Common Session Management Mistakes in Spring MVC
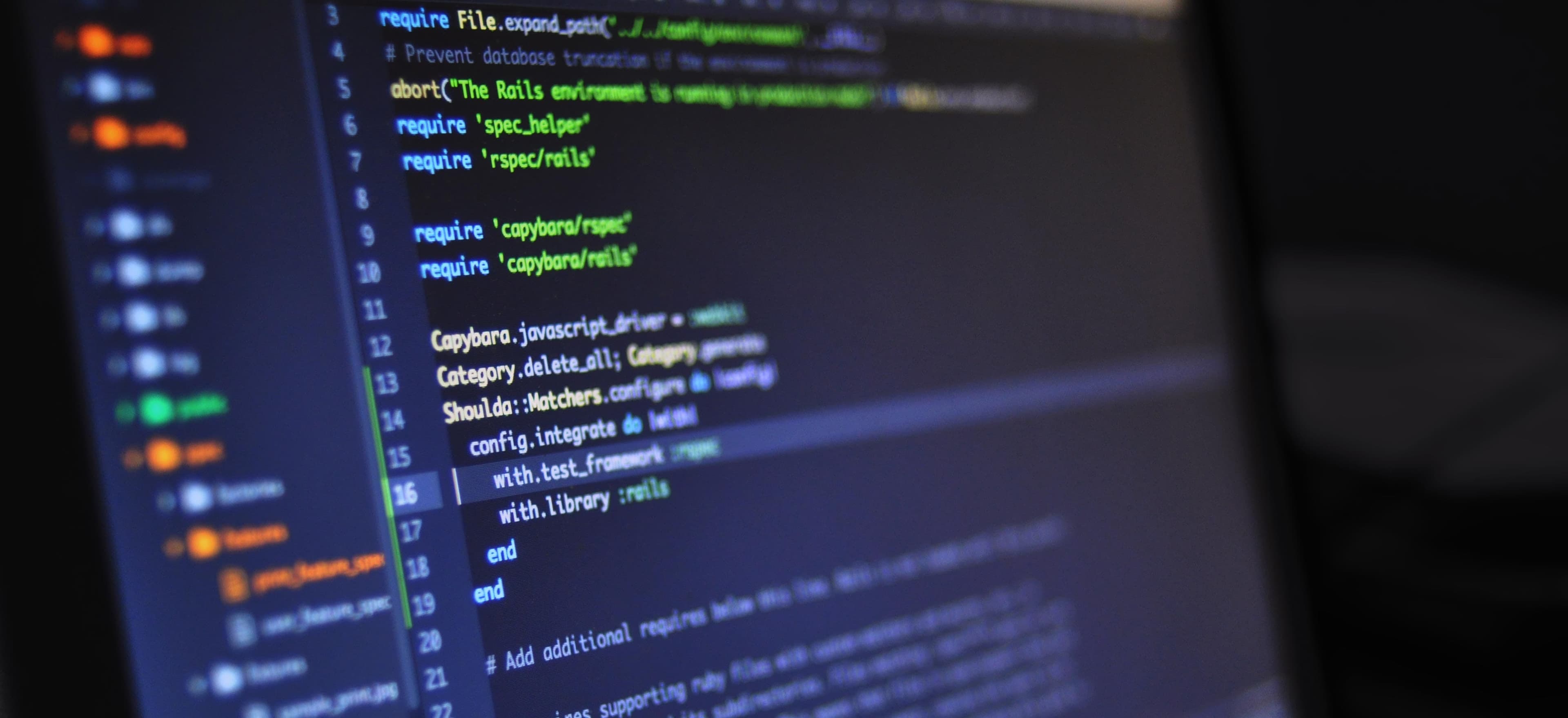
- Published on
Common Session Management Mistakes in Spring MVC
Session management is a critical component of web application architecture. With Spring MVC widely adopted for building robust applications, understanding how to manage user sessions properly is essential. This blog post will address common session management mistakes in Spring MVC and how to avoid them.
Table of Contents
- Understanding Sessions in Spring MVC
- Common Mistakes
- Best Practices for Session Management
- Conclusion
Understanding Sessions in Spring MVC
In Spring MVC, sessions are a mechanism for maintaining state across multiple requests from the same user. When a user logs into a web application, a session is created. Attributes can be stored in the session, facilitating quicker access to user data and application state.
Using Java, we can manage sessions effectively. Consider a simple example:
@Controller
public class UserController {
@GetMapping("/login")
public String login(HttpSession session) {
// Logic for user authentication goes here
// Storing user info in session
session.setAttribute("user", authenticatedUser);
return "home";
}
}
In this snippet, we demonstrate how to store user data in a session after a successful login, allowing subsequent requests to reference this data.
Common Mistakes
Now that we have established what sessions are and their importance, let’s delve into some common mistakes developers make during session management in Spring MVC.
1. Not Using HTTPS
Not securing your application through HTTPS can expose session data to attackers. An unencrypted connection allows data, including sensitive session identifiers, to be intercepted. Always enforce HTTPS in your applications.
To implement HTTPS:
- Obtain a valid SSL certificate.
- Redirect traffic from HTTP to HTTPS.
2. Storing Sensitive Data
Developers may be tempted to store sensitive user information, like passwords or personal identification numbers, in session attributes. This practice poses a significant security risk.
Instead, only store non-sensitive identifiers:
session.setAttribute("userId", authenticatedUser.getId());
Only ever store references or IDs in sessions, not sensitive data. This helps mitigate risks if a session is hijacked.
3. Session Fixation Attacks
Session fixation attacks occur when an attacker sets a user's session ID to one known to them. If the user then logs in, the attacker can use the known ID to impersonate the user.
To combat this issue:
- Always regenerate the session ID upon authentication.
Here’s a code snippet demonstrating session ID regeneration:
@RequestMapping("/login")
public String login(HttpSession session) {
// After successful authentication
session.invalidate(); // Invalidate current session
session = request.getSession(true); // Create new session
return "redirect:/home";
}
4. Ignoring Session Timeout
Another mistake is neglecting to define proper session timeout policy. Sessions that don't expire can lead to unauthorized use if a user forgets to log out.
To set session timeout in application.properties
:
spring.session.timeout=30m
This configuration sets the session timeout to 30 minutes. Make sure users are informed of the timeout duration to enhance user experience.
5. Poor Session Expiry Management
Handling expiration and inactivity times properly is crucial. Without a clear strategy, users may encounter issues while using the application. A session should expire after a specified period of inactivity, aiding in security and resource management.
Implement a listener for session expiration:
@Component
public class SessionListener implements HttpSessionListener {
@Override
public void sessionCreated(HttpSessionEvent se) {
// Logic when session is created
}
@Override
public void sessionDestroyed(HttpSessionEvent se) {
// Logic when session expires
}
}
This listener helps monitor the lifecycle of a session.
6. Not Invalidating Sessions on Logout
One of the fundamental steps in session management is properly invalidating the session when a user logs out. Failing to do so leaves the session available for potential attacks.
Ensure session invalidation during logout:
@PostMapping("/logout")
public String logout(HttpSession session) {
session.invalidate(); // Invalidate session to prevent access
return "redirect:/login";
}
Always invalidate sessions at logout to enhance security.
Best Practices for Session Management
To ensure effective session management in Spring MVC, adhere to the following best practices:
- Use HTTPS: Always use Secure connections to protect session data.
- Store Minimal Data: Only store non-sensitive user attributes.
- Regenerate Session ID: Regenerate session IDs upon authentication.
- Implement Session Timeout: Define clear timeout settings.
- Invalidate on Logout: Always invalidate sessions when the user logs out.
Consider reviewing Spring Security documentation for a more comprehensive understanding of secure session management practices.
Final Considerations
Session management is vital for developing secure and user-friendly web applications in Spring MVC. By avoiding common mistakes and following best practices, you can safeguard your application against various threats and enhance the user experience. Emphasizing security through proper session handling will not only protect your users but also maintain the integrity of your application.
By understanding the key principles outlined in this post, you are now better equipped to manage sessions effectively in your Spring MVC applications. Remember that ongoing learning and adaptation are crucial in the ever-evolving landscape of web security.