Troubleshooting GitLab CI/CD Pipeline for Gradle Releases
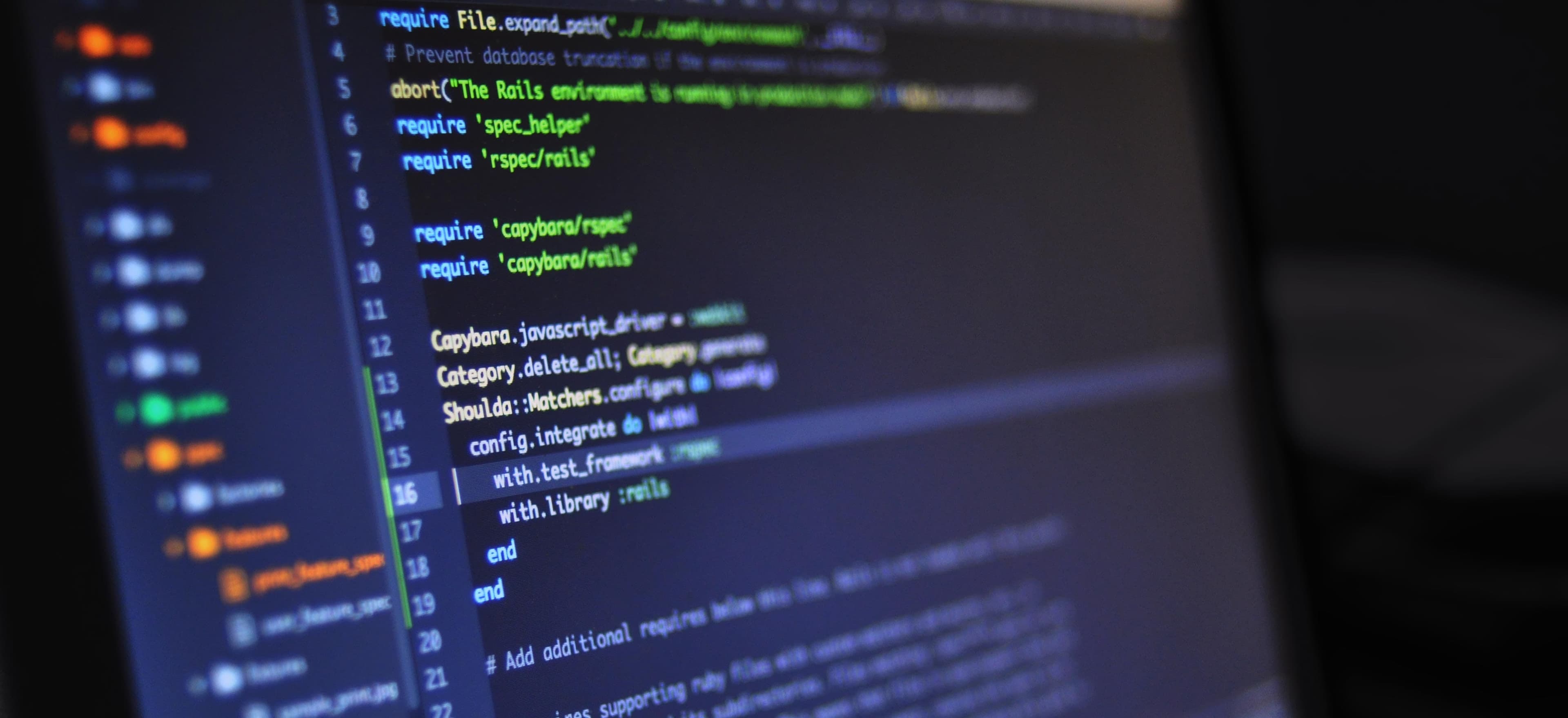
- Published on
Troubleshooting GitLab CI/CD Pipeline for Gradle Releases
In the fast-paced world of software development, continuous integration and delivery (CI/CD) are essential. GitLab offers a robust platform for implementing CI/CD, particularly for projects built using Gradle. However, developers occasionally encounter issues that can hinder deployment processes. This blog post will guide you through common problems faced in GitLab CI/CD pipelines for Gradle projects, provide troubleshooting tips, and showcase code snippets that highlight the best practices.
Understanding GitLab CI/CD for Gradle
Before diving into the troubleshooting section, let's briefly revisit GitLab CI/CD and how it integrates with Gradle. Gradle is a powerful build automation tool used for Java projects. With GitLab CI/CD, you can automate the process of testing, building, and deploying your Gradle applications.
The .gitlab-ci.yml file is central to the GitLab CI/CD configuration. Here's a simple example:
stages:
- build
- test
- deploy
build_app:
stage: build
script:
- ./gradlew build
test_app:
stage: test
script:
- ./gradlew test
deploy_app:
stage: deploy
script:
- ./gradlew deploy
only:
- master
Explanation
- Stages: Defines the pipeline's stages, including build, test, and deploy.
- Jobs: Each job corresponds to a stage and uses the
script
keyword to execute Gradle commands. - Deployment Conditions: The
only
directive ensures that deployment occurs only from themaster
branch. This prevents premature releases from unverified branches.
With this foundational understanding, let's address common issues.
Common Issues and Troubleshooting Steps
1. Gradle Wrapper Issues
Problem: The pipeline fails to recognize the Gradle wrapper or doesn't use the correct version.
Solution: Ensure you are using the Gradle wrapper in your scripts. The Gradle wrapper (gradlew
) ensures that the appropriate Gradle version is used in builds, regardless of the installation on the pipeline runner.
Check your .gitlab-ci.yml script:
script:
- chmod +x gradlew # Make sure gradlew is executable
- ./gradlew build # Use the Gradle wrapper
Why it matters: Using the Gradle wrapper prevents discrepancies between development and CI environments. Always execute builds with ./gradlew
to maintain consistency.
2. Incorrect Environment Variables
Problem: Your pipeline fails due to missing required environment variables.
Solution: Verify that all necessary environment variables are defined in your GitLab CI/CD settings. You can define these in your project settings under CI/CD -> Variables. For example, if you're using a different database than in your local environment, set the proper credentials.
Example:
variables:
DATABASE_URL: $CI_DATABASE_URL # Ensure this is set in GitLab CI/CD variables
Why it matters: Environment variables are crucial for maintaining environments that differ from your local setup, ensuring your application can connect to required services during builds.
3. Gradle Caching Issues
Problem: Slow builds or failed builds due to Gradle cache corruption.
Solution: Clear the Gradle cache in your CI pipeline to avoid issues from cached artifacts. You can add a cache clearing step:
before_script:
- ./gradlew cleanCache
Note: Ensure you have a clean task defined in your Gradle build file, or manually delete the cache directory.
Why it matters: Cached dependencies can become stale, leading to unexpected issues during builds. By clearing the cache, you force Gradle to download the latest dependencies.
4. Gradle Dependency Resolution Failures
Problem: Builds fail due to dependency resolution issues, which indicates that Gradle couldn't find one or more dependencies.
Solution: Verify your build.gradle
file for potential issues in how dependencies are declared:
dependencies {
implementation 'org.springframework:spring-core:5.3.9'
testImplementation 'junit:junit:4.13.2'
}
Ensure that the repositories you use for dependency resolution are reachable from the GitLab CI runner, and consider using a mirror repository if your default repositories are slow or unreachable.
Why it matters: Proper dependency management ensures your application has everything it needs to build without issues. Review your repositories if you encounter resolution errors.
5. Timeout Errors
Problem: Pipelines failing due to timeout issues.
Solution: Increase the timeout duration in your CI/CD settings or reconfigure your jobs to optimize build times. In your .gitlab-ci.yml
, set a custom timeout for specific jobs:
build_app:
stage: build
script:
- ./gradlew build
timeout: 30 minutes
Why it matters: Longer-running builds, especially in larger projects, may need more time to complete. Ensure that your job settings reflect the actual needs of your project.
Advanced Troubleshooting Techniques
If you're still encountering issues, here are some advanced troubleshooting techniques:
- Use
--info
or--debug
flags: In your Gradle scripts, add these flags to get more detailed output, which can help in diagnosing build issues.
script:
- ./gradlew build --info
-
Check Runner Logs: Explore the GitLab runner logs for insights into why jobs are failing. Often, they provide critical error messages.
-
Remote Debugging: Consider running your GitLab runner locally to debug pipelines interactively. This allows you to step through builds as they execute.
In Conclusion, Here is What Matters
Troubleshooting GitLab CI/CD pipelines for Gradle releases can be challenging, but understanding the common issues and employing best practices can alleviate many of these headaches. Utilizing the Gradle wrapper, managing environment variables, handling dependencies efficiently, and optimizing builds are essential strategies.
For further assistance, I recommend checking the Gradle documentation for an in-depth understanding of Gradle functionalities, and GitLab CI/CD documentation for comprehensive CI/CD best practices.
By implementing the insights from this post, you'll be better equipped to maintain smooth and successful CI/CD pipelines for your Gradle projects. Remember—the goal is to automate and simplify your deployment processes while ensuring reliability and consistency. Happy coding!