Choosing Between getResourceAsStream and FileInputStream in Java
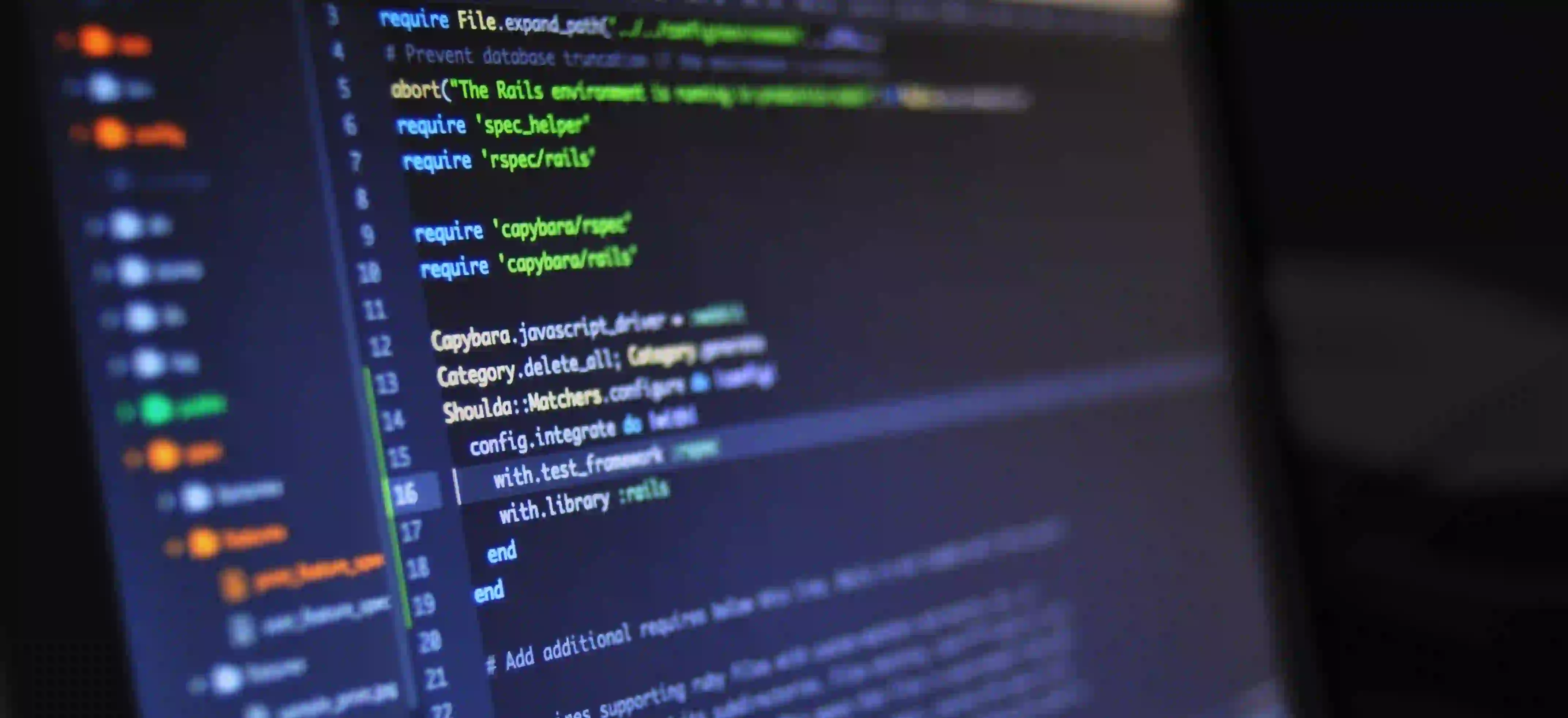
Choosing Between getResourceAsStream and FileInputStream in Java
When it comes to reading files in Java, developers often face a common dilemma: which method to use for file I/O operations? The two popular options are getResourceAsStream
and FileInputStream
. Each method has its own advantages and use cases.
In this article, we’ll dive deep into both approaches, compare them, and help you understand when to use each one. By the end, you'll be equipped with the knowledge to choose the right file reading technique for your Java applications.
Overview of FileInputStream
FileInputStream
is a part of Java's java.io
package. It is used for reading raw byte streams from a file. This is particularly useful when you need to handle binary data or when you specifically know the path to the file in the file system.
Example of FileInputStream
import java.io.FileInputStream;
import java.io.IOException;
public class FileInputStreamExample {
public static void main(String[] args) {
FileInputStream fis = null;
try {
// Create a FileInputStream by providing the absolute path
fis = new FileInputStream("path/to/your/file.txt");
int data;
while ((data = fis.read()) != -1) {
// Processing the byte data
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Commentary
In the example above, FileInputStream
opens a file at a specified path and reads it one byte at a time. While this method is straightforward, it has limitations. For instance, the file must exist in the file system, and the path must be accessible during execution.
Overview of getResourceAsStream
On the other hand, getResourceAsStream
is a method from the ClassLoader
class. It provides a way to read files from the classpath, which is handy for accessing resources packaged within JAR files or located within the application structure.
Example of getResourceAsStream
import java.io.InputStream;
import java.io.IOException;
public class GetResourceAsStreamExample {
public static void main(String[] args) {
InputStream inputStream = null;
try {
// Load the resource using the class loader
inputStream = GetResourceAsStreamExample.class.getResourceAsStream("/resources/file.txt");
if (inputStream == null) {
throw new IOException("Resource not found");
}
int data;
while ((data = inputStream.read()) != -1) {
// Processing the byte data
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (inputStream != null) {
inputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Commentary
In the code above, getResourceAsStream
accesses a file located within a resources folder in the classpath. Notice how we use the /resources/file.txt
reference to point to the file. This method abstracts away the underlying filesystem interaction, making it easier to manage resources within complex applications or JAR files.
When to Use FileInputStream
Absolute Paths
Use FileInputStream
when you need to read files from known absolute paths. This scenario is typical in applications that deal with local files which are guaranteed to exist on the filesystem.
Binary Data
If you require raw access to binary data, FileInputStream
is preferred since it allows you to handle byte streams directly.
Error Reporting
Because you have direct access to files, you can offer detailed error messages if a file cannot be found or accessed, thus improving user experience.
Example Scenario
If your application generates logs or temporary files, managing them with FileInputStream
allows you to easily read and process those files.
When to Use getResourceAsStream
Accessing Resources in JAR Files
If you're working with resources packaged within a JAR file, getResourceAsStream
is an absolute must. It simplifies loading files irrespective of their location within the jar.
Classpath Flexibility
Using getResourceAsStream
promotes portability. It decouples your code from the filesystem, making it easier to share or deploy the application in different environments without modifications.
Simplified Resource Management
When dealing with multiple environments or packaging, getResourceAsStream
allows for a cleaner and more maintainable way to reference resource files.
Example Scenario
For example, if you’re writing a configuration loader for your application, leveraging getResourceAsStream
ensures consistent access to your configuration files bundled in your application.
Comparing getResourceAsStream and FileInputStream
Let's summarize the key differences and considerations when choosing between the two options:
| Feature | FileInputStream | getResourceAsStream | |---------|----------------|---------------------| | Location | File system (absolute/relative path) | Classpath/resource | | Use case | Fast access to local files | Access bundled resources in JAR | | Portability | Dependent on filesystem structure | More portable; environment independent | | Error Handling | Detailed error messages based on path | May throw NullPointerException if not found | | Data Type | Can handle binary data | Limited to stream-based data |
A Final Look
In conclusion, choosing between getResourceAsStream
and FileInputStream
depends on the specific use case of your Java application.
- Use
FileInputStream
if you have files stored on the local file system and need straightforward access to raw byte data. - Use
getResourceAsStream
when your resources are bundled within your application (e.g., JAR files) and you want to maintain flexibility and portability.
Both methods have their own strengths. By understanding them deeply, you can make a more informed choice, benefiting your application development and maintenance processes.
For more information on file handling in Java, you can refer to the official Java I/O Documentation.
Further Reading
- Java I/O Tutorial
- Java Resources and Class Loaders
By choosing the right technique, you optimize performance and improve the overall architecture of your applications. Happy coding!