TDD Myths: Does It Hinder Innovative Design?
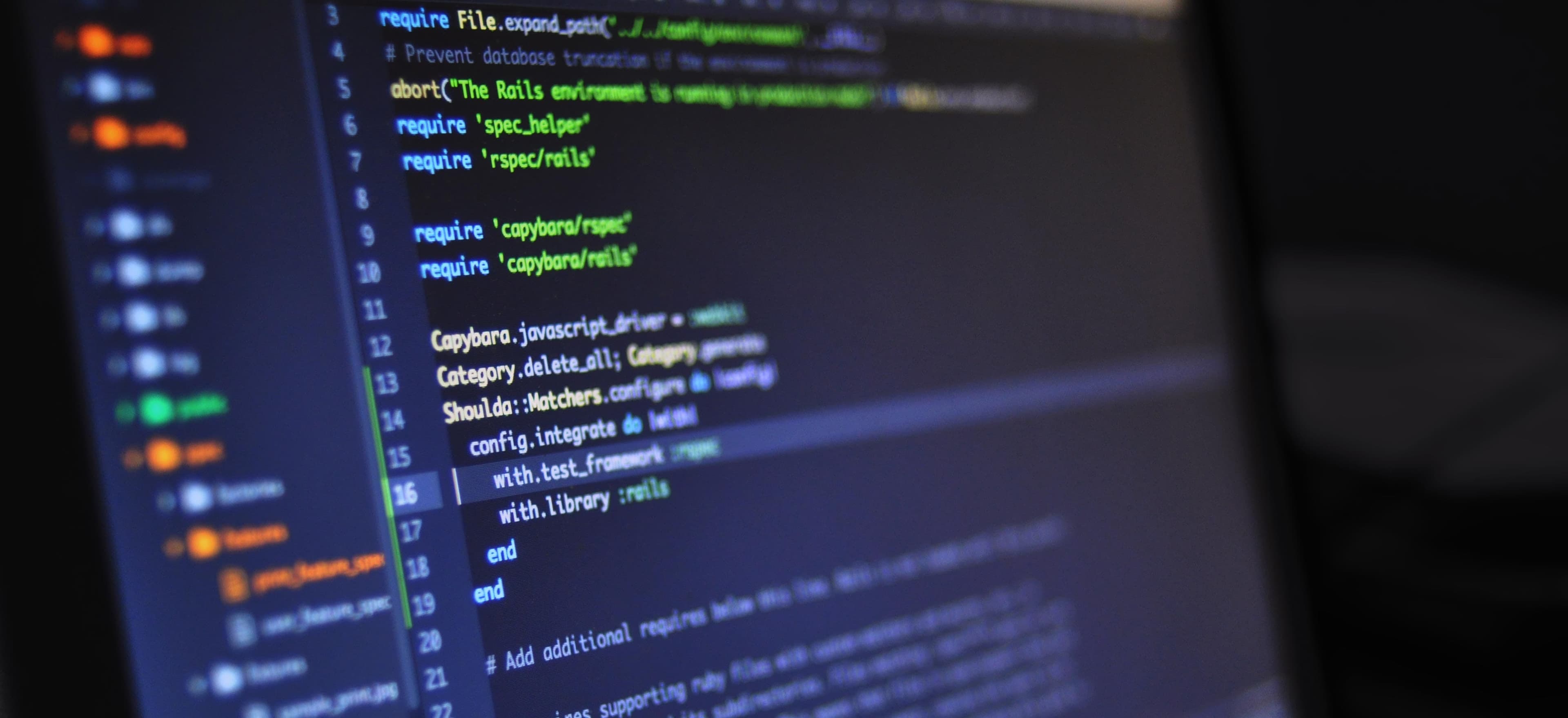
- Published on
TDD Myths: Does It Hinder Innovative Design?
Test-Driven Development (TDD) has garnered significant attention in the software development community. It is often praised for enhancing code quality and encouraging better design practices. However, there remains an ongoing debate about its potential limitations, including whether TDD stifles innovative design. In this blog post, we will explore these myths, dissect the realities of TDD, and see how it can both enhance and potentially hinder creativity in software design.
Table of Contents
- What is TDD?
- Common Myths Surrounding TDD
- How TDD Promotes Good Design
- The Case for Innovation: Does TDD Hinder Creativity?
- Conclusion
What is TDD?
Test-Driven Development is a software development approach where tests are written before the actual code. This process typically follows these steps:
- Write a Test: A test reflecting a specific function or feature should be created first.
- Run the Test: The test should fail initially since the corresponding feature has not yet been implemented.
- Write Code: Implement just enough code to ensure the test passes.
- Refactor: Clean up the code while ensuring that all tests still pass.
Example of TDD in Java
Below is a simple example demonstrating TDD in Java:
// Step 1: Write a test
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
// Step 3: Write the code to pass the test
class Calculator {
public int add(int a, int b) {
return a + b; // Simple addition implementation
}
}
Commentary
In this code snippet, we start by defining the expected behavior via a test before implementing the corresponding functionality in the Calculator
class. This ensures that our design is driven by requirements, which can lead to better alignment with user needs.
Common Myths Surrounding TDD
-
TDD is Time-Consuming: Many developers argue that writing tests first prolongs the development cycle.
-
TDD Limits Design Freedom: There is a belief that TDD constrains developers to implement features only in ways that are testable.
-
TDD is Only for Unit Testing: While TDD is often associated with unit tests, it can also be applied to integration and acceptance testing.
-
Formal Testing Is Boring: Beginners may find it cumbersome and may not see the value TDD brings.
-
TDD is a One-Size-Fits-All Approach: Each project is unique, and TDD may not suit every development requirement.
How TDD Promotes Good Design
Even though there are myths, TDD can lead to good design in multiple ways:
- Improved Code Quality: Since tests are written first, code becomes less prone to bugs and issues.
- Refactoring with Confidence: Developers can enhance or modify existing code knowing that the accompanying tests will catch regressions.
- Code Readability: New developers can more easily understand what the code is supposed to achieve just by looking at the tests.
- Early Bug Detection: Since tests are written first, students can identify issues before they escalate.
Example of Refactoring in TDD
To illustrate the benefit of the refactoring process in TDD, consider the following code:
class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
// Refactoring to create an interface
interface MathOperation {
int operate(int a, int b);
}
class Adder implements MathOperation {
@Override
public int operate(int a, int b) {
return a + b;
}
}
class Subtractor implements MathOperation {
@Override
public int operate(int a, int b) {
return a - b;
}
}
By refactoring, we decouple the operations into separate classes. This increases code maintainability while ensuring that all existing tests continue to pass.
The Case for Innovation: Does TDD Hinder Creativity?
While TDD promotes structured design, some argue that it can create rigidity in an otherwise flexible development approach. Here’s how:
Creativity is Constrained by Tests
Writing tests before code might influence developers to adhere strictly to predefined designs, limiting their creative potential. The fear of breaking the tests can inhibit exploration of alternative solutions or creative approaches.
Overemphasis on Code
Focusing heavily on tests could lead developers to miss the big picture of end-user requirements. For instance, if developers fixate solely on passing tests, they may neglect broader aspects such as usability and user experience.
Risk of Overengineering
In their pursuit of satisfying tests, developers might end up writing overly complex code. Consequently, the initial straightforward design could become convoluted as developers adjust the code just to adhere to TDD principles.
Balancing TDD with Innovation
-
Flexible Approach: It is important to see TDD as a tool and not a dogma. Having a more flexible approach allows development teams to adapt the practice to their needs.
-
Embrace Exploration: It's essential to keep space for innovation in the development process. Design sprints, brainstorming, and prototyping sessions can complement TDD practices.
-
Leverage Pair Programming: Working alongside another developer can provide perspectives that may suggest alternative solutions, bridging creativity with TDD.
The Last Word
TDD is a powerful approach to software development, but it is not without its challenges. By addressing the myths surrounding TDD, we can understand its true nature in promoting good design. While it can potentially hinder innovation if misapplied, a balanced approach can harness the strengths of TDD while allowing room for creative solutions.
For further reading on TDD, consider exploring the resources at Martin Fowler's TDD page and Kent Beck's writings on Extreme Programming.
By seeing TDD as a framework rather than a limit, we can find innovative ways to improve our code while meeting user needs effectively.