Streamlining Parameterization with DataProvider in TestNG
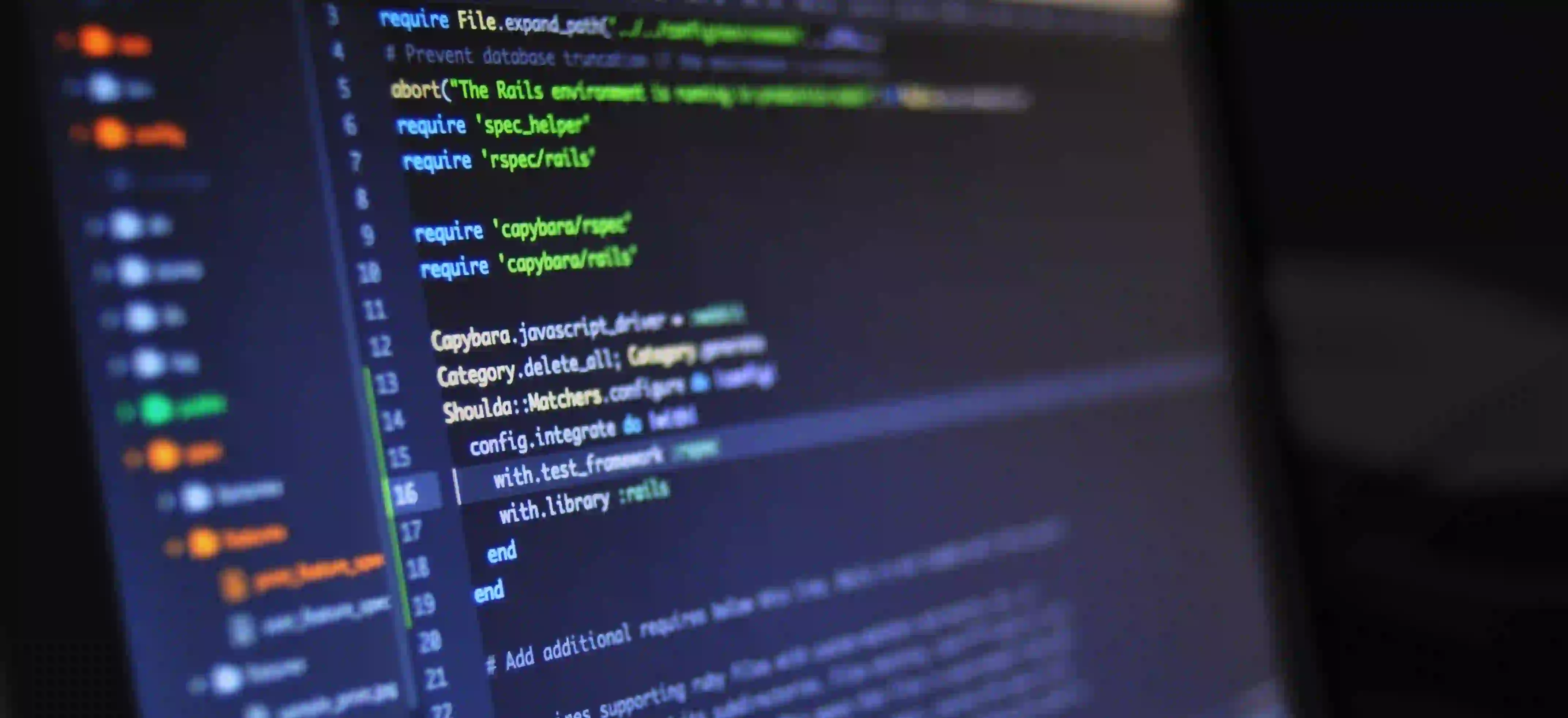
Streamlining Parameterization with DataProvider in TestNG
Testing is an essential aspect of software development. In Java, TestNG is a robust testing framework that allows developers to write test cases with ease. One of the critical features of TestNG is its data-driven testing capabilities, primarily achieved through the use of the DataProvider annotation. This feature ensures your tests are more flexible and dynamic by allowing you to run the same test method with different inputs.
In this blog post, we will explore the concept of parameterization in TestNG using DataProviders. Along the way, we will enhance our understanding of how to streamline test execution, improve code maintainability, and boost test coverage.
What is Parameterization in Testing?
Parameterization in testing allows you to execute the same test with different sets of input data. This technique is useful for scenarios where the same functionality needs to be validated across various parameters. Instead of writing multiple methods for different input combinations, Parameterization helps you achieve this in a cleaner, more efficient way.
Why Use DataProvider?
- Simplicity: Instead of annotating each method with multiple parameters, DataProviders consolidate this into a single location.
- Flexibility: Easily change test data in the DataProvider without altering test logic.
- Reusability: DataProviders can be reused across multiple test classes or methods.
Implementing TestNG DataProvider
To showcase DataProvider, let’s start with a simple test case — a calculator application that adds two numbers.
Step 1: Setting Up TestNG
First, ensure you have TestNG set up in your Java project. You can add TestNG using Maven by adding the following dependency in your pom.xml
:
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.3.0</version>
<scope>test</scope>
</dependency>
Step 2: Creating the Calculator Class
Let’s create a simple Calculator
class that has an addition method.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Step 3: Building the Test Class with DataProvider
Now, we’ll create a test class to validate the addition method using a DataProvider.
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
import static org.testng.Assert.assertEquals;
public class CalculatorTest {
Calculator calculator = new Calculator();
@DataProvider(name = "additionData")
public Object[][] additionData() {
return new Object[][] {
{1, 2, 3}, // Test case 1: 1 + 2 = 3
{5, 5, 10}, // Test case 2: 5 + 5 = 10
{0, 0, 0}, // Test case 3: 0 + 0 = 0
{-1, 1, 0}, // Test case 4: -1 + 1 = 0
{Integer.MAX_VALUE, 1, Integer.MIN_VALUE} // Test case 5: Edge case
};
}
@Test(dataProvider = "additionData")
public void testAdd(int a, int b, int expected) {
assertEquals(calculator.add(a, b), expected, "Sum of " + a + " and " + b + " should be " + expected);
}
}
What's Happening in This Code?
-
DataProvider Annotation: The
@DataProvider
annotation specifies thatadditionData()
serves as the source of test data. The name attribute identifies it for use in the test method. -
Data Format: The
additionData()
method returns a two-dimensionalObject
array. Each inner array represents test data for one test case. -
Test Method: The
testAdd
method is annotated with@Test
and references the DataProvider by its name. It receives parameters for each test case defined inadditionData()
. -
Assertion: The
assertEquals
method checks the actual result against the expected value for each input set.
Running the Tests
To run your tests with TestNG, you can use an IDE (like IntelliJ IDEA or Eclipse) with TestNG support, or you can run your tests from the command line using Maven:
mvn test
Step 4: Analyzing the Results
Once your tests execute, you’ll see output in the console or in the TestNG Results tab of your IDE. Each test case will either pass or fail depending on whether the assertion holds true.
Advantages of Using DataProvider in TestNG
- Easier Maintenance: Modify test data in a single DataProvider method instead of changing multiple test methods.
- Clearer Test Cases: Your test method implementation remains focused on testing logic, improving readability.
- Increased Test Coverage: Testing multiple scenarios with minimal effort helps cover edge cases more thoroughly.
Real-world Application Scenarios for DataProvider
- Web Form Testing: Validate multiple scenarios for user input fields (e.g., login tests).
- API Testing: Test REST APIs with various request parameters and expected responses.
- Performance Testing: Assess application behavior with various data sets simulating user loads.
For even more insights on how to leverage TestNG effectively, you can browse these TestNG Basics and DataProvider Guide.
Closing Remarks
Parameterization using DataProvider in TestNG is a powerful feature designed to make your testing process more efficient. By adopting this methodology, you can enhance your test coverage, simplify maintenance, and keep your testing structured.
With careful implementation, your tests can remain robust against changes and easily extensible for future scenarios. TestNG's intuitive approach to parameterization allows you to enforce a higher standard in your testing practices.
Happy Testing! 🌟