Overcoming Conflicts When Mapping Objects to XML Schemas
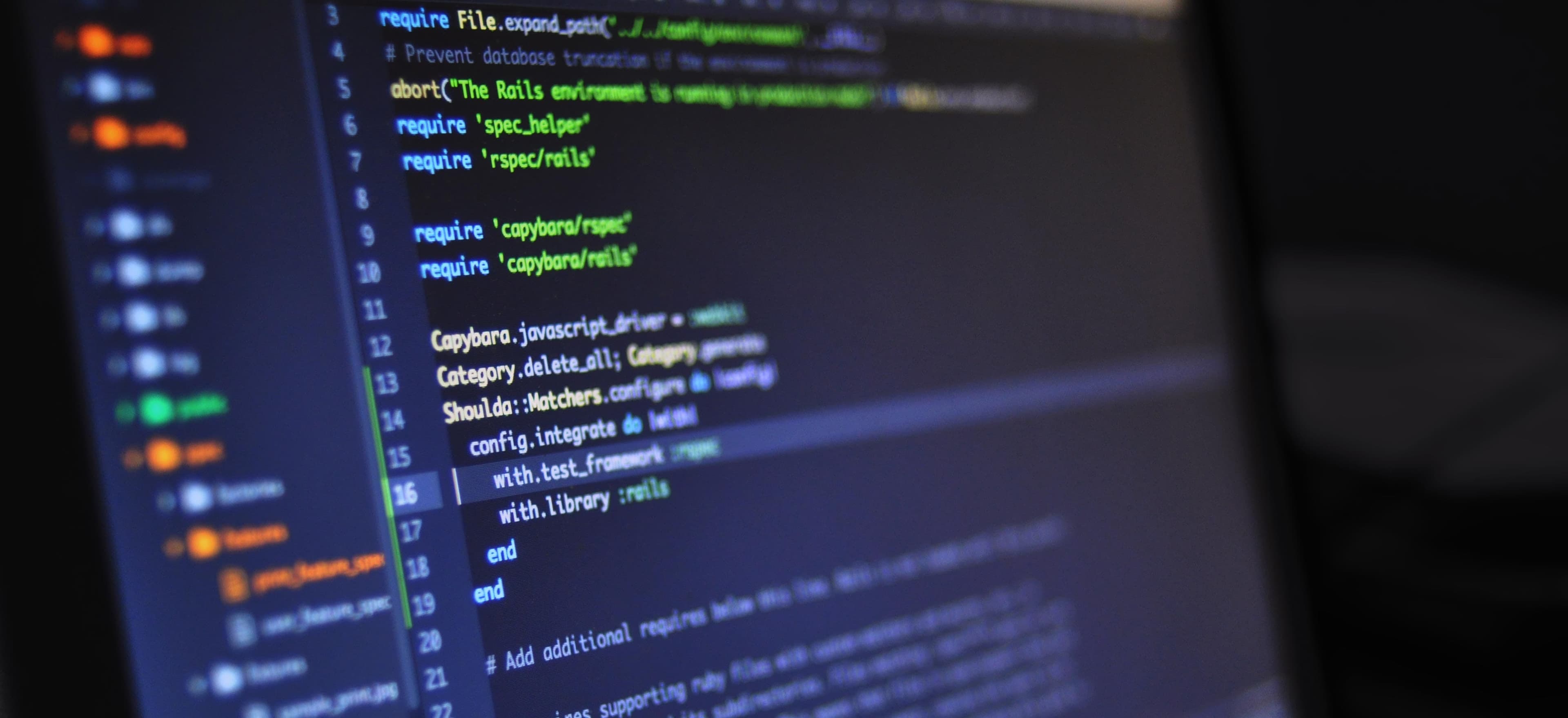
- Published on
Overcoming Conflicts When Mapping Objects to XML Schemas
Mapping Java objects to XML schemas can be a daunting task for developers, especially given that object-oriented programming (OOP) and XML may have fundamentally different structures. Conflicts often arise due to differences in how data types are represented, how complex objects are handled, or even how attributes and elements are defined. This blog post will delve into these challenges, examine effective solutions, and provide valuable code snippets to illustrate best practices in resolving conflicts when mapping objects to XML.
Understanding the Basics
Before tackling the conflicts, let’s revisit the components involved in the mapping process. Java objects are instances of classes, encapsulating data and behavior. On the other hand, XML (eXtensible Markup Language) is a markup language used to store and transport data in a structured way.
The Challenges of Mapping
Some common challenges faced include:
- Type Mismatches: XML schemas define strict data types, while Java may allow more flexible representation of these types.
- Hierarchical Structure: XML emphasizes tree structures, which can cause conflicts with Java’s object hierarchy.
- Naming Conflicts: Inconsistent naming conventions can lead to difficulties in mapping attributes and child elements.
- Element vs. Attribute Representation: XML allows data to be represented as either elements or attributes, leading to potential interpretation discrepancies.
Solution Strategies
To overcome these conflicts, let’s utilize a few strategies that can simplify the mapping process.
1. JAXB for XML Binding
Java Architecture for XML Binding (JAXB) is a key technology simplifying XML manipulation. It allows you to convert Java objects into XML and vice versa. Below is a basic setup using JAXB.
Code Snippet
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import java.io.File;
// Define your Java class
@XmlRootElement
public class Person {
private String name;
private int age;
// Default constructor is necessary for JAXB
public Person() {}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@XmlElement // Annotate your fields
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlElement
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
// Marshalling to XML
public void marshalToXML() {
try {
Person person = new Person("John Doe", 30);
JAXBContext context = JAXBContext.newInstance(Person.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.TRUE);
marshaller.marshal(person, new File("person.xml"));
} catch (JAXBException e) {
e.printStackTrace();
}
}
Commentary
In the snippet above, we have defined a Person
class with JAXB annotations. The @XmlRootElement
annotation indicates the entry point for marshalling. By using JAXB, we avoid manual parsing and ensure that the structure of the XML directly reflects our Java model. This method allows for streamlined conflict resolution due to consistent mapping rules.
For deeper insights into JAXB and its functionalities, you can check the official JAXB documentation.
2. Custom Mapping with Simple XML Framework
Should JAXB not meet your needs—particularly in cases with complex mappings—consider using frameworks like Simple XML. It provides fine-grained control over mapping.
Code Snippet
import org.simpleframework.xml.Element;
import org.simpleframework.xml.Root;
import org.simpleframework.xml.Serializer;
import org.simpleframework.xml.core.Persister;
@Root
public class Company {
@Element(name = "companyName")
private String name;
@Element(name = "foundedYear")
private int yearFounded;
// Default constructor
public Company() {}
// Parameterized constructor
public Company(String name, int yearFounded) {
this.name = name;
this.yearFounded = yearFounded;
}
public static void main(String[] args) {
Serializer serializer = new Persister();
File result = new File("company.xml");
try {
// Serialize the Java object to XML
Company company = new Company("Tech Inc", 2021);
serializer.write(company, result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
In this example, we used Simple XML for conversion. The @Root
and @Element
annotations allow mapping to simpler XML structures. This approach is more flexible and often easier to customize, providing the freedom to resolve conflicts without being heavily locked into Java's native mapping conventions.
3. Naming Scheme Management
Perhaps the most overlooked aspect of XML mapping conflicts lies in naming conventions. A robust naming scheme can prevent ambiguity and ensure consistency.
Best Practice
- Stick to a convention: Use consistent casing, such as camelCase or snake_case.
- Define a mapping layer: Create a dedicated class to handle name conversions between Java and XML.
public class NameMapper {
public static String toXMLName(String javaName) {
// Convert camelCase to snake_case
return javaName.replaceAll("([a-z])([A-Z])", "$1_$2").toLowerCase();
}
}
In Conclusion, Here is What Matters
Mapping Java objects to XML schemas need not be an intimidating process. By leveraging powerful tools such as JAXB and Simple XML, managing naming conventions, and keeping a keen eye on potential conflicts, you can ensure a smoother, more efficient workflow.
Whether you're working on a small project or a complex enterprise application, understanding these methodologies will greatly enhance the way you handle XML data binding in Java. To delve deeper into object serialization, explore articles on object transformation techniques, such as JSON and XML transformations.
Incorporate these lessons into your development workflow. You'll not only overcome mapping conflicts but also write cleaner, more maintainable code. Happy coding!