Common Gradle Errors Beginners Face in Java Projects
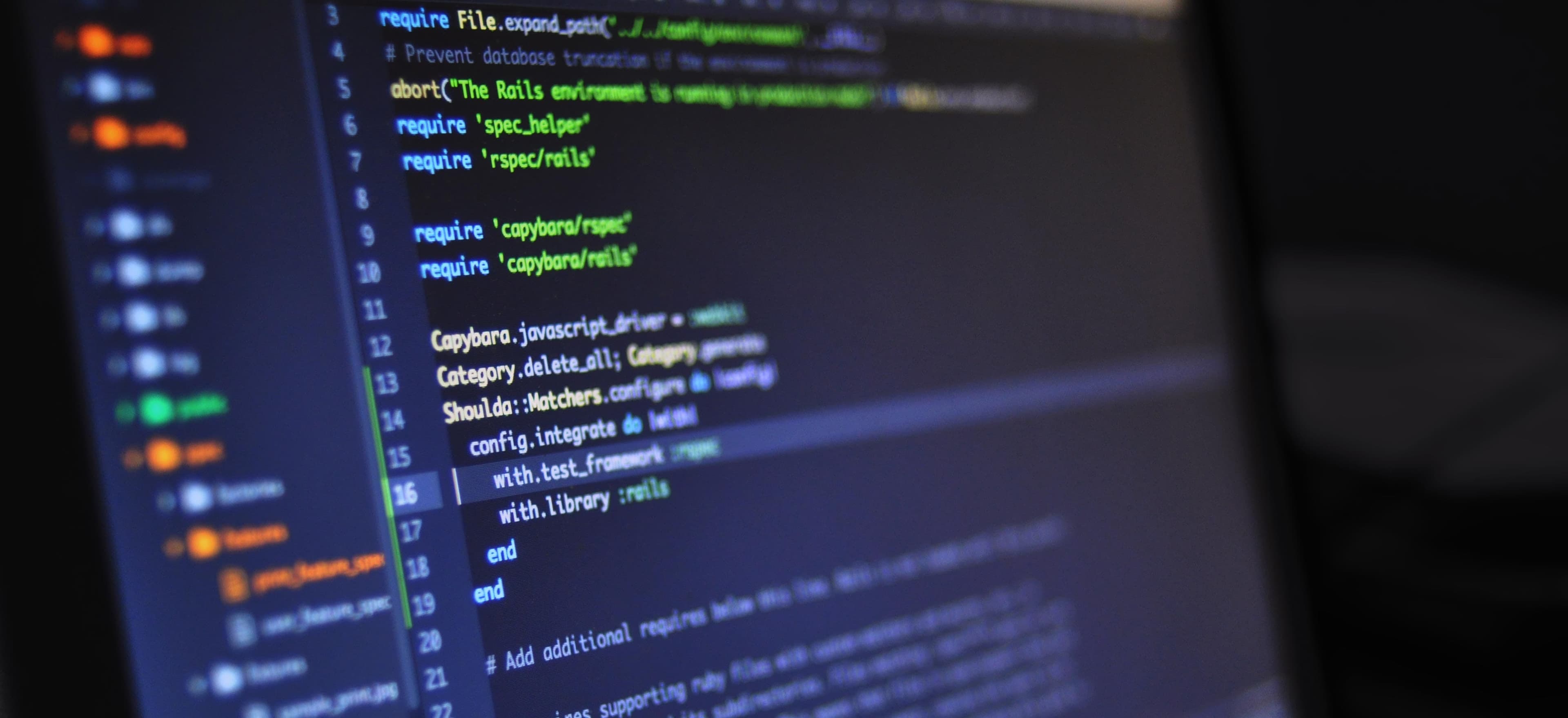
- Published on
Common Gradle Errors Beginners Face in Java Projects
Gradle has transformed the way developers build and manage Java projects. Its flexibility and powerful features make it a favorite in the software development community. However, as with any tool, beginners often face hurdles when they are just starting out. In this blog post, we will explore common Gradle errors that Java developers encounter and provide actionable solutions to help you overcome them.
What is Gradle?
Before we dive into the errors, let us briefly explain what Gradle is. Gradle is an open-source build automation tool that is used primarily for Java applications but also supports other languages. It uses Groovy or Kotlin DSL (Domain-Specific Language) to define project configurations. This flexibility allows for seamless integration with various development environments and cloud services.
1. Gradle Wrapper Issues
The Gradle Wrapper is designed to ensure that everyone working on the project uses the same Gradle version. However, beginners often run into issues when the wrapper is not set up correctly.
Common Error Message:
Could not determine the dependencies of task ':app:compileJava'.
Solution:
-
Create or update your
gradle-wrapper.properties
file to define the proper Gradle version. You can generate it by running:gradle wrapper --gradle-version=your_version_here
-
After updating, execute:
./gradlew build
This will ensure that the correct Gradle version is used.
Commentary:
Using the Gradle Wrapper prevents compatibility issues among team members. Always ensure the version is up to date.
2. Dependency Resolution Errors
Dependency management is a critical aspect of any project. Beginners often forget to declare library dependencies in the build.gradle
file.
Common Error Message:
Could not resolve all dependencies for configuration ':app:compileClasspath'.
Solution:
Check your build.gradle
file for completeness and accuracy. Here is an example of how to declare dependencies properly:
dependencies {
implementation 'org.springframework:spring-web:5.3.8' // Use latest version
testImplementation 'junit:junit:4.13.2'
}
Commentary:
Misdeclared dependencies can lead to runtime errors. Using the latest versions can also help you leverage new features and security fixes.
3. Plugin Application Failure
Sometimes, when developers try to apply plugins, they encounter errors due to plugin misconfigurations.
Common Error Message:
Plugin with id 'java' not found.
Solution:
Make sure you add the correct plugin declaration in the build.gradle
file:
plugins {
id 'java'
}
If you are using an external plugin, ensure it is declared correctly:
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath 'com.github.johnrengelman.shadow:shadow-gradle-plugin:7.1.0'
}
}
Commentary:
Use the correct plugin version to avoid issues. Always refer to the plugin's official site for the right implementation.
4. Gradle Daemon Problems
The Gradle Daemon is a background process that helps in speeding up the build process. However, it can sometimes cause issues, especially for beginners.
Common Error Message:
A problem occurred configuring root project 'project_name'.
Solution:
If you encounter Daemon issues, you might want to stop the daemon and restart Gradle:
./gradlew --stop
You can also add the following line to your gradle.properties
to reduce memory consumption:
org.gradle.daemon=false
Commentary:
Managing the Daemon wisely can save you time and system resources. Regularly check your Daemon processes to avoid conflicts.
5. Incorrect Project Structure
A proper project structure is essential for any Gradle project. Beginners often place files in incorrect directories.
Common Error Message:
Could not find property 'sourceSets' on root project 'project_name'.
Solution:
Follow the standard Gradle structure:
project_root
|-- build.gradle
|-- settings.gradle
|-- src
| |-- main
| | |-- java
| | |-- resources
| |-- test
| |-- java
| |-- resources
Your build.gradle
should reference the correct source sets:
sourceSets {
main {
java {
srcDirs = ['src/main/java']
}
resources {
srcDirs = ['src/main/resources']
}
}
}
Commentary:
Correct project structuring helps in maintaining clarity and coherence in your development process. Following established conventions can save you time and effort.
6. Outdated Cache Issues
Gradle can run into problems with its cache system, leading to unexpected behavior.
Common Error Message:
Could not resolve all files for configuration ':app:compileClasspath'.
Solution:
Clear the Gradle cache using:
./gradlew clean
./gradlew build --refresh-dependencies
This forces Gradle to ignore caches for a fresh build.
Commentary:
Caching issues can often hinder productivity. Regularly refreshing dependencies ensures the latest libraries and their configurations are always utilized.
7. Environmental Configuration Mistakes
Setting up your development environment properly is crucial. Beginners may experience issues due to incorrect JAVA_HOME configurations or unsupported JDK versions.
Common Error Message:
Unsupported major.minor version 52.0
Solution:
-
Check your
JAVA_HOME
environment variable to confirm it points to the JDK path. -
Make sure you are using a supported version of JDK:
java -version
-
Update the JDK if necessary and ensure that the Gradle version is compatible.
Commentary:
Different Gradle versions might have different compatibility requirements with JDK. Make a habit of checking documentation for recommended setups.
The Last Word
Gradle can be a powerful tool in your Java development toolkit if configured correctly. By understanding and addressing these common errors, beginners can significantly streamline their build process. Moreover, keeping abreast of updates and following best practices will put you on a path to success.
For more in-depth tutorials on Gradle, consider visiting the Gradle User Manual.
Embrace the learning curve—each mistake is an opportunity to grow. Whether you are crafting a simple console application or a complex enterprise system, mastering Gradle will undeniably enhance your development experience. Happy coding!
Checkout our other articles