Overcoming Spring Retry Integration Challenges in Your Project
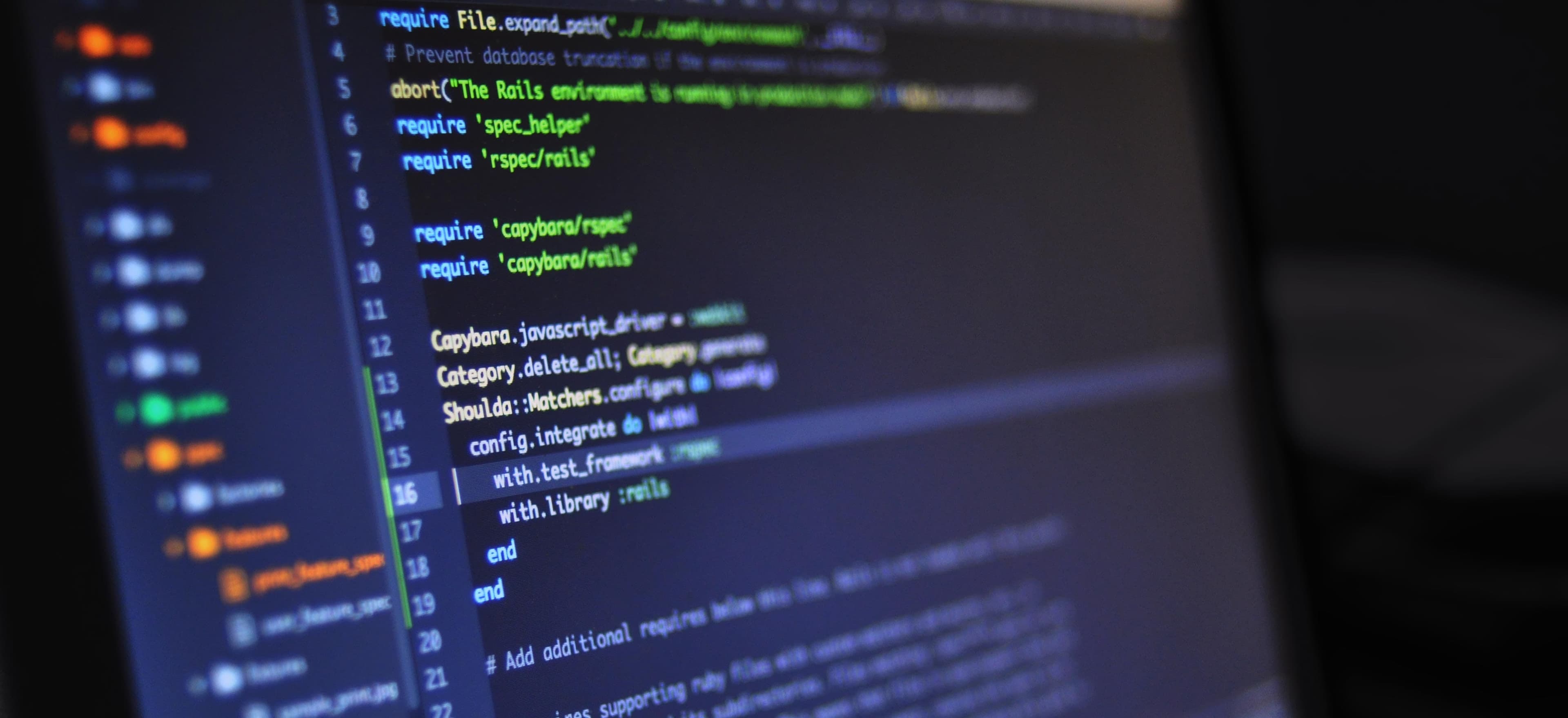
- Published on
Overcoming Spring Retry Integration Challenges in Your Project
In today's cloud-native applications, resilience is paramount. Network failures and temporary glitches are no longer mere inconveniences—which is where Spring Retry comes into play. This powerful framework allows developers to gracefully handle retryable operations, ensuring that your application remains robust in the face of adversity. However, integrating Spring Retry effectively can pose challenges.
In this post, we'll discuss common integration issues with Spring Retry, present practical solutions, and demonstrate how to implement Spring Retry in your projects effectively.
What is Spring Retry?
Spring Retry is a module from the Spring Framework that facilitates automatic retries for operations that may fail occasionally. This is especially useful for services that might experience short-lived failures, such as remote APIs or database calls.
Key Features
- Customizable: You can set up backoff strategies, limit the number of retries, and even differentiate between different types of exceptions.
- Aspect-Oriented: You can apply retries either programmatically or through annotations.
- Flexible: It works seamlessly with Spring's core features and integrates well with other Spring projects.
Common Integration Challenges
1. Understanding Configuration Options
With several configuration choices available, such as annotations, XML, or Java configuration, determining the best approach for your project can be challenging.
2. Exception Handling
Not all exceptions should trigger a retry. Establishing rules for which exceptions to catch can significantly impact application behavior.
3. State Management
If you're dealing with stateful operations (like transactions), retries can create complications. You need to ensure operations can be retried without adverse effects.
4. Backoff Strategies
Choosing the right backoff strategy is crucial. Techniques like exponential backoff might be fitting in some circumstances, while fixed delays could work better in others.
Setting Up Spring Retry
Let's explore how to implement Spring Retry in a realistic scenario. First, ensure your Maven project includes the necessary dependency:
<dependency>
<groupId>org.springframework.retry</groupId>
<artifactId>spring-retry</artifactId>
<version>latest-version</version>
</dependency>
Basic Configuration
For basic usage, you can annotate your service methods with @Retryable
, specifying the exceptions to handle:
import org.springframework.retry.annotation.Backoff;
import org.springframework.retry.annotation.EnableRetry;
import org.springframework.retry.annotation.Retryable;
import org.springframework.stereotype.Service;
@Service
@EnableRetry
public class RemoteService {
@Retryable(value = { RemoteServiceException.class }, maxAttempts = 3, backoff = @Backoff(delay = 2000))
public String callExternalService() {
// Simulate a call to an external service
// This method will be retried if it throws RemoteServiceException
return "Success!";
}
}
Commentary on the Code
- @EnableRetry: This annotation enables the Spring Retry capabilities within your class.
- @Retryable: It specifies the exceptions the operation should retry on, the maximum attempts, and the backoff delay.
- Why It Works: By configuring retries, you create a resilient method capable of handling transient issues, enhancing your application’s reliability.
Exception Handling
One of the significant challenges is deciding which exceptions should trigger a retry. Some exceptions indicate genuine failure, while others may not.
To refine your retry logic, use a combination of @Retryable
and defined exception classes. For example:
@Retryable(value = {TransientException.class}, include = { SpecificTransientException.class })
public String getData() {
// Implementation that may throw TransientException
}
With this setup, you ensure that specific exceptions lead to retries, keeping the process efficient and targeted.
Managing Stateful Operations
State management becomes critical if your operations involve transactions or external state persistence. If a retry occurs after a state change, you might end up with unintended consequences.
To address this, consider using the transactional context provided by Spring.
Example:
import org.springframework.transaction.annotation.Transactional;
// Marking the method to be transactional
@Transactional
@Retryable(value = { DatabaseTransientException.class })
public void updateData(Data data) {
// update database logic
}
Why Use Transactions?
By wrapping the operation in a transaction, you ensure that if a retry occurs, it does so within a consistent and reliable state, protecting against partial updates.
Backoff Strategies
Choosing the right backoff strategy can directly influence application behavior, especially under heavy load. Spring Retry provides a way to customize this.
Exponential Backoff Example
For instance, you might want exponential backoff for network calls:
@Retryable(value = { NetworkException.class },
backoff = @Backoff(delay = 500, multiplier = 2))
public String fetchData() {
// Simulated network fetch
return "Data";
}
Here, the delay starts at 500 milliseconds and doubles with each failed attempt. This approach mitigates overwhelming a failing system.
Integrating with Spring Boot
Spring Boot simplifies the setup process with a default Microservices architecture. Simply add the dependency and enable retry in your main application class:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableRetry
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Why Spring Boot?
Leveraging Spring Boot’s autoconfiguration makes it easier to develop microservices with resilience seamlessly integrated.
My Closing Thoughts on the Matter
Integrating Spring Retry presents several challenges, from understanding configurations to managing state and choosing backoff strategies. However, through proper setup and best practices, you can elevate your application’s robustness.
For further reading, check out the official Spring Retry Documentation and delve deeper into using Spring AOP with Retry.
Remember, the goal of resilience is not just to make things work but to enhance user experience—even in the face of failures. By integrating Spring Retry effectively, you ensure your applications are better prepared for the unpredictability of modern networks and user demands. Happy coding!