Effective Use of Classname Filters in OSGi Test Suites
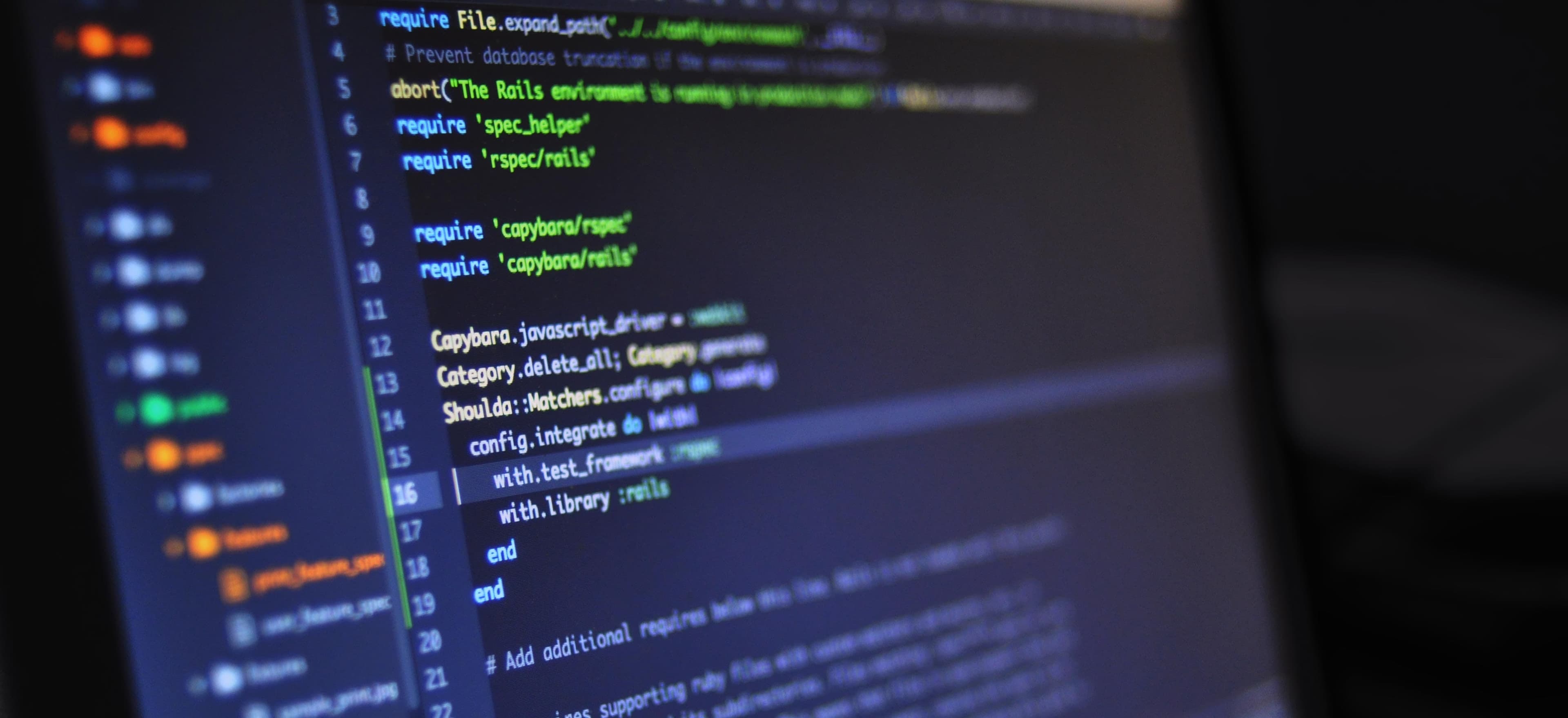
- Published on
Effective Use of Classname Filters in OSGi Test Suites
The Open Services Gateway initiative (OSGi) is a popular framework for developing modular applications in Java, particularly noteworthy in enterprise solutions. One critical aspect of ensuring quality in OSGi-based applications is robust testing, specifically through OSGi test suites. When working with OSGi, class name filters become an essential tool for selecting which tests or components to execute. This blog post delves deep into using classname filters effectively in OSGi test suites, ensuring that you write maintainable and reliable code.
Table of Contents
- Introduction to OSGi Testing
- Understanding Classname Filters
- Why Use Classname Filters?
- Implementing Classname Filters
- Best Practices
- Conclusion
Diving Into the Subject to OSGi Testing
Testing within the OSGi framework can be daunting due to its dynamic nature. OSGi enables modules (known as bundles) to be installed, started, stopped, and updated at runtime. This flexibility necessitates a testing approach that can dynamically select relevant tests based on the bundles and the runtime environment.
In OSGi, JUnit is often used for writing unit tests. These tests can be executed in various environments, including integration with continuous integration (CI) systems. As your application grows, efficiently managing tests becomes essential.
Understanding Classname Filters
Classname filters are tools for selectively running tests based on the class names. They allow developers to define patterns of test classes they want to execute. This can help with performance optimization, as it prevents unnecessary executions of tests unrelated to recent changes.
For example, if you are working on a UserService
feature, you might want to run only tests that include "UserService" in their classname.
Example of Classname Filter
Using the maven-surefire-plugin
, we can define filters directly in the Maven pom.xml
file:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<includes>
<include>**/*UserServiceTest.java</include>
</includes>
</configuration>
</plugin>
</plugins>
</build>
In this case, only tests with "UserService" in their names will be included.
Why Use Classname Filters?
Using classname filters has several benefits:
1. Performance Optimization
Only running the relevant tests speeds up the testing process. In large applications, you might have hundreds of tests that don't need to run every time you make a minor change.
2. Focused Testing
Running a specific set of tests allows for more controlled and meaningful feedback. Rather than getting overwhelmed with results, you will only see those related to your current development focus.
3. Convenience in CI/CD Pipelines
In continuous integration pipelines, it can be advantageous to run a specific subset of tests rather than the entire suite, especially if previous tests have validated the other areas of the application.
Implementing Classname Filters
To implement classname filters effectively, you can utilize various testing frameworks along with Maven or Gradle plugins.
JUnit and Maven Surefire
As demonstrated above, use the Surefire plugin to specify includes and excludes directly in your pom.xml
.
Example
Assuming you have the following classes:
- UserServiceTest
- OrderServiceTest
- UserServiceIntegrationTest
You'll want to run tests only under the UserService
. By implementing the filter as shown earlier, your maven-surefire-plugin
configuration would include tests with UserService
in the name.
Gradle Build System
If you use Gradle, the implementation is also straightforward. You can configure filters in your build.gradle
file like so:
test {
filter {
includeTestsMatching 'com.example.UserService*'
}
}
This will run any tests starting with UserService
.
OSGi Testing Framework
When using OSGi testing frameworks (like Apache Felix or OSGi JUnit), the filters can also be applied for more native testing capabilities. Take a look at how to run filtered tests in Apache Felix using the org.osgi.test.cases
package:
@RunWith(FrameworkJUnit4ClassRunner.class)
public class UserServiceTest {
@Test
public void testUserCreation() {
// Your test logic
}
}
To make it more selective, customize your testing launch configurations to include specific test classes, integrating them within the OSGi container.
Best Practices
- Name Your Tests Clearly: Use descriptive names for your test classes. This helps in creating more effective filters.
- Organize Your Tests: Group related tests into their respective packages. This way, filtering by the package name can often encapsulate various related tests.
- Regular Cleanup: Periodically assess your test suite and remove or refactor old or irrelevant tests.
- Use Annotations: Leverage JUnit annotations like
@Category
for grouping if your project uses more complex categorizations. - Document Test Filters: Maintain clear documentation regarding which filters are being used, so that your team understands the approach and can follow it consistently.
Final Thoughts
Managing tests efficiently in OSGi applications is crucial for maintaining a healthy software lifecycle. Effective use of classname filters enhances the testing experience by allowing developers to focus on relevant tests, reduce execution time, and ultimately improve code quality.
With the strategies highlighted in this guide, you are now equipped to implement classname filters successfully within your OSGi test suites. If you have any more questions or wish to share your experiences, please comment below or consider interacting with the community at the OSGi Alliance forums.
Developing a deep understanding of testing paradigms and practices, particularly with classname filters, will significantly enhance your OSGi application’s performance. Happy coding!
Checkout our other articles