Managing Consistency in Transactional NoSQL Databases
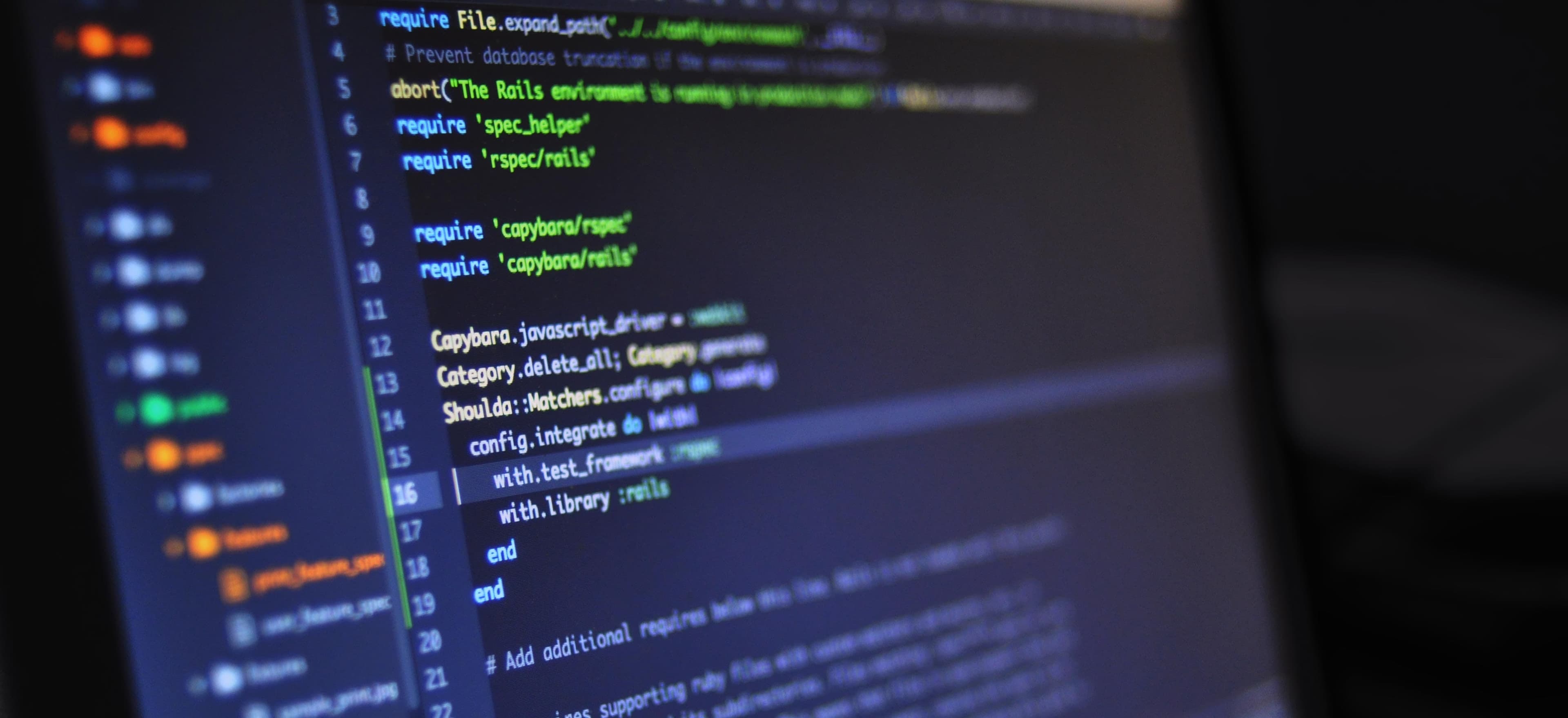
- Published on
Managing Consistency in Transactional NoSQL Databases
In the evolving world of databases, NoSQL systems have emerged as a vital solution, especially when handling large volumes of data with flexible schemas. However, as businesses continue to adopt NoSQL for its scalability and performance, the question arises: how do we manage consistency in transactional NoSQL databases? This article dives deep into the aspects of consistency, the challenges associated with it, and the strategies for achieving it in a NoSQL environment.
Understanding Consistency
What is Consistency?
Consistency refers to the state of a database where all data meets a specific standard and adheres to defined constraints. The ACID (Atomicity, Consistency, Isolation, Durability) properties are typically associated with traditional relational databases. However, NoSQL databases sometimes favor availability and partition tolerance over strict consistency. This trade-off is exemplified by the CAP theorem.
The CAP Theorem
The CAP theorem states that a distributed data store can only guarantee two out of the following three properties at the same time:
- Consistency: Every read gets the most recent write or an error.
- Availability: Every request gets a response, whether successful or failure.
- Partition Tolerance: The system continues to operate despite network partitions.
In many cases, NoSQL databases sacrifice consistency for availability and partition tolerance. For instance, Cassandra uses an eventual consistency model, which raises the stakes for developers to manage data consistency manually.
Types of NoSQL Databases
There are various types of NoSQL databases, each designed for specific use cases:
- Document Stores: e.g., MongoDB, CouchDB
- Key-Value Stores: e.g., Redis, DynamoDB
- Column-Family Stores: e.g., Cassandra, HBase
- Graph Databases: e.g., Neo4j, ArangoDB
Transactional NoSQL Databases
Transactional NoSQL databases, like MongoDB with its multi-document transactions, enable operations across multiple records while ensuring consistency. MongoDB uses a model that supports ACID transactions, promoting safer data operations.
Why is Consistency Important?
Managing consistency is crucial for several reasons:
- Data Integrity: Ensures that all data adheres to the rules set by the business logic.
- User Trust: Consistent data fosters user confidence in applications.
- Business Decision Making: Consistent data allows for accurate reports and analytics.
Strategies for Managing Consistency in NoSQL Databases
Achieving consistency in a NoSQL database can be a challenge, but various strategies can be employed:
1. Use of ACID Transactions
Many NoSQL databases now support ACID transactions. For example, MongoDB enables transactions that span multiple documents.
const session = client.startSession();
session.startTransaction();
try {
await collection1.insertOne({ key: "value1" }, { session });
await collection2.updateOne({ key: "value2" }, { $set: { field: "updatedValue" } }, { session });
await session.commitTransaction();
} catch (error) {
console.error("Transaction aborted due to an error: ", error);
await session.abortTransaction();
} finally {
session.endSession();
}
Why Use ACID Transactions?
Utilizing transactions ensures all operations within the block are executed successfully, or none at all. This is particularly important in scenarios where data integrity across different contexts is critical.
2. Distributed Consensus Algorithms
Consensus algorithms like Raft and Paxos ensure all nodes in a distributed system agree on the state of the data. This can be vital for applications requiring strong consistency.
-
Raft: Focuses on consensus for maintaining a distributed log. It's simpler to understand than Paxos and has become quite popular.
-
Paxos: A more complex method often used in distributed systems for agreement on values.
Implementing these algorithms can help manage replicas efficiently, ensuring that all nodes reflect the same data.
3. Quorum Reads and Writes
A quorum is the minimum number of votes required before proceeding with a transaction. This is especially effective in distributed database scenarios.
For example, in a database leveraging quorum-based replication:
// Simple pseudocode
readResult = database.readFromNNodes(n);
if (quorumAchieved(readResult)) {
return mergeResults(readResult);
}
Why Use Quorum Reads and Writes?
Quorum operations ensure that a read or write is only considered successful when a minimum number of replicas respond. It reduces the chances of reading stale data while balancing performance and consistency.
4. Data Versioning
Data versioning allows you to keep track of changes made to records. Each change is assigned a unique version, thus providing a clear history of modifications.
class Document:
def __init__(self, content):
self.content = content
self.version = 0
def update(self, new_content):
self.version += 1
self.content = new_content
Why Use Data Versioning?
Versioning allows systems to access previous states of data, enabling effective conflict resolution and maintaining integrity during concurrent operations.
5. Event Sourcing
Event sourcing captures changes to the application state as a sequence of events, making it possible to recreate the current state from its history.
class Event:
def __init__(self, event_type, data):
self.event_type = event_type
self.timestamp = datetime.now()
self.data = data
# Store events
events = []
events.append(Event("USER_SIGNUP", {"username": "user1"}))
Why Use Event Sourcing?
Using event sourcing can lead to better auditing, since each action is recorded, making it much easier to track changes and troubleshoot issues.
Challenges in Achieving Consistency
Despite the aforementioned strategies, consistency in NoSQL databases does come with its set of challenges:
-
Latency: Ensuring consistency can introduce additional latency, as transactions may have to wait for acknowledgments from multiple nodes.
-
Complexity: Implementing consensus algorithms and other strategies can add complexity to the system architecture.
-
Scalability: Maintaining consistency in a highly scalable environment requires careful design to maintain performance.
The Closing Argument
Managing consistency in transactional NoSQL databases requires a combination of techniques tailored to the specific use case. As organizations continue to leverage the strengths of NoSQL databases, understanding the balance between consistency, availability, and partition tolerance becomes crucial.
By using ACID transactions, distributed consensus algorithms, quorum reads/writes, data versioning, and event sourcing, businesses can significantly improve their data integrity and availability.
Additional Resources
For further reading on the intricacies of NoSQL databases and consistency management, consider these resources:
- MongoDB Transactions
- Understanding the CAP Theorem
Investing time in mastering these techniques will surely pay off as you build scalable, resilient applications capable of maintaining consistency in a distributed system.
Checkout our other articles