Optimizing Performance with Lucene MMapDirectory vs ByteBuffers
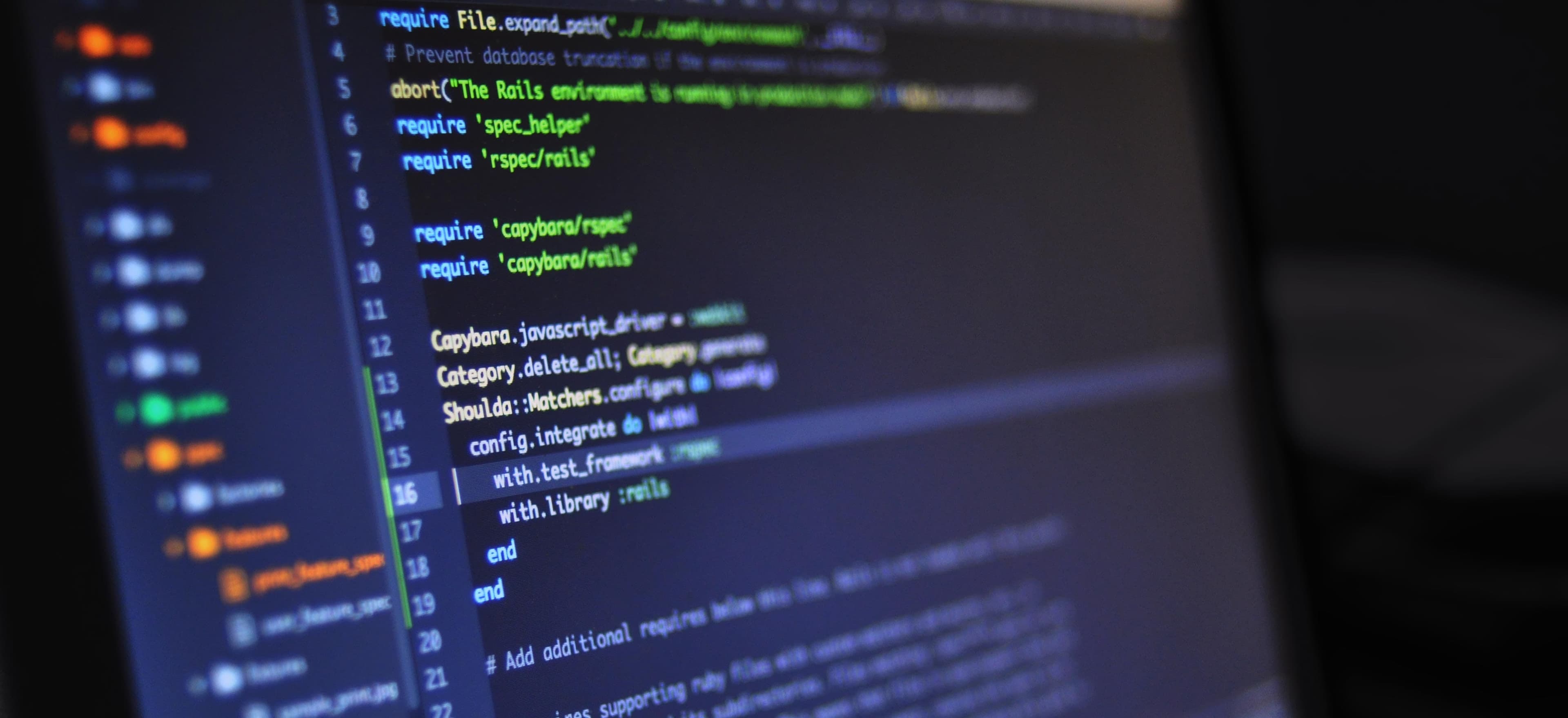
- Published on
Optimizing Performance with Lucene MMapDirectory vs ByteBuffers
In the world of information retrieval and indexing, Apache Lucene is a cornerstone, providing powerful tools for managing text-based search functionality. When it comes to performance, understanding the underlying mechanisms of how Lucene handles file storage can dramatically affect your application’s speed and efficiency. This blog post will delve into two essential components of Lucene: MMapDirectory
and ByteBuffers
. Through this exploration, we will highlight their differences, relevant scenarios, and best practices for optimizing performance.
What is Lucene?
Apache Lucene is a high-performance, full-featured text search engine library written in Java. It is used for indexing and searching text, providing powerful features such as scoring, filtering, and sorting of search results. Lucene is primarily an indexing library, which means it does not come with a user interface; rather, it provides the tools necessary to create a search engine.
Understanding MMapDirectory
MMapDirectory
is a part of Lucene's file management system that uses memory-mapped files. This mechanism allows files to be mapped into memory space, enabling faster access to data without the overhead of conventional file I/O operations.
Advantages of MMapDirectory
- Speed: Memory-mapped files provide faster access than standard file read operations because they utilize the operating system's virtual memory capabilities.
- Automatic Paging: The operating system handles paging, allowing for efficient use of memory. This means that only the necessary parts of the file are loaded into memory.
- Reduced Latency: As data is accessed directly from memory, the latency associated with disk I/O is significantly minimized.
When to Use MMapDirectory
- When working with large datasets that fit into memory.
- For applications that demand high-speed data access.
- In scenarios where read-only access to indexes is needed.
Code Example of MMapDirectory
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.MMapDirectory;
import java.nio.file.Paths;
public class LuceneMMapExample {
public static void main(String[] args) {
try {
// Creating a memory-mapped directory for the index
Directory directory = new MMapDirectory(Paths.get("indexDirectory"));
// Adding documents and setting up the index
// ...
System.out.println("MMapDirectory created successfully.");
// Properly close the directory
directory.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we initialize an MMapDirectory
pointing to the "indexDirectory". This allows Lucene to interact with the index using memory-mapped files. The performance benefits become apparent with larger indexes, where traditional file handling would introduce latency.
Exploring ByteBuffers
ByteBuffer
is part of the Java NIO package and provides an efficient way to handle binary data. In the context of Lucene, ByteBuffers
can be utilized for buffer management, enabling more controlled memory manipulation.
Advantages of ByteBuffers
- Flexible Memory Management: ByteBuffers allow for manual control of byte storage in memory, which can help optimize read and write operations.
- Direct Buffer Support: ByteBuffers support direct buffers, which can be allocated and deallocated directly in native memory, thus reducing garbage collection overhead.
- Efficient Data Processing: ByteBuffers facilitate bulk operations and can reduce the number of method calls, thereby increasing performance.
When to Use ByteBuffers
- In applications where fine-tuned memory management is critical.
- For scenarios that involve high-frequency read and write operations where performance is paramount.
- When working with complex data structures that require efficient binary representation.
Code Example of ByteBuffers
import java.nio.ByteBuffer;
public class ByteBufferExample {
public static void main(String[] args) {
// Allocating a ByteBuffer with a capacity of 64 bytes
ByteBuffer buffer = ByteBuffer.allocate(64);
// Writing data to the buffer
buffer.putInt(42);
buffer.putDouble(3.14);
// Preparing buffer for reading
buffer.flip();
// Reading data
int intValue = buffer.getInt();
double doubleValue = buffer.getDouble();
System.out.println("Integer Value: " + intValue);
System.out.println("Double Value: " + doubleValue);
}
}
In this example, a ByteBuffer
is instantiated with a capacity of 64 bytes. Data is written into the buffer, and then flipped for reading. This controlled memory access can be particularly useful when you need to manipulate binary data efficiently, especially in high-performance systems.
Comparing MMapDirectory and ByteBuffers
Now that we have explored both MMapDirectory
and ByteBuffers
, it is essential to understand how they compare in terms of usage and performance.
| Feature | MMapDirectory | ByteBuffers | |-------------------------|-------------------------------------------|-----------------------------------| | Access Speed | Fast access via memory mapping | Fast but depends on management | | Memory Efficiency | Automatically managed by the OS | Requires manual management | | Use Cases | Read-heavy applications | Write-intensive or complex operations | | Complexity | Simple to implement | Requires more coding effort |
Hybrid Usage
In many scenarios, combining both MMapDirectory
with ByteBuffers
can provide optimal performance. For instance, you can use MMapDirectory
for your indexing storage while employing ByteBuffers
for caching frequently accessed data in memory.
Bringing It All Together
Optimizing performance in Apache Lucene involves a careful analysis of your application's read and write patterns, memory management requirements, and the size of the data set. MMapDirectory
excels in read-heavy applications where speed is critical, while ByteBuffers
offer fine-tuned control for high-performance data processing.
By understanding these two components, you can significantly enhance your search engine's performance. Choosing the right approach depends on your specific use case and data characteristics.
For more information, check out the official Apache Lucene Documentation and Java NIO ByteBuffer Guide.
The key takeaway? Analyze your needs, understand the tools at your disposal, and optimize accordingly. Performance matters—make the right choices to ensure your application shines.