How DI Containers Can Ruin Your Code Quality
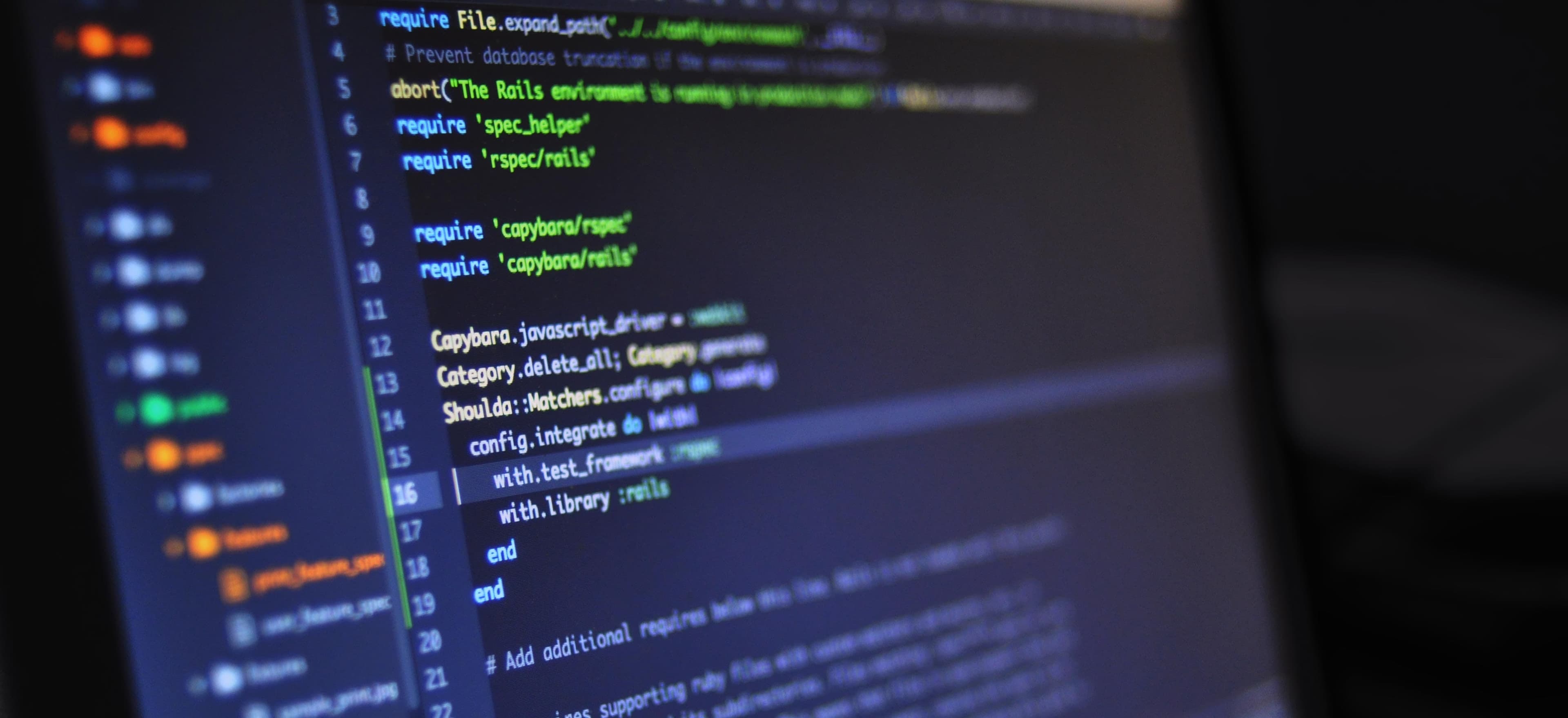
- Published on
How DI Containers Can Ruin Your Code Quality
Dependency Injection (DI) containers have become a popular tool among Java developers. They are often touted as a way to streamline application development by managing object creation, controlling dependencies, and promoting loose coupling. However, lurking beneath their elegant facade, DI containers can lead developers into various pitfalls that ultimately degrade code quality. In this post, we will explore how DI containers can impact your code's maintainability, readability, and testability.
The Allure of DI Containers
At their core, DI containers provide a way to automate the wiring of dependencies in your application. By handling object creation and injection, they promise cleaner code and simpler testing. In theory, this results in:
- Decoupled Components: Each component can focus on its primary task without worrying about how its dependencies are instantiated.
- Easier Tests: You can easily fake or mock dependencies, which can lead to more straightforward testing strategies.
However, this is where the complexity starts to creep in.
1. Complexity Hides Behind Abstraction
When you employ a DI container, your application's dependencies are managed by a third-party system. While this can simplify some tasks, it often obscures how objects are instantiated and their dependencies are resolved.
Example of a Simple DI Setup
Consider a basic example where we have a UserService
that requires a UserRepository
.
public class UserRepository {
public User findById(int id) {
// Query for user
return new User(id, "John Doe");
}
}
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUser(int id) {
return userRepository.findById(id);
}
}
When using a DI container, all of this wiring is abstracted:
@Configuration
public class AppConfig {
@Bean
public UserRepository userRepository() {
return new UserRepository();
}
@Bean
public UserService userService(UserRepository userRepository) {
return new UserService(userRepository);
}
}
Why This Matters
While this code looks clean, the actual construction and lifecycle management can become obscured as your application grows.
When developers new to the project come in, they might have no idea where the UserRepository
instance is created, making it difficult to trace bugs or make alterations. This obscured behavior can ultimately lead to confusion, leading to increased onboarding time for new developers.
2. Overuse of Singletons
DE containers often encourage the use of singleton patterns. While singletons might seem efficient, they can manifest serious issues such as:
- Statefulness: If your singleton instances maintain state, this can lead to unpredictable behavior, especially in concurrent environments.
- Tight Coupling: Over-reliance on singletons makes changing these classes difficult without refactoring various parts of your application.
Example of a Singleton Repository
public class UserRepository {
private static UserRepository instance;
private UserRepository() {}
public static synchronized UserRepository getInstance() {
if (instance == null) {
instance = new UserRepository();
}
return instance;
}
}
Why This Matters
While using singletons might reduce the need for dependency management, it comes with significant trade-offs. Testing the UserRepository
class in isolation becomes challenging, as its state persists across tests, leading to false positives or negatives.
3. Hiding Dependencies
A common anti-pattern in DI containers is the tendency to inject dependencies at a very high level, thereby hiding the real dependencies of a class. This can lead to code that is misleading or difficult to maintain.
Example of Hiding Dependencies
public class OrderService {
private final UserService userService;
public OrderService(UserService userService) {
this.userService = userService;
}
}
Here, the OrderService
appears to depend solely on UserService
. However, what if UserService
itself has many more dependencies? It becomes harder to assess how well encapsulated the responsibilities of each component are.
Why This Matters
This form of dependency hiding can lead to:
- Monolithic Classes: As classes start pulling in more dependencies without clear justification, they become fat and less manageable.
- Rigidity: Making isolated changes in one class can inadvertently affect others due to tightly coupled dependencies.
4. Increased Learning Curve
While DI containers provide a lot of functionalities, their complexity can introduce a steep learning curve, especially for junior developers. Concepts like scopes, lifecycle management, and configuration can be overwhelming.
Even simple configurations can quickly spiral into a mess of annotations and XML, which can make straightforward code less approachable.
Example of Advanced Configuration
@Configuration
public class AdvancedConfig {
@Bean
@Scope("prototype")
public UserService userService(UserRepository userRepository) {
return new UserService(userRepository);
}
@Bean
@Lazy
public UserRepository userRepository() {
return new UserRepository();
}
}
Why This Matters
If new developers struggle to understand these concepts, code reviews and team collaboration may suffer, leading to lower overall code quality.
5. Difficulties in Refactoring
Refactoring is a necessary part of keeping code clean and maintainable. When dependencies are managed by a DI container, refactoring can become cumbersome. Why?
- Hidden Dependencies: When dependencies are automatically injected, it can be tough to track down where all instances are being used.
- Configuration Changes: Any refactor involving changes to the class structure might result in a cascade of changes in the DI configuration that are hard to manage.
Example of Refactoring Challenge
Say you decide to introduce a PaymentService
that depends on UserService
. Refactoring this into an existing DI container can lead to intricate and sometimes painful changes in various layers of your application.
Why This Matters
Failure to adapt to changes or confusion in how dependencies are structured can lead to bugs. Developers may also end up focusing more on the DI container rather than the application logic itself.
My Closing Thoughts on the Matter
DI containers can undoubtedly bring many advantages, including easier management of object lifecycles and improved testability. However, it is essential to be wary of their downsides.
To maintain high code quality, developers should emphasize clarity, manage complexity cautiously, and avoid design patterns that could lead to maintainability issues. Consider using manual dependency management in simpler cases or carefully architecting your DI container configurations to avoid these pitfalls.
For further reading on best practices with dependency injection, you may want to check out resources like Spring Framework Documentation and Java Dependency Injection: A Beginner's Guide.
By understanding the potential impact of DI containers on your code quality, you can make informed decisions that keep your codebase pristine and agile throughout its lifecycle. Happy coding!