Why Premature Microservices Can Sabotage Your Project
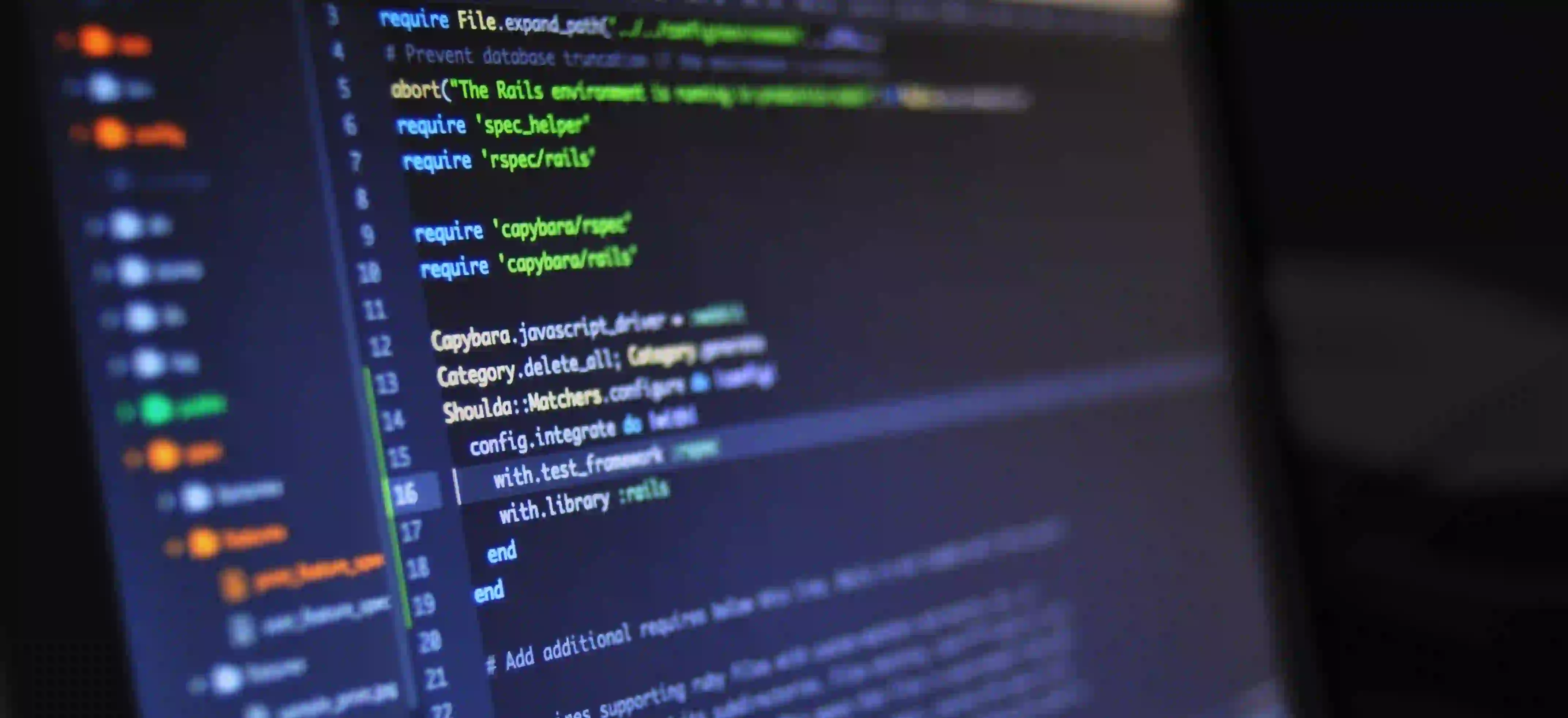
Why Premature Microservices Can Sabotage Your Project
In the world of software development, the allure of microservices is undeniable. They promise scalability, flexibility, and faster deployments. However, when premature microservices are adopted, they often introduce more problems than they solve. In this blog post, we will explore the pitfalls of adopting a microservices architecture too early in your project and provide insights on when and how to implement this architectural style effectively.
Understanding Microservices
Before diving into the pitfalls, it's essential to understand what microservices are. Microservices is an architectural style that structures an application as a collection of small, loosely coupled services. Each service represents a specific business capability and can be developed, deployed, and scaled independently.
The appeal of microservices lies in their potential to:
- Improve the speed and reliability of software delivery
- Decentralize development and allow teams to work independently
- Enhance the system’s scalability by enabling independent scaling of services
However, these advantages come with a set of challenges, especially when implemented too early in a project.
The Dangers of Premature Microservices
1. Increased Complexity
When microservices are introduced prematurely, they can lead to increased complexity in your applications. Instead of having a single codebase to manage, you're now juggling multiple services, each potentially written in different programming languages or frameworks.
This complexity can lead to higher maintenance costs and introduce new challenges such as:
- Inter-Service Communication: Understanding how services communicate with one another can become challenging. If you're using REST APIs or messaging queues, it requires additional overhead in managing those systems.
// Example Communication via REST
@RestController
@RequestMapping("/api/v1/orders")
public class OrderController {
@Autowired
private OrderService orderService;
@PostMapping
public ResponseEntity<Order> createOrder(@RequestBody Order order) {
Order createdOrder = orderService.createOrder(order);
return new ResponseEntity<>(createdOrder, HttpStatus.CREATED);
}
}
In the above Java snippet, you see an endpoint for creating an order. This simple implementation communicates over HTTP, but when multiple services exist, there's a need for sophisticated error handling and retry mechanisms which complicate the codebase.
2. Overhead in Deployment
With microservices, each service may require its own deployment pipeline. This can mean that instead of one CI/CD pipeline, your team must manage multiple pipelines, leading to significant overhead:
- Configuration: Each microservice might need different configurations according to the environment, increasing deployment complexity.
- Monitoring: Monitoring performance and logging become exponentially harder with many microservices, as they can fail independently, making it challenging to pinpoint issues.
Consider this example of a simple CI/CD pipeline configuration for a microservice:
# Sample CI/CD Configuration for a Microservice in YAML
version: '3'
services:
api:
build:
context: ./api
dockerfile: Dockerfile
ports:
- "8080:8080"
networks:
- app-network
networks:
app-network:
While this might look straightforward, imagine repeating this for dozens of services. The management and orchestration of such a deployment can quickly turn cumbersome.
3. Team Overhead
When adopting microservices prematurely, teams need to be well-aligned in their technology stacks and workflows. In the early stages, you might end up with small teams working on different services with minimal coordination, leading to:
- Inconsistent Technologies: Different teams may choose different technologies that complicate integrations.
- Communication Issues: A lack of communication can lead to misaligned objectives and wasted efforts.
4. Premature Optimization
A common pitfall in software development is premature optimization. Developers often try to optimize for scale before the application is even under heavy load. When teams start to split their application into microservices without fully understanding the domain, they risk overengineering.
5. Increased Latency
Microservices often require service-to-service calls. Each call over the network introduces latency that can accumulate quickly. This is especially pronounced when there is insufficient consideration of how frequently services interact. As illustrated in the following code snippet, a service that requires multiple calls can introduce noticeable delays:
// Pseudo-code for processing an order
public Order processOrder(Order order) {
Customer customer = customerService.getCustomer(order.getCustomerId());
Inventory inventory = inventoryService.checkInventory(order.getItemId());
if (!inventory.isAvailable()) {
throw new InventoryException("Item not available");
}
paymentService.processPayment(order.getPaymentInfo());
return orderService.completeOrder(order);
}
In this example, the processOrder
method involves multiple network calls to different services, which could lead to significant latency if not handled properly.
When to Embrace Microservices
So, when is the right time to consider microservices?
-
Business Domains are Well-defined: Ensure your project scope is well-defined. Microservices work best when there is a clear division between services based on business domains.
-
Scalability Needs: If your application is seeing substantial traffic that necessitates scaling, consider microservices.
-
Growing Teams: Microservices can be beneficial when your teams are big enough to warrant working independently and parallelly on features.
-
Continuous Integration: If you have well-established CI/CD practices in place, the transition to microservices will be smoother.
-
Legacy System Dependencies: If you're facing challenges with a monolith and want to start isolating functionalities into services to avoid continuous integration issues, it may be worthwhile to incrementally migrate.
Wrapping Up
Microservices are a powerful tool but are not always the right fit for every project right out of the gate. Understanding the ramifications of adopting microservices too early can help you avoid significant pitfalls. Take time to evaluate your needs, assess your project complexity, and ensure that your team is ready for this ambitious architectural journey.
If you're interested in diving deeper into microservices, consider reading Martin Fowler's article on Microservices for a comprehensive overview of the architecture.
Embarking on the microservices journey is exciting, but like any architectural decision, it must be made with careful consideration. Remember, premature microservices can indeed sabotage your project—so be strategic, be deliberate, and ensure you're ready before taking the plunge.