Mastering Stream Conversion in Java 8: A Common Pitfall
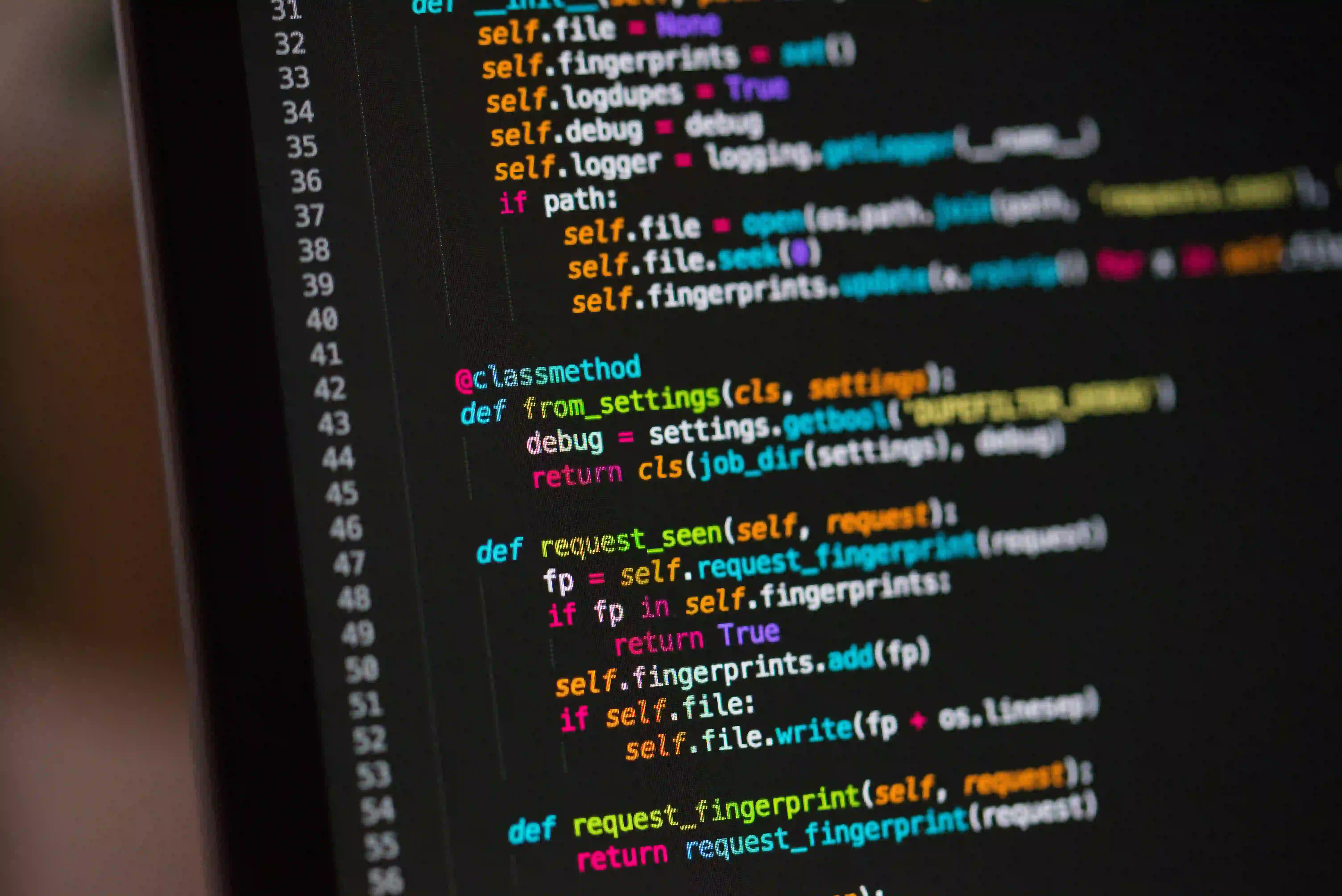
Mastering Stream Conversion in Java 8: A Common Pitfall
Java 8 introduced Streams as a powerful abstraction for processing data collections in a functional style. However, despite its advantages, there are common pitfalls developers can encounter when converting data using Streams. In this blog post, we will delve into these pitfalls and equip you with the knowledge needed to master stream conversion effectively.
Understanding Streams
A Stream in Java is a sequence of elements that supports various methods that can be performed on them. Streams can be created from a variety of data sources, such as collections, arrays, or I/O channels. The beauty of Streams lies in their ability to express complex data processing queries succinctly.
Key Characteristics of Streams
- No storage: A Stream does not store elements. Instead, it conveys elements from a data source like a collection, array, or I/O channel.
- Functional in nature: Operations on a Stream are typically done with functions that take advantage of lambda expressions.
- Laziness: Many Streams operations are lazy. They do not compute their results until they are required.
- Possibly unbounded: While some Streams are finite, some can be unbounded as in the case of an infinite stream generation.
A Fundamental Stream Operation: Conversion
In the context of data processing, conversion generally refers to the transformation of one set of data into another. For example, converting a list of strings into a list of their lengths or filtering out certain elements based on specific criteria.
Common Pitfall: Inefficient Stream Conversions
One common pitfall when dealing with Streams is improper handling of intermediate transformations that lead to inefficient code execution. For instance, one may be tempted to create multiple nested operations that could have been accomplished in a single pass.
Let's explore a practical example to illustrate this problem.
Example Code: Inefficient Stream Transformation
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Edward");
// Inefficient conversion
List<Integer> nameLengths = names.stream()
.map(name -> name.length()) // First operation: map to lengths
.filter(length -> length > 3) // Second operation: filter lengths greater than 3
.collect(Collectors.toList()); // Collect the results
System.out.println(nameLengths);
}
}
In the code above, the map()
operation generates a stream of lengths, and then the filter()
operation processes those lengths. This could result in unnecessary iterations over the original dataset.
Why is it inefficient?
- Each intermediate operation processes the entire dataset. As multiple transformations are chained, they lead to multiple passes over the data which negatively affects performance.
- The operations consume memory as intermediate results are maintained.
Stream Optimization: The Better Way
Now, let’s refactor the code to improve efficiency. We can combine transformations into a single pass using a more advanced approach.
Example Code: Optimized Stream Transformation
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Edward");
// Optimizing the conversion
List<Integer> nameLengths = names.stream()
.filter(name -> name.length() > 3) // Filter first
.map(name -> name.length()) // Then map to lengths
.collect(Collectors.toList()); // Collect the results
System.out.println(nameLengths);
}
}
What did we change?
- Moved the
filter()
operation to precede themap()
operation. This way, if the string length is less than or equal to 3, it is filtered out before additional processing occurs. - This change ensures that only the necessary elements are processed by the
map()
operation, thus minimizing overhead.
Utilizing FlatMap: Another Common Pitfall
Another common stream conversion pitfall is improper use of flatMap()
. The flatMap()
operation is used when each element of a stream is mapped to multiple elements in the resulting stream.
Let’s take a look at an example where developers often misuse flatMap()
.
Example Code: Misuse of flatMap
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class FlatMapExample {
public static void main(String[] args) {
List<List<String>> listsOfNames = Arrays.asList(
Arrays.asList("Alice", "Bob"),
Arrays.asList("Charlie", "David"),
Arrays.asList("Edward"));
// Incorrect use of flatMap
List<String> allNames = listsOfNames.stream()
.map(nameList -> nameList.stream()) // Creates a stream of streams
.collect(Collectors.toList()); // Collect as List of streams
System.out.println(allNames);
}
}
Why is this incorrect?
- The code above creates a stream of streams instead of a single stream of names.
- This results in a collection containing multiple inner streams, which is not what is typically intended.
Correcting the Code
Let’s use flatMap()
properly to flatten the structure.
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class FlatMapExample {
public static void main(String[] args) {
List<List<String>> listsOfNames = Arrays.asList(
Arrays.asList("Alice", "Bob"),
Arrays.asList("Charlie", "David"),
Arrays.asList("Edward"));
// Correct use of flatMap
List<String> allNames = listsOfNames.stream()
.flatMap(List::stream) // Flattens the structure
.collect(Collectors.toList()); // Collect as single List
System.out.println(allNames);
}
}
Explanation
- The use of
flatMap(List::stream)
flattens the inner lists into a single stream of names, condensing them into one cohesive collection. - This approach preserves the efficiency of the pipeline and accurately captures your intended data manipulation.
Final Considerations
Mastering Stream conversions in Java 8 is critical for writing efficient and maintainable code. Common pitfalls, such as inefficient processing and improper usage of flatMap()
, can lead to unreliable and sluggish applications.
By refactoring your stream operations thoughtfully and understanding the implications of each transformation, you will harness the full power of Java Streams.
For more detailed insights on Java Streams, you may find the following resources useful:
Happy coding!