Mastering JPQL Joins: Common Pitfalls and Solutions
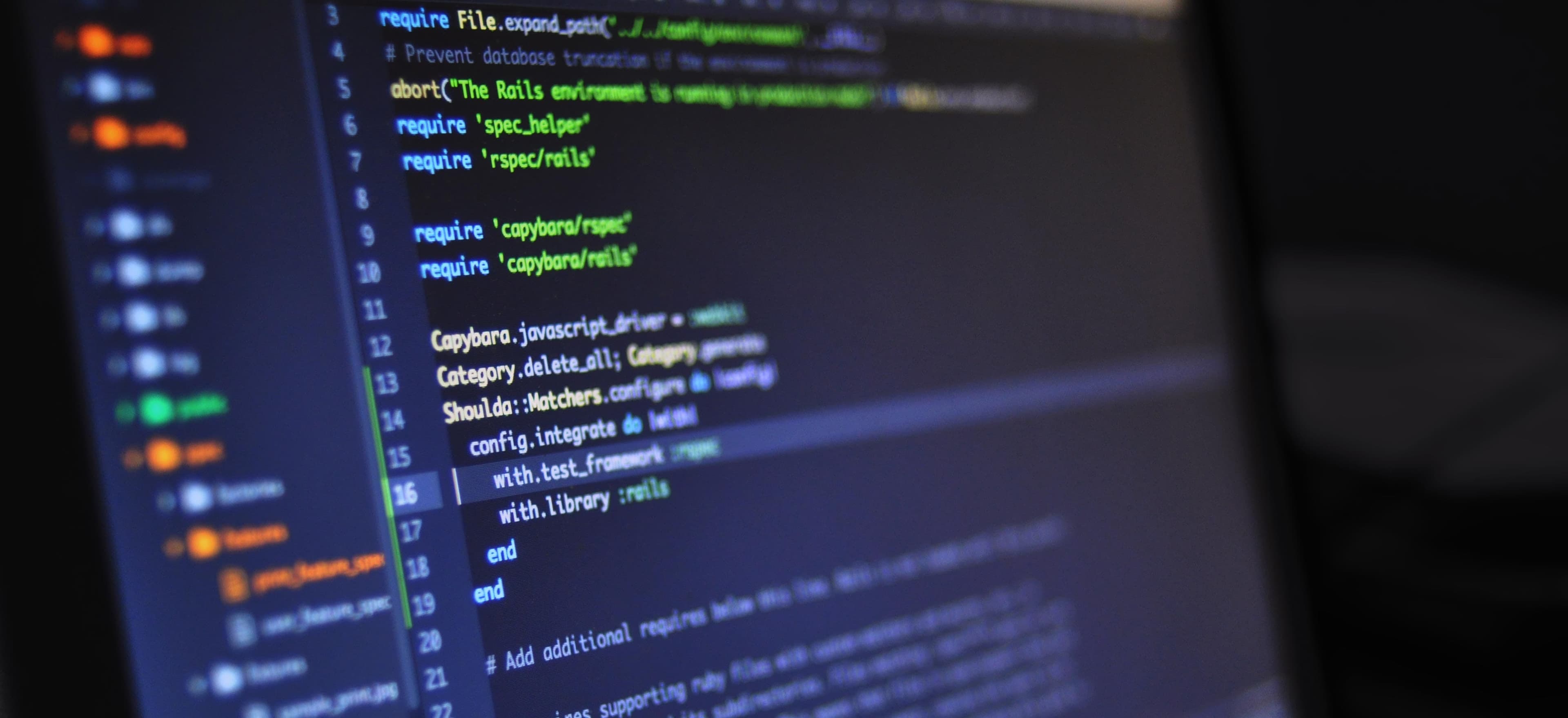
- Published on
Mastering JPQL Joins: Common Pitfalls and Solutions
Java Persistence Query Language (JPQL) provides a powerful way to apply SQL-like queries to Java entity objects. As developers dive deeper into the complexities of JPQL, particularly with joins, they often encounter common pitfalls. Understanding these pitfalls and how to navigate through them can significantly enhance your data querying effectiveness. This blog post aims to explore these challenges while providing actionable solutions to master JPQL joins.
Understanding JPQL and Joins
Before we delve into the pitfalls, it's essential to recap what JPQL is and how joins work in this context. JPQL is an object-oriented query language that operates on Java objects instead of database tables.
What is a Join in JPQL?
A join enables you to query related entities as a single result. For example, if you have an Author
entity and a related Book
entity, you can join these two entities based on their relationship.
There are three primary types of JPQL joins:
- Inner Join: Retrieves records that have matching values in both entities.
- Left (Outer) Join: Retrieves all records from the left entity and matching records from the right entity.
- Right (Outer) Join: Retrieves all records from the right entity and matching records from the left one.
Basic Join Syntax
Here is a quick look at the syntax used for a join in JPQL:
SELECT a
FROM Author a JOIN a.books b
WHERE b.title = :title
In this example, we are selecting Author
entities that have books with a specific title.
Common Pitfalls in JPQL Joins
Let's dive into some common pitfalls that developers encounter when using JPQL joins, along with practical solutions.
Pitfall 1: Confusing JOIN with INNER JOIN
Many developers, especially those transitioning from SQL, often confuse JOIN
with INNER JOIN
. In JPQL, just using JOIN
is synonymous with INNER JOIN
. This can lead to misunderstandings when dealing with left or right outer joins.
Solution
Always be clear about which join type you need. Use the specific keywords to avoid ambiguity:
- For inner joins, just use
JOIN
- For outer joins, explicitly specify
LEFT JOIN
orRIGHT JOIN
Pitfall 2: Implicit Joins and Result Size
With implicit joins, it may seem that you get more records at first glance. However, the result set may include duplicates if you don't manage your selections properly.
Solution
Use DISTINCT
to avoid duplicate results when fetching entity records.
SELECT DISTINCT a
FROM Author a JOIN a.books b
WHERE b.title = :title
Pitfall 3: Forgetting to Specify Fetch Type
Another common issue is overlooking the fetch type when defining relationships in your entity classes. Failing to do so may result in suboptimal data retrieval, causing performance overhead.
Solution
Define fetch types in your entity relationships thoughtfully. Consider using FetchType.LAZY
and FetchType.EAGER
appropriately.
Example Entity Definition
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(mappedBy = "author", fetch = FetchType.LAZY)
private List<Book> books;
// getters and setters
}
By setting FetchType.LAZY
, you delay the loading of the associated Book
records until they are specifically requested.
Pitfall 4: Misunderstanding Joins on Inheritance
If you're using inheritance, you may think you can join on a subclass. However, JPQL joins work only by entity type, which sometimes leads to incomplete data retrieval.
Solution
Be aware of the type hierarchy when crafting your queries. If you need data from subclasses, explicitly specify the type:
SELECT b
FROM Book b JOIN b.author a
WHERE TYPE(a) = FictionAuthor
Pitfall 5: Incorrect Alias Usage
Using aliases incorrectly can lead to confusing and erroneous queries. Mismanagement of aliases commonly results in runtime exceptions or incorrect results.
Solution
Always ensure your aliases are declared and utilized properly within your queries. Keep them concise and distinct to avoid ambiguity.
SELECT a
FROM Author a JOIN a.books b
WHERE a.name = :name
Pitfall 6: Failing to Utilize Named Parameters
Hardcoded values in your JPQL can make your queries less maintainable and more vulnerable to SQL injection risks.
Solution
Utilize named parameters for maintainable queries.
SELECT a
FROM Author a JOIN a.books b
WHERE b.title = :bookTitle
Remember to set the parameters on your Query
object:
Query query = entityManager.createQuery("SELECT a FROM Author a JOIN a.books b WHERE b.title = :bookTitle");
query.setParameter("bookTitle", "Effective Java");
To Wrap Things Up
Mastering JPQL joins involves not just understanding their syntax but also being aware of the common pitfalls. As demonstrated, the right knowledge around join types, fetching strategies, alias usage, and parameterization can greatly enhance your effectiveness in querying related data.
As a Java developer, staying informed about best practices in JPQL will not only boost your current project performance but will also pave the way for greater scalability and maintainability in future applications.
To learn more about JPQL and to deepen your understanding of Java Persistence API (JPA), check out the Official JPA Documentation.
Final Code Example
Here’s a cohesive example utilizing the concepts discussed in this post:
public List<Author> findAuthorsByBookTitle(EntityManager entityManager, String title) {
Query query = entityManager.createQuery("SELECT DISTINCT a "
+ "FROM Author a JOIN a.books b "
+ "WHERE b.title = :bookTitle");
query.setParameter("bookTitle", title);
return query.getResultList();
}
This method retrieves distinct authors based on book titles while efficiently managing the query parameters.
By following these guidelines and solutions, you can effectively harness the power of JPQL joins while avoiding common development pitfalls. Happy querying!
Checkout our other articles