Common Pitfalls When Writing MapReduce Tasks in Java
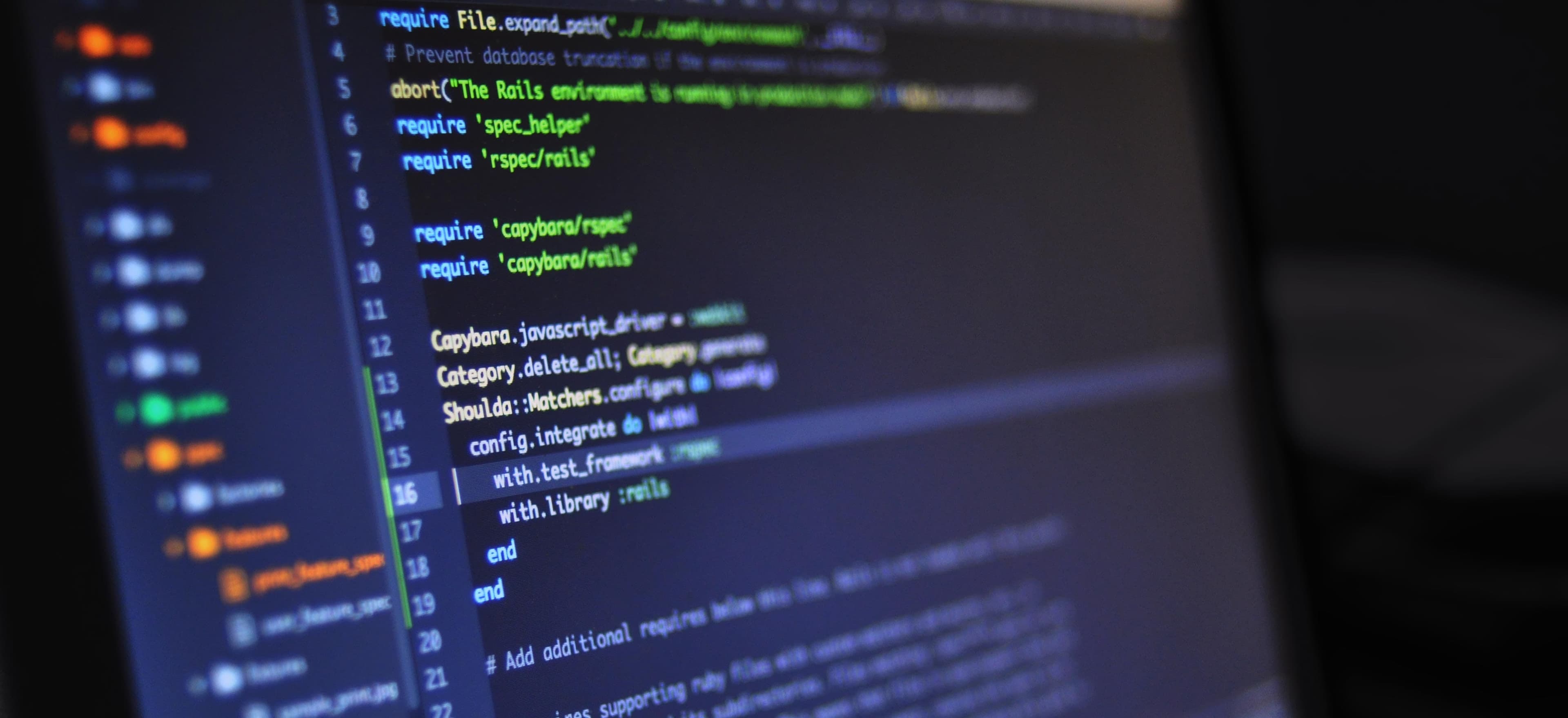
- Published on
Common Pitfalls When Writing MapReduce Tasks in Java
MapReduce is a powerful programming model designed for processing and generating large datasets. When implemented correctly, it can optimize performance and efficiency in data processing. However, there are some common pitfalls developers encounter while writing MapReduce tasks in Java. This article will guide you through these pitfalls and how to avoid them.
Understanding the Basics of MapReduce
Before diving into common pitfalls, let’s quickly summarize the MapReduce paradigm. The MapReduce process consists of two main functions: Map and Reduce.
- Map: This function processes input data, generating key-value pairs as intermediate output.
- Reduce: This function takes the intermediate key-value pairs from the Map function and aggregates them into final results.
Here’s a simple illustration of a MapReduce job in Java:
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class WordCount {
public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
String[] words = value.toString().split("\\s+");
for (String w : words) {
word.set(w);
context.write(word, one);
}
}
}
public static class IntSumReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
private IntWritable result = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class);
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
This example outlines a simple Word Count application, which counts the occurrences of each word in a dataset. Now that we have the basics down, let's explore the common pitfalls in writing MapReduce tasks.
Common Pitfalls When Writing MapReduce Tasks
1. Not Properly Configuring Your Hadoop Environment
One of the most significant missteps developers make is failing to configure their Hadoop environment correctly. Issues such as incorrect HDFS paths, misconfigured resource allocation, or failing to set up environment variables can lead to job failures.
Solution: Always double-check your Hadoop configuration files, including core-site.xml
, hdfs-site.xml
, and mapred-site.xml
. Understand the structure and ensure that paths and resource management settings are correctly specified.
2. Ignoring Data Types
In Java MapReduce, the choice of data types for input and output can significantly impact performance. Often, developers overlook the importance of serialization, leading to increased execution time and resource usage.
Example Mistake:
Using String
for keys or values can create extra overhead. Instead, use Hadoop's built-in types like Text
or IntWritable
.
Correct Usage:
public class MyMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
// Use Text instead of String for the key
}
3. Insufficient Memory Management
An essential aspect of running efficient MapReduce jobs is managing memory correctly. Failure to do so can lead to Java Heap Space
errors during job execution.
Solution: Tune your job's memory settings using parameters such as mapreduce.map.memory.mb
and mapreduce.reduce.memory.mb
.
Additionally, monitor your job’s memory usage. Adjust the number of mappers and reducers to ensure optimal resource usage.
4. Underestimating the Importance of Local Aggregation
Sometimes developers neglect to enable the combiner, which can perform local aggregations before sending data to the reducers. This oversight can lead to unnecessary data shuffling across the network, compromising performance.
Solution: Always include a combiner class when appropriate. In the Word Count example above, IntSumReducer
acts as both the reducer and combiner.
5. Not Using Counters for Monitoring
Effective monitoring is vital for diagnosing issues during job execution. Developers often miss opportunities to leverage Hadoop's built-in counters to track the number of processed records, successes, and failures.
Solution: Use counters in your job, set up in the Mapper or Reducer classes:
public class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
private static enum Counter {
LINES_PROCESSED
}
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
context.getCounter(Counter.LINES_PROCESSED).increment(1);
// rest of the map code
}
}
6. Hardcoding Values
Avoid hardcoding configurations and values within the code. This practice can lead to inflexibility and maintenance issues.
Solution: Use configuration files or job parameters for values that may change from environment to environment. For example:
Configuration conf = new Configuration();
conf.set("input.path", args[0]);
conf.set("output.path", args[1]);
7. Not Understanding the Data Flow
Understanding the data flow between the Map and Reduce phases is imperative for writing efficient MapReduce jobs. Trivial mistakes in the data handling can lead to unexpected results or crashes.
Solution: Document how data flows through your application. Keep track of the transformations and anticipate how the data will look after each phase.
8. Not Handling Large Datasets Carefully
When dealing with exceptionally large datasets, developers can run into performance bottlenecks. By not implementing optimizations such as partitioning and sorting, jobs can take longer than necessary.
Solution: Use optimizations like custom partitioners, which help in distributing workloads evenly among reducers.
9. Forgetting Cleanup Code
Cleanup is essential in every application, especially when processing large amounts of data. Failing to close streams or deallocate resources can lead to memory leaks.
Solution: Always implement a cleanup method in your Mapper and Reducer:
@Override
protected void cleanup(Context context) throws IOException, InterruptedException {
// Clean up code here, like closing streams or disconnecting from services
}
Final Thoughts
Writing efficient and reliable MapReduce tasks in Java is a challenging endeavor. It requires a solid understanding of Hadoop's architecture and various considerations that can impact performance and accuracy.
By avoiding common pitfalls such as improper configuration, ignoring data types, and failing to leverage counters for monitoring, you can enhance your MapReduce job's performance and reliability.
Stay updated with the latest practices in Apache Hadoop and keep experimenting with different optimizations. Over time, you'll refine your skills and become adept at managing large datasets with ease. Happy coding!