Overcoming Common Pitfalls in Java PriorityQueue Usage
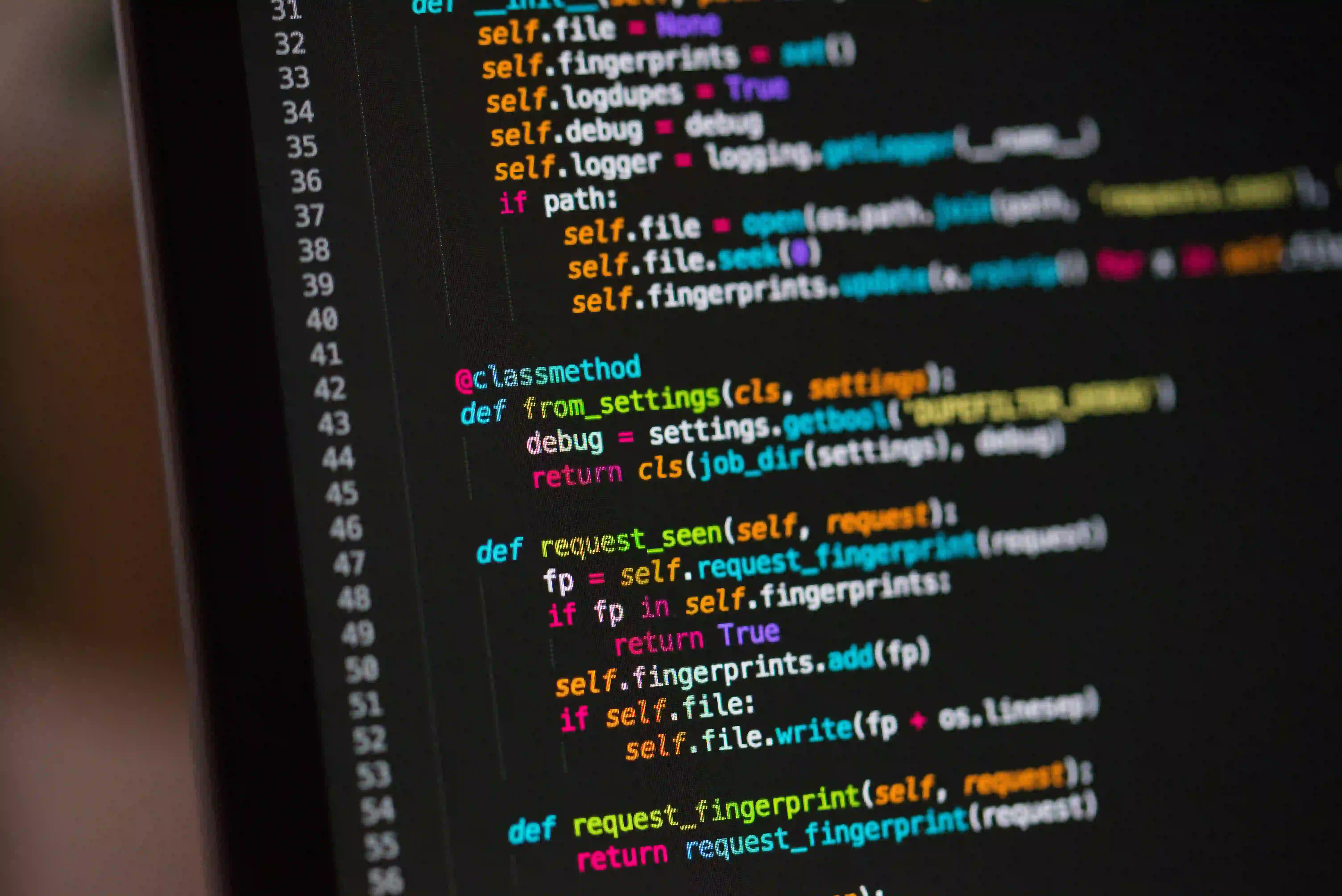
Overcoming Common Pitfalls in Java PriorityQueue Usage
Java's PriorityQueue
is an essential tool for programmers looking to manage collections of elements based on their priority. While its functionality is robust, missteps can lead to inefficiencies or unexpected behaviors. In this blog post, we’ll explore common pitfalls encountered when using PriorityQueue
in Java, discuss practical solutions, and provide illustrative code examples to cement your understanding.
What is a PriorityQueue?
Before we dive into common mistakes, let's clarify what a PriorityQueue
is. It is part of the Java Collections Framework and is implemented as a heap data structure. In a PriorityQueue
, elements are ordered according to their natural ordering or by a comparator provided at queue construction time. This makes it possible to efficiently access the highest (or lowest) priority element.
PriorityQueue<Integer> pq = new PriorityQueue<>();
pq.add(5);
pq.add(1);
pq.add(3);
System.out.println(pq.poll()); // Outputs 1 (lowest priority)
In the snippet above, 1
is returned first as it has the lowest value in the queue. Understanding this basic behavior will help guide your usage of PriorityQueue
.
Common Pitfalls and How to Overcome Them
1. Not Implementing Comparable or Comparator Correctly
One of the most common pitfalls involves failing to implement the Comparable
interface or providing a proper Comparator
. If the elements do not define a natural ordering, you must specify how elements should be ordered.
Example of Incorrect Implementation:
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
}
// Usage
PriorityQueue<Person> pq = new PriorityQueue<>();
pq.add(new Person("Alice", 30));
pq.add(new Person("Bob", 20));
Why This Fails: Without a comparator or Comparable
implementation, the PriorityQueue
does not know how to order Person
objects.
Correct Implementation:
import java.util.PriorityQueue;
import java.util.Comparator;
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
}
class AgeComparator implements Comparator<Person> {
public int compare(Person a, Person b) {
return Integer.compare(a.age, b.age);
}
}
// Usage
PriorityQueue<Person> pq = new PriorityQueue<>(new AgeComparator());
pq.add(new Person("Alice", 30));
pq.add(new Person("Bob", 20));
System.out.println(pq.poll().name); // Outputs "Bob"
In this corrected code, we define an AgeComparator
, which ensures that Person
objects are ordered by age in the priority queue.
2. Misunderstanding the Polling Order
Another frequent mistake is assuming that the poll()
method always retrieves the largest element. In a min-heap structure like PriorityQueue
by default, it retrieves the least element based on the specified ordering.
Example to Illustrate Polling:
PriorityQueue<Integer> pq = new PriorityQueue<>();
pq.add(5);
pq.add(1);
pq.add(3);
System.out.println(pq.poll()); // Outputs 1
System.out.println(pq.poll()); // Outputs 3
System.out.println(pq.poll()); // Outputs 5
Key Takeaway: Always remember that the element with the lowest priority (or highest in the case of a max heap) will be returned when calling poll()
.
3. Thread Safety Issues
The PriorityQueue
is not thread-safe, which means that if multiple threads access a PriorityQueue
instance simultaneously, and at least one of the threads modifies it, external synchronization is necessary.
Recommended Approach:
Use Collections.synchronizedList()
or consider using PriorityBlockingQueue
, which offers a thread-safe alternative.
import java.util.concurrent.PriorityBlockingQueue;
PriorityBlockingQueue<Integer> pbq = new PriorityBlockingQueue<>();
pbq.add(5);
pbq.add(1);
pbq.add(3);
System.out.println(pbq.poll()); // Outputs 1, and is thread-safe
4. Not Estimating Initial Capacity
Sometimes, programmers instantiate a PriorityQueue
without considering its initial capacity. A PriorityQueue
can expand as needed, but if you know the number of elements in advance, specifying the initial capacity can enhance performance, minimizing reallocations.
int initialCapacity = 10;
PriorityQueue<Integer> pq = new PriorityQueue<>(initialCapacity);
Best Practices for Using PriorityQueue
-
Use a Comparator When Necessary: Always define a
Comparator
for custom objects meant for aPriorityQueue
. -
Understand the Complexity: While
add()
andpoll()
operations work in O(log n) time, assessing the scale of your data can inform how to best structure your program. -
Choose the Right Queue: If you need a thread-safe implementation, avoid using
PriorityQueue
directly. Instead, considerPriorityBlockingQueue
or wrapping it with synchronization. -
Avoid Large Comparisons: If your comparison logic involves complex calculations, it can lead to performance issues. Try to keep comparisons lightweight.
-
Use Generics for Type Safety: Always parameterize your
PriorityQueue
to avoidClassCastExceptions
.
Wrapping Up
While Java's PriorityQueue
is a powerful tool, being aware of its pitfalls ensures efficient and correct usage. From implementing proper comparators to recognizing polling behavior, these insights are critical for developing robust Java applications.
For deeper insights into Java collections and concurrency, consider checking out Oracle's Java Collections Documentation and Java Concurrency in Practice.
By applying the best practices outlined in this article, you can effectively overcome common challenges and harness the full power of Java's PriorityQueue
. Happy coding!