Common Pitfalls in Resource Dependency Injection in Java EE 7
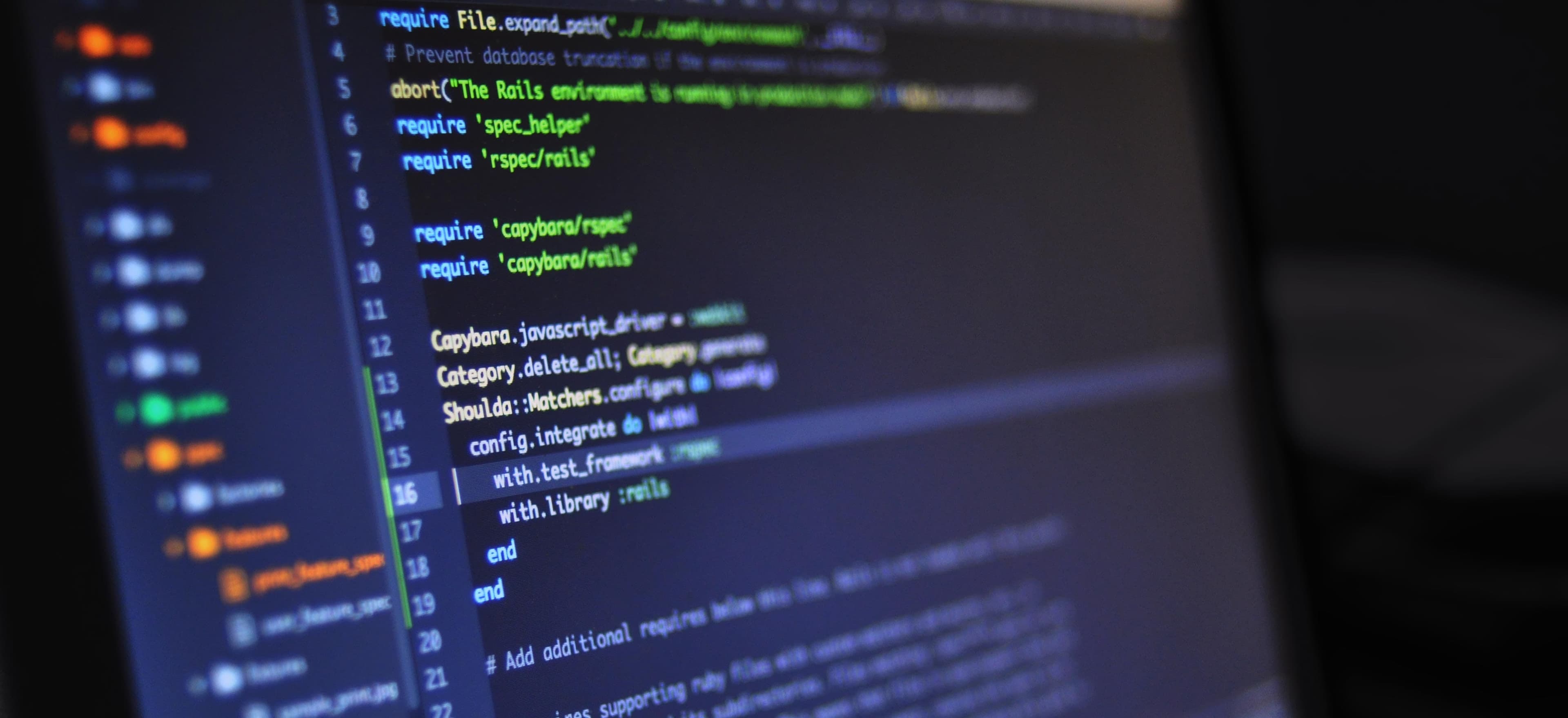
- Published on
Common Pitfalls in Resource Dependency Injection in Java EE 7
Dependency Injection (DI) is a fundamental pattern in modern Java EE (Jakarta EE) applications. One of its main goals is to make the codebase easier to manage, test, and maintain. Resource dependency injection in particular allows you to declare dependencies on the fly instead of hardcoding them, promoting a clean separation between the business logic and the resource configuration. However, DI is not without its pitfalls—especially in Java EE 7.
In this blog post, we’ll discuss some common pitfalls that developers frequently encounter when using resource dependency injection in Java EE 7. By understanding these pitfalls and how to avoid them, you can ensure your applications are robust, maintainable, and efficient.
Understanding Resource Dependency Injection
Before diving into the pitfalls, let’s briefly explore what resource dependency injection means in Java EE 7. Resource DI allows you to inject resources such as DataSource, JMS connections, and EJBs directly into your Java classes. Using annotations like @Resource
, you can greatly simplify the resource management of your application.
import javax.annotation.Resource;
import javax.sql.DataSource;
public class MyService {
@Resource(name = "jdbc/myDataSource")
private DataSource dataSource;
public void execute() {
// Business logic that uses the dataSource
}
}
Here, @Resource
tells the Java EE container to automatically manage the dataSource
in an efficient manner, so you don’t have to worry about the underlying complexity.
Common Pitfalls in Resource Dependency Injection
1. Failing to Specify Resource Lookup Names
One of the most frequent mistakes is not specifying the correct lookup names for the resources. Ensure that the name
attribute in the @Resource
annotation corresponds accurately to the resource defined in the corresponding configuration file (usually web.xml
or ejb-jar.xml
).
Example
@Resource(name = "jdbc/myDataSource")
private DataSource dataSource;
If jdbc/myDataSource
does not exist or is incorrectly spelled, your application will fail at runtime, causing considerable debugging time.
2. Over-using Constructor Injection
While constructor injection has its advantages, over-using it for resource injection in Java EE can lead to complex initialization sequences. Java EE’s managed components are expected to undergo a lifecycle managed by the container, and constructor injections can bypass that lifecycle.
Consider the following example:
public class MyService {
private final DataSource dataSource;
public MyService(DataSource dataSource) {
this.dataSource = dataSource;
}
}
This approach forces you to manually manage the dataSource
, thereby increasing the risk of runtime errors. Use field injection instead, as shown previously, to let the container manage the lifecycle and initialization of resources.
3. Mixing Resource Injection with Manual Initialization
Combining resource DI with manual instantiation leads to confusion and can break the DI principle of "inversion of control." The application will become challenging to manage, and you may inadvertently create tight coupling between classes.
Example
public class MyService {
@Resource(name = "jdbc/myDataSource")
private DataSource dataSource;
public MyService() {
this.dataSource = new DataSource(); // This is incorrect
}
}
In this case, you're instantiating DataSource
manually, which undermines the benefits of DI. Stick to using either injection or manual instantiation for clarity.
4. Ignoring Scoping Issues
Java EE components have defined lifecycles (request, session, application, etc.). Ignoring the scope of your injected resources can lead to memory leaks or unexpected behaviors. For instance, injecting a session-scoped bean into an application-scoped bean will retain state longer than expected.
Solution
Be aware of the scopes and ensure you’re injecting resources consistent with their life cycles. Always match the scope of your resources to the requirements of your application component.
5. Not Understanding Exception Handling
Other resources like JMS or EJBs can throw checked exceptions, and these must be handled properly. Overlooking exception management can lead to resource leaks and application instability.
Example
import javax.annotation.Resource;
import javax.jms.ConnectionFactory;
import javax.jms.JMSException;
import javax.jms.JMSContext;
public class MyJmsService {
@Resource
private ConnectionFactory connectionFactory;
public void sendMessage(String message) {
try (JMSContext context = connectionFactory.createContext()) {
// Send message
} catch (JMSException e) {
e.printStackTrace(); // Log and handle appropriately
}
}
}
Always ensure to handle exceptions gracefully while ensuring resource cleanup (here using try-with-resources).
6. Poor Testing Strategies
The reliance on DI to manage resource dependencies can affect your testing strategies. For instance, if you have hard-coded dependencies in your classes, unit tests will become cumbersome. While DI simplifies the main application, you should still consider how to mock these resources in your tests.
Solution
Leverage frameworks like Mockito to create mock instances of injected resources in your tests:
import static org.mockito.Mockito.*;
@Test
public void testYourService() {
DataSource dataSource = mock(DataSource.class);
MyService service = new MyService(dataSource);
// Write your test logic here
}
The Closing Argument
Resource dependency injection simplifies the management of resources in Java EE 7 applications, but improper handling can lead to maintenance and performance issues. By avoiding the common pitfalls outlined above and adhering to best practices, you can leverage the full power of DI to create robust and maintainable applications.
For further reading on Java EE and its features, check out the official documentation on Java EE or consider exploring Best Practices for Dependency Injection.
Armed with this knowledge, take a moment to reflect on your current practices and implement changes that will propel your Java EE applications forward.
Happy coding!