Common Issues with jMetro Version 5: Troubleshooting Guide
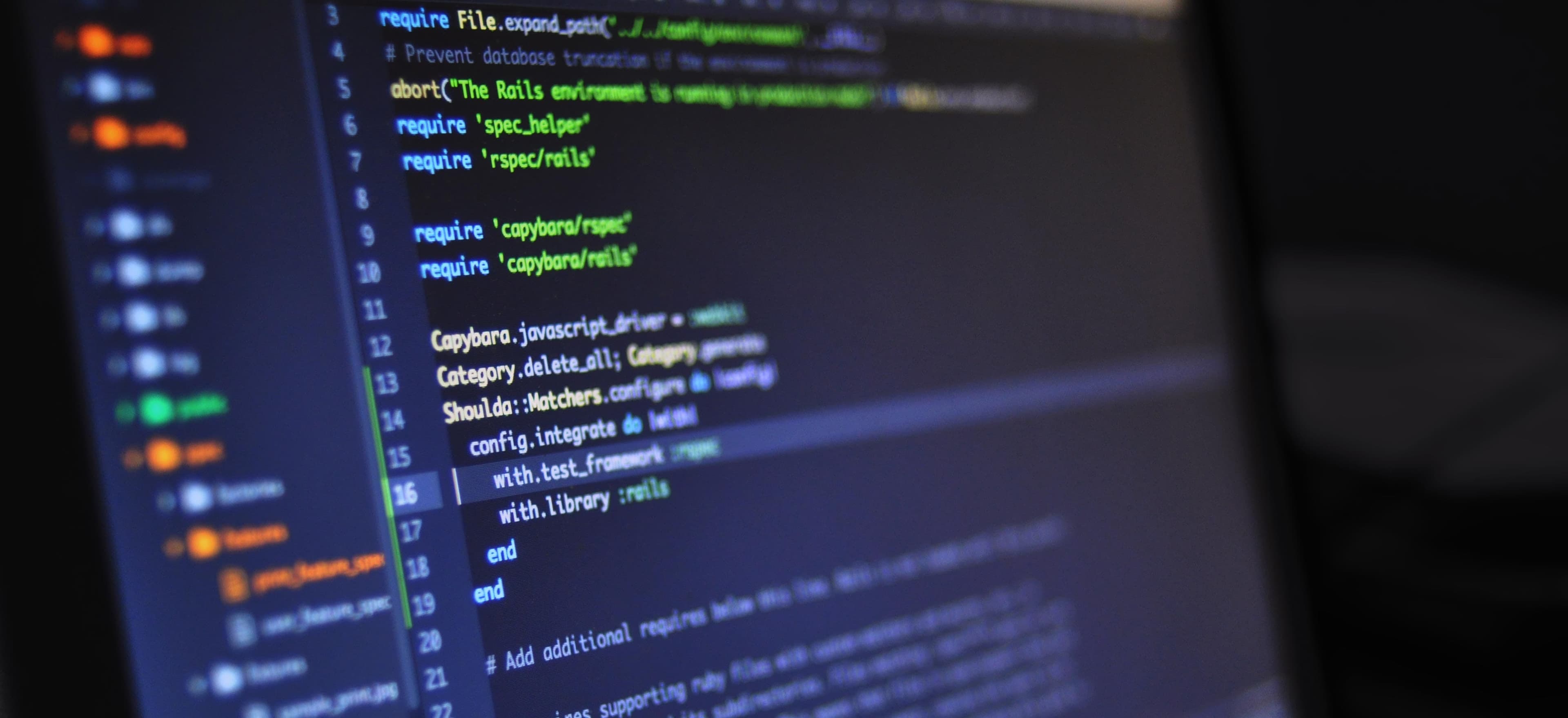
- Published on
Common Issues with jMetro Version 5: Troubleshooting Guide
jMetro is a popular styling framework that brings a Windows Metro user interface feel to JavaFX applications. However, it’s not without its quirks. In this troubleshooting guide, we will delve into common issues associated with jMetro Version 5, offering solutions and insights on how to optimize your application for a smooth user experience.
Table of Contents
Understanding jMetro
jMetro is a CSS framework designed for JavaFX applications. It allows you to create modern, sleek interfaces resembling Windows apps. JMetro simplifies the application’s visual aesthetics with easy configuration and customizations. However, as with any framework, users occasionally encounter issues that can disrupt development and user experience.
Common Issues
1. UI Rendering Problems
Issue: Flickering UI elements during transitions.
Solution: Flickering issues often stem from the rendering pipeline or overlapping styles. This can be resolved by ensuring your CSS is not conflicting.
// This example shows how to set a basic layout in JavaFX
Button button = new Button("Click Me");
button.setStyle("-fx-background-color: #0078D7; -fx-text-fill: white;");
// To reduce flickering, use a layout manager appropriately
StackPane root = new StackPane();
root.getChildren().add(button);
Scene scene = new Scene(root, 300, 200);
stage.setScene(scene);
Why: Using a dedicated layout manager like StackPane
allows JavaFX to manage rendering optimally, reducing the likelihood of flickering during UI transitions.
2. CSS Not Applying
Issue: Sometimes, the styles defined in your jMetro CSS files do not appear to be applied.
Solution: Ensure that you have correctly added the jMetro CSS styles to your application. Double-check your import paths and make sure they are relative to your source files.
// Load jMetro styles in your JavaFX application
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.control.Button;
import javafx.stage.Stage;
public class MyApp extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click Me");
StackPane root = new StackPane();
root.getChildren().add(button);
Scene scene = new Scene(root, 300, 250);
// Properly load jMetro CSS
scene.getStylesheets().add("path/to/jMetro5.css");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why: The getStylesheets()
method is crucial here. It ensures that the styles are correctly applied to the scene, affecting all relevant nodes.
3. Performance Lags
Issue: Your application may experience lags, especially when heavy CSS files are included or the UI is cluttered.
Solution: Keep a minimal CSS structure. While jMetro offers extensive styling options, avoid excessive use of complex transitions or animations.
To enhance performance, consider profiling your JavaFX application to identify bottlenecks.
// Sample of light CSS usage for better performance
.button {
-fx-background-color: #0078D7;
-fx-text-fill: white;
-fx-padding: 10px;
}
.label {
-fx-font-size: 14px;
}
Why: Simplifying your CSS can dramatically improve rendering times. By minimizing complexity, you allow the JavaFX rendering engine to handle UI updates more efficiently.
4. Compatibility Issues
Issue: Certain components may not behave as expected due to version mismatches among Java, JavaFX, and jMetro.
Solution: Verify that you are using compatible versions of Java, JavaFX, and jMetro. You can check the jMetro GitHub page for a table outlining supported versions.
Also, ensure your local setup matches the project's configuration. The pom.xml
is necessary to check if the dependencies are correctly defined.
<dependencies>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>15.0.1</version>
</dependency>
<dependency>
<groupId>com.jmetro</groupId>
<artifactId>jMetro</artifactId>
<version>5.0.1</version>
</dependency>
</dependencies>
Why: Mismatched versions can lead to unexpected behavior. Before diving into complex debugging, always ensure that your software stack is aligned.
Best Practices
- Keep it Simple: Limit CSS complexity to improve performance.
- Documentation and Community: Utilize forums and GitHub issues for common fixes and recommendations. The JavaFX community is a valuable resource for questions and discussions.
- Regularly Update: jMetro actively evolves, so keep your dependencies up to date to benefit from fixes and new features.
- Profile Your Application: Regularly use profiling tools to track performance metrics.
The Bottom Line
Troubleshooting issues with jMetro Version 5 doesn’t have to be a daunting task. By understanding common problems such as UI rendering issues, CSS not applying, performance lags, and compatibility concerns, you can take a proactive approach to your JavaFX applications. Following best practices, maintaining clear documentation, and engaging with the community will ensure that your applications not only function correctly but also deliver a seamless user experience.
By remaining informed and proactive, you can harness the full potential of jMetro in your Java applications. Happy coding!