Why Java's Synchronized Might Be Your Best Bet Again
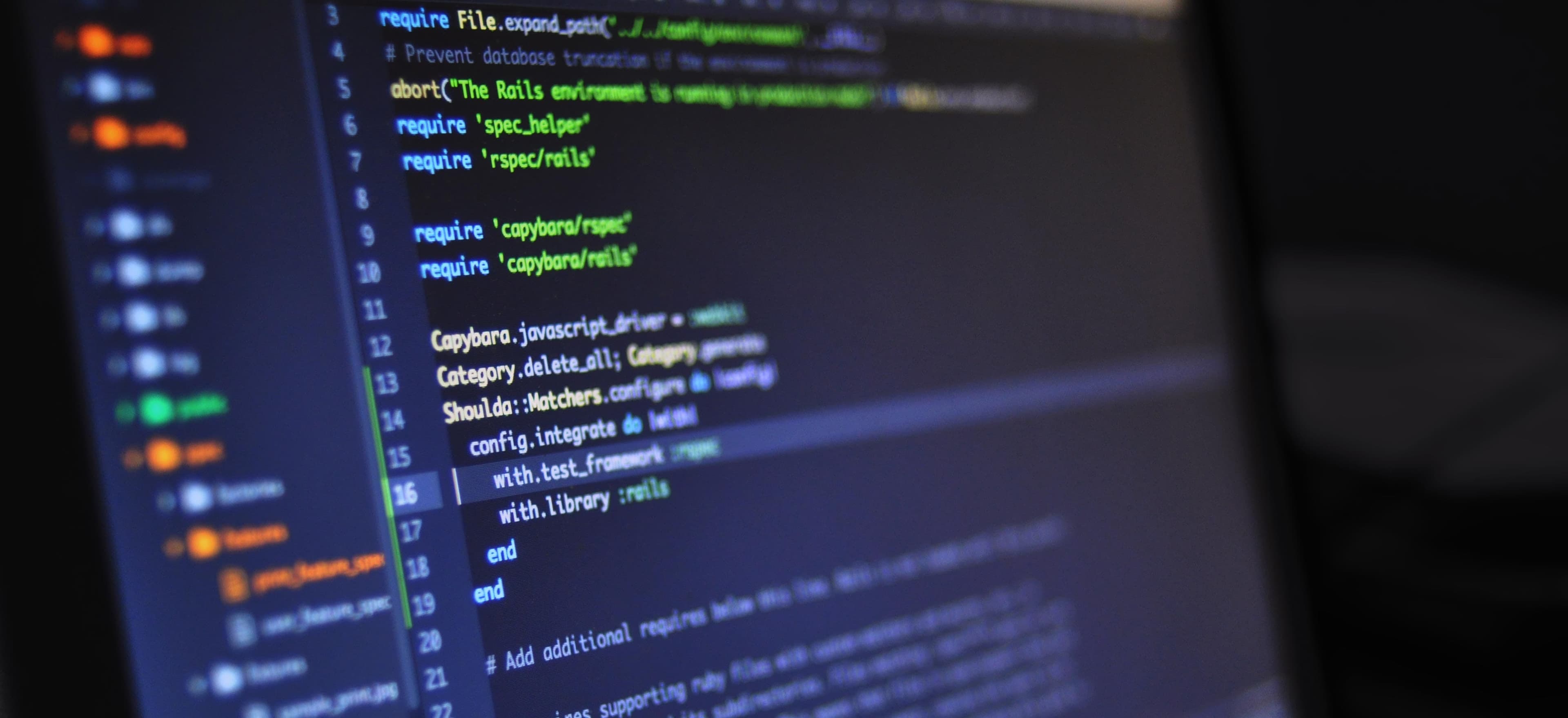
- Published on
Why Java's Synchronized Might Be Your Best Bet Again
In the world of concurrent programming, Java has long provided a robust framework for managing multiple threads. While recent developments have led to an array of sophisticated concurrency constructs, the classic synchronized
keyword remains a potent tool. In this post, we will explore why synchronized
might still be your best bet when tackling concurrency in Java.
Understanding the Basics of Synchronization
Before diving deep, let's clarify what synchronization in Java entails. Concurrency allows multiple threads to execute simultaneously, which can greatly enhance performance. However, it can also lead to issues such as race conditions, deadlocks, and inconsistent data states.
The synchronized
keyword provides a mechanism to ensure that only one thread can access a block of code or object at a time, thus preventing concurrency-related issues.
How to Use synchronized
Here's a basic example of how you can use synchronized
in Java:
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In the above code, the increment
and getCount
methods are marked as synchronized
. This means that if one thread is executing either of these methods, other threads attempting to execute them will be blocked until the first thread completes its operation.
Why Use synchronized
?
-
Simplicity: The
synchronized
keyword is easy to understand and implement. If you are new to Java or concurrent programming, masteringsynchronized
is a great starting point. -
Intrinsic Locking: It automatically provides lock acquisition and release, better than manual lock handling which can easily lead to bugs.
-
Visibility Guarantees: The Java Memory Model ensures that changes made by one thread are visible to others once it releases the lock. This means you can avoid stale data without complex mechanisms.
-
Less Overhead: Compared to some advanced constructs such as
ReentrantLock
,synchronized
can have lower overhead for simple scenarios.
Trade-offs of synchronized
While elegant, synchronized
comes with its own set of challenges and trade-offs:
-
Performance: In high-contention environments, where many threads are competing for the same lock, performance can degrade. Threads may spend time blocked on each other.
-
Less Fine-Grained Control: Advanced locking mechanisms like
ReentrantLock
allow more granular control over locking behaviors (such as timeout and interruptibility).
When to Use synchronized
Despite its limitations, here are scenarios when opting for synchronized
is ideal:
1. Basic Thread Safety
When straightforward thread safety is required without complex locking strategies, synchronized
serves as a reliable solution.
public class SharedResource {
private int value;
public synchronized void updateValue(int newValue) {
value = newValue;
}
public synchronized int readValue() {
return value;
}
}
2. Simple Critical Sections
If your critical section is small and straightforward, the added complexity of more advanced constructs may not be necessary.
3. Cost-Effective for Low Contention
In use-cases with low contention, the performance penalty of using synchronized
can be negligible compared to the benefits it provides.
Best Practices for Using synchronized
To make the most out of synchronized
, consider these best practices:
1. Keep Synchronized Blocks Short
The shorter your synchronized sections, the less time the locks are held, allowing other threads to work efficiently.
public void task() {
// Do non-critical work here
synchronized (this) {
// Only critical work interacts with shared resources here
}
// Continue doing non-critical work
}
This approach minimizes the time spent in locked state, reducing contention for the lock.
2. Prefer Method-Level Synchronization
In many cases, synchronizing the entire method is easier to maintain and understand than using synchronized blocks. Use this judiciously to prevent unnecessary locking.
3. Avoid Nested Synchronization
Don't synchronize on multiple locks if possible. This can lead to deadlocks. Stick to a single lock per synchronized block or method whenever you can.
Revisiting Java Concurrency with Java 17+
In light of the advancements in Java, you might wonder if the introduction of newer features like CompletableFuture
or the java.util.concurrent
package diminishes the role of synchronized
.
The truth is that while those tools are indispensable for certain concurrent programming paradigms, the simplicity and reliability of synchronized
cannot be overlooked. Newer libraries are built on foundations of basic concurrency concepts, including synchronized
.
The use of CompletableFuture
, for instance, can be combined with synchronized methods for a more efficient execution model, especially when it comes to managing shared state.
Closing Remarks
In a landscape dominated by evolving technologies and methodologies, Java's synchronized
keyword remains a cornerstone of thread safety in concurrent environments. Its simplicity, effectiveness, and established track record mean it won't be replaced anytime soon.
While you might have advanced alternatives at your disposal, never underestimate the power of this classic tool. In certain scenarios, synchronized
could indeed be your best bet.
Further Reading
- Java Concurrency in Practice by Brian Goetz
- Java Documentation: Synchronization
By keeping these insights in mind, you can effectively leverage Java's synchronization capabilities and write more robust multithreaded applications.