController
@RequestMapping("/api/users")
public class UserController {
@PostMapping("/register")
public ResponseEntity<String> registerUser(@Valid @RequestBody User user) {
// Registration logic here
return ResponseEntity.ok("User registered successfully");
}
}
Why? By leveraging Hibernate Validator, you abstract away a lot of boilerplate code, enabling clean and maintainable input validation.
Testing Input Validation
Testing is a critical stage in ensuring input validation works as intended. Use unit tests to validate the user inputs.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class UserTest {
@Test
public void testValidUsername() {
assertTrue(isValidUsername("User_123"));
assertFalse(isValidUsername("User@123"));
}
@Test
public void testValidEmail() {
assertTrue(isValidEmail("test@example.com"));
assertFalse(isValidEmail("test@.com"));
}
// Additional tests for password and other fields
}
Why? Testing input validation rigorously ensures that no vulnerabilities exist and that business rules are always applied.
Lessons Learned
In conclusion, mastering input validation is essential for robust API security. By implementing effective validation techniques, leveraging libraries like Hibernate Validator, and rigorously testing your inputs, you can significantly reduce risks associated with vulnerabilities and safeguard your applications against potential threats.
Investing in secure input validation practices will not only enhance security but also foster trust among users who rely on your API services. Remember that security is an ongoing process. Stay informed and adapt to new threats as they arise.
For further reading on API security best practices, you can explore OWASP's REST Security Cheat Sheet.
Feel free to share your thoughts, questions, or additional tips in the comments section below!
Checkout our other articles