Mastering Factory Patterns Without If-Else Statements
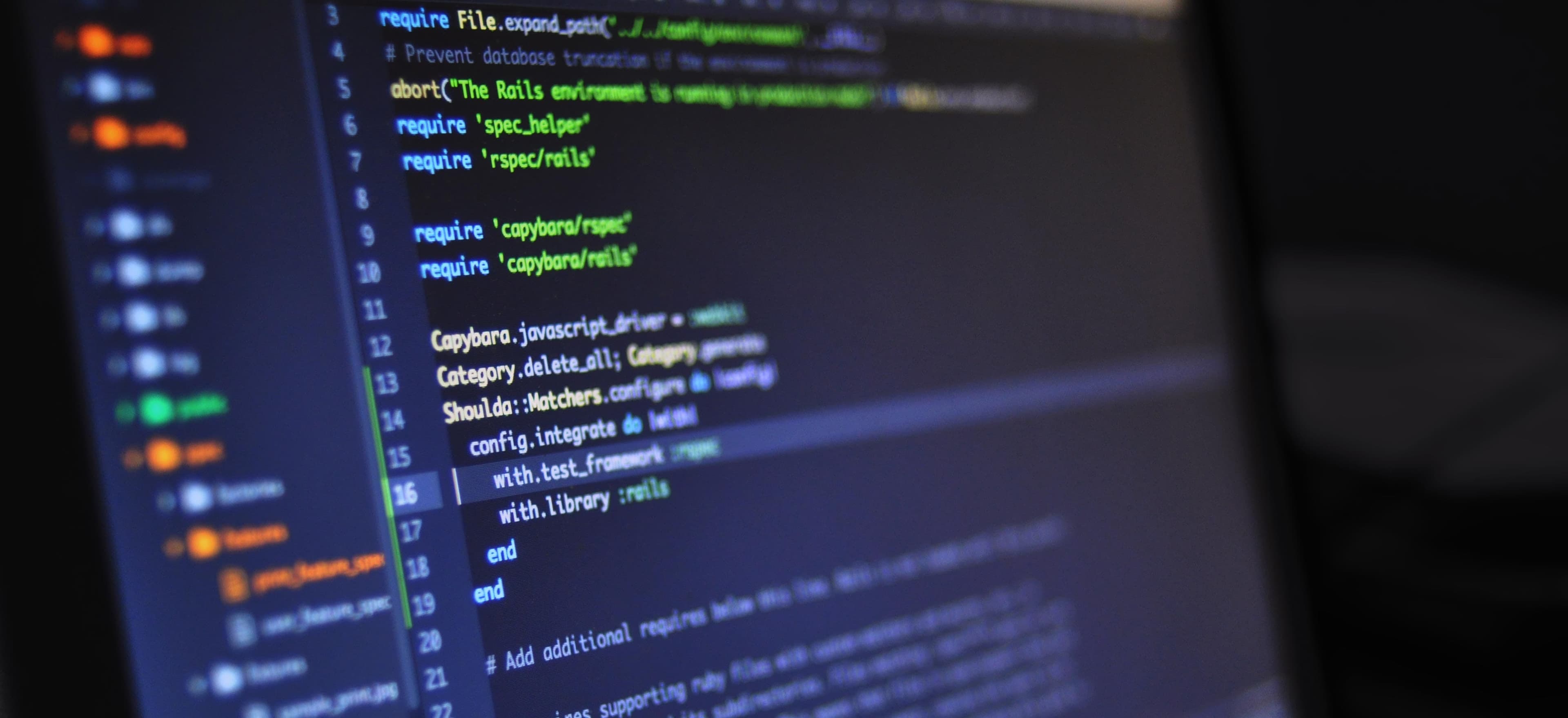
- Published on
Mastering Factory Patterns Without If-Else Statements
The Factory Design Pattern is a creational pattern used to create objects. It allows for object creation without having to specify the exact class of the object that will be created. This is especially useful in scenarios where your code might need to evolve, adapting to changes in class types. In this post, we will dive deep into the Factory Pattern, explore its advantages, and demonstrate how to implement it without relying on cumbersome if-else statements.
Understanding Factory Patterns
Before we dive into the code, let’s establish a firm understanding of what we mean by Factory Patterns. The fundamental idea behind a Factory Pattern is to create a component (or factory) that is responsible for instantiating a certain type of class based on the inputs provided.
Types of Factory Patterns
-
Simple Factory Pattern: This is not a formal design pattern, but rather a simple creation pattern that allows for easy object creation.
-
Factory Method Pattern: This involves a method for creating objects where subclasses can alter the type of objects that will be created.
-
Abstract Factory Pattern: This provides an interface for creating families of related or dependent objects without specifying their concrete classes.
The Dangers of If-Else Statements
Using if-else statements for object creation can lead to code that is hard to maintain and tightly coupled. For example, imagine a scenario where you are creating different vehicle types based on user inputs. Relying on if-else statements can lead to complex and unwieldy code like this:
if (vehicleType.equals("Car")) {
return new Car();
} else if (vehicleType.equals("Bike")) {
return new Bike();
} else if (vehicleType.equals("Truck")) {
return new Truck();
} // And so on...
This kind of code makes it difficult to extend, as introducing a new vehicle type requires modifications to existing code.
How to Implement the Factory Pattern
Let’s implement the Factory Method without using if-else statements, allowing for an easier and cleaner approach to object creation.
Step 1: Define a Vehicle Interface
Start by defining a Vehicle
interface that all vehicles will implement.
public interface Vehicle {
void drive();
}
Step 2: Create Concrete Classes
Next, create concrete classes like Car
, Bike
, and Truck
that implement the Vehicle
interface.
public class Car implements Vehicle {
@Override
public void drive() {
System.out.println("Driving a car");
}
}
public class Bike implements Vehicle {
@Override
public void drive() {
System.out.println("Riding a bike");
}
}
public class Truck implements Vehicle {
@Override
public void drive() {
System.out.println("Driving a truck");
}
}
Step 3: Create the Vehicle Factory
Instead of using if-else statements, use a Map
to associate vehicle types with their respective classes. This will allow for cleaner code and better maintainability.
import java.util.HashMap;
import java.util.Map;
public class VehicleFactory {
private static final Map<String, Supplier<Vehicle>> vehicleMap = new HashMap<>();
static {
vehicleMap.put("Car", Car::new);
vehicleMap.put("Bike", Bike::new);
vehicleMap.put("Truck", Truck::new);
}
public static Vehicle createVehicle(String vehicleType) {
Supplier<Vehicle> vehicle = vehicleMap.get(vehicleType);
if (vehicle != null) {
return vehicle.get();
} else {
throw new IllegalArgumentException("Vehicle type not supported");
}
}
}
Benefits of This Approach
- Decoupled Code: By using a
Map
for vehicle creation, you isolate the vehicle creation logic from the rest of your code. - Easy Extensibility: Adding a new vehicle type is now as simple as adding a new entry to the
vehicleMap
, without touching existing code. - Readability: The logic remains clean, avoiding the clutter that comes with cascading if-else structures.
Step 4: Using the Vehicle Factory
Now let’s see how to leverage the VehicleFactory
in your application.
public class Main {
public static void main(String[] args) {
Vehicle vehicle1 = VehicleFactory.createVehicle("Car");
vehicle1.drive();
Vehicle vehicle2 = VehicleFactory.createVehicle("Bike");
vehicle2.drive();
Vehicle vehicle3 = VehicleFactory.createVehicle("Truck");
vehicle3.drive();
}
}
Final Thoughts
By adopting a factory pattern with a Map
based approach, you eliminate the need for if-else statements in your code. This not only enhances readability but also significantly improves maintainability and scalability. As your application grows, this pattern allows you to easily integrate new types into your system.
For more advanced design patterns and their implementations, consider exploring the Design Patterns in Java outright.
The Closing Argument
In conclusion, mastering factory patterns in Java involves understanding the principles of object creation without having your application depend on specific implementations. This enables cleaner code and easier maintenance.
If you found this post insightful, leave a comment below! Are there other topics you would like to explore? Let’s have a discussion. Happy coding!