Understanding Hibernate's Complex Architecture: Key Challenges
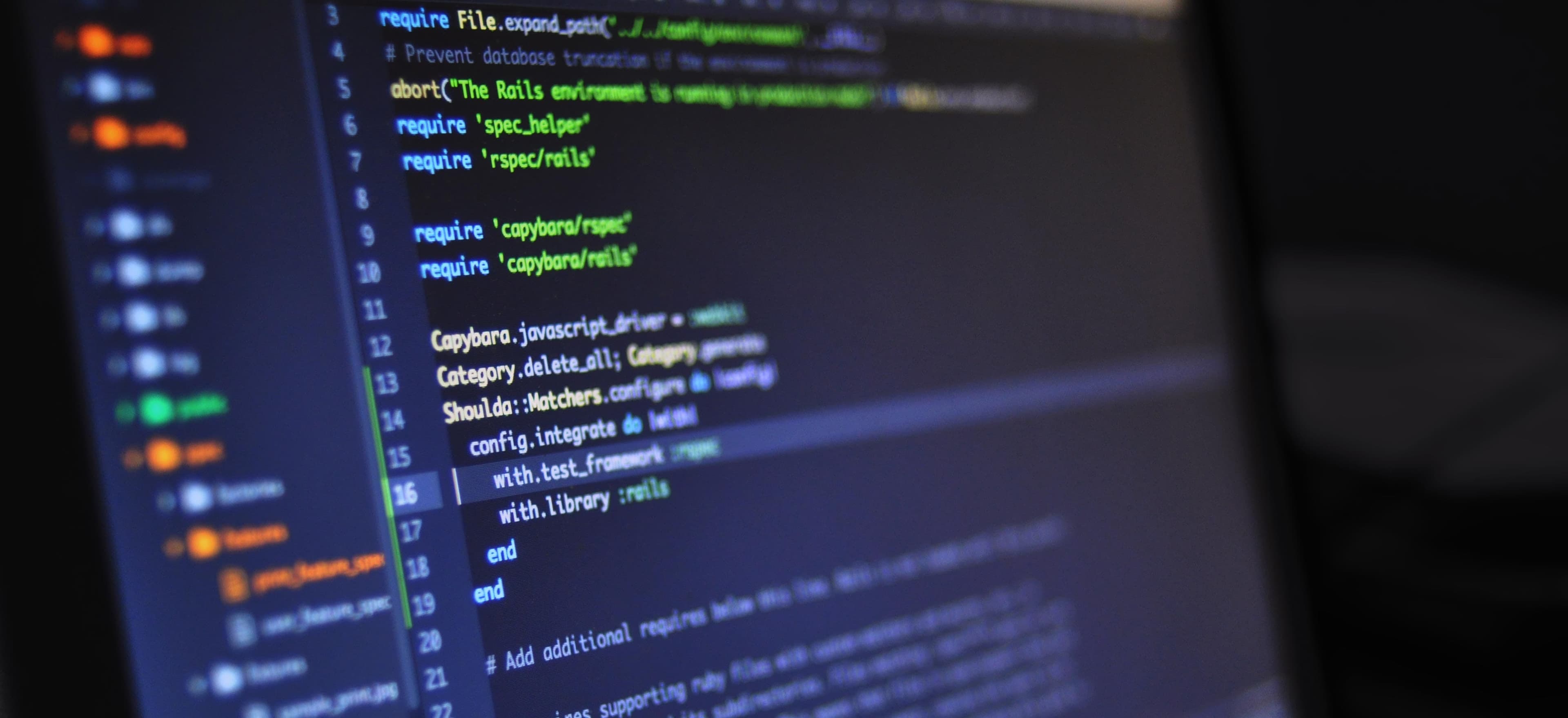
- Published on
Understanding Hibernate's Complex Architecture: Key Challenges
Hibernate is one of the most popular Object-Relational Mapping (ORM) frameworks in Java, allowing developers to interact with databases in a more convenient way while managing the complexities of data persistence. Its powerful features and high flexibility make it a requisite tool for many enterprise applications. However, its architecture is complex, and understanding it is crucial for effective implementation and performance optimization. In this post, we will delve into Hibernate’s architecture, unravel the challenges it presents, and discuss approaches to overcome them.
Table of Contents
- What is Hibernate?
- Hibernate Architecture Overview
- Core Components
- Configuration
- Key Challenges with Hibernate
- Solutions to Hibernate Challenges
- Conclusion
What is Hibernate?
Hibernate simplifies database interaction by mapping Java objects to database tables. It frees developers from writing complex SQL queries, allowing them to focus on business logic. Hibernate supports multiple databases and is adaptable, making it a top choice for Java developers. To learn more about the basics of Hibernate, you can consult the official Hibernate documentation.
Hibernate Architecture Overview
To fully grasp the challenges associated with Hibernate, it is essential to understand its core architecture.
Core Components
Hibernate's architecture comprises several key components:
-
Configuration: This is where Hibernate is configured with properties, including database URL, dialect, and connection credentials.
-
SessionFactory: The SessionFactory is a thread-safe object that creates session instances on demand. It acts as a factory for
Session
objects, which facilitate database interactions. -
Session: A single-threaded, short-lived object that encapsulates a unit of work with the database. It serves as a factory for transaction boundaries.
-
Transaction: Manages the transaction boundaries for database operations.
-
Query: This object is responsible for executing HQL or SQL queries.
Let's take a look at how we can configure Hibernate:
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
Configuration configuration = new Configuration();
// Load configuration from Hibernate configuration file
configuration.configure("hibernate.cfg.xml");
// Build SessionFactory
SessionFactory sessionFactory = configuration.buildSessionFactory();
Explanation: In this snippet, we are loading Hibernate configuration from an XML file and building a SessionFactory
. The SessionFactory
is fundamental in managing multiple sessions.
Configuration
The configuration can be done using XML or programmatically via code. In a practical scenario, an XML configuration would typically include properties that define the database connection, dialect, and logging configuration.
Example configuration in hibernate.cfg.xml
:
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydb</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">password</property>
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
</session-factory>
</hibernate-configuration>
Explanation: This XML snippet outlines how to connect to a MySQL database by specifying the driver, URL, and dialect. Each property plays a critical role in establishing the correct database environment.
Key Challenges with Hibernate
Despite its advantages, Hibernate presents several challenges:
-
Complexity of Configuration and Mapping: Configuring Hibernate properly can be daunting. Developers must understand the nuances between various mapping types (e.g., One-to-One, One-to-Many) and their implications for performance and usability.
-
Performance Issues: Hibernate can introduce overhead during data retrieval. N+1 select problems, lazy loading traps, and caching can all complicate performance tuning.
-
Debugging Difficulties: Understanding Hibernate logs and debugging issues can be complex, especially for newcomers. The abstraction Hibernate provides means that sometimes error messages can be cryptic.
-
Version Compatibility: As Hibernate evolves, certain features or configuration methods may become deprecated. Keeping an application up-to-date with Hibernate’s changes can lead to compatibility challenges.
Solutions to Hibernate Challenges
There are effective strategies to deal with these challenges:
Simplifying Configuration and Mapping
- Annotations over XML: Use JPA annotations instead of XML to specify mapping configurations whenever possible. This reduces configuration clutter and makes the code more readable.
For example, a simple User entity might look like this:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username")
private String username;
// Getters and setters omitted for brevity
}
Explanation: In this example, annotations clearly define the entity’s table and columns, providing a more straightforward configuration approach.
Performance Optimization Techniques
-
Batch Fetching: Instead of retrieving objects one by one (the N+1 problem), use batch fetching to optimize performance. This can be configured within the mappings or through the
@BatchSize
annotation. -
Second-Level Cache: Utilize Hibernate’s built-in second-level caching mechanism to reduce database access. Choose an appropriate caching provider like Ehcache or Hazelcast.
Debugging Strategies
- Enable SQL Logging: During development, enable SQL logging to monitor the queries executed by Hibernate. This can be configured in your Hibernate properties:
hibernate.show_sql=true
hibernate.format_sql=true
Explanation: These properties help visualize the SQL queries Hibernate generates, allowing developers to debug issues efficiently.
Maintainability and Versioning
-
Regular Updates: Stay abreast of Hibernate updates and deprecations. Regularly update your project dependencies to leverage enhancements and security patches.
-
Testing: Implement unit tests and integration tests in your application to ensure that changes in Hibernate versions do not inadvertently break functionality.
To Wrap Things Up
Hibernate is a powerful and sophisticated ORM solution that greatly benefits Java developers by handling the interactions between application objects and the database. Understanding its architecture is essential, as it allows developers to navigate and overcome the challenges that accompany its complexity.
By embracing modern configuration methods, optimizing performance, using effective debugging strategies, and maintaining up-to-date practices, developers can harness Hibernate's capabilities while minimizing pitfalls. Whether you're starting a new project or working on an existing one, thoughtful consideration of Hibernate's architecture will lead to better design and improved performance.
For further reading about Hibernate's features and best practices, refer to the Hibernate official documentation and explore additional resources available in the community.
By mastering Hibernate, you open the doors to building robust, scalable Java applications. Happy coding!