Resolving Orphan Removal Issues in Hibernate By Example
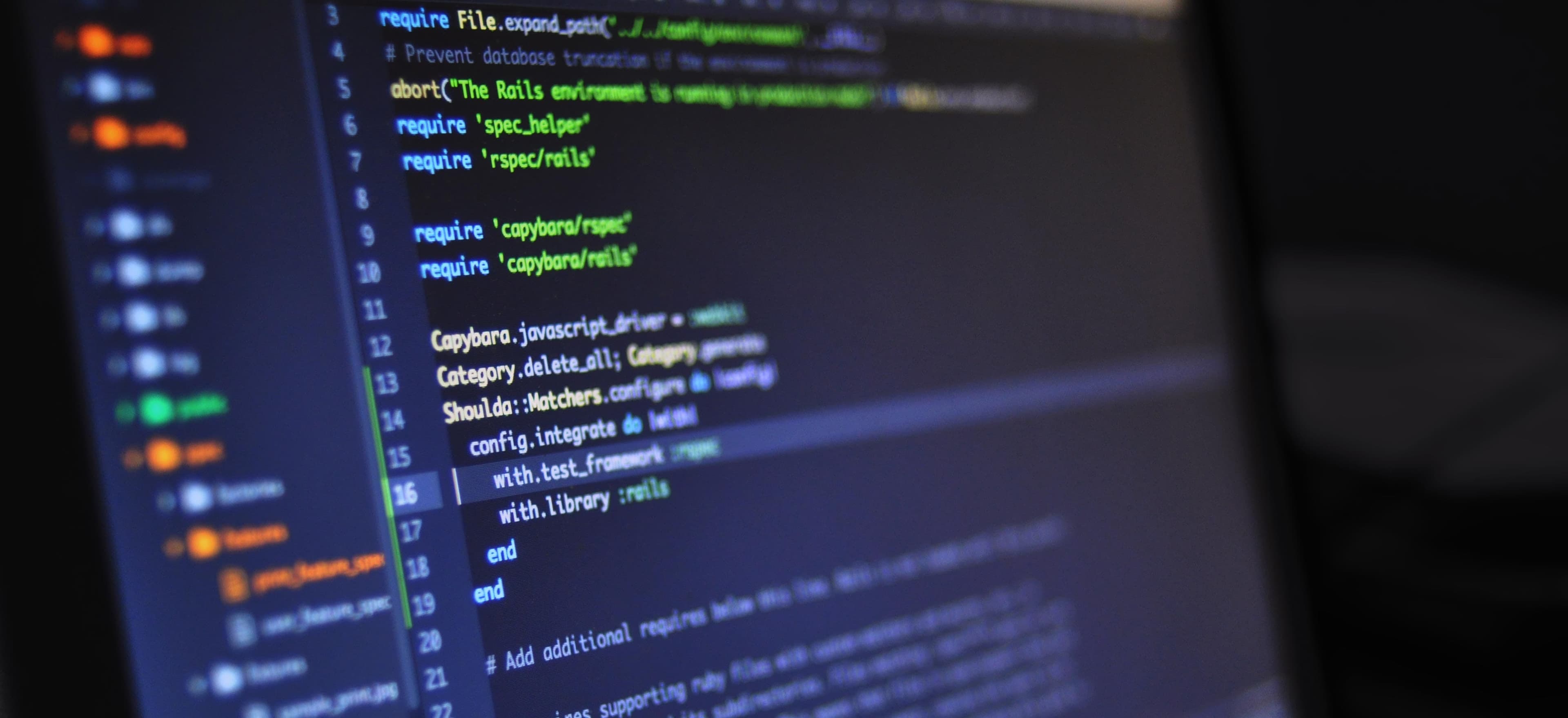
- Published on
Resolving Orphan Removal Issues in Hibernate: An In-Depth Guide
Hibernate is a powerful Object-Relational Mapping (ORM) framework for Java applications. It simplifies database interactions by providing an abstraction layer over JDBC. However, like any powerful tool, it can present challenges, particularly when it comes to managing lifecycle states of entities. One such challenge is the concept of orphan removal. In this blog post, we will explore what orphan removal means in Hibernate, the problems it can introduce, and how to resolve these issues effectively.
Understanding Orphan Removal
What is Orphan Removal?
In Hibernate, orphan removal refers to the automatic deletion of child entities when they are removed from their parent entity's collection. For instance, consider a Cart
entity that contains multiple Item
entities:
@Entity
public class Cart {
@OneToMany(mappedBy = "cart", orphanRemoval = true, cascade = CascadeType.ALL)
private List<Item> items = new ArrayList<>();
// getters and setters
}
In this scenario, if an Item
is removed from the items
list, Hibernate handles the deletion of that Item
from the database automatically. This feature can help prevent orphaned records but can also lead to unintentional deletions when not managed properly.
Challenges of Orphan Removal
Common Issues
-
Unintended Deletion: As mentioned, inadvertently removing a child entity from a collection can lead to unintended deletions. This might happen if the removal is performed based on user interactions, such as removing an item from a shopping cart.
-
Entity State Management: Understanding entity state and lifecycle is vital. If you're working with detached entities or merging changes, orphan removal might execute in ways you didn't anticipate.
-
Performance Concerns: If you have a large number of child entities being managed, frequent changes to collections can lead to performance degradation.
How to Resolve Orphan Removal Issues
1. Understanding the Cascade Types
Before diving into code examples, it’s essential to understand the role of cascade
in the context of orphan removal:
CascadeType.ALL
: All operations will be cascaded (including orphan removal).CascadeType.PERSIST
: Only persist operations will cascade.CascadeType.REMOVE
: Only remove operations will cascade.
By explicitly choosing the cascade type, you gain better control over how child entities interact with their parent. For example, you can prevent the unwanted deletion of child entities by selecting only CascadeType.PERSIST
.
2. Implementing Safeguards
Example: Using a DTO Pattern
One effective strategy to safeguard against unintended deletions is to utilize a Data Transfer Object (DTO). A DTO can encapsulate the data structure without directly affecting the entity relationships.
Here is how you can implement a DTO for a cart operation:
public class CartDTO {
private List<Long> itemIds;
// getters and setters
}
Using this CartDTO
, you can transfer only the necessary data and do not directly manipulate the Cart
entity's collection. This makes it less likely for you to unintentionally trigger orphan removal.
3. Explicitly Remove Child Entities
If you need to remove a child entity but want to ensure it doesn’t get orphaned, consider using explicit removal methods. This way, you have full control over deletion:
@Transactional
public void removeItemFromCart(Cart cart, Item itemToRemove) {
cart.getItems().remove(itemToRemove); // This will trigger orphan removal automatically
// Instead, let's do this
itemToRemove.setCart(null); // Detach the item without triggering orphan removal
// Persist the change if necessary
session.merge(cart); // Ensure the cart is merged back to the session
}
By removing the association rather than the entity itself from the parent collection, you avoid triggering orphan removal.
4. Using Soft Deletes
When orphan removal is too risky, consider implementing a soft delete strategy through an isDeleted
flag pattern. Instead of deleting records, you simply mark them as deleted:
@Entity
public class Item {
private boolean isDeleted = false;
// Mark as deleted
public void markAsDeleted() {
this.isDeleted = true;
}
// getters and setters
}
With this approach, your database remains intact, while you control which records should be shown or hidden at an application level.
Closing the Chapter
Orphan removal in Hibernate is a powerful yet potentially troublesome feature. Understanding its implications and managing its usage carefully can save developers significant headaches.
Through using aware strategies such as DTO patterns, explicit removal, and soft delete implementations, you can maintain data integrity and prevent unintended data loss.
If you’re eager to delve deeper into Hibernate and ORM design, consider exploring additional resources such as Hibernate Documentation and other Java ORM best practices.
By mastering orphan removal, you can leverage the full power of Hibernate while avoiding common pitfalls, leading to more robust applications. Happy coding!
References
By understanding these elements, you’re well on your way to confidently resolving orphan removal issues in Hibernate.
Checkout our other articles